Javascript Grid: Cell Binding, Grid Sparklines, Charts, And More
Are you finding it difficult to organize and present massive volumes of information in your web applications? Handling row data through a JavaScript Grid or data grids has become one of the key obstacles for various developers today. At a rapid pace, more and more users are searching for applications that offer efficient data filtering and tree data structures. These applications provide insights based on data analytics. In fact, tree grid and data-centric apps can enhance the speed of making business decisions by 80%.
This blog aims to eliminate the troubles faced with data handling in JavaScript-based web applications. We will look at the mighty Sencha JavaScript Grid and compare it to other grid solutions like AG Grid. You will learn its useful features such as CSS grid implementation. You will also be able to utilize cell binding, sparklines, and charts within your grid container for seamless data presentation.
We will also demonstrate the use of data grids for managing large amounts of row data. Features include locked columns, infinite scrolling, and effective tree data structures. These will help make your applications more efficient. This blog has solutions to improve performance and the user experience, especially when managing records or presenting interactive dashboards.
How Does JavaScript Grid Work?
JavaScript Grid is used to present and administer data, offering a flexible and efficient solution for handling large datasets. The grid is a two-dimensional representation of data, where information is reorganized based on certain parameters. This allows users to perform actions like row grouping, sorting, cutting, arranging, or even editing data in real time, mimicking the functionality of Google Sheets.
The grid acquires its contents from APIs or databases, processing the information to make it usable. This data is then displayed in a container div for easy interaction. For example, actions such as row selection or sorting a column are performed instantly, without needing a page reload. The JavaScript data grid also supports column virtualization, ensuring that only the visible data is loaded, thus improving performance when handling large datasets.
Customization is a key feature, allowing users to display information in a way that suits their needs. They can hide columns, adjust row height, or even create customizable cells with specific formatting. Additionally, cell content can be tailored to meet specific requirements, offering a high degree of control. Grids can also export data to CSV or Excel formats.
Bryntum Grid is an example of a pure JavaScript grid solution that works efficiently with large datasets, using features like pagination, infinite scroll, and virtualization to enhance performance.
How to Make a Grid in JavaScript?
Making a grid with JavaScript is easy. It arranges information into rows and columns. HTML tables are used. CSS is for decoration. JavaScript enables changes when needed.
First, create the basic structure. Write the <table>, <thead>, and <tbody> tags. Rows (<tr>) will have cells (<td> or <th> for header).
Example:
<table id="dataGrid">
<thead>
<tr>
<th>Name</th>
<th>Age</th>
<th>City</th>
</tr>
</thead>
<tbody>
</tbody>
</table>
Insert this table into your document. You can load data from an API or use an array. Loop through data, create rows and append them to the table.
Example:
const data = [
{ name: "John", age: 30, city: "New York" },
{ name: "Jane", age: 25, city: "London" }
];
const tableBody = document.querySelector("#dataGrid tbody");
data.forEach(item => {
const row = `<tr><td>${item.name}</td><td>${item.age}</td><td>${item.city}</td></tr>`;
tableBody.innerHTML += row;
});
The last step is to design the grid with CSS. Add borders, padding, and other styles to improve visibility. This simple grid can later be enhanced with sorting or filtering features.
What is Ext JS Grid?
Ext JS Grid is one of the most advanced high-performance data grid controls. It is designed for efficient handling and display of large volumes of data. It works seamlessly with millions of records, making it ideal for applications dealing with huge data sets. Users can also customize font size and background color, providing a highly customizable experience.
The grid includes features like range selection, filtering, grouping, and infinite scroll, ensuring smooth and efficient data handling for users. Full customization allows users to configure the grid as needed, whether in vanilla JavaScript or other frameworks.
Data export is available in different formats, such as CSV, TSV, HTML, PDF, and XLS. This allows for easier treatment and distribution of data. The grid is cross-browser tested, ensuring accuracy and redo actions for optimal user control.
Advanced Ext JS Grid customization allows for flexible data presentation, and users can easily add UI elements. These features make Ext JS Grid a powerful tool for corporate applications, ensuring smooth communication between data and interfaces.
