Recursion vs. Iteration: Choosing the Right Approach in Ext JS
Recursion versus iteration is one of the common debates in programming. In a recursion, a function calls itself until it reaches a base condition. It’s often used for problems that can be broken down into similar sub-problems. Iteration, on the other hand, repeats a set of instructions in a loop until a condition is met. Typically, iteration is more memory-efficient than recursion, as it doesn’t need multiple function calls. It’s important to select the right approach when working with most JS frameworks. Ext JS, being a comprehensive JavaScript framework, requires the same. In this article, we will discuss this matter in greater detail.
Understanding Recursion in Ext JS
Let’s start our tutorial, by understanding recursion in JS. For that, we need to know what is recursion, how it works in a web app framework, and the pros and cons of using recursion.
Definition of Recursion
Ext JS, a popular JavaScript framework, enhances code execution efficiency and user interface responsiveness through its integration with the virtual DOM concept.
Recursion in the Ext JS framework refers to the technique where a function calls itself within its own definition to solve a problem. It’s a powerful programming technique used to simplify developing web applications that handle complex, nested data structures common in Ext JS. Recursion is particularly useful in Ext JS for traversing and manipulating hierarchical data, like UI web components and DOM elements. It breaks down a large problem into smaller, more manageable instances, each solved with the same recursive logic.
How Recursion Works in JavaScript
In a JavaScript framework, as in Ext JS, a recursive function continues to call itself with modified parameters until it reaches a base case. The base case is important as it prevents infinite recursion and eventually terminates the recursive calls. Let’s understand this with a code example.
In this example, we’ll use a recursive function to determine the depth of a nested structure, such as a menu. This is a typical use case in Ext JS applications.
var menu = {
text: 'File',
items: [{
text: 'New',
items: [{
text: 'Project'
}, {
text: 'File'
}]
}, {
text: 'Open'
}]
};
function calculateDepth(item) {
if (!item || !item.items) {
return 1;
}
var maxDepth = 0;
Ext.each(item.items, function(subItem) {
var depth = calculateDepth(subItem);
maxDepth = Math.max(maxDepth, depth);
});
return maxDepth + 1;
}
var depth = calculateDepth(menu);
console.log('Depth of the menu:', depth);
In this example, we define a simple menu structure with nested items. The calculateDepth function serves as the recursive function. It checks if the current item has nested items (children). If there are nested items, it recursively calls itself for each child, calculating their depth. The function returns the maximum depth found among the children, plus one for the current level. Finally, we call calculateDepth on our menu object and log the result.
Pros and Cons of Using Recursion in Ext JS
On the pros side, recursion simplifies code for complex algorithms in Ext JS. When you use recursion for complex problems that are well-suited to this approach, it makes the code more readable and maintainable. Recursion is particularly suitable for problems related to tree structures, which are prevalent in Ext JS UI components.
Regarding the cons, recursive solutions can be less efficient and slower than iterative solutions in Ext JS. Moreover, deep recursion can lead to stack overflow errors in Ext JS, especially with large data sets. Furthermore, debugging recursive code can also be more challenging. Additionally, recursion in Ext JS may consume more memory, as each function call maintains its own set of variables.
Examples of Recursive Functions in Ext JS
A common example of recursive functions in Ext JS is their use in recursively traversing the Component tree. Additionally, recursive algorithms are often employed for dynamically building or updating UI components based on hierarchical data. Another practical example is the recursive data binding functions in Ext JS. These functions effectively bind parent-child components within a nested structure.
Embracing Iteration in Ext JS
Before starting to use iteration in JS frameworks, you need to know the basics of it. In this section, you will learn, its definition, different iteration methods, and the pros and cons of using it in JS frameworks like Ext JS.
Definition of Iteration
Iteration in programming or JS frameworks refers to the technique of repeating a set of instructions in a loop until a specific condition is met. In Ext JS and other JS frameworks, iteration is often used for executing code blocks repeatedly. This is commonly used for processing arrays or collections of UI components. This method contrasts with recursion in that it uses a straightforward loop structure instead of function calls to itself
Different Iteration Methods in JavaScript
There are 4 common iteration methods in JS frameworks. Those are
- For Loop: in JS frameworks, the for loop is widely used for iterating over arrays or collections with a known number of elements.
