Powerful Data Visualization Using JavaScript Carousels And NASA APIs
When it comes to presenting information visually, carousels are a great way to get the job done. A carousel shows only one of its pages at a time but allows you to browse through multiple full-screen pages using a simple swipe gesture. You can think of a carousel as a single active item, with the rest of the items stretching away to the left and right. Indicator dots let the user know how many screens are available for them to swipe through.
In this blog post, we’ll look at how we can use NASA APIs to create beautiful JavaScript image carousels for powerful data visualization and analysis. This is one of our many extjs tutorial for beginners.
How can I get images from NASA APIs?
NASA provides a bunch of APIs that give you access to a variety of astronomical data. This data includes APOD, Asteroids NeoWs, DONKI, Earth, EONET, EPIC, and Exoplanet, to name a few. To use these APIs, you need to have an access token. You can create your access token by registering on the NASA portal with your email address and password.
For demonstration activities throughout this tutorial, we will use the following API calls to NASA to get the images for our Sencha carousel.
- APOD (current day)
- APOD (specific day)
- Earth imagery
APOD API (current day)
let request = new XMLHttpRequest(); request.open("GET", "https://api.nasa.gov/planetary/apod?api_key=<YOUR_API_KEY_HERE>"); request.send(); request.onload = () => { if (request.status === 200) { // by default the response comes in the string format, we need to parse the data into JSON console.log(JSON.parse(request.response)); } else { console.log(`error ${request.status} ${request.statusText}`); } };
In the code above, we are calling NASA’s planetary APOD API with no optional query parameters. It means that it will return the astronomical picture of the day for the current day.
Notice that the api_key query parameter specifies a placeholder in the code snippet, You should replace this with your personal API access token before making the API call.
Earth Imagery API
let request = new XMLHttpRequest(); request.open("GET", "https://api.nasa.gov/planetary/earth/imagery?lon=100.75&lat=1.5&date=2014-02-01&api_key=<YOUR_API_KEY_HERE>"); request.send(); request.onload = () => { if (request.status === 200) { // by default the response comes in the string format, we need to parse the data into JSON console.log(JSON.parse(request.response)); } else { console.log(`error ${request.status} ${request.statusText}`); } };
Above, we are calling NASA’s earth imagery API with a series of query parameters. The API will return an earth image at the specified latitude and longitude values.
Notice that the api_key query parameter specifies a placeholder in the code snippet. Replace it with your personal API access token before making the API call.
APOD API (specific day)
let request = new XMLHttpRequest(); request.open("GET", "https://api.nasa.gov/planetary/apod?date=2020-05-05&api_key=<YOUR_API_KEY_HERE>"); request.send(); request.onload = () => { if (request.status === 200) { // by default the response comes in the string format, we need to parse the data into JSON console.log(JSON.parse(request.response)); } else { console.log(`error ${request.status} ${request.statusText}`); } };
Here, we are calling NASA’s planetary APOD API with the date as an optional query parameter. This means that it will return the astronomical picture of the day for the specified date.
Again, replace the api_key query parameter’s placeholder with your personal API access token before making the API call.
You can find numerous other NASA APIs at api.nasa.gov.
How can I set up a simple horizontal carousel?
The response of each API call we used above will either return a link to the image on NASA’s server or an image directly. We can use these links to generate a simple horizontal carousel as follows.
Ext.create('Ext.Carousel', { fullscreen: true, items: [{ html: '<p><img src="https://apod.nasa.gov/apod/image/2107/PIA24542_fig2_1100c.jpg"></p>' }, { html: '<p><img src="https://api.nasa.gov/planetary/earth/imagery?lon=100.75&lat=1.5&date=2014-02-01&api_key=<YOUR_API_KEY_HERE>"></p>' }, { html: '<p><img src="https://apod.nasa.gov/apod/image/2005/CarinaPerspective_Fairbairn_960.jpg"></p>' }] });
What is an easy way to make a vertically-oriented carousel?
For the vertical carousel, add a new direction key to generate the carousel vertically.
Ext.create('Ext.Carousel', { fullscreen: true, direction: 'vertical', items: [{ html: '<p><img src="https://apod.nasa.gov/apod/image/2107/PIA24542_fig2_1100c.jpg"></p>' }, { html: '<p><img src="https://api.nasa.gov/planetary/earth/imagery?lon=100.75&lat=1.5&date=2014-02-01&api_key=<YOUR_API_KEY_HERE>"></p>' }, { html: '<p><img src="https://apod.nasa.gov/apod/image/2005/CarinaPerspective_Fairbairn_960.jpg"></p>' }] });
As you can see, creating carousels in JavaScript applications is pretty simple and quick. With minimum code requirements, you can easily create an API-driven image carousel that is dynamic and changes according to the API responses.
