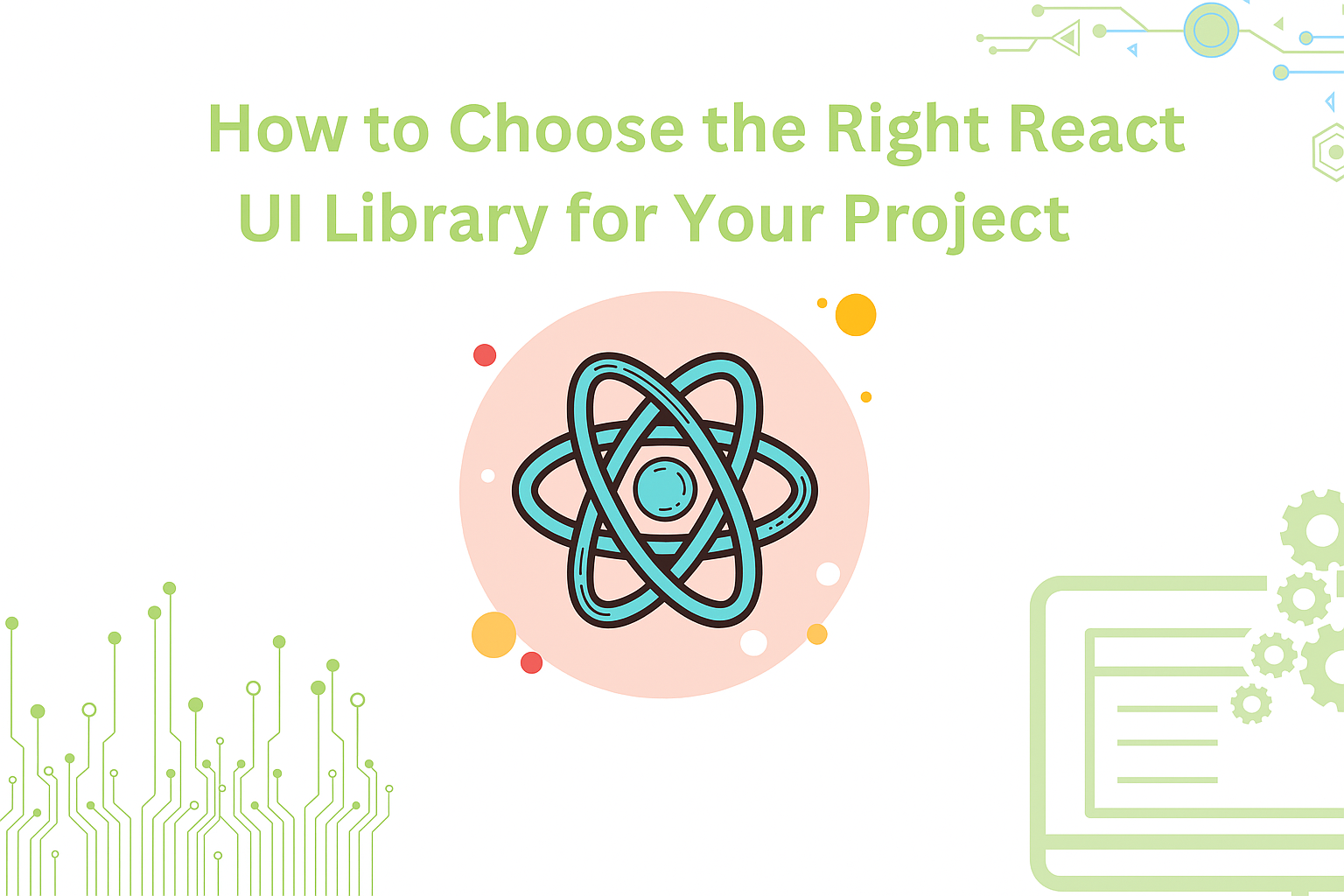
React is perhaps the most widely used web app-building framework right now. Many developers also pair it with Tailwind CSS for fast styling. It's quick, versatile & supported by a huge community. But for creating real-world applications, this alone isn't…
Subscribe to our newsletter
Be the first to learn about new Sencha resources and tips.