Hidden JavaScript Concepts That Will Make You A Better Programmer in 2024
JavaScript is one of the most important programming languages of web development. It helps us achieve interactive and engaging websites for our users and customers. JS frameworks are everywhere, whether you’re working on the front end or the back end. But here’s the thing: Do you know all the cool tricks JavaScript can do? Are you using it to the max? In 2024, keeping up with the latest JavaScript Framework concepts is key to being a top-notch programmer.
Let’s break it down. This blog is all about showing you the hidden sides of JavaScript. We’re discussing the concepts that can level up your coding skills and make you a pro. In the fast world of JavaScript, staying updated is a must. Therefore, we have written this blog for our fellow developers. Let’s get started!
What Are ECMAScript 2024 Updates?
In 2024, JavaScript got a major upgrade with ECMAScript. It brought in new features that made life easier for developers. Let’s dig into these changes.
First up, we got pattern matching. It makes complex logic way simpler. Then, there are record types, making how we organize data more straightforward. And then, there comes an improved syntax, making our code-writing smoother and easier.
So, why do these changes matter? Well, they boost how fast we work. With pattern matching and record types, our code becomes cleaner. Hence, saving us time and avoiding mistakes. The better syntax isn’t just a fancy thing. It means even beginners can code more easily.
ECMAScript 2024 helps us write code that’s clear as day. And because the code is cleaner, it runs better. That means our programs are more efficient and speedy.
There are three more new things:
1. Well-Formed Unicode Strings
This handles special strings with specific characters. Two new tools are added:
`String.prototype.isWellFormed`
It checks if a string has those special characters.
const str1 = "ab\uD800";
const str2 = "abc";
console.log(str1.isWellFormed()); //false
console.log(str2.isWellFormed()); //true
`String.prototype.toWellFormed`
It changes the string to replace those special characters.
const str1 = "ab\uD800";
const str2 = "abc";
console.log(str1.toWellFormed()); //ab�
console.log(str2.toWellFormed()); // abc
2. Regular Expression v Flag with Set Notation and Properties of Strings
Now, when using regular expressions, there’s a new feature called the `v` flag. It helps with special characters and makes matching letters easier.
3. Atomics.waitAsync
This is a cool method that waits for something in the computer memory. It’s now faster and doesn’t stop other things from happening.
const arrayBuffer = new SharedArrayBuffer(1024);
const arr = new Int32Array(arrayBuffer);
// Wait for position 0 in the memory, expecting a result of 0, waiting for 500 ms
Atomics.waitAsync(arr, 0 , 0 , 500); // { async: true, value: Promise {<pending>}}
// Notify wakes up the memory, and the waiting is done
Atomics.notify(arr, 0); // { async: true, value: Promise {<fulfilled>: 'ok'} }
What is Advanced Asynchronous Programming?
Advanced Asynchronous Programming goes deep into how we handle tasks that don’t happen one after the other in JavaScript. We look closely at Promises and async/await. It helps us make sure we understand them well and avoid common mistakes like callback hell.
This isn’t just theory; we bring it to real life by using examples from everyday coding. We learn how to deal with errors properly and make our code more reliable and smooth.
But it doesn’t stop there. We also get into something called reactive programming using libraries like RxJS. This is like a supercharged way of handling tasks that are not straightforward. It makes our code more responsive and easier to manage.
The best part? We see all this in action with real examples. We figure out how to use these advanced techniques to make our code work better, especially in big and complicated programs. So, Advanced Asynchronous Programming isn’t just for the experts; it’s for anyone who wants to write code that’s powerful and efficient.
What is Meta Programming?
Meta Programming in JavaScript means looking at how our code can understand and change itself. We’ll focus on two things: proxies and reflections, which make our code more dynamic.
Proxies work like helpers. They let us control how our objects behave. For example, we can make an object change its actions based on certain conditions. For example, accessing properties or calling methods.
Reflection is like our code looking in the mirror while it’s running. It allows our code to peek at itself and even change things on the spot. This helps us better grasp how our code works.
In the real world, metaprogramming solves tricky problems. Let’s look at some simple examples:
👉Using proxies, we can make an object behave differently depending on what’s happening in our program.
👉If we’re building a set of tools for others to use, metaprogramming helps us create tools that can handle different jobs without needing constant updates.
👉When dealing with user input, metaprogramming can help ensure the data follows specific rules. It’s like having a filter that checks and fixes the data before storing it, preventing issues later on.
What Are the Different Memory Management Techniques?
In JavaScript, memory management involves two main things.
