Using Page Object Model in Sencha Test
The Page Object Design Pattern is a great way to organize tests. This method helps with test code maintenance in the long run and reduces code duplication thereby enhancing test engineer productivity.
In the example below, we are creating tests in Sencha Test using Jasmine syntax. Get more information about Jasmine syntax.
If you take an application login screen as an example, you may want to write a series of tests that will try a combination of invalid and valid credentials. As your tests grow, you’ll be making reference to more elements and components in each of your tests:
describe('Login', function() {
it('Should not login when invalid credentials are given', function() {
ST.element('@email')
.focus()
.type('[email protected]');
ST.element('@password')
.focus()
.type('invalidpassword');
ST.element('@submit')
.click();
// Check user hasn't logged in
});
The above test verifies if the user is denied login with invalid credentials, and the test below allows the user to login with valid credentials.
it('Should login with valid credentials', function() {
ST.element('@email')
.focus()
.type('[email protected]');
ST.element('@password')
.focus()
.type('validpassword');
ST.element('@submit')
.click();
// Check user has logged in
});
});
As you can see in the two tests above, we have multiple references to the same locators @submit
, @email
, and @password
.
In large test suites, it becomes messy and difficult to maintain these locators when they need to be updated.
This is where a Page Object Model can help. A Page Object Model is just a JavaScript object that can sit at the top of your test suite and hold all the locators in one place, where they can be maintained and updated efficiently.
Using a Page Object Design
If we take the above example, we can refactor it to use a Page Object Model as follows:
describe('Login', function() {
var Page = {
emailField: function() {
return ST.element('@email');
},
passwordField: function() {
return ST.element('@password');
},
submitButton: function() {
return ST.element('@submit');
}
};
it('Should not login when invalid credentials are given', function() {
Page.emailField()
.focus()
.type('[email protected]');
Page.passwordField()
.focus()
.type('invalidpassword');
Page.submitButton()
.click();
// Check user hasn't logged in
});
it('Should login with valid credentials', function() {
Page.emailField()
.focus()
.type('[email protected]');
Page.passwordField()
.focus()
.type('validpassword');
Page.submitButton()
.click();
// Check user has logged in
});
});
When we want to reference one of our elements or components, instead of using ST.element('@email')
, we can reference our Page Object Model instance Page.emailField()
.
Using a Page Object Model puts all the locators in one place, and allows you to name the Page Objects with a more descriptive display name, rather than working with sometimes vague (and complex) locators.
We hope this was helpful. Add your questions or comments below about using the design pattern.
Learn More
If want to learn more about Sencha Test and you’re in the Boston or DC area, join us for a hands-on Sencha Test workshop. In this 4-hour introductory workshop designed for QA and test automation engineers, you’ll become familiar with Sencha Test and walk through labs that help you create and execute tests for a database-driven Ext JS 6 application.
