How To Implement MVVM Architecture in Ext JS Web Application
In today’s advanced software development world, using a software architectural or design pattern is essential. It allows developers to write clean and structured code for their apps. Moreover, implementing a design pattern makes maintenance of the app easy. On the contrary, without a software architectural pattern, you could end up with a monolithic app whose UI code would be tightly coupled with the business logic or the backend code. This makes app maintenance quite difficult and costly, especially as your app grows in size and scope and you start adding more features. Hence, most developers today use a software design pattern, such as MVVM architecture, to address these issues.
The MVVM architecture is a user interface-level design pattern that helps separate the business logic of an application from its UI (user interface) and is best suited for large and complex projects. And since the Ext JS framework is designed for creating large enterprise-level apps, it uses MVVM architecture.
In this article, we’ll discuss the basics of MVVM architecture and how you can implement it in your Ext JS web applications.
What Is MVVM Architecture?
MVVM or Model-View-ViewModel architecture is a software architecture or design pattern that cleanly and clearly separates an application’s business logic from its user interface controls. Here is how the key MVVM components work:
Model
Model classes essentially contain your app’s data. You can think of it as the app’s domain model, which typically includes a data model and business and validation logic. This MVVM component handles the abstraction of the data sources. The model works together with ViewModel to get and store data but isn’t aware of the View.
View
The View is essentially the UI of the app. Hence, it includes the presentational part/UI logic of the app that defines the layout, structure, and appearance of the components that the user interacts with. In other words, the View doesn’t contain any application logic and data. View communicates with the View Model about the user’s actions to trigger operations and is updated every time there is a change in data from the View Model.
View Model
ViewModel acts as a bridge between the View and the Model. It deals with the data relevant to the View and the UI operations we want to perform, such as business validations, updating specific parts of the website, etc. It basically manages the presenting and updating of the data for the View. The View Model is also responsible for sending data from the Model class or classes to the View.
When Should You Use MVVM Patterns?
MVVM architectural pattern is mostly used for large, complex projects where multiple developers/ team members are responsible for app development and maintenance. For small projects with simple user interfaces that don’t require extensive testing, using the MVVM pattern could be overkill. Moreover, some occasions don’t require the use of binding and implementing it on such occasions can be a burden on memory. You should enable it only when you’re expecting the data in the view model changing at run time to be updated in the view.
What Are The Benefits Of MVVM Architecture?
When used for the right project, the MVVM architectural pattern offers several benefits:
Easier and Quicker Development
When we separate the view from the application logic using the MVVM pattern, various teams can work on different components at the same time. For example, designers can work on building the UI (View) while a team of developers can focus on building the app logic (Model and View Model.) This makes app development quicker and easier.
Easy Maintenance
One of the biggest benefits of using the MVVM pattern is the way it simplifies app maintenance. When we separate different app components, the code is simpler and easier to understand for every developer. This, in turn, makes app maintenance easier. Moreover, it also makes it easier to modify the existing features and add new features to the app.
Easy Testing
Testing the UI of an app is quite challenging and time-consuming. However, with the MVVM pattern, the Model and View Model aren’t dependent on the View. This allows developers to easily write tests for these two components without the need to use the View. Moreover, unit testing of the ViewModel is easier with MVVM.
Cons of Using MVVM Patterns
- Using the MVVM pattern for small projects that requires creating simple user interfaces can be overkill.
- Since data bindings are declarative, it is sometimes difficult to debug them compared to imperative code.
Resource: View Model and Data Binding
What is the Difference Between MVC and MVVM?
Here are the key differences between the MVC pattern and the MVVM pattern:
- MVC is a software architectural pattern that allows us to separate an app into three key logical components, Model, View, and Controller. In contrast, the MVVM pattern makes it possible to build the user interface of an app separately from the application logic.
- The entry point to the app in MVC is the controller, whereas the entry point to the app in MVVM is the View.