How Can I Do Cell Binding With the JavaScript Ext JS Grid?
Cell binding is a powerful tool in Ext JS Grid. You can use cell binding to create formulas that automatically set cell styles. As an example, take a look at this table of Flight Arrivals:
To create the table shown above, use the following code:
// x-red, x-green and x-blue are non-standard, defined dynamically at the bottom.
Ext.define('MyApp.view.Main', {
extend: 'Ext.grid.Grid',
title: 'Reykjavik Flight Arrivals',
itemConfig: {
viewModel: {
formulas: {
toolHeartIconCls: function(get) {return get('record.favorite') ? 'x-fa fa-heart x-red' : 'x-fa fa-heart';},
toCellCls: {
bind: '{record.to}',
get: function(to){
// Destination (to) is passed to this function, which can return
// a value based on the data. In this case, we're just simulating
// whether the flight is late.
return ['x-red', 'x-green', 'x-blue'][Ext.Number.randomInt(0, 2)];
}
}
}
}
},
items: [{
xtype: 'label',
docked: 'top',
margin: 4,
html: '<i class="fa fa-square x-green"></i> Early <i class="fa fa-square x-blue"></i> On-time <i class="fa fa-square x-red"></i> Late'
}],
columns: [{
text: 'Date',
xtype: 'datecolumn',
dataIndex: 'date',
format: 'F j'
}, {
xtype: 'gridcolumn',
text: 'Airline',
dataIndex: 'airline',
width: 120
}, {
text: 'To',
dataIndex: 'to',
cell: {
bind: {
cls: '{toCellCls}'
}
},
width: 160
}, {
text: 'Scheduled',
dataIndex: 'plannedDeparture',
align: 'center'
}, {
text: 'Favorite',
cell: {
tools: [{
bind: {
iconCls: '{toolHeartIconCls}'
},
handler: function(grid, context) {
context.record.set('favorite', !context.record.data.favorite);
}
}]
},
align: 'center'
}],
store: {
type: 'store',
autoLoad: true,
fields: [{name: 'date',type: 'date',dateFormat: 'j. M'}],
proxy: {
type: 'ajax',
url: 'departures.json',
reader: {rootProperty: 'results'}
}
}
});
Ext.application({
name: 'MyApp',
mainView: 'MyApp.view.Main',
launch: function(){
var css = Ext.util.CSS.createStyleSheet();
Ext.util.CSS.createRule(css, '.x-red', 'color:red !important;');
Ext.util.CSS.createRule(css, '.x-green', 'color:green !important;');
Ext.util.CSS.createRule(css, '.x-blue', 'color:blue !important;');
}
});
For another approach, you can use a view model formula to determine cell and tool CSS styles.
Take a look at this portion of the code to see how:
toCellCls: {
bind: '{record.to}',
get: function(to){
return ['x-red', 'x-green', 'x-blue'][Ext.Number.randomInt(0, 2)];
}
Here, destination (to) is passed to the function. It can return different flight statuses, including Early, On-time, and Late, which are represented by ‘x-green’, ‘x-blue’, and ‘x-red’.
You can play with the code at Sencha Fiddle.
How Can I Build Sparklines With a JavaScript Grid?
Sparklines are another useful way to present information. In Ext JS Grid, you can place Sparklines easily. Let’s take a look at this example:
Here, you can place sparklines easily by simply clicking on one of the options available in Sencha Ext JS Grid. Tour style choices include Line, Box, and Bullet. To add the same functionality to your web application, you can use this code:
Ext.define('Fiddle.view.Main', {
extend: 'Ext.Panel',
title: 'Choose a Sparkline',
padding: 16,
layout: {
type: 'vbox',
align: 'start'
},
scrollable: true,
defaults: {
margin: 8
},
referenceHolder: true,
items: [{
xtype: 'textfield',
reference: 'values',
label: 'Values',
labelWidth: 50,
value: '6,10,4,-3,7,2', // Comma-delimited numbers only!