Check out the full documentation for building visual stunning carousels with Ext JS.
Ready to get started with Sencha Ext JS? Head over to Sencha Ext JS and create beautiful carousels now.
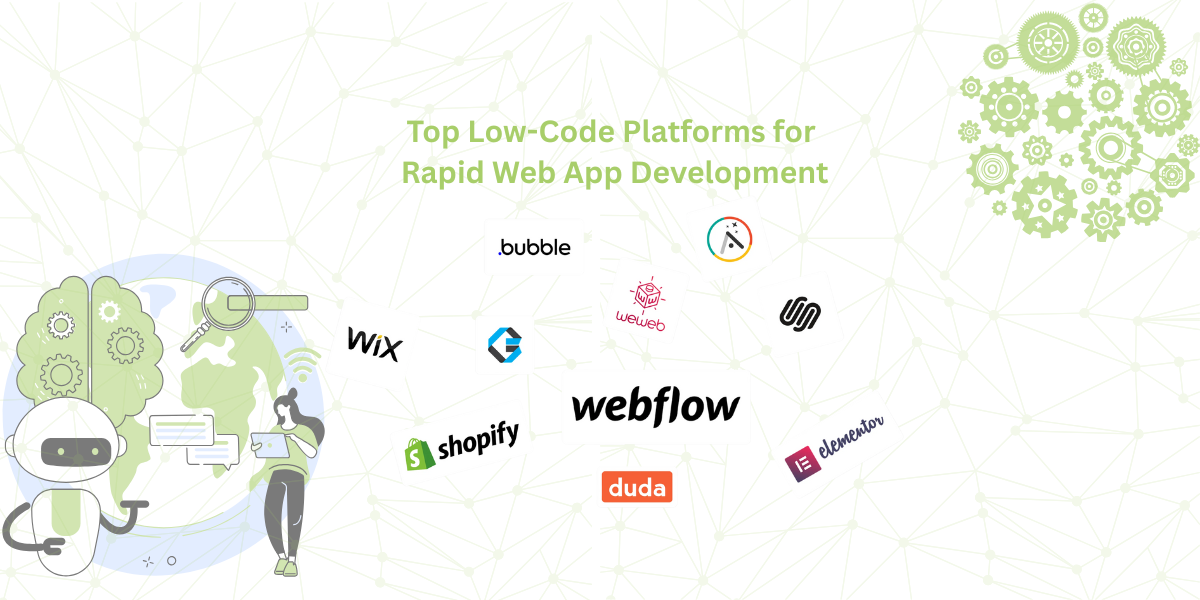
The importance of low-code platforms in business is growing, with estimates suggesting that they will…
