Building Data-Intensive Applications with ReExt
Data is everywhere right now. Seriously, humans generate about 2.5 quintillion bytes every day. It’s kind of wild when you think about it.
And if you build apps, you’ve probably felt the pressure. More data means your apps need to keep up and handle way more than they used to. From real-time transactions to complex analytics, designing data-intensive applications is no longer optional; it’s a must.
This is where things like scalability and speed really count. Whether you’re building data intensive applications for enterprise platforms or designing data-intensive application pages for high-traffic dashboards, performance needs to stay sharp.
That’s why more developers are paying attention to ReExt. It combines React and Ext JS in a way that really works when you are dealing with large amounts of data. It is not just about loading the data. What matters is keeping things fast, smooth, and making sure the interface stays solid even when the workload gets heavy.
In this blog, we’ll dig into how ReExt makes it easier to design data intensive applications from the ground up. We’ll also look at how it supports application performance monitoring tools to keep your builds running lean.
So if you’re into building apps that scale and perform, stick around, this one’s for you.
Why Use ReExt for Data-Intensive Applications?
These days, apps are all about data. Whether you’re designing data intensive applications or dashboards that update in real time, performance really matters. That’s where ReExt steps in. It blends the power of Ext JS with the flexibility of React, giving you a solid toolkit to build fast, modern UIs.
If you’re building data intensive applications that need to handle thousands or even millions of records, ReExt makes that manageable. Its grid components are built for speed. They support smooth pagination, filtering, and sorting, even with massive datasets. It’s ideal for memory-intensive applications where performance can’t lag.
And let’s talk about real-time data handling. No delays are affordable if you are working with financial apps or real-time analysis tools. ReExt supports quick data binding and smart state management, keeping things responsive even as data updates constantly.
So if you’re into designing data-intensive applications pages or just need a solid UI for a heavy-lifting app, ReExt is worth a serious look. It’s also a great match for teams focusing on building data intensive applications without sacrificing usability or speed. Throw in application performance monitoring tools and you’ve got everything covered.
Why ReExt for Modern Development?
Let’s discuss why so many developers are using ReExt lately. This thing rocks for modern apps, total game-changer. You want tools that are easy to set up, can scale as you grow, and won’t buckle under heavy data.
No fuss, just gets the job done. Perfect for real-world use. React really shines here. It has a component based structure that really helps you build small, reusable pieces. These components work together smoothly and efficiently, and also help to update in the future.
But when you’re designing data intensive applications, clean structure alone isn’t enough. You also need to handle complex data using some UI components, without slowing the system. In this scenario, Ext JS is really helpful. It has ready-to-use components like grids, charts, forms, and tree views, perfect for building memory-intensive applications. When you want your app to be responsive, these features really support it.
ReExt mixes the best of both worlds. You get React’s lightweight, reactive flow and Ext JS’s pro-grade components, all in one stack. Whether you’re building data intensive applications from scratch or revamping older ones, it just fits in easily.
Even better, ReExt supports high-performance rendering, lazy loading, and optimized updates. So when you’re dealing with memory-intensive applications, performance doesn’t take a hit. If you’re looking for memory-intensive applications examples, think of dashboards with live data, real-time analytics, or large-scale data visualizations. And with the right application performance monitoring tools, you’ll catch slowdowns before users even notice.
How ReExt Helps in Designing Data-Intensive Applications
Designing data-intensive applications isn’t just about showing a bunch of numbers. In fact, it’s about how smoothly your app handles, processes, and displays that data. ReExt really makes this part easier by giving you ready-to-use tools that just work.
Take a reporting dashboard, for example. If you’re pulling in customer, sales, and inventory info, ReExt’s grid component lets you lay it all out in a clean, interactive table. You can sort, filter, and edit right inside the grid, and even hook it up to live updates.
If you need to show trends, just drop in a few charts to give your users the bigger picture.
When your app starts dealing with heavier data, like in memory-intensive applications, ReExt keeps things light with smart loading. Instead of loading all data at once, it supports lazy loading and pagination, which helps to load only that part of the data that the user wants.
Amazingly, UI really makes life easier when dealing with data changes. It all managed automatically; you don’t need to put extra code or make any changes for this specifically. That’s a huge plus when you’re working on real-time dashboards or apps where the data’s always moving.