- In MVC, the Controller handles the user input, whereas, in MVVM, the view takes the user input.
- In MVC, there are ‘one to many’ relationships between Controller and View. On the other hand, MVVM has ‘one to many’ relationships between View and View Model.
What Is Ext JS?
Ext JS is a robust front-end Javascript framework used by over 100,00 enterprise customers worldwide, including Apple, Cisco, Americal Airlines, and Canon. It is a low-code solution that offers all the features you need to build highly functional and secure enterprise-level web and mobile applications.
Here are some of the key features of the Ext JS framework:
High-Performance UI Components
Ext JS comes with more than 140 high-performance UI components that you can instantly use in your apps. These include buttons, icons, carousels, text fields, menus, calendars, sliders, checkboxes, and many more. All these pre-built components are fully-tested and work together flawlessly in any web app, eliminating the need to write lengthy code and debug it. Developers can also customize the components as per their project’s requirements. Ext JS also supports two-way data binding.
Blazing-Fast Grid
Ext JS comes with one of the fastest and most efficient JavaScript data grids. The robust grid can process millions of records without slowing down the app or compromising performance. Moreover, it offers a variety of sophisticated grid features, such as sorting, grouping, row expander, scrolling, drag-and-drop, and more. Ext JS also offers a wide range of charts and graphs and supports data-driven document package (D3) visualizations as well.
Cross-browser and Cross-Platform Support
With many other JS frameworks in the market, you get limited capabilities for desktops. Ext JS, on the other hand, provides a single codebase for cross-platform development. It delivers outstanding user experience across desktops, smartphones, and tablets. Moreover, all its components are tested to be used across browsers.
Highly Secure
Ext JS is undoubtedly one of the most secure frameworks in the market. And this is one of the core reasons why so many enterprises use Ext JS instead of open-source frameworks.
Kaseware, an investigative platform to combat security threats, used Ext JS to design a beautiful platform and achieve maximum security in their app development. And this is with Dorian Deligeorges, CEO & Founder of Kaseware, had to say about Ext JS:
“With Ext JS, we were able to better enforce security within our code base. If you are building an Angular app, you are constantly searching for a widget for this and a widget for that. You don’t know if they are maintained or if there is any embedded malware or nefarious code in them. Using Ext JS, we rarely had to go outside the framework, and our platform has some really complex security and functionality requirements.“
How Can I Use the MVVM Pattern with Ext JS?
Since Version 5 and above, Ext JS has been making use of the MVVM pattern. Previously it was using the MVC architecture. In addition to the features offered by the MVC, the Ext JS MVVM pattern makes it possible to manage the View separately into its own MVC pattern, also called the View Package.
Here is how the Ext JS MVVM pattern works:
1) The Model handles the interface of the application’s retrieval and data definition.
2) The View deals only with the presentational parts of the app, such as component configuration and layout.
3) The View Model is responsible for presenting and updating the data for the View.
4) There is also a View Controller consisting of the details of the event handlers and lifecycle methods activated by user input (View) and stores loading (View Model.)
Implementing the MVVM Pattern With Ext JS App
You can build a Sencha app using Sencha Cmd, which is essentially a productivity and performance optimization tool for building Ext JS apps. To learn more about building Ext JS with Sencha Cmd, check out our Getting Started Guide.
