Event Handling in JavaScript: A Practical Guide With Examples
Back in the day, websites used to be static, meaning users could only view the content but not interact with it. However, we can now create highly interactive user interfaces thanks to JavaScript and JS frameworks. Specifically, event handlers in JavaScript are what allow us to build dynamic web pages and deliver interactive experiences. In the modern web development landscape, events are essentially user actions that occur as a result of user interaction with the web page, such as submitting a form, clicking a button, playing a video on the web page, minimizing the browser window, etc. Event handling allows developers to verify and handle these actions to deliver a more responsive and engaging user experience.
Hence, understanding how events work and how to handle them efficiently is essential for every developer looking to create modern web applications. This article will discuss all the ins and outs of event handling in JavaScript. We’ll also briefly discuss how a good JavaScript framework like Ext JS handles events.
Understanding Events in JS (JavaScript)
Events are essentially the actions that occur on a web app due to user interaction, such as clicking a button. In JavaScript, when an event occurs, the app fires the event, which is kind of a signal that an event has occurred. The app then automatically responds to the user in the form of output, thanks to event handlers in JavaScript. An event handler is essentially a function with a block of code that is executed or triggered when a specific event fires.
Sometimes, when an event occurs, it triggers multiple events. This is because web elements in an app are often nested. This is where event propagation comes in. Event propagation involves capturing and bubbling phases as the event travels across the DOM hierarchy. We’ll discuss these phases later in the article.
There are common types of events:
- Keyboard/touch events: Occur when a user presses or releases a key on the keyboard or performs an action with a touch-enabled smartphone, laptop or tablet.
- Click events: Fires when a user clicks on a button or other such web element.
- Mouse hover events: These events are fired when a user performs an action with the mouse, such as scrolling a page or moving the cursor.
- Form/submit events: Triggered when a user submits a form, modifies it, or resets it.
- Drag and drop events: Occurs when a user drags and drops an element on the web page, such as dragging and dropping an image on a file uploader.
Event Listeners
An event listener is essentially a JavaScript function that waits for a specific event to occur and then executes a callback function to respond to that event. Event listeners and event handlers are often considered the same thing. However, in essence, they work together to respond to an event. As the name suggests, the listener listens for the event, and the handler is the code that is executed in response to that event.
There are two common built-in event listener methods in JavaScript: addEventListener and removeEventListener. The addEventListener() method enables us to attach an event handler to an element. We can also add multiple event handlers to an element. removeEventListener() allows us to remove an event listener/handler from a specific element.
Event Object
When an event occurs, it belongs to a specific event object. The event object is essentially the argument passed into the callback/event handler function. It provides information about the event, such as the target element, the type of event, etc. It also contains additional properties for the specific event type.
Here are common event properties:
- target: Represents the element that fired the event.
- type: Tells about the specific type of the event, such as click or submit
- keyCode: Used for keyboard events. It contains the Unicode value of the key pressed by the user
Here is an example code demonstrating the use of the event object (Click event):
<!DOCTYPE html>
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title> Example of using Event Object</title>
</head>
<body>
<button id="myButton">Click me!</button>
<script>
var button = document.getElementById("myButton");
button.addEventListener("click", function(event) {
// Accessing event properties
console.log("Type of Event: " + event.type);
console.log("Target Element: " + event.target);
});
</script>
</body>
</html>
Basic Event Handling Examples
Based on the concepts we discussed in the previous sections, here is an example for creating a simple button-click event:
<!DOCTYPE html>
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Button-Click Event</title>
</head>
<body>
<button id="myButton">Click me!</button>
<script>
var button = document.getElementById("myButton");
// Adding an event listener
button.addEventListener("click", function() {
alert("Button is clicked!");
});
</script>
</body>
</html>
Here is a basic example demonstrating how to handle form submissions:
<!DOCTYPE html>
<head>
<title> Handling Form Submission </title>
</head>
<body>
<form id="myForm">
<label for="username">Username:</label>
<input type="text" id="username" name="username">
<br>
<label for="password">Password:</label>
<input type="password" id="password" name="password">
<br>
<input type="submit" value="Submit">
</form>
<script>
var form = document.getElementById("myForm");
// Adding an event listener for form submission
form.addEventListener("submit", function(event) {
event.preventDefault(); // Preventing the default form submission
// Accessing form data
var username = document.getElementById("username").value;
var password = document.getElementById("password").value;
//form validation
console.log("Username: " + username);
console.log("Password: " + password);
});
</script>
</body>
</html>
Preventing Default Behavior
Web browsers often have a default behavior for certain events. When such an event occurs, the browser’s default behavior is triggered in response to that event. preventDefault() provides us with a way to stop or prevent this default behavior.