- While and Do-While Loops: These loops are used when the number of iterations is not known in advance. They will be executed as long as the condition remains true.
- forEach Method: Arrays in JS frameworks offer the forEach method. This allows a function to execute for each array element. This is widely used in Ext JS for iterating over component collections.
- for…in and for…of Loops: The for…in loop iterates over object keys, while the for…of loop is used for iterating over iterable objects like arrays, strings, or NodeList objects. This method is useful in DOM manipulation in Ext JS and other JS frameworks.
Pros and Cons of Using Iteration in Ext JS
Iteration results in faster execution compared to recursion. Therefore, it plays an important role in enhancing the performance of Ext JS applications. Moreover, it’s more memory-efficient, as it doesn’t add multiple frames to the call stack. Also, iterative loops are generally easier to understand and debug. As a result, with iteration, code maintenance is simpler in Ext JS.
There are a few cons of iteration as well. Those are
- Iterative solutions can sometimes be less elegant or straightforward than recursive ones
- In Ext JS, complex nested structures may be more cumbersome to handle iteratively as opposed to recursion.
- Iteration may lead to more verbose code, especially for operations naturally suited to recursion.
Comparing Recursion and Iteration in Ext JS
Let’s compare recursion and iteration to use them most effectively in JS frameworks. In this section, we compare them based on their differences in terms of performance, memory usage, code clarity, and appropriate use cases. Extjs is one of the best JavaScript frameworks. Therefore, most of the facts mentioned here are accurate for other frameworks as well such as Angular and React. Angular is an open source JavaScript framework and React is an open source JavaScript library. React specifically follows to way data binding.
Performance Considerations
Recursion is often slower in Ext JS due to the overhead of multiple function calls. This is especially noticeable in cases of deep recursive calls or large data sets. On the other hand, iteration offers better performance, particularly in Ext JS, as loop constructs are more efficiently handled by JavaScript engines.
Moreover, recursion can lead to stack overflow errors in cases of very deep recursion. In contrast, iteration is more suited for performance-critical tasks in Ext JS, such as rendering large data sets in grids or lists.
Memory Usage Implications
Recursion increases memory usage because each recursive call adds a new frame to the call stack. Iteration uses less memory as it doesn’t involve the overhead of multiple call stack frames. The cumulative memory overhead of recursion can become significant in complex Ext JS applications. Thus, for memory-constrained environments like client-side Ext JS applications, iteration is preferred.
Code Readability and Maintainability
Recursion offers more elegant and concise solutions for complex problems in JavaScript web frameworks. However, the inherent complexity in understanding recursive calls can pose challenges in debugging. On the other hand, iteration is generally easier to understand and debug due to its straightforward nature. Although iteration results in more verbose code, it aligns well with the structured approach of Ext JS and other JavaScript libraries.
Use Cases for Recursion and Iteration in Ext JS
Recursion is ideal for tasks that deal with hierarchical data structures, such as traversing and manipulating DOM trees or Ext JS component hierarchies. It’s also suited for algorithms that naturally fit the recursive style.
Iteration is best suited for straightforward, repeatable tasks such as processing arrays or updating UI elements. It’s also suitable for scenarios where the number of repetitions is known, or for processing large data sets without deep nesting.
Best Practices for Choosing Between Recursion and Iteration
In Ext JS, which is the best JavaScript framework, the decision between using recursion and iteration is important. There are some best practices and guidelines to help developers make the correct decision when selecting between them. In this section, we focus on that. I’ll write it as a set of four steps.
Guidelines for Deciding When to Use Recursion
These are some of the guidelines you can use when deciding to use recursion in your Ext JS JavaScript framework.
Analyze the Problem Structure – Examine if the problem has a naturally recursive structure. This includes tasks like tree traversals or operations on hierarchical data. If the problem breaks down into smaller, similar sub-problems, it’s a strong indicator for recursion.
Assess the Depth of Recursion – Estimate the depth of recursion required. If it’s relatively shallow and won’t lead to stack overflow, recursion can be a good option.