}, {
xtype: 'segmentedbutton',
reference: 'type',
items: [
{value: 'sparklineline', text: 'Line'},
{value: 'sparklinebox', text: 'Box'},
{value: 'sparklinebullet', text: 'Bullet'},
{value: 'sparklinediscrete', text: 'Discrete'},
{value: 'sparklinepie', text: 'Pie'},
{value: 'sparklinetristate', text: 'TriState'}
],
listeners: {
change: 'up.insertSparkline'
}
},{
xtype: 'container',
reference: 'sparklines',
defaults: {
margin: 8,
padding: 8,
style: 'border: thin solid gray'
},
layout: {
type: 'hbox',
wrap: true
}
}],
insertSparkline: function (segmentedButton, value) {
const old = this.down('sparkline');
const s = this.lookup('values').getValue().replace(/\s/g, '');
const values = s.split(',').map(val => Number(val));
const config = {
xtype: 'container',
layout: 'hbox',
defaults: {
margin: 4
},
items: [{
xtype: 'label',
html: values.join(', ')
}, {
xtype: value,
values: values,
height: 25,
width: 100
}]
};
this.lookup('sparklines').add(config);
}
});
Ext.application({
name: 'Fiddle',
mainView: 'Fiddle.view.Main'
});
Want to try for yourself? You can find the source code here.
How Can I Build Powertful JavaScript Charts With Ext JS Grid?
Nothing helps you to effectively reveal important information to your users more than charts. By utilizing Ext JS Grid, you can create beautiful charts. Here is an example:
You can create the chart shown above in EXT JS Grid by using this code:
Ext.define('MyApp.view.Main', {
extend: 'Ext.Panel',
layout: {
type: 'vbox',
align: 'stretch'
},
viewModel: {
stores: {
dashboard : {type:'dashboard'}
}
},
referenceHolder: true,
items: [{
xtype: 'container',
flex: 1,
layout: {
type: 'hbox',
align: 'stretch'
},
items: [{
xtype: 'cartesian',
reference: 'barChart',
bind: '{dashboard}',
flex: 1,
interactions: ['itemhighlight'],
animation: {
easing: 'easeOut',
duration: 300
},
axes: [{
type: 'numeric',
position: 'left',
fields: 'price',
minimum: 0,
hidden: true
}, {
type: 'category',
position: 'bottom',
fields: ['name'],
label: {
fontSize: 11,
rotate: {
degrees: -45
},
renderer: 'onBarChartAxisLabelRender'
}
}],
series: [{
type: 'bar',
label: {
display: 'insideEnd',
field: 'price',
orientation: 'vertical',
textAlign: 'middle'
},
xField: 'name',
yField: 'price'
}],
listeners: {
itemhighlight: 'up.onChartHighlight'
}
}, {
// Radar chart will render information for a selected company in the list.
xtype: 'polar',
reference: 'radarChart',
flex: 1,
store: {
fields: ['Name', 'Data'],
data: [
{ 'Name': 'Price', 'Data': 100 },
{ 'Name': 'Revenue %', 'Data': 100 },
{ 'Name': 'Growth %', 'Data': 100 },
{ 'Name': 'Product %', 'Data': 100 },
{ 'Name': 'Market %', 'Data': 100 }
]
},
theme: 'Blue',
interactions: 'rotate',
insetPadding: '15 30 15 30',
axes: [{
type: 'category',
position: 'angular',
grid: true,
label: {
fontSize: 10
}
}, {
type: 'numeric',
miniumum: 0,
maximum: 100,
majorTickSteps: 5,
position: 'radial',
grid: true
}],
series: [{
type: 'radar',
xField: 'Name',
yField: 'Data',
showMarkers: true,
marker: {
radius: 4,
size: 4,
},
style: {
opacity: 0.5,
lineWidth: 0.5
}
}]
}]
}, {
xtype: 'container',
flex: 1,
layout: {
type: 'hbox',
align: 'stretch'
},
items: [{
xtype: 'grid',
reference: 'grid',
listeners: {
select: 'up.onGridSelect'
},
flex: 2,
bind: {
store: '{dashboard}'
},
columns: [{
text: 'Company',
flex: 1,
dataIndex: 'name'
}, {
text: 'Price',
width: null,
dataIndex: 'price',
formatter: 'usMoney'
}, {
text: 'Revenue',
width: null,
dataIndex: 'revenue',
renderer: 'onColumnRender'
}, {
text: 'Growth',
width: null,
dataIndex: 'growth',
renderer: 'onColumnRender',