Here is how to implement the Model in Ext JS:
Ext.define('Earthquakes.model.Earthquake', {
extend: 'Ext.data.Model',
fields: [{ // redefine a field in the dataset
type: 'date',
name: 'timestamp',
dateFormat: 'c'
}],
proxy: {
type: 'ajax',
url: 'https://apis.is/earthquake/is', // access to applicaiton data
reader: {
rootProperty: 'results'
}
}
});
Here is an example code for creating Views in Ext JS:
Ext.define('Earthquakes.view.main.MainView', {
extend: 'Ext.Panel',
requires: [
'Earthquakes.view.Grid',
'Earthquakes.view.main.MainViewController',
'Earthquakes.view.main.MainViewModel'
],
controller: 'mainviewcontroller', // aliasing in MainViewController.js
viewModel: 'mainviewmodel', // aliasing in MainViewModel.js
layout: 'fit',
bind: {
title: '{earthquakestitle}' // property bound to vm in MainViewModel.js
},
items: [{
xtype: 'earthquakesgrid', // aliasing in Earthquakesgrid.js
bind: {
store: '{earthquakes}' // property bound to vm in MainViewModel.js
},
listeners: {
select: 'onSelect' // user event handler detailed in
}, // MainViewController.js
}]
});
The example code below shows how to develop the ViewModel in Ext JS:
Ext.define('Earthquakes.view.main.MainViewModel', {
extend: 'Ext.app.ViewModel',
alias: 'viewmodel.mainviewmodel', // referenced in MainView.js
requires: [
'Earthquakes.model.Earthquake'
],
data: {
earthquakestitle: 'Earthquakes In Iceland' // binding provided for View's title property
},
stores: {
earthquakes: {
type: 'store',
model: 'Earthquakes.model.Earthquake', //data access and definition
sorters: ['timestamp'],
autoLoad: true
}
}
});
Here is the sample code for the View Controller:
Ext.define('Earthquakes.view.main.MainViewController', {
extend: 'Ext.app.ViewController',
alias: 'controller.mainviewcontroller', // used to instantiate in MainView.js
requires: [
'Ext.Dialog'
],
showAlert: function(){
// dynamic reference to data from view model
let title = this.getViewModel().get('earthquakestitle');
let dialog = Ext.create({
xtype: 'dialog',
data: {
title: title // data from view model back to view
},
tpl: '<h1>{title}</h1>', // template with mark up injected with data from model
closable: true,
buttons: {
ok: function () {
dialog.destroy();
}
}
});
dialog.show();
},
// lifecycle event handler
init: function(){
this.showAlert();
},
// user event handler
// referenced in MainView.js
onSelect: function(grid, records) {
let data = records[0].data;
let time = Ext.Date.format(data.timestamp, 'F j, g:i a');
let s = 'A magnitude ' + data.size + ' earthquake occurred ' + data.humanReadableLocation + '.';
let dialog = Ext.create({
xtype: 'dialog',
title: time,
html: s,
buttons: {
ok: function () {
dialog.destroy();
}
}
});
dialog.show();
}
});
Establishing Communication between View, ViewModel, and Model:
The screenshots below show the linkage between these components of the MVVM architecture:
You can check out the Ext JS app built with the MVVM pattern here.
Also Read: Ext JS MVVM Pattern
Conclusion
MVVM is a software development design pattern that enables the separation of the application logic from its user interface. It makes app testing and maintenance simpler and easier. Moreover, it also allows us to easily add new features to our app. Hence, the MVVM pattern is best suited for large, complex apps, such as enterprise-level apps. And since Ext JS allows you to develop enterprise-grade apps, it makes use of the MVVM architecture to enable you to build high-performance apps quickly. This article shows how to implement the MVVM pattern in your Ext JS web app.
Frequently Asked Questions (FAQs)
What is MVVM architecture?
MVVM is a software design pattern that allows us to separate the application logic from its user interface.
What’s the difference between MVC vs MVVM?
MVC enables us to separate an app into three logical components, Model, View, and Controller. The MVVM pattern, on the other hand, makes it possible to build the user interface of an app independently from the application logic.
Which apps should use MVVM?
MVVM is best for large apps with complex UIs. For small apps with simpler UIs, it could be overkill.