Integrating Ext JS with React using ReExt
You can watch this video on integrating Ext JS with React components using ReExt. Let’s explain it through code examples.
Bringing two big frameworks together might sound tricky, but ReExt makes the whole process smoother than you’d expect. ReExt acts like a connector between Ext JS and React, which really comes in clutch when you’re working on data intensive applications and want to combine the strengths of both frameworks.
To dive in, check out the official ReExt site at https://www.sencha.com/products/reext. It’s packed with docs, quick-start guides, and examples to help you get rolling without the usual hassle.
If you’re using Visual Studio Code, there’s a ReExt Designer extension that really speeds things up. It gives you a visual way to design apps by dragging and dropping components. You don’t need to write everything from scratch.
Here’s how to get the extension set up:
- Open the Extensions tab in your VS Code
- Hit the three-dot menu in the top-right corner
- Click on ‘Install from VSIX…’ and just go through the steps—it’s pretty straightforward
Once it’s installed, you are good to go. You can directly start building layouts and dropping in components, without coding everything by hand. Easy, right? Perfect for data-heavy apps or memory-intensive projects. Also great for quick prototypes when you need to move fast.
Quick Start Without VS Code Extension
If you prefer using the terminal, here’s how to set up your project quickly.
MacOS – Using Vite:
npm create vite@latest reextvite -- --template react-swc
cd reextvite
npm install @gusmano/reext@latest
cp node_modules/@gusmano/reext/dist/example/ReExtData.json src/ReExtData.json
cp node_modules/@gusmano/reext/dist/example/App.jsx src/App.jsx
cp node_modules/@gusmano/reext/dist/example/main.jsx src/main.jsx
npx vite --open
Windows – Using Vite:
npm create vite@latest reextvite -- --template react-swc
cd reextvite
npm install @gusmano/reext@latest
xcopy node_modules\@gusmano\reext\dist\example\ReExtData.json src\ReExtData.json /Y
xcopy node_modules\@gusmano\reext\dist\example\App.jsx src\App.jsx /Y
xcopy node_modules\@gusmano\reext\dist\example\main.jsx src\index.js /Y
npx vite --open
React App Setup:
npx create-react-app reextcra
cd reextcra
npm install @gusmano/reext@latest
cp node_modules/@gusmano/reext/dist/example/ReExtData.json src/ReExtData.json
cp node_modules/@gusmano/reext/dist/example/App.jsx src/App.js
cp node_modules/@gusmano/reext/dist/example/main.jsx src/index.js
npm start
Managing Extensive Data Sets in Development
Handling big chunks of data in app development can be tough. If you’re designing data intensive applications, how you manage that data can really impact your app’s performance. That’s where ReExt steps in with a solid set of pre-built components that are built to handle the load.
Take the grid component. It smoothly manages thousands of records. You can sort, filter, and paginate without writing the logic yourself. ReExt also has built-in state management and real-time data binding, so your app stays fresh and in sync.
You can also make your UI your own. ReExt provides the customisation option for styling and theming. Whether it’s a work dashboard or a student-facing tool, you can make it match your brand without much effort.
And when it comes to performance, especially in data intensive applications, speed really matters. ReExt is smart about what it updates. It only refreshes what’s changed, which helps keep things running smoothly, even on slower devices or memory-hungry setups.
State Management Example
// Define context
const AppContext = React.createContext();
// Provide context in App component
const App = () => {
const [state, setState] = useState(initialState);
return (
< AppContext.Provider value={{ state, setState }}>
< MyComponent />
< /AppContext.Provider>
);
};
// Consume context in child component
const MyComponent = () => {
const { state, setState } = useContext(AppContext);
// Use state and setState here
};
Data Binding Example
// Define a model
Ext.define('MyApp.model.User', {
extend: 'Ext.data.Model',
fields: ['name', 'email']
});
// Create a store
const store = Ext.create('Ext.data.Store', {
model: 'MyApp.model.User',
data: [
{ name: 'John Doe', email: '[email protected]' },
{ name: 'Jane Doe', email: '[email protected]' }
]
});
// Use data binding in a component
const MyComponent = () => {
const store = Ext.getStore('MyStore');
return (
< ReExt xtype="grid"
store={store}
columns={[
{ text: 'Name', dataIndex: 'name', flex: 1 },
{ text: 'Email', dataIndex: 'email', flex: 1 }
]}
/>
);
};
Best Practices for Building Data-Intensive Applications
Building data-heavy apps isn’t just about choosing tools. It’s how you use them that counts. You want speed, reliability, and the ability to scale without headaches. Nail that, and you’ve got something solid. Keep it simple, focus on what works, and avoid overcomplicating things. That’s the real win.