For instance, when a user submits a form, the browser automatically initializes a request to the server. This results in page reload or navigation to a new page, affecting the user experience. Developers can use preventDefault() to stop this default behavior and handle form submission asynchronously without causing a page to reload. For example, in the above code, we’ve used preventDefault() to stop or prevent the default form submission behavior.
Advanced Event Handling
Event Delegation
Event delegation in JavaScript is an advanced technique for handling events more efficiently. In event delegation, we add or attach an event listener/listeners to a common parent element. This way, we don’t have to attach the event listener to each element separately. Events are processed and monitored as they traverse the DOM hierarchy. Event delegation is common in popular javascript frameworks
Here is an example of event delegation:
<ul id="myList">
<li>Item A</li>
<li>Item B</li>
<li>Item C</li>
</ul>
<script>
var listitems = document.getElementById("myList");
listitems.addEventListener("click", function(event) {
if (event.target.tagName === "LI") {
alert("Clicked on: " + event.target.textContent);
}
});
</script>
Handling Keyboard Events
Handling keyboard events, such as key down and key up, allows us to:
- Respond to user interactions/inputs with the keyboard
- Implement keyboard shortcuts
- Validate user input in real-time, etc.
Key Down and Key Up are two main types of mouse events. A key-down event occurs when a user presses a key on the keyboard. A key-up event is triggered when a user releases the key after it is pressed down.
Here is an example code for handling a key-down event:
document.addEventListener("keydown", function(event) {
console.log("Key pressed: " + event.key);
});
Here is an example code for handling a key-up event:
document.addEventListener("keyup", function(event) {
console.log("Key released: " + event.key);
});
Handling Touch and Mobile Events
Handling touch and mobile events to create a responsive and touch-friendly design, providing an intuitive way to interact with the web app.
Here is an example code for Touchstart, Touchmove, and Touchend:
element.addEventListener("touchstart", function(event) {
console.log("Touch started: ", event.touches[0].clientX, event.touches[0].clientY);
});
element.addEventListener("touchmove", function(event) {
console.log("Touch moved: ", event.touches[0].clientX, event.touches[0].clientY);
});
element.addEventListener("touchend", function(event) {
console.log("Touch ended");
});
Other touch and mobile events include:
- Gesture events, such as gesturestart, gesturechange, and gestureend. These events are used for gestures like pinch-zoom.
- orientationchange event used for detecting changes in device orientation.
Also Read: Angular vs Ext JS: Which JavaScript Framework Should You Use?
Event Bubbling and Capturing
As aforementioned, event bubbling and capturing are a part of the event propagation process. In event bubbling, the event starts from the same target element that fired the event. It then bubbles up or propagates through its parent and ancestor elements in the DOM till it reaches the root element. This allows you to handle the event in a parent element instead of the target element. Event bubbling is the default event behaviour on elements.
In event capturing, the event traverses from the outermost parent or ancestor element to the target element. It is also called event trickling.
Custom Events
JavaScript also allows you to create and dispatch custom events designed to meet your specific application needs. For instance, you can create custom events for cross-component state management.
Here is how to create a custom event:
// Syntax: new CustomEvent(eventType, options)
var customEvent = new CustomEvent('customEventName', { detail: { key: 'value' } });
Here is how to dispatch the event:
// Syntax: element.dispatchEvent(event)
document.getElementById('myElement').dispatchEvent(customEvent);
Best Practices and Tips
- Combine multiple events that trigger similar actions into one event listener.
- Optimize event callback functions for faster response times.
- Avoid attaching event listeners directly to a global scope.
- Utilize event delegation.
- Use event capturing only when needed. Otherwise, use bubbling.
- Remove redundant event listeners.
- Optimize DOM manipulations for better performance.
Also read: Best JavaScript libraries.
Event Handling in Ext JS
Ext JS is a leading Javascript framework for creating high-performance web and mobile applications. It offers over 140+ pre-built components and supports MVVM architecture and two-way data binding. Events are a core concept in the Ext JS framework that enables your code to react to changes in your app. Here is an example code for button-click event in Ext JS:
Ext.create('Ext.Button', {
text: 'Click Me',
renderTo: Ext.getBody(),
listeners: {
click: function() {
Ext.Msg.alert('Success!', 'I was clicked!');
}
}
});
You can learn more about handling events in Ext JS here.