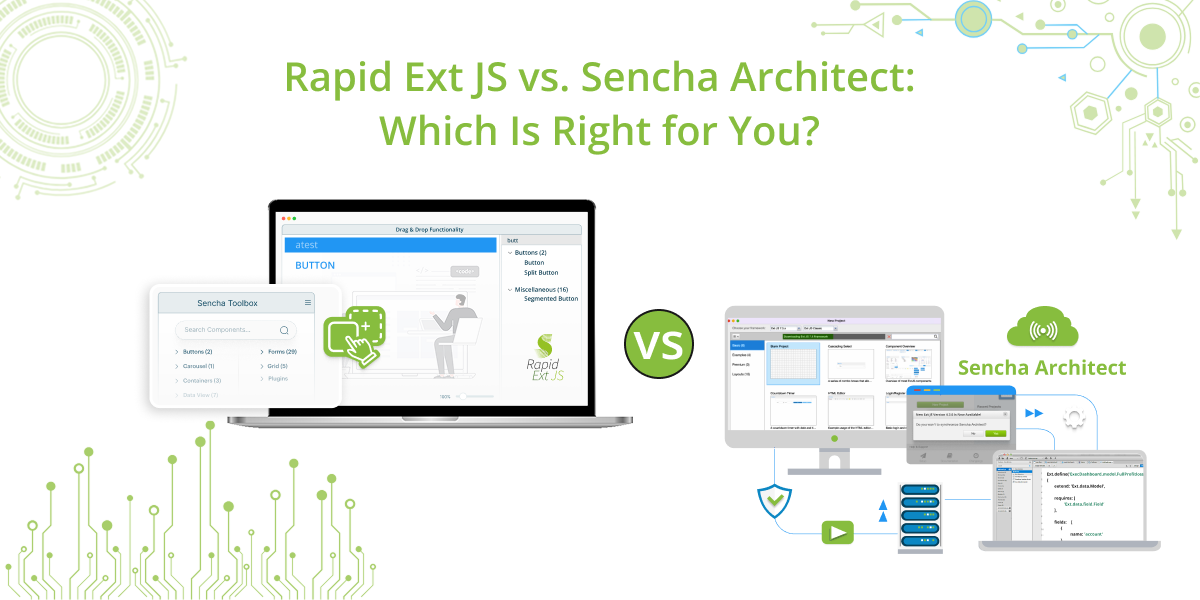
When it comes to developing robust, enterprise-grade web applications, Sencha provides some of the most…
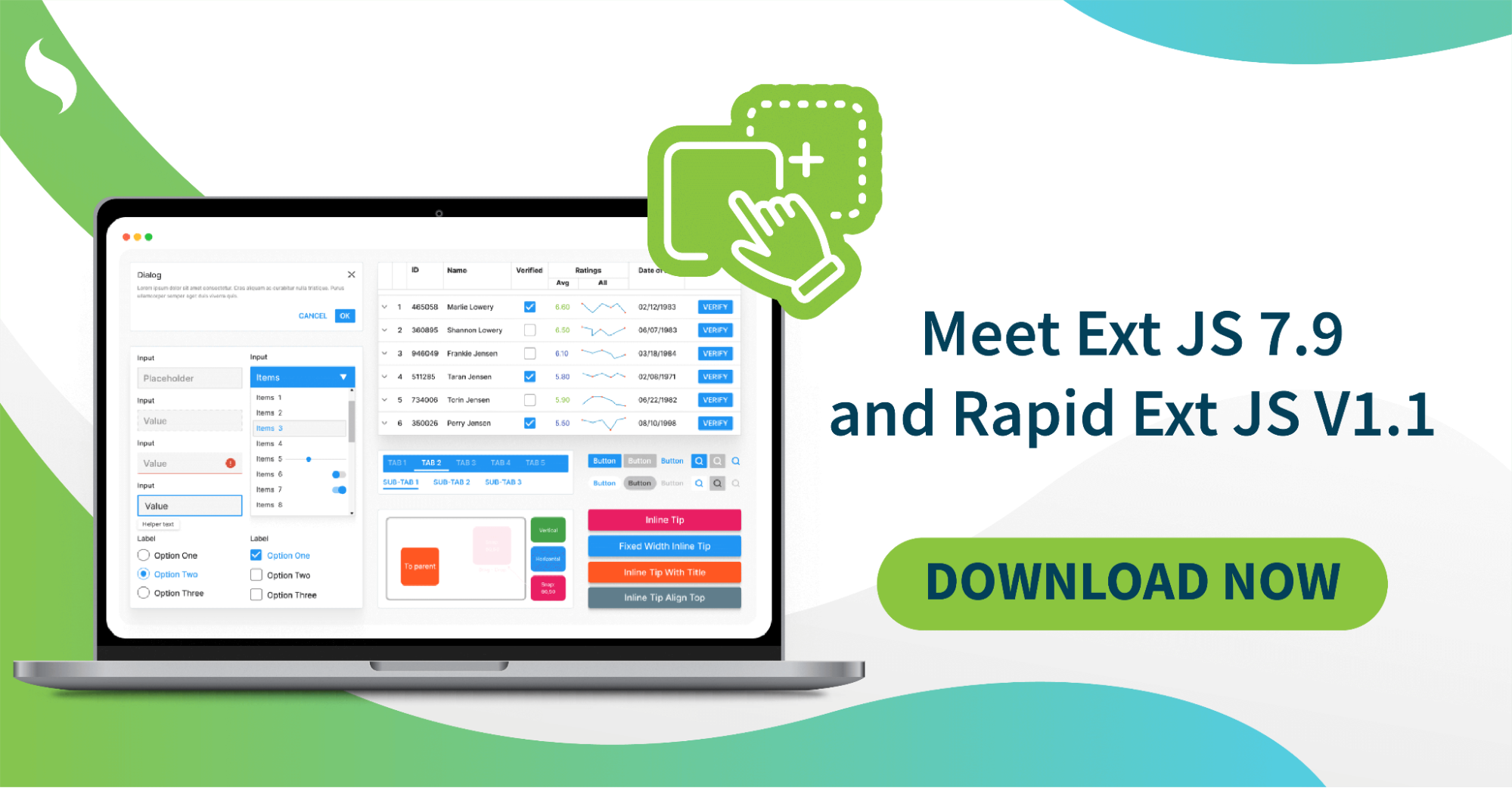
The Sencha team is excited to announce the latest Ext JS version 7.9 and Rapid…