Store and Access Data the Smart Way
Before starting, ensure your database is well-planned. Always start with a solid database. Indexed databases and clean schema design can save you from slow queries later.
If your app hits the same data over and over, add a caching layer to speed things up. For most use cases, you’ll choose between PostgreSQL and MongoDB. That choice depends on the structure and size of your data.
Don’t Load Everything at Once
For memory-intensive applications, loading too much data upfront is a bad idea. Use lazy loading, infinite scroll, or pagination. These methods help reduce memory use and keep things responsive. ReExt components make this easier to implement from the start.
Use the Right Data Structures
Your app’s speed often depends on how you organise data. Arrays, trees, and hash maps can really change how efficiently your app handles tasks like sorting or searching. The choice is really important, especially when you are creating applications that handle large datasets.
Handle State Properly
When you’re working with large datasets, managing state gets complicated. Tools like Redux or the Context API in React help keep your app’s state predictable. This matters a lot when designing data-intensive applications that change in real time.
Track Performance Often
Use application performance monitoring tools like Lighthouse or Datadog to catch slowdowns early. These tools show you where memory or network issues are slowing things down.
Build APIs That Can Handle Load
Your APIs need to scale, too. Make sure they can handle big queries, are secure, and have things like caching and rate limiting built in. It’s a must for any data intensive application.
Make the UI User-Friendly
Designing data-intensive pages isn’t just about speed. It’s also about making data easy to understand. Use charts, filters, and visuals to help users explore. ReExt has widgets that help with this.
Keep Improving
As your app grows, keep refining. Refactor old code, update your tools, and stay current. That’s how you keep things running smoothly.
Conclusion
ReExt is a powerful tool for anyone building or designing data-intensive applications. It simplifies how developers manage and present large volumes of data. By merging the capabilities of Ext JS and React, it creates a smooth, reliable experience for both users and developers.
Its smart handling of UI, memory usage, and performance monitoring makes it ideal for everything from enterprise tools to lightweight apps that still deal with heavy datasets. Whether you’re building reporting dashboards, admin panels, or transactional platforms, ReExt ensures your app remains responsive and clean.
With the best practices we’ve shared, along with the setup guides and usage examples, you should feel confident designing your next big data-driven solution using ReExt and other React frameworks.
FAQs
How to design data-intensive applications?
Start by choosing scalable technologies like ReExt. Use smart data loading, efficient UI components, and monitoring tools to ensure performance.
How to access application data?
Accessing data usually involves APIs connected to databases or services. Use Ext JS stores and React state/hooks for a structured flow.
How to build data-intensive applications?
Use a combination of frameworks like ReExt, and follow best practices like lazy loading, state management, and performance profiling.
What is application performance monitoring?
App performance monitoring means watching how your app runs in real time. It checks stuff like speed, how well it handles heavy loads, and memory use. You spot issues fast and keep things running smoothly for users.
What are application performance monitoring tools?
Apps run smoother when you spot slowdowns fast. Tools like Google Lighthouse, New Relic, or Chrome DevTools show you where things lag. Datadog’s handy, too. They all help tweak performance so your app works better for users.
Which one of the following is considered a data-intensive application?
Examples include CRMs, analytics dashboards, video streaming platforms, financial monitoring tools, and e-commerce engines.
Register yourself at ReExt to build high-quality, data-intensive applications in 2024.