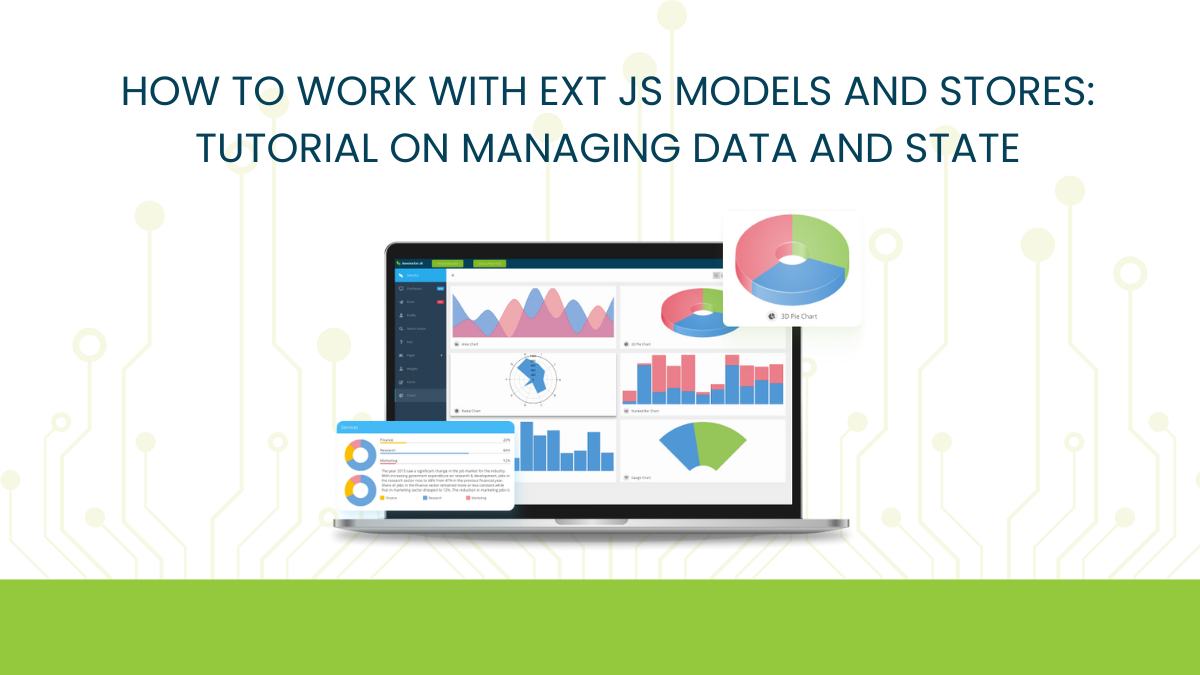
Ext JS is a popular JavaScript framework for creating robust enterprise-grade web and mobile applications.…
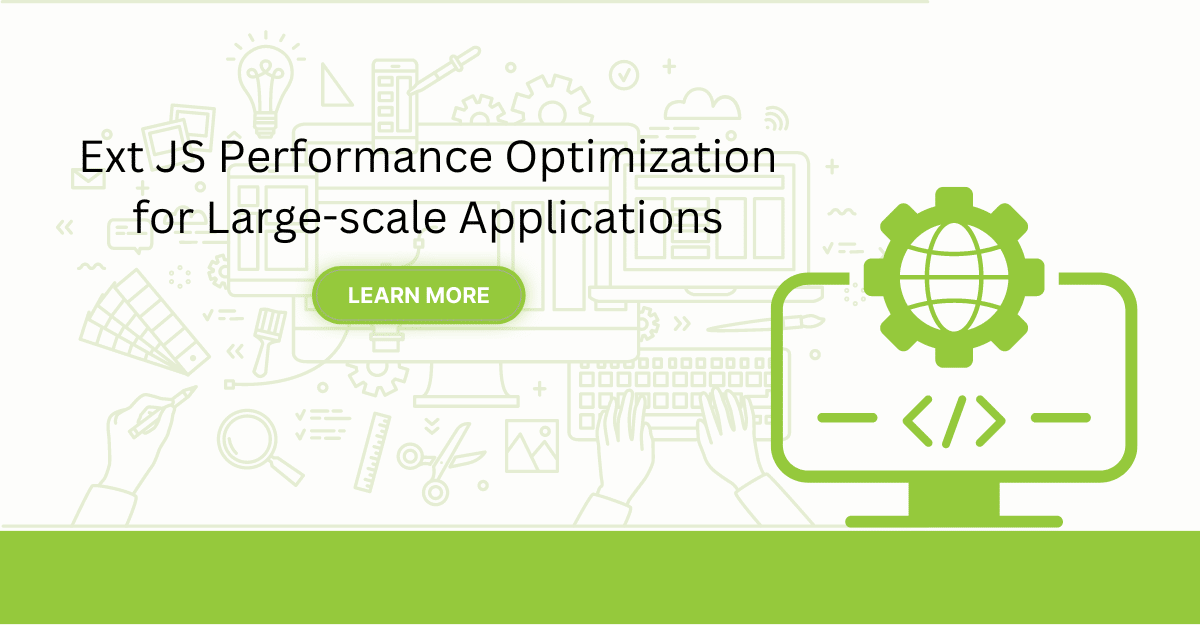
