UI Components: They Are Not As Difficult As You Think
As a web application developer, you need a deep understanding of UI components. You also need to have a clear idea about how users interact with them. This will help you develop user-friendly web applications. There are numerous JavaScript libraries and frameworks that can help you build an attractive user interface. Which, however, is the best one? Does it enable you to easily create a beautiful interface for your app? In this post, you will find all the details.
What are UI components?
UI components are the most integral parts of a web application. They consist of all the app’s styles and components, such as buttons, input fields, icons, and text. Essentially, they are the core building blocks for all applications.
UI components are responsible for interactions between the user and the application. They facilitate an effective user experience, and they allow the users to navigate through the application easily. UI components also help them to efficiently perform various functions, like adding/editing information, filtering data, and selecting a date on the calendar.
Why should you use UI components?
- Quickly create the user interface of your web application
- Reduce code duplication by using reusable components
- Save development time and money
- Easily update the UI when required
How can I easily create UI components for my JavaScript application?
You can easily create UI components for your JavaScript web application using Sencha Ext JS. It is the most comprehensive framework for building data-intensive apps for all modern devices.
The UI of Ext JS applications is made up of widgets. They are known as components, and they are subclasses of the Ext.Component class. You can easily extend them to create a customized component for your web application.
Also Read: 10 Best React UI Component Libraries in 2025
How can Sencha Ext JS help me in quickly creating UI components?
Sencha Ext JS allows you to reuse code. As a result, you can quickly create UI components. In addition, you don’t need to do a lot of coding. You can create UI components with just a few lines of code. Let’s take a look at some practical examples.
How can I quickly create a calendar for scheduling events?
The Ext JS calendar is a powerful UI component for creating custom calendars. It allows you to use the date and event data, just like Google Calendar. Here is an example:
With the calendar, you can easily schedule your work and personal events. It has a minimalistic design. To create it, you have to follow these steps:
1. First, you need to define a new custom class, called KitchenSink.view.calendar.Month. Then you have to extend Ext.panel.Panel. You also need to define the type of UI component, which is calendar-month-view.
Ext.define('KitchenSink.view.calendar.Month', { extend: 'Ext.panel.Panel', xtype: 'calendar-month-view',
2. Then you have to add this code:
requires: [ 'KitchenSink.data.calendar.Month', 'Ext.calendar.panel.Month', 'Ext.calendar.List' ], width: 1000, height: 600,
3. Next, you have to create the viewModel.
viewModel: { data: { value: Ext.Date.getFirstDateOfMonth(new Date()) }, stores: { calStore: { type: 'calendar-calendars', autoLoad: true, proxy: { type: 'ajax', url: '/KitchenSink/CalendarMonth' } } } },
4. Now, you have to specify the layout, bind, and titleAlign fields.
layout: 'border', bind: { title: '{value:date("M Y")}' }, titleAlign: 'center',
5. Finally, you have to specify the items field.
items: [{ region: 'west', title: 'Calendars', ui: 'light', width: 150, bodyPadding: 5, collapsible: true, items: { xtype: 'calendar-list', bind: '{calStore}' } }, { region: 'center', xtype: 'calendar-month', visibleWeeks: null, timezoneOffset: 0, gestureNavigation: false, bind: { value: '{value}', store: '{calStore}' } }] })
Source Code:
You can find the source code right here.
How can I quickly build a login form?
Sencha Ext JS includes the form panel, a basic UI component with form handling capabilities. You can use it whenever you need to collect data from the user. Using it, you can quickly build a login form. Here is an example:
This is a simple login form that accepts the user ID and password. To create it, you have to follow these simple steps:
1. First, you have to define a new custom class, called KitchenSink.view.form.LoginForm. Then you have to extend Ext.form.Panel. You also need to define the type of UI component, which is form-login.
Ext.define('KitchenSink.view.form.LoginForm', { extend: 'Ext.form.Panel', xtype: 'form-login',
2. Next, you have to specify title, frame, width, bodyPadding, and defaultType fields.
title: 'Login', frame:true, width: 320, bodyPadding: 10, defaultType: 'textfield',
3. Then you have to specify the items for “user ID”, “password”, and “Remember me” fields of the form.
items: [{ allowBlank: false, fieldLabel: 'User ID', name: 'user', emptyText: 'user id' }, { allowBlank: false, fieldLabel: 'Password', name: 'pass', emptyText: 'password', inputType: 'password' }, { xtype:'checkbox', fieldLabel: 'Remember me', name: 'remember' }],
4. Now, you have to specify the Register and Login buttons.
buttons: [ { text:'Register' }, { text:'Login' } ],
5. Finally, you have to specify the defaults.
defaults: { anchor: '100%', labelWidth: 120 } });
Source Code:
You can view the source code right here.