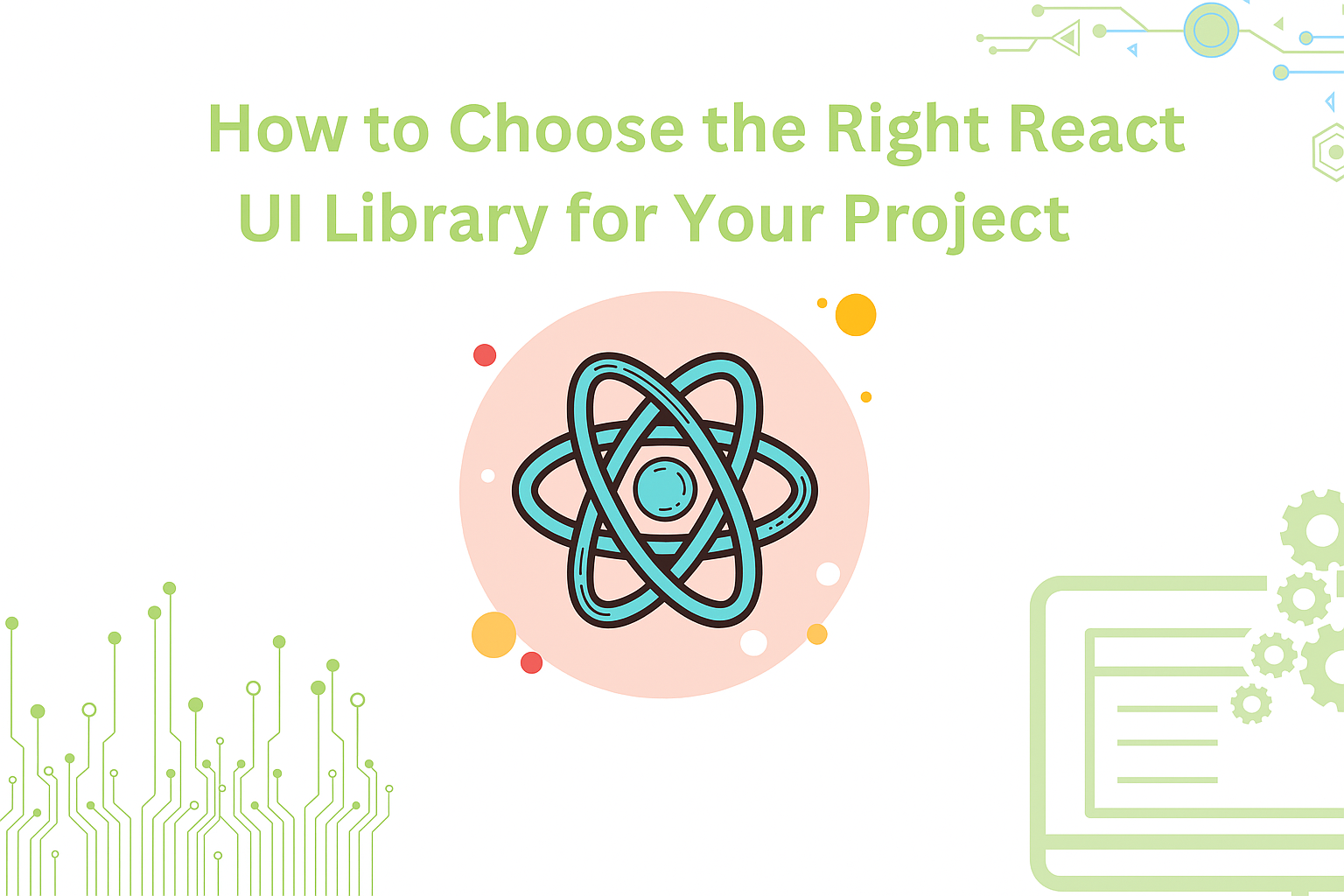
React is perhaps the most widely used web app-building framework right now. Many developers also…
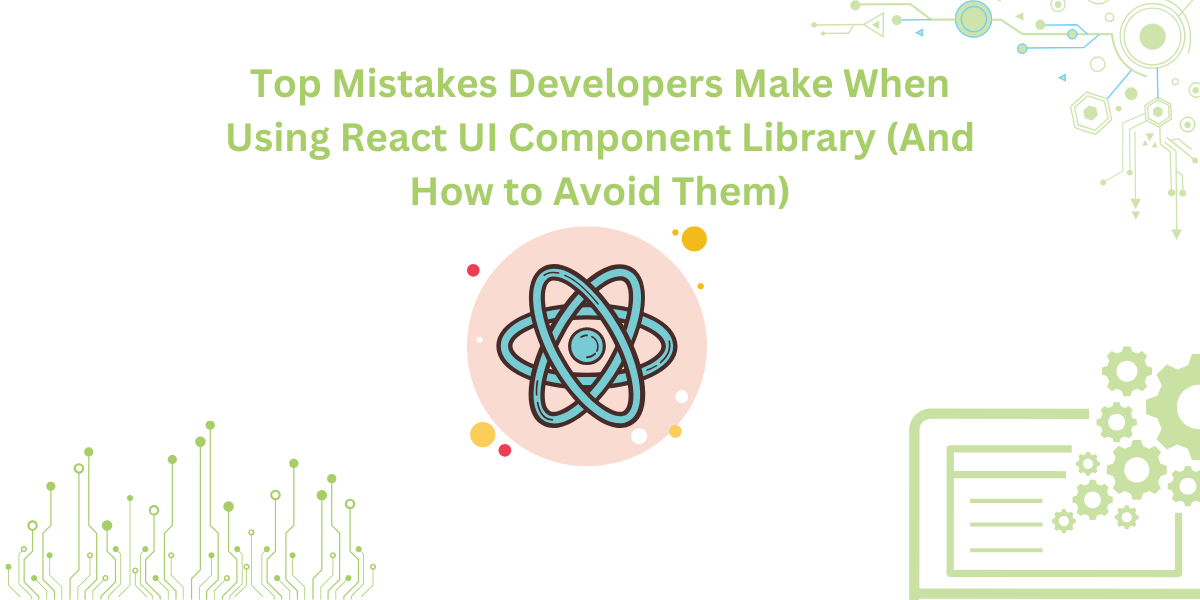