hidden: true
}, {
text: 'Product',
width: null,
dataIndex: 'product',
renderer: 'onColumnRender',
hidden: true
}, {
text: 'Market',
width: null,
dataIndex: 'market',
renderer: 'onColumnRender',
hidden: true
}]
}]
}],
onChartHighlight: function(chart, item) {
if (item) {
var grid = this.lookup('grid');
grid.ensureVisible(item.record, {
select: true
});
}
},
onGridSelect: function(grid, records){
var record = records[0];
if (record){
this.highlightCompanyPriceBar(record);
this.updateRadarChart(record);
}
},
updateRadarChart: function(record) {
var chart = this.lookup('radarChart'),
store = chart.getStore();
store.setData([
{ Name: 'Price', Data: record.get('price') },
{ Name: 'Revenue %', Data: record.get('revenue') },
{ Name: 'Growth %', Data: record.get('growth') },
{ Name: 'Product %', Data: record.get('product') },
{ Name: 'Market %', Data: record.get('market') }
]);
},
highlightCompanyPriceBar: function(record) {
var barChart = this.lookup('barChart'),
store = barChart.getStore(),
series = barChart.getSeries()[0];
barChart.setHighlightItem(series.getItemByIndex(store.indexOf(record)));
return this;
},
});
// Mock up some data
Ext.define('KitchenSink.store.Dashboard', {
extend: 'Ext.data.Store',
alias: 'store.dashboard',
fields: [
{ name: 'name' },
{ name: 'price', type: 'float' },
{ name: 'revenue', type: 'float' },
{ name: 'growth', type: 'float' },
{ name: 'product', type: 'float' },
{ name: 'market', type: 'float' }
],
data: (function() {
var data = [
['3M Co'],
['AT&T Inc'],
['Boeing Co.'],
['Citigroup, Inc.'],
['Coca-Cola'],
['General Motors'],
['IBM'],
['Intel'],
['McDonald\'s'],
['Microsoft'],
['Verizon'],
['Wal-Mart']
],
i, l, item, rand;
for (i = 0, l = data.length, rand = Math.random; i < l; i++) {
item = data;
item[1] = Ext.util.Format.number(((rand() * 10000) >> 0) / 100, '0');
item[2] = ((rand() * 10000) >> 0) / 100;
item[3] = ((rand() * 10000) >> 0) / 100;
item[4] = ((rand() * 10000) >> 0) / 100;
item[5] = ((rand() * 10000) >> 0) / 100;
}
return data;
})()
});
Ext.application({
name: 'MyApp',
mainView: 'MyApp.view.Main'
})
You can experiment further with the code here.
What JavaScript Tips and Tricks for Grids Are There?
Locked Columns
Because there are so many ways to display data in Ext JS Grid, you are only limited by your imagination. To illustrate another one of your options, you can create locked columns easily. Here is an example:
To build this locked column, you have to use the following lines:
Ext.define('MyApp.view.Main', {
extend: 'Ext.grid.locked.Grid',
title: 'Reykjavik Flight Departures',
columns: [{
locked: true, // true, 'left' or 'right'
text: 'Airline',
dataIndex: 'airline',
width: 240
}, {
xtype: 'datecolumn',
locked: true,
text: 'Date',
dataIndex: 'date',
format: 'M j, Y',
width: 120
}, {
text: 'To',
dataIndex: 'to',
width: 200
}, {
text: 'Scheduled',
dataIndex: 'plannedDeparture',
align: 'center'
}, {
text: 'Status',
dataIndex: 'realDeparture',
width: 200
}, {
text: 'Summary',
tpl: 'On {date:date("F j")}, <tpl if="airline">{airline}<tpl else>a</tpl> flight to {to} is scheduled to depart at {plannedDeparture}.',
dataIndex: 'airline', // This determines the sort sequence
width: 300
}],
store: {
type: 'store',
autoLoad: true,
fields: [{name: 'date',type: 'date',dateFormat: 'j. M'}],
proxy: {
type: 'ajax',
url: 'departures.json',
reader: {rootProperty: 'results'}}
}
});
Ext.application({
name: 'MyApp',
mainView: 'MyApp.view.Main'
});
Here, in the columns that you want to lock, you must set locked to true
looking for the source code. Find it here.
