Ext JS and ES2015/6/7 – Modernizing the Ext JS Class System
The JavaScript language today is hardly recognizable when compared to the JavaScript of a few short years ago. The changes range from small pieces of syntax (such as loops, variables, and functions), up to the level of classes for creating coherent functional units and extend all the way to connecting these pieces into applications via modules.
Today, through the widespread use of tools such as Babel, Traceur, webpack, and others, many developers have embraced these new language features and their code can still run on “those” browsers.
In my session at SenchaCon 2016 (Ext JS and ES2015/6/7 – Modernizing the Ext JS Class System), we’ll talk about how this evolution in language and tools affects the applications we write using Ext JS. We’ll look at how the class
keyword compares with Ext.define
, and how class names line up with modules. And we’ll compare the two end-states of an upgraded application: one with minimal changes to existing code and one that fully embraces all of the new language features.
Don’t have your ticket yet for SenchaCon 2016? Register today.
You can try the new and updated features of Javascript Web Framework which help to modernize your team and apply modern techniques when building applications.
Sneak Peak
Class Declarations
Let’s take a simple utility class that might be found in an Ext JS application today and see what an upgrade might look like.
Ext.define('App.util.Foo', { extend: 'App.some.Base', mixins: 'Ext.mixin.Observable', config: { value: null } constructor: function (config) { this.mixins.observable.constructor.call(this, config); }, updateValue: function (value, oldValue) { this.fireEvent('valuechange', value, oldValue); } });
The following diagram shows the important pieces produced by the code above:
If you look at the constructor property on the prototype, you’ll see that it points to the method we passed to Ext.define
. This approach, while flexible, is not compatible with the new class
keyword. Using the class
keyword produces the following, immutable relationship:
App.util.Foo === App.util.Foo.prototype.constructor
Minimal Upgrade
The minimal upgrade requires us to rename the “constructor” method to avoid this conflict. The new name is simply “construct
“:
Ext.define('App.util.Foo', { extend: 'App.some.Base', mixins: 'Ext.mixin.Observable', config: { value: null } construct (config) { this.mixins.observable.construct.call(this, config); }, updateValue (value, oldValue) { this.fireEvent('valuechange', value, oldValue); } });
Though not necessary, we’ve also taken advantage of the new function property syntax for object literals and removed the “: function
” from our methods.
Because the new Ext.define
internally produces a standard class
, we gain some benefits as well. Consider the following diagram showing what is generated by the upgraded Ext.define
call above:
Because class constructors are now prototype-chained to their superclass constructors, effectively all static members are inheritableStatics for free!
Maximum Upgrade
At the opposite end of the upgrade continuum is a complete upgrade to all the new language features and options. Let’s look at what the fully upgraded version of the above class might look like:
import { define } from 'extjs-kernel'; // module names not final import { Observable } from 'extjs-core'; import { Base } from 'app-some'; @define({ mixins: Observable, config: { value: null } }) export default class Foo extends Base { updateValue (value, oldValue) { this.fireEvent('valuechange', value, oldValue); } }
In this version, we’re taking advantage of several new class system features as well as some upcoming JavaScript features.
If you haven’t seen the @define
syntax before it’s called a decorator, and it’s an exciting language feature currently being worked through the standardization process. It’s available today via the magic of transpilers. Transpilers are tools that compile JavaScript-to-JavaScript or more precisely, Advanced-JavaScript-to-Browser-Friendly-JavaScript. Through the @define
decorator, you can apply all the additional powers of the Ext JS class system to this standard class
.
We also removed the construct/constructor
methods in this example. This is because the construct
method of Ext.Base
now provides a Common Object Lifecycle. When called with a single object parameter or if the class uses the Config System, the necessary steps to initialize the instance are handled automatically.
Last, we’re using the new, standard import
and export
keywords. Support for these is also by virtue of transpilers (because no browser yet supports them). To learn more of the details, you can also attend Ross Gerbasi’s talk: The Modern Toolchain.
Choices, Choices
Because there is no one-size-fits-all approach, the overarching goal of this upgrade is to minimize what must be done to existing code while at the same time enabling developers to usefully modernize JavaScript techniques and practices.
To hear first-hand what else is coming, be sure to attend my session at SenchaCon 2016!