✔️First, there’s “Garbage Collection,” which means JavaScript automatically cleans up memory that’s not being used anymore. This stops the unnecessary data from piling up and keeps things running smoothly.
✔️Second is “Memory Leaks,” which happen when the memory isn’t let go of properly. This can make your program slow down over time or, in some cases, cause it to crash. So, it’s important to know about these things to make sure your JavaScript applications work well and don’t run into problems with memory.
Optimizing Memory Usage
- Use memory wisely to make applications faster.
- Avoid using more memory than needed.
- Choose efficient ways to store and manage data.
Tips and Tools for Fixing Memory Issues
- Check your code regularly for potential memory problems.
- Use debugging tools to find and solve issues.
- Keep an eye on memory use with monitoring and logging.
- Follow good practices like limiting global variables.
- Test your code to make sure it uses memory well.
What Are the Functional Programming Patterns?
Functional programming in JavaScript involves using higher-order functions, which treat functions like regular variables. This helps keep code organized and easy to reuse. Let’s explore them.
✔️Closures are functions that remember where they were created. They’re useful for keeping data private and organized.
✔️Currying is like breaking down a function with many parts into smaller, simpler functions. It makes code more flexible.
✔️Immutability means once you create data, you can’t change it. This makes code more predictable and helps prevent bugs.
What is WebAssembly Integration?
WebAssembly, or Wasm, is a tech tool that makes web browsers run really fast for complex tasks. It acts like a special language that browsers understand. Hence, bringing almost-native speed to web applications when they need it most.
You can team up WebAssembly with JavaScript to get the best results. JavaScript is great for many things, but sometimes it’s not as fast as we need.
👉WebAssembly lets us write the super-fast parts of our code in languages like C or Rust and easily connect them with our web applications.
For example, you can use WebAssembly to speed up jobs like handling images or doing complicated math. By using WebAssembly, your applications can respond quicker and give users a smoother experience.
In real life, WebAssembly is a big help in areas like online gaming, video editing, or scientific simulations. This means better performance for things that need a lot of computing power right in your web browser.
What Are the Security Best Practices in JavaScript Applications?
Let’s take a look at the security best practices for JavaScript applications.
1.Cross-Site Scripting (XSS)
Scammers can inject harmful scripts into web pages. These scripts might steal info or mess with how a page looks.
✔️Solution
- Check Input Make sure the stuff users type doesn’t have bad scripts.
- CSP Headers Set rules to only allow certain trusted things on your site.
- Encode Output Change user input so that it can’t run scripts.
2.Cross-Site Request Forgery (CSRF)
Trick users into doing something they didn’t mean to on a trusted site.
✔️Solution
- Use Tokens Put special codes in forms to make sure requests are legit.
- SameSite Cookies Set rules to stop certain requests.
- Use POST Do important actions with the POST method.
Safe Coding Tips
- Look at what users type in and make sure it won’t break anything.
- Use checks on the server side too.
- Only allow trusted things on your site with CSP headers.
- Check and update these rules often.
- Add special codes to forms to check if requests are real.
- Use SameSite cookies to block unauthorized requests.
- Always use HTTPS to keep data safe when moving around.
- Use security headers like HSTS to make your app more secure.
Tools for a Strong Security Setup
- Use tools like ESLint or JSHint to find and fix possible issues in your code.
- Regularly look at and update third-party tools to fix known problems.
- Get a Web Application Firewall (WAF) to add an extra layer of safety to your app.
- Always check your code for security problems.
Also Read: JS Frameworks: JavaScript for Device Characteristic Detection
What Are the Advanced Debugging Techniques?
Herea are some advanced techniques that you must know
✔️Put breakpoints where you think the problem is. Look at variables and step through the code to find issues.
✔️Set watchpoints on things you care about. Get notified when they change to find unexpected behavior.
✔️Use the browser’s built-in tool to see what’s using a lot of CPU or memory. Fix functions using too much and make your code faster.
Tips for Debugging Complex Apps
✔️Use `async/await` to make debugging asynchronous code easier. It makes the code look simpler.
✔️Use logging and error tracking tools in production. They help find errors without bothering users.
✔️Turn on source maps in your development. It helps you debug minified or changed code.
✔️Use console logs to follow your code. Include important info like variable values to find issues quickly.
✔️Learn keyboard shortcuts for DevTools. It helps you do things faster, like going through code or checking elements.
✔️Keep learning about browser updates. They add new tools that make debugging easier.
Conclusion
JavaScript is important for building interactive websites in 2024. This blog has shared some cool JavaScript tricks that can make you a better programmer. ECMAScript 2024 brought updates, like pattern matching and improved syntax, making your code cleaner and easier. These changes help you work faster and avoid mistakes.