Conclusion
In the web development process, events refer to user actions, such as such as clicking a button, minimizing the browser window, or submitting a form. Event handling in JavaScript and JavaScript frameworks allows us to respond to user actions and interactions and create dynamic and interactive websites. This article explores various concerts related to event handling in JavaScript with examples.
FAQs
What is event handling in JavaScript?
Event handling in JS refers to using event listeners to wait for an event to occur on an element and responding to that event using event handlers or callback functions.
How do I attach an event listener to an element?
You can use JavaScript’s built-in addEventListener() method to attach an event to an element.
What is the event object in JavaScript?
The event object in JS is essentially the argument passed into the callback/event handler function. It provides valuable information about the event, such as the target element, the type of event, etc.
What are the most popular JavaScript frameworks?
Best JavaScript frameworks and JavaScript libraries include Ext JS, React and Angular. Ext JS offers 140+ high-performance pre-built components for developing web applications quickly. React is another popular JavaScript framework known for creating customized and reusable elements and virtual DOM. Angular is another open-source JavaScript framework that utilizes component-based architecture and allows developers to build high-performance single-page applications.
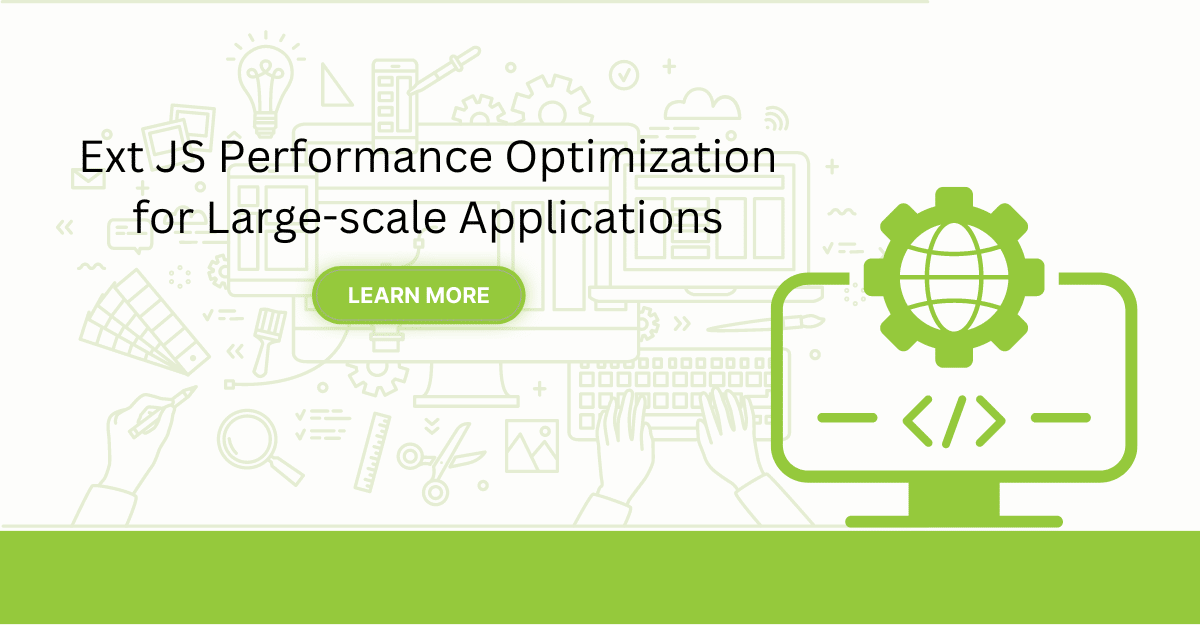
Are you facing issues with Ext JS applications’ performance as they scale up? Don’t worry!…
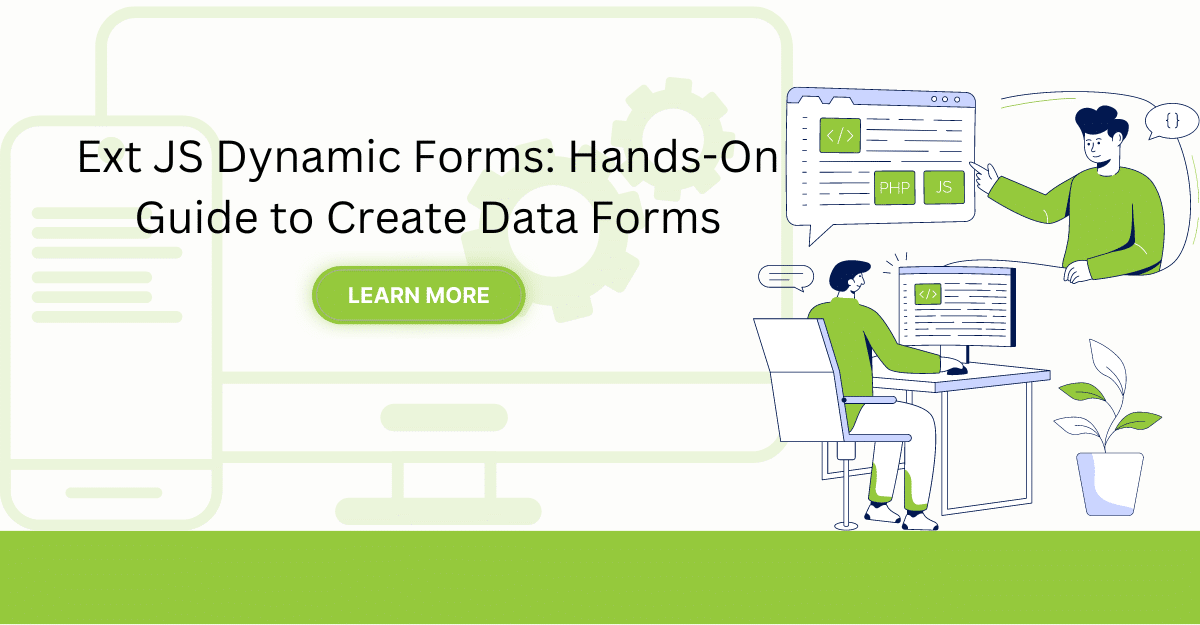
Dynamic forms are changing the online world these days. ExtJS can help you integrate such…
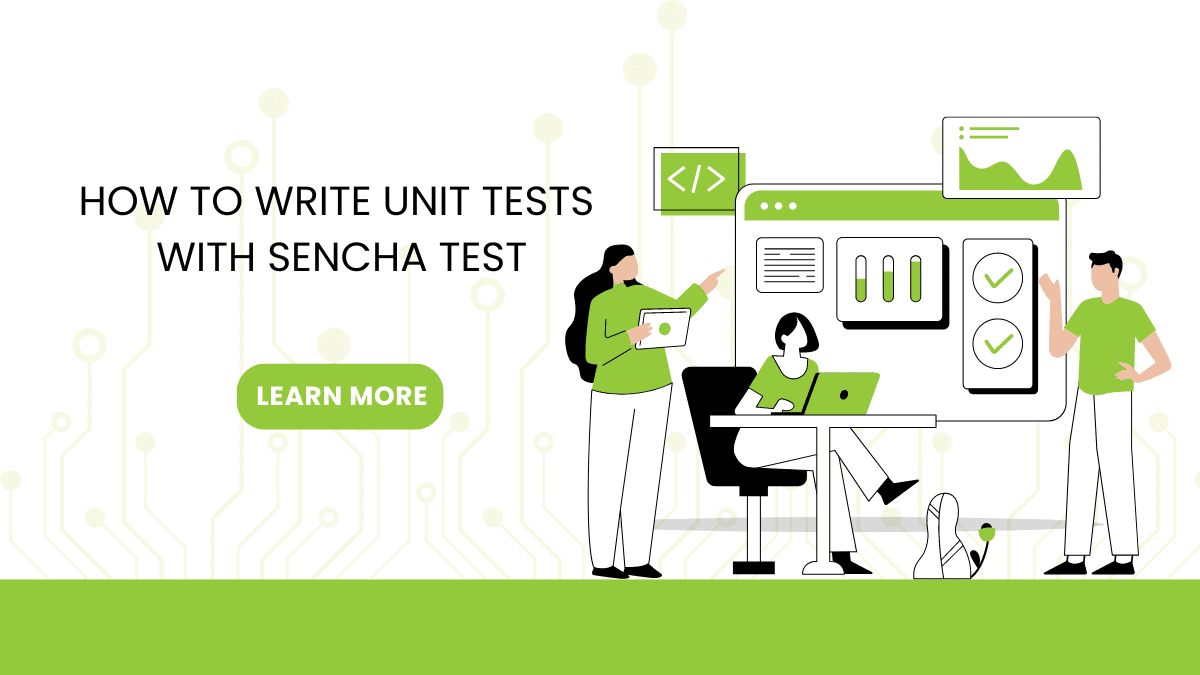
In modern software development, unit testing has become an essential practice to ensure the quality…