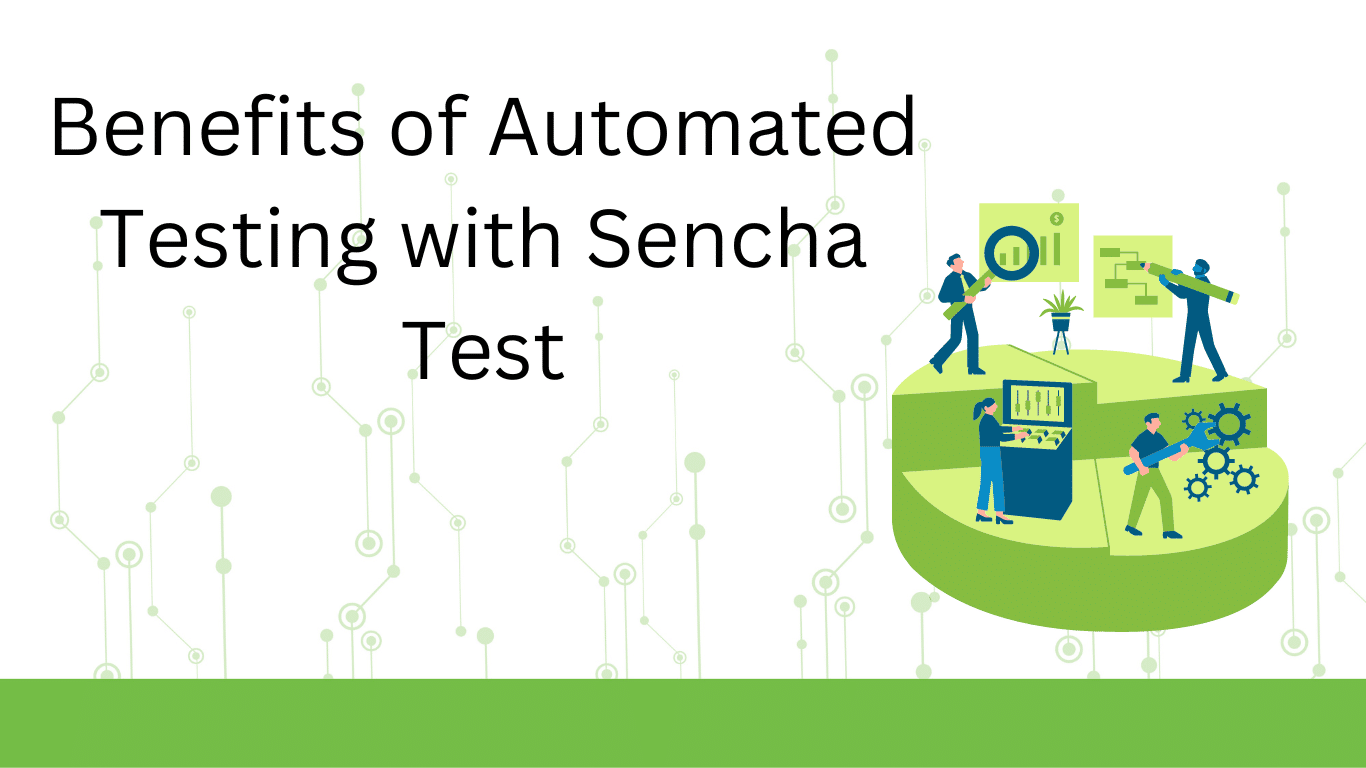
Software testing has always been a critical part of software development. It allows us to…
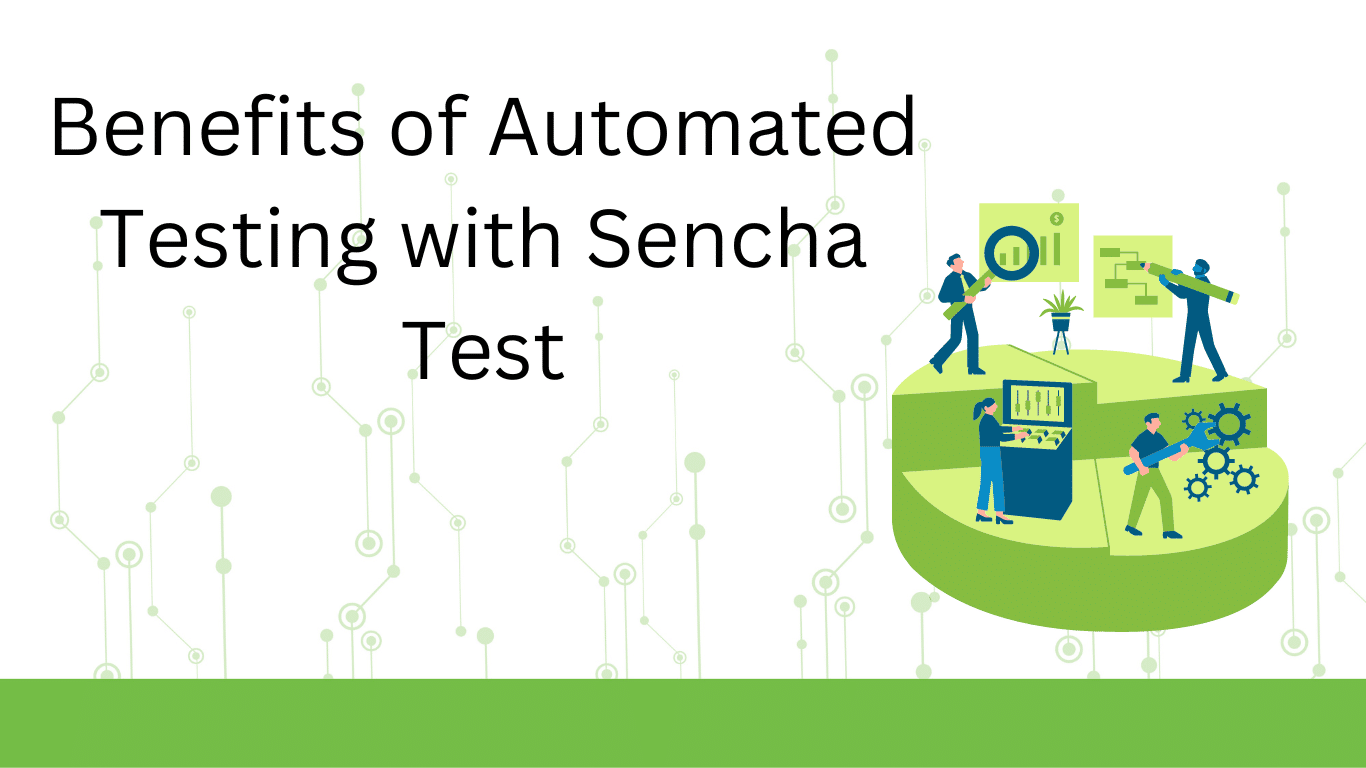