Join us in Boston – March 7th
Join us in Washington D.C. – March 9th
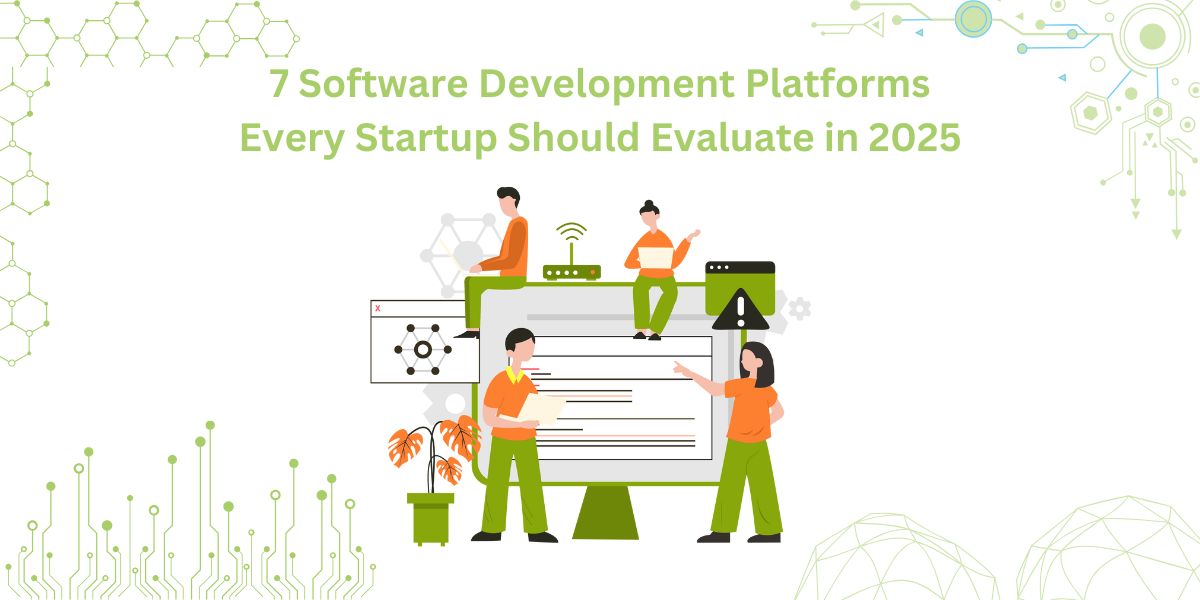
Did you know that nearly 90% of startups fail? And one of the biggest reasons…
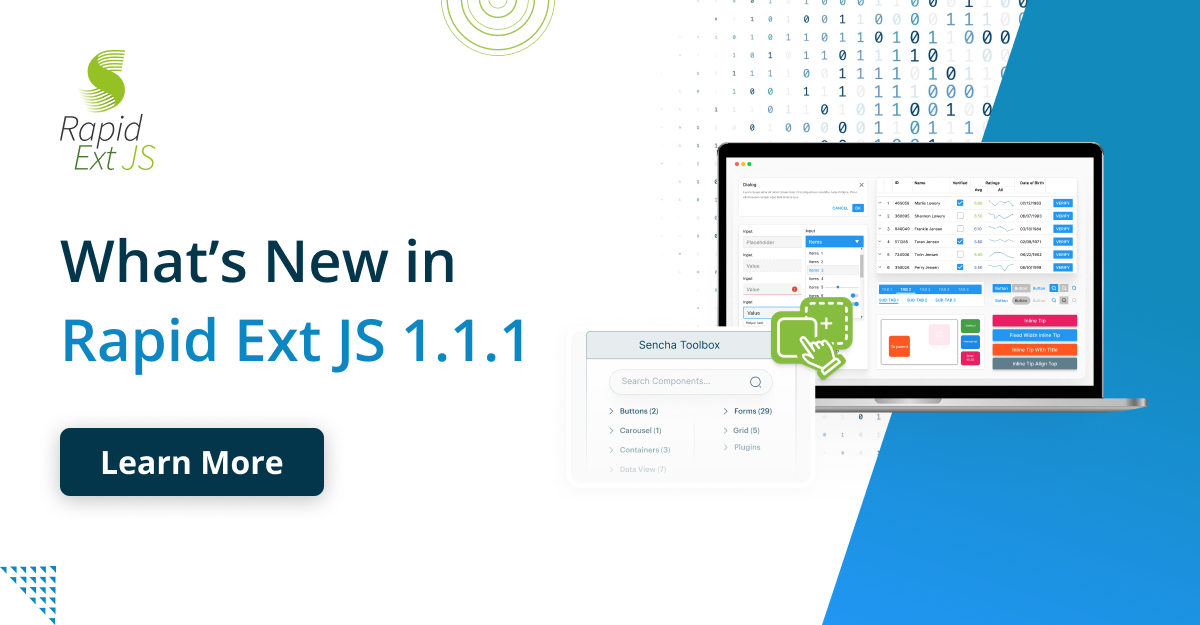