How can I effortlessly create a carousel?
Carousels enable you to effortlessly swipe through multiple pages, providing several advantages. For example, it allows you to browse through different products easily and quickly find the item you are looking for.
Sencha Ext JS allows you to place any UI component into the carousel. Here is an example:
This is a carousel with two pages. The first page shows the names on the roster. If you click on the second dot of the carousel controls, you will get into the second page, which contains a login form.
To create this carousel, you have to follow these steps:
1. First, you need to create an instance of Ext.Carousel. Then you have to set the fullScreen field to true.
Ext.create('Ext.Carousel', { fullscreen: true,
2. Next, you have to specify the items in the carousel.
items: [ { xtype: 'list', items: { xtype: 'toolbar', docked: 'top', title: 'Roster' }, store: { fields: ['name'], data: [ {name: 'Jon'}, {name: 'Sansa'}, {name: 'Arya'}, {name: 'Robb'}, {name: 'Bran'}, {name: 'Rickon'} ] }, itemTpl: '{name}' }, { xtype: 'fieldset', items: [ { xtype: 'toolbar', docked: 'top', title: 'Login' }, { xtype: 'textfield', label: 'Name' }, { xtype: 'passwordfield', label: 'Password' } ] } ] });
Source Code:
You can view the source code right here.
As you can see, the Sencha Ext JS allows you to effortlessly use UI components. It enables you to do more with just a few lines of code. As a result, you can quickly build the UI of your web application. It helps you significantly boost your productivity.
Should I rely on Sencha Ext JS for building UI components?
Sencha Ext JS is a powerful JavaScript framework. It has various UI components, which you can easily customize. You can effortlessly create a beautiful user interface for your application by using them. It doesn’t require a lot of coding, and you can get the job done with just a few lines of code. Overall, Sencha Ext JS can make your life a lot easier. You should definitely consider using it to build your web apps’ UI components.
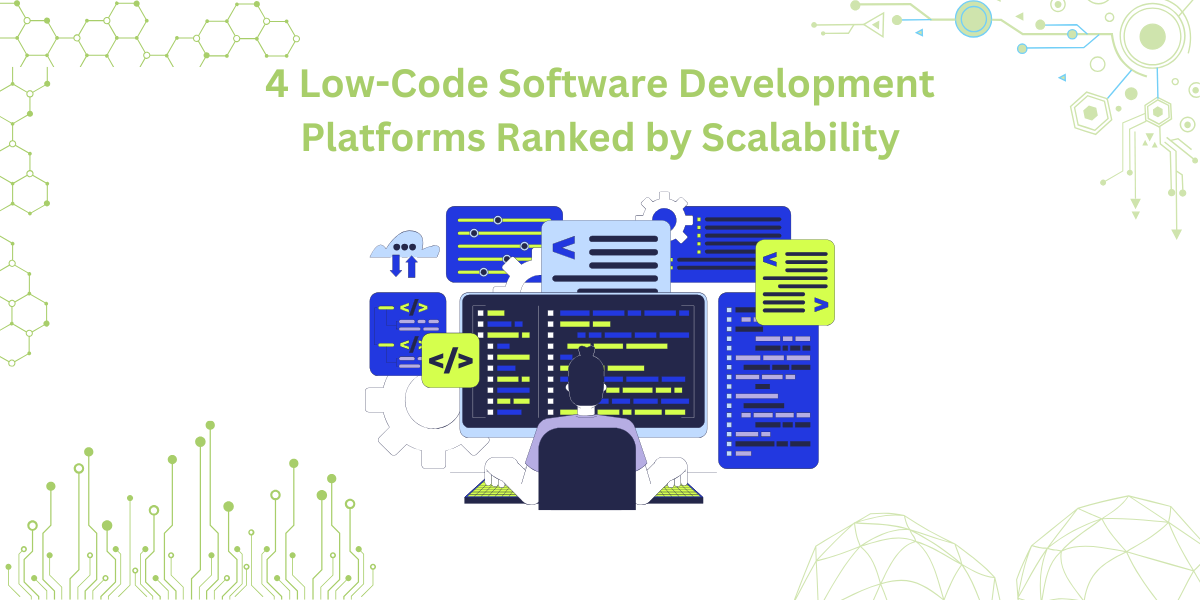
By 2026, Gartner says 80% of apps will be built using low code tools. That’s…
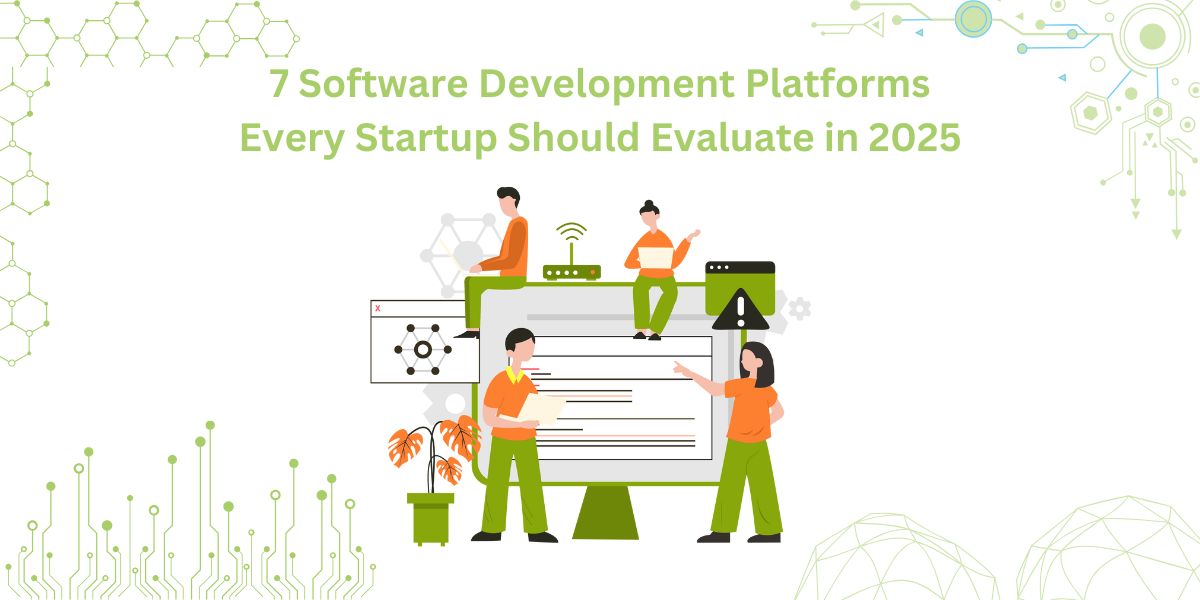
Did you know that nearly 90% of startups fail? And one of the biggest reasons…
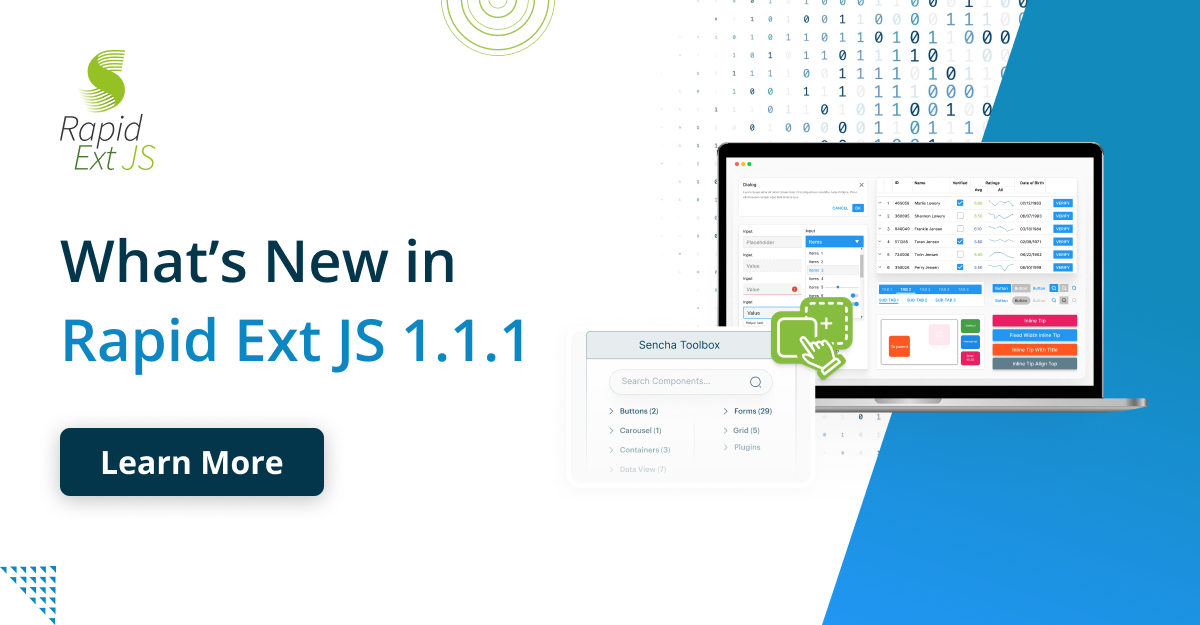
The Sencha team is excited to announce the latest Sencha Rapid Ext JS 1.1.1 release…