Are you facing issues with Ext JS applications’ performance as they scale up? Don’t worry!…
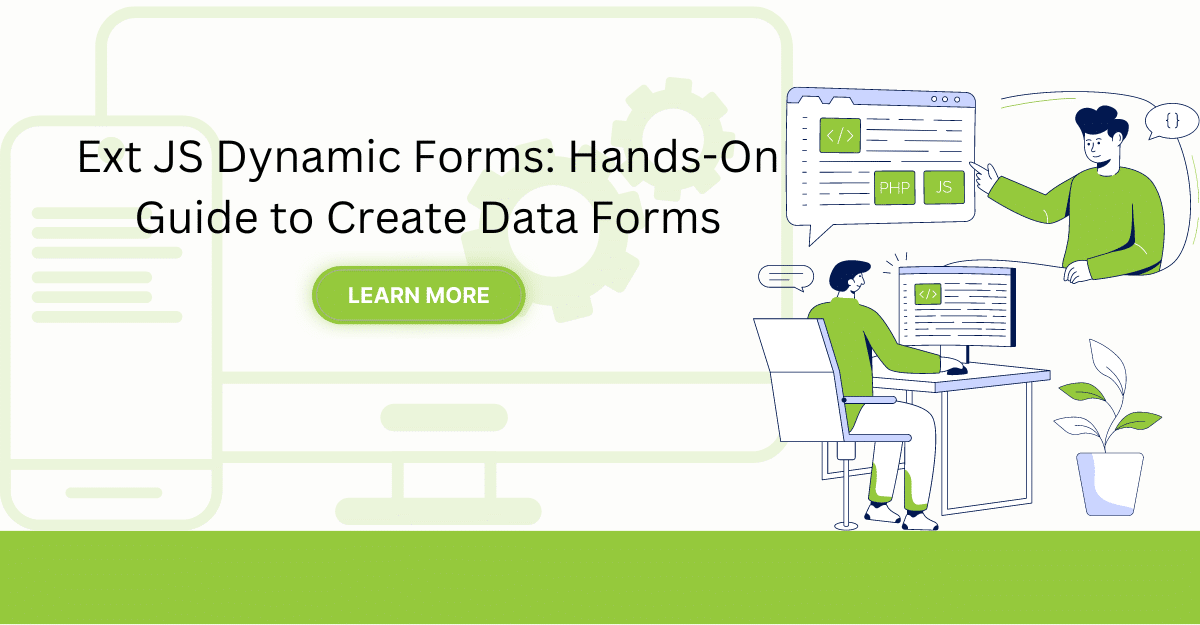
Dynamic forms are changing the online world these days. ExtJS can help you integrate such…