How Can I Build Infinite Scrolling JavaScript Grids?
Where you have a large amount of data to display, you may want to try Infinite Scrolling. In Ext JS Grid, Infinite Scrolling lets your users scroll through thousands of records smoothly. Here is an example:
You can implement Infinite Scrolling functionality in your web application using the following code example:
Ext.define('MyApp.view.Main', {
extend: 'Ext.grid.Grid',
title: 'Businesses',
columns: [
{ xtype: 'rownumberer', width: 55},
{ text: 'Name', dataIndex: 'name', flex : 2},
{ text: 'Address', dataIndex: 'full_address', flex : 3 },
{ text: 'City', dataIndex: 'city', flex: 1 }
],
store: {
type: 'virtual',
pageSize: 200,
proxy : { type : 'ajax', url : '//nameless-tundra-27404.herokuapp.com/go/?fn=bigdata', reader : { type : 'json', rootProperty : 'data' } },
autoLoad: true
}
});
Ext.application({
name: 'MyApp',
mainView: 'MyApp.view.Main'
});
Play around more with the code here.
Where Can I Get This Powerful JavaScript Grid?
As you can see, Sencha JavaScript framework gives you the power to efficiently display data to the users through charts and sparklines. Also, it lets you leverage other useful functionality like Locked Columns and Infinite Scrolling. If you want to find out more check out the Ext JS Grid documentation.
Sencha Ext JS is a powerful JavaScript library for building interactive web applications. Try it now for free and create your own data-intensive web apps.
Conclusion
JavaScript Grids, particularly the Sencha Ext JS Grid, are effective tools. They assist in managing and displaying large volumes of data. They do so in an organized way. Cell binding, sparklines, and charts enhance data presentation. These features make the grid easier to use.
The scope of customization is immense. You can use locked columns and infinite scrolling. These features make data handling simpler. The option to export data in various formats adds flexibility.
Sencha Ext JS Grid is ideal for modern applications. It increases productivity and improves processes. This helps developers tackle common challenges. It leads to faster decision-making and better data handling.
Sencha Ext JS Grid is an impressive product. It is designed for complex web application development. With these advanced features, managing large datasets becomes easier.
FAQs
What Is a Grid Control in JavaScript?
Grid control in JavaScript organizes data into rows and columns for display.
What Is the Best JavaScript Grid?
Sencha Ext JS is one of the best JavaScript grids for large datasets.
How Do You Create a Data Grid in JavaScript?
Create data grids using HTML tables, CSS, and JavaScript functions.
How to Print a Grid in JavaScript?
Use JavaScript’s window.print() method to print grids in the browser.
Sign Up for free at Ext JS to use the best JavaScript grids for your data-intensive applications.