Check for Readability and Maintainability – Write a basic version of both recursive and iterative solutions. Compare the two for readability and maintainability. If the recursive version is significantly clearer and easier to understand, it might be the better choice.
Consider the Performance Impact – Profile the recursive solution to evaluate its performance, especially if working with custom elements with large data sets. If the performance is comparable to iterative solutions and meets the application’s requirements, recursion can be used.
Guidelines for Deciding When to Use Iteration
These guidelines will help you decide whether to use iteration in an application developed with Ext JS or all JS frameworks.
Evaluate Dataset Size and Performance Needs – Consider the size of the dataset. Iteration is often more suitable for large or flat data structures for any javascript library.
Analyze Problem Complexity – Determine if the problem can be solved with a straightforward loop. If the solution is simple and does not involve nested structures, iteration is likely more efficient.
Consider Memory Constraints – Evaluate the memory usage of both approaches. In web development, iterative solutions typically use less memory than recursive ones. If your Ext JS application has memory constraints, iteration may be the preferable option.
Assess Code Maintainability – Develop simple versions of both iterative and recursive solutions. Compare the maintainability of the two. If the iterative solution is easier to understand and modify, particularly for team projects, it should be favored.
Balancing Performance and Code Clarity
As you already know, iteration is often more performance-efficient, while recursion can provide more elegant and readable solutions. Therefore, it’s important to use both and balance performance and code clarity in JavaScript development. When coding, developers should consider the readability, maintainability, and performance impact of each approach. Ultimately, this choice should enhance the application’s functionality without compromising on code quality or user experience.
Case Studies and Examples
One of the common uses of recursion in Ext JS is to dynamically build a nested menu structure based on a hierarchical data model. Recursion is also often used in implementing search algorithms for features like filtering items.
Developers use iterations mostly for processing large datasets in JavaScript grid components. Another common use case of iteration is employing iterative loops to attach event handlers to multiple UI elements. This helps to ensure consistent behavior across the application.
Tips and Tricks for Optimal Code Performance
These are some tips and tricks you can use to achieve optimal code performance in recursion.
- Where possible, use tail recursion, a form of recursion where the recursive call is the last operation in the function. This optimizes performance and stack usage.
- Store the results of expensive function calls and return the cached result when the same inputs occur again.
- Define a limit for recursion depth to prevent stack overflow errors, especially in scenarios with potentially deep recursive calls.
These tips and tricks can help achieve optimal code performance in iteration.
- Minimize DOM Manipulations when you deal with a JavaScript grid
- Prefer “for loops” over other loop types, like forEach, as they tend to be more performant.
Also Read: How to Create Professional Design with JavaScript Grid
Conclusion
For all popular JavaScript frameworks, choosing between recursion and iteration in Ext JS depends on the task at hand. Recursion is best suited for complex, hierarchical structures, offering a more elegant approach. In contrast, iteration stands out in handling large datasets and performance-intensive tasks with greater memory efficiency. The key is to balance the requirements of the specific problem with the overall performance and maintainability of the application. Developers should use Ext JS’s robust tools for performance profiling, continuously learning and adapting their approach for optimal outcomes in creating web apps.
FAQs
Can recursion impact performance in JavaScript?
Yes, recursion can impact performance, especially in cases of deep recursion and large datasets.
How does recursion work in JavaScript?
In JavaScript, recursion works by a function calling itself within its own definition. This will happen until it reaches a base case which terminates the recursive calls.
How do I avoid stack overflow errors when using recursion?
To avoid stack overflow errors in recursion, ensure efficient base cases, limit recursion depth, and consider using tail recursion optimization where applicable.