Software testing has always been a critical part of software development. It allows us to…
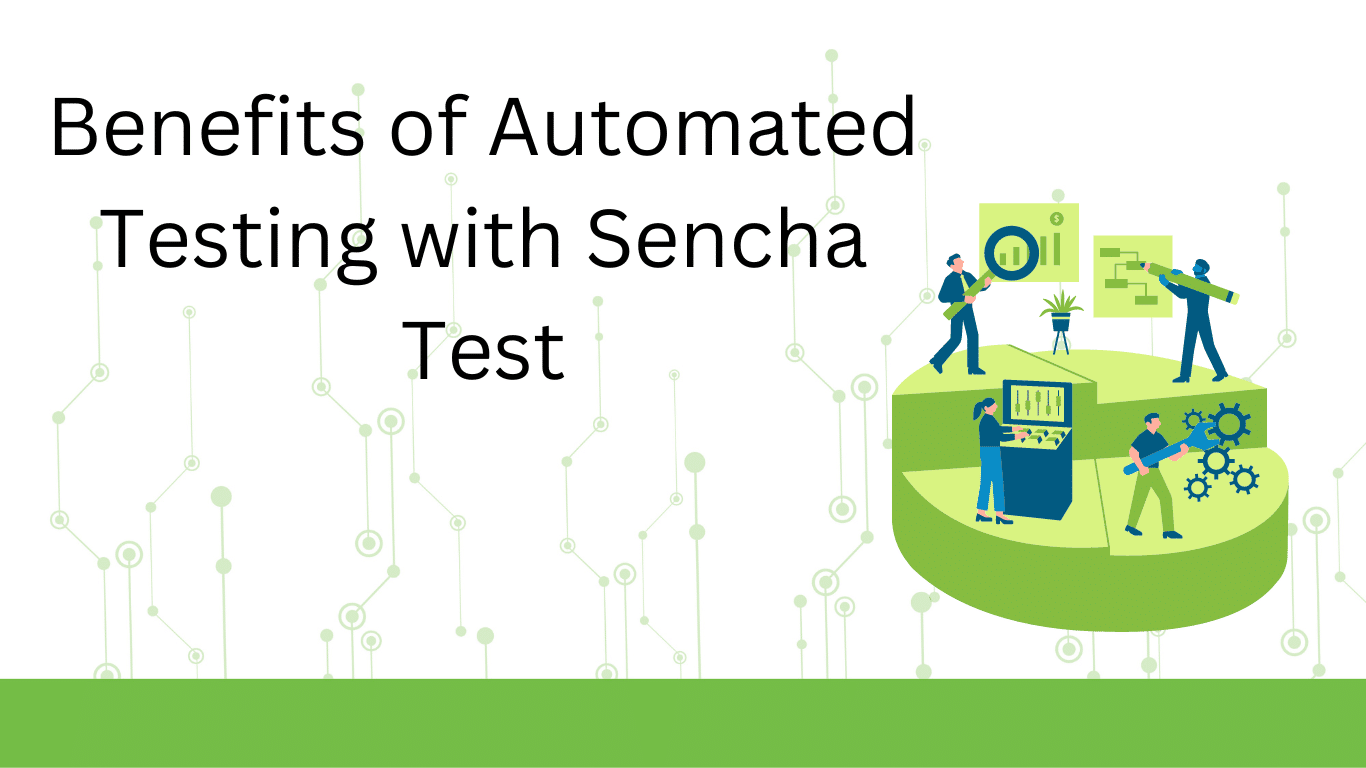
Software testing has always been a critical part of software development. It allows us to…