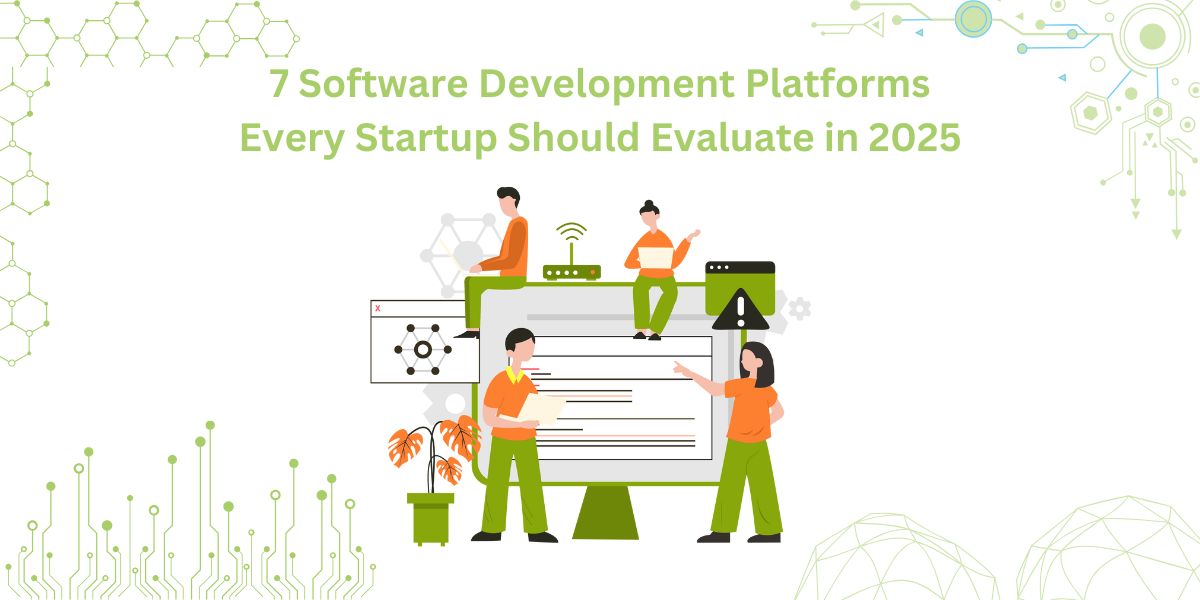
Did you know that nearly 90% of startups fail? And one of the biggest reasons…
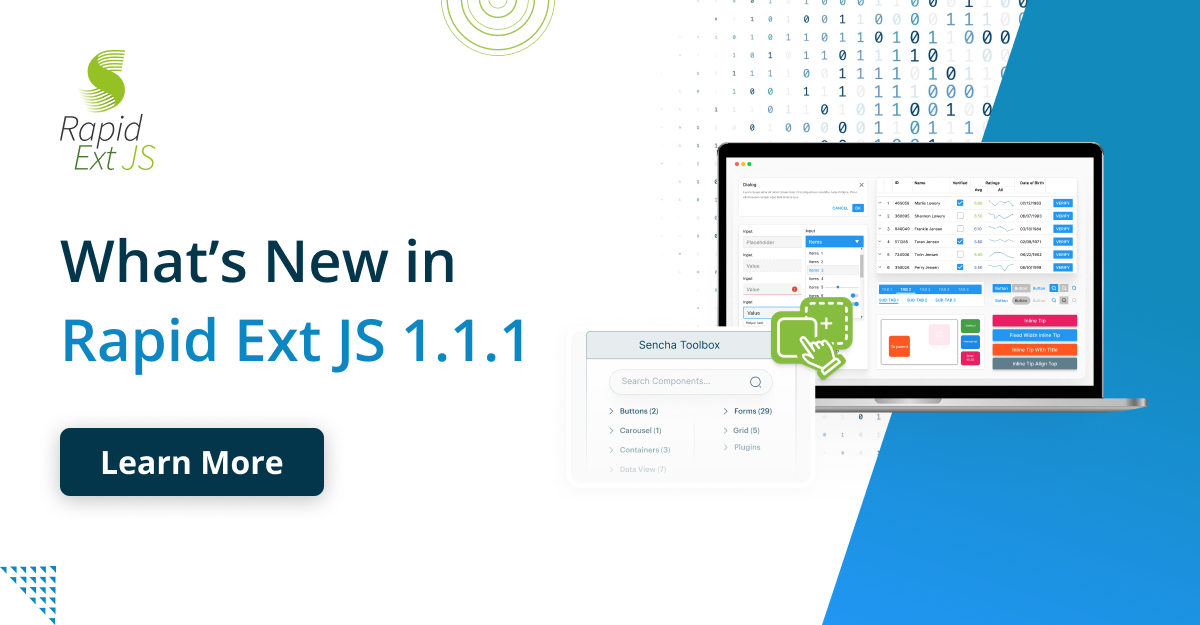
The Sencha team is excited to