Elevate your JavaScript skills and conquer recursive challenges in Sencha
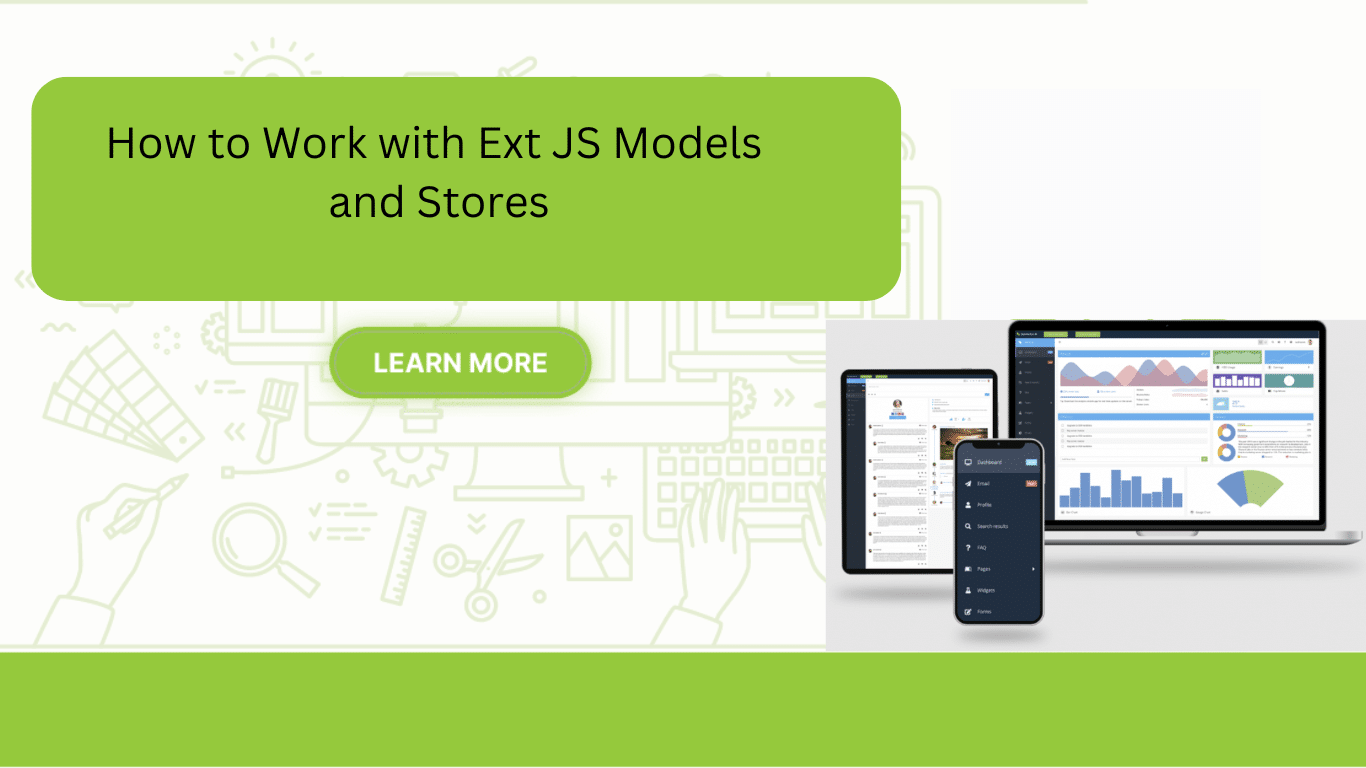
Ext JS is a popular JavaScript framework for creating robust enterprise-grade web and mobile applications.…