Understanding advanced topics like asynchronous programming, metaprogramming, and memory management can make your code more powerful and reliable. Functional programming patterns and WebAssembly integration also add useful tools to your programming toolbox.
For security, watch out for Cross-Site Scripting (XSS) and Cross-Site Request Forgery (CSRF). Advanced debugging techniques can help you find and fix problems in your code.Remember, keeping up with the latest JavaScript concepts is key to being a top-notch programmer in 2024. Happy coding!
FAQs
What Makes JavaScript Pivotal in 2024?
JavaScript’s pivotal role in 2024 lies in enabling dynamic, engaging web experiences for users.
How Can I Optimize Asynchronous Tasks?
Optimize asynchronous tasks by using async/await, managing promises efficiently, and minimizing unnecessary delays.
How Can I Manage Memory Effectively in JavaScript?
Optimize memory usage in JavaScript by choosing efficient data storage and regularly checking for issues.
Is React a JS Framework?
Yes. React JS is a simple framework. However, it lacks advanced features like Ext JS.
Sign Up at Ext JS today – Get an Enhanced JavaScript Framework for Your Business Platform.
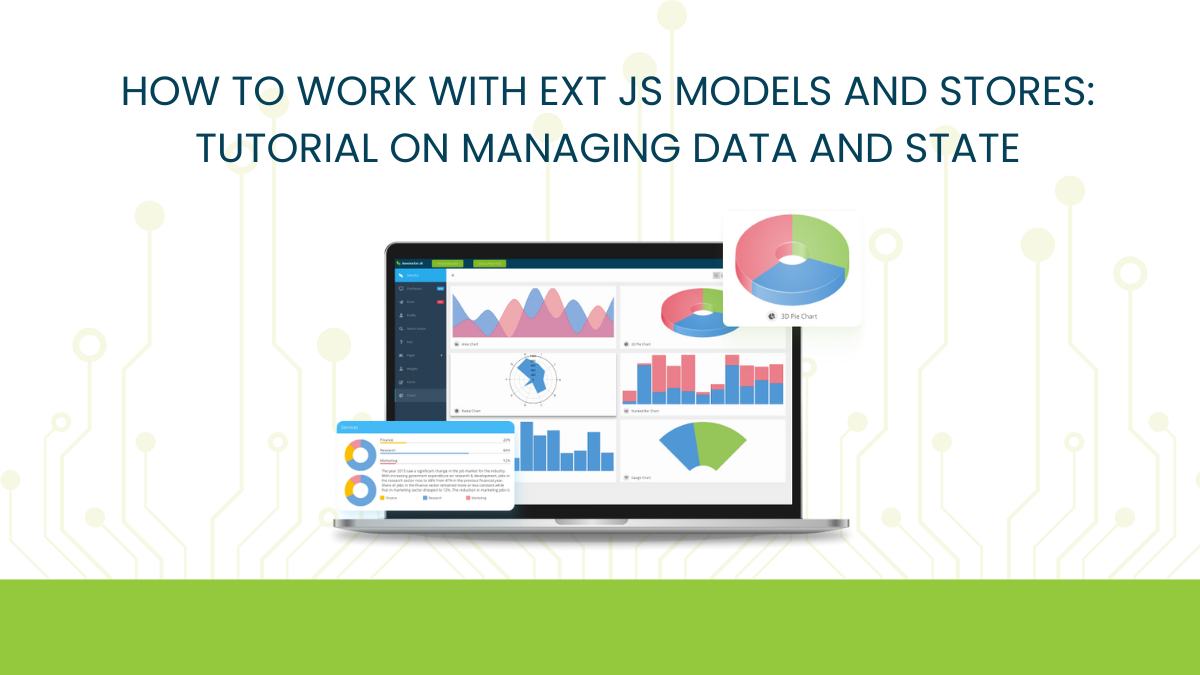
Ext JS is a popular JavaScript framework for creating robust enterprise-grade web and mobile applications.…
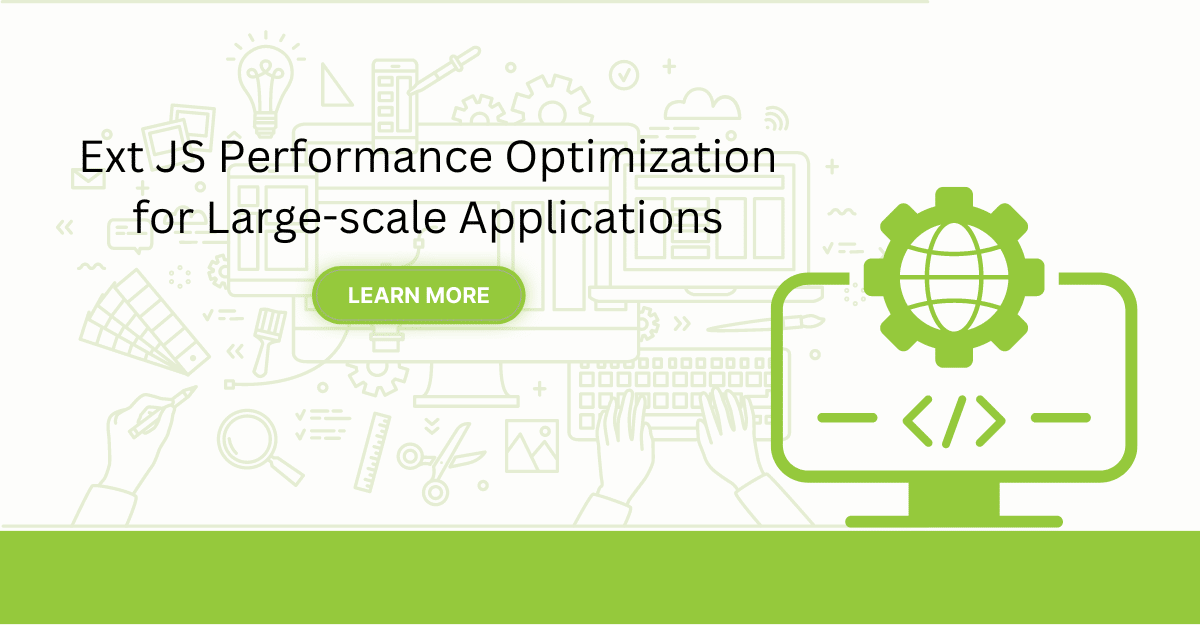
Are you facing issues with Ext JS applications’ performance as they scale up? Don’t worry!…
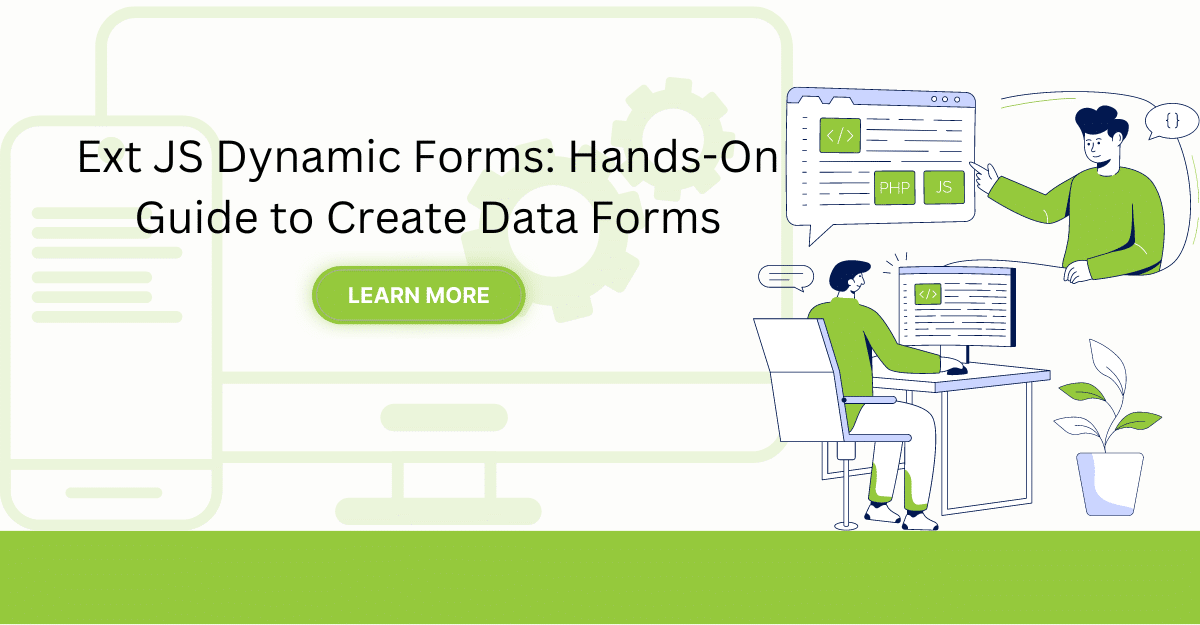
Dynamic forms are changing the online world these days. ExtJS can help you integrate such…