The Sencha team is excited to announce the latest Sencha Rapid Ext JS 1.1.1 release…
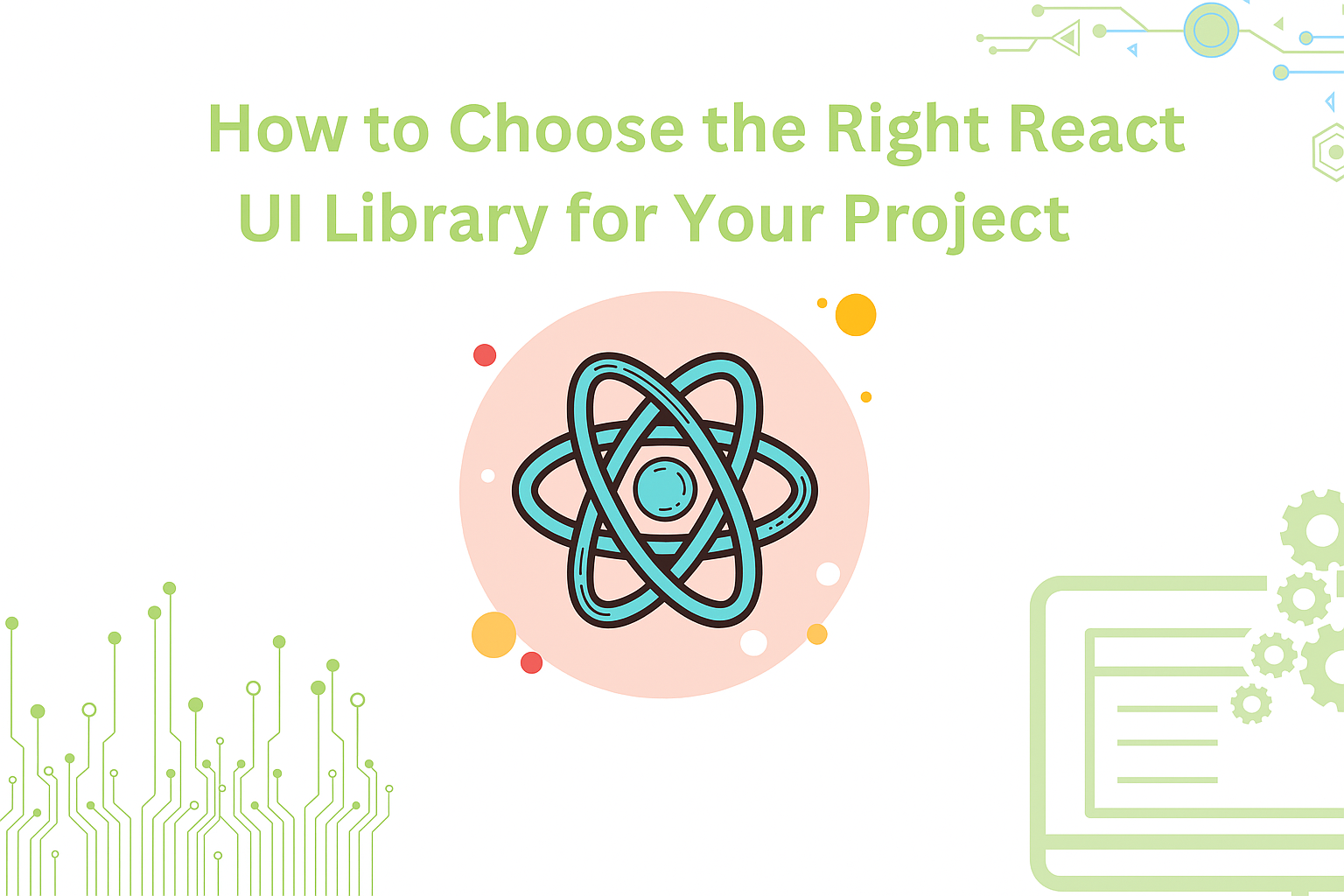
React is perhaps the most widely used web app-building framework right now. Many developers also…