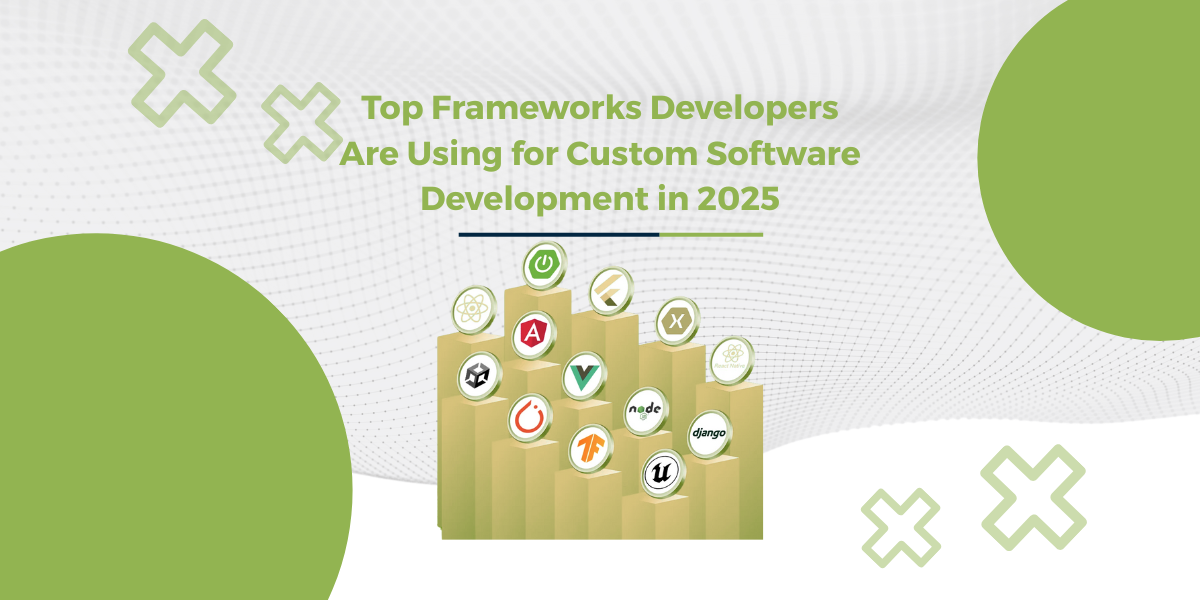
We’re seeing it more every year; teams aren’t settling for plug-and-play tools anymore. In healthcare, finance, logistics, and other data-heavy industries, there’s a clear shift. People are choosing custom software development. Because the old way of buying off the shelf…
Subscribe to our newsletter
Be the first to learn about new Sencha resources and tips.