announce the latest Sencha Rapid Ext JS 1.1.1 release…
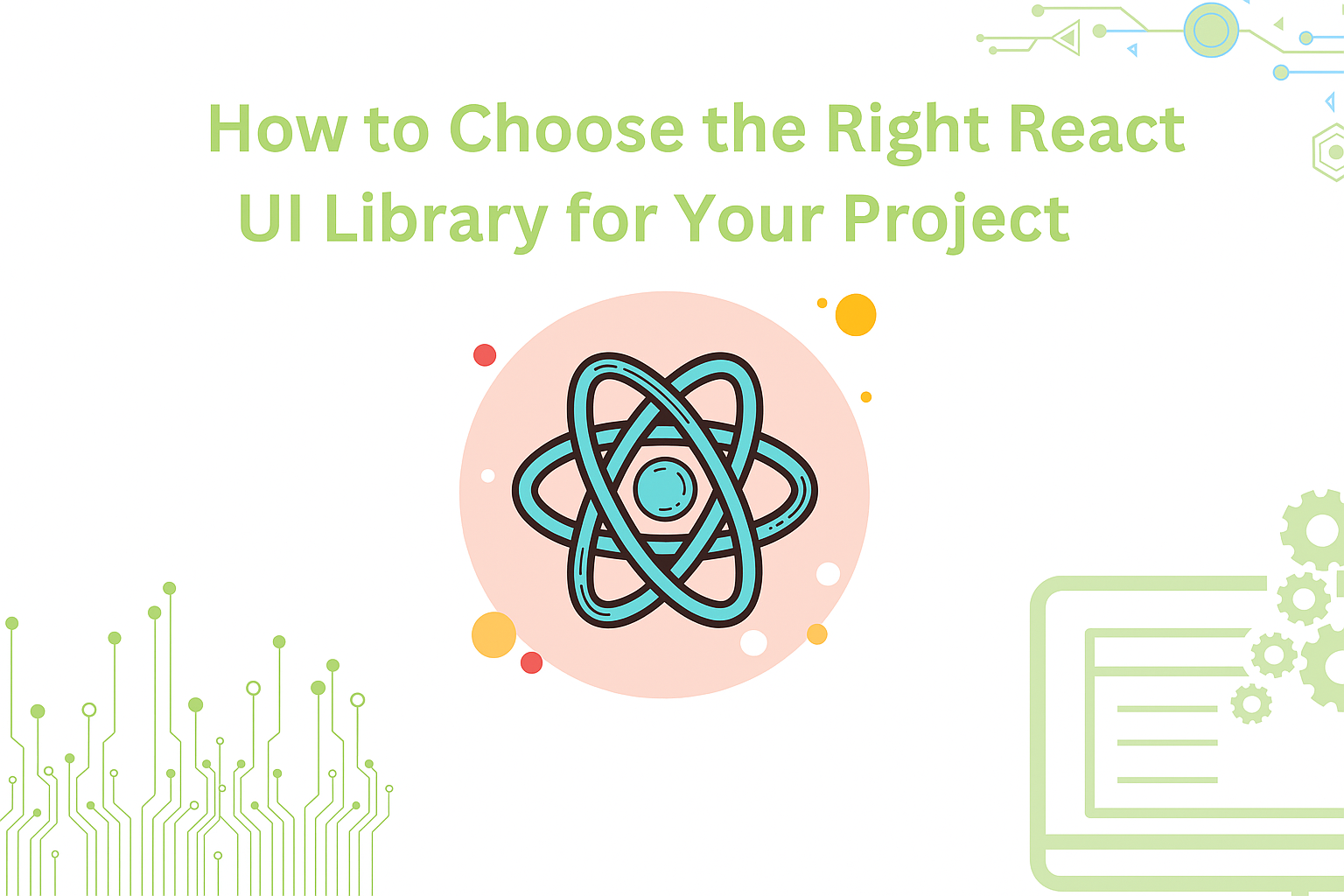
React is perhaps the most widely used web app-building framework right now. Many developers also…