React’s everywhere. If you’ve built a web app lately, chances are you’ve already used it.…
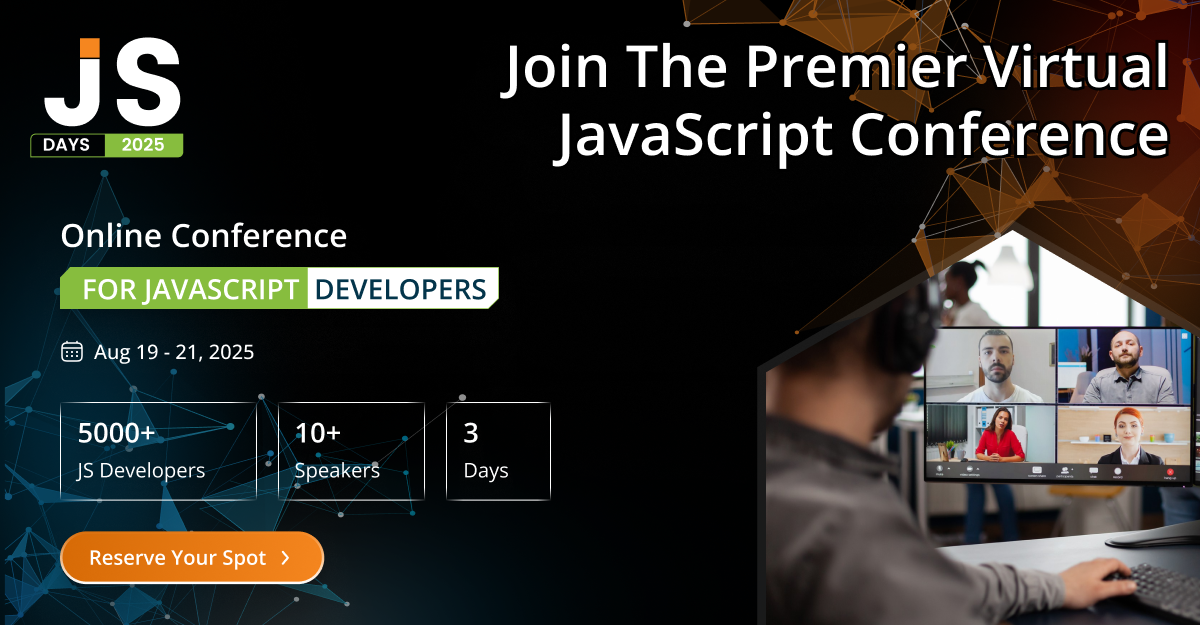
Join 5,000+ developers at the most anticipated virtual JavaScript event of the year — August…