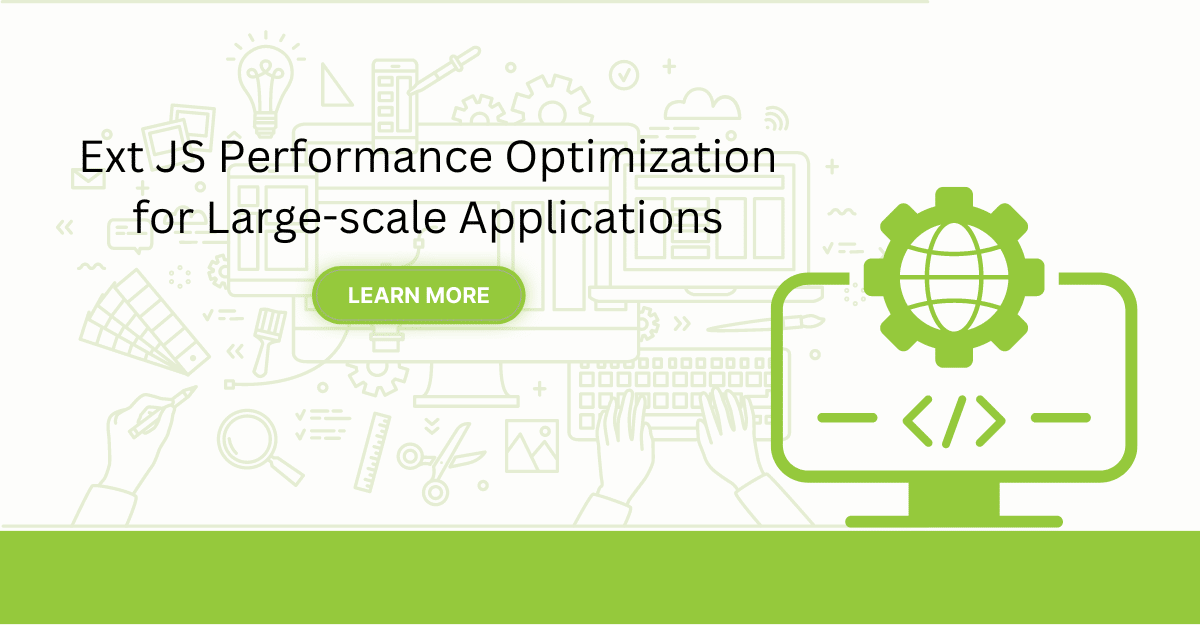
Are you facing issues with Ext JS applications’ performance as they scale up? Don’t worry!…
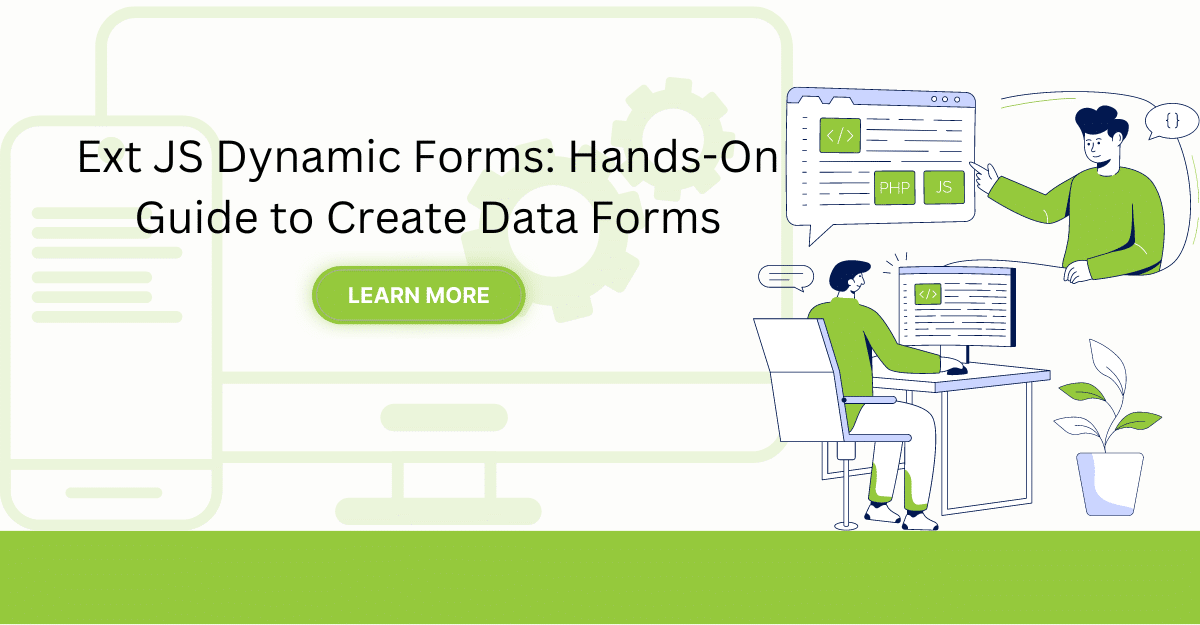
Dynamic forms are changing the online world these days. ExtJS can help you integrate such…