Cross-Platform Application Development with Ext JS
As the need to create applications that cater to different devices and operating systems is increasing, cross-platform app development is becoming immensely popular. It saves development time and resources and provides access to a broader audience base, enhancing brand visibility. But cross-platform app development has its own set of challenges and hurdles. For instance, you must ensure your app can adapt to various screen sizes seamlessly. Moreover, different platforms have their own ecosystem (UI patterns, capabilities, and design guidelines). Thus, the app’s performance can vary from platform to platform. And providing a native-like user experience on various devices and platforms (Android, iOS, Windows, etc.) can be challenging. This is where JS frameworks designed for cross-platform app development come in handy.
Ext JS is an example of such a framework. Ext JS is a leading JavaScript framework for creating highly functional web and mobile applications. The efficient framework is designed to facilitate cross-platform development. For instance, it offers features like 140+ high-performance components, responsive design capabilities, an efficient layout manager, theming and styling options, two-way data binding, and more.
This article will discuss the basic concept of cross-platform app development and how Ext JS can help with it. We’ll explore the core concepts and features of Ext JS, implementing cross-platform app development with Ext JS, and more.
Getting Started with Ext JS for Cross-Platform Development
Understanding Cross-Platform Development Fundamentals
People today use different devices with different operating systems (Android, iOS, Windows, etc). Cross-platform development ensures your app works flawlessly on all these devices and platforms without having to create the app for each platform from scratch. With cross-platform app development, developers need to write the app’s source code once. They can then compile it for multiple platforms. Developers often rely on cross-platform JS or JavaScript frameworks and tools to further accelerate the app development process.
Thus, cross-platform development saves costs, time, and resources. Moreover, developing web applications that work across platforms gives you access to a broader use base, increasing brand visibility.
Setting Up Your Development Environment
Download Ext JS
The first step to getting started with Ext JS is downloading it from Sencha’s website ( https://www.sencha.com). You can choose the community edition for free or start with the trial version.
Extract the Ext JS zip file
Next, unzip the Ext JS trial version zip file, which contains various JavaScript and CSS files, such as:
- Ext.js
- Ext-all.js
- Ext-all-debug.js
- Ext-all-dev.js
- Multiple theme-based CSS files
Include the Ext JS library
You must include the Ext JS library in your project to create a Sench app. You can include the library locally by downloading it. Or you can use a CDN instead, which eliminates the need to download the Ext JS library:
<script src="https://cdn.sencha.com/ext-7.0.0/classic/ext-all.js"></script>
<link rel="stylesheet" type="text/css" href="https://cdn.sencha.com/ext-7.0.0/classic/theme-triton/resources/theme-triton-all.css">
Installing a Code Editor
Next, you’ll need a code editor, like Notepad, Notepad++, or Visual Studio, to write the app’s code.
Installing Sencha cmd
You can also use Sencha cmd, a command line tool designed specifically to create Ext JS applications. To create an Ext app with Sencha cmd, simply open your terminal or command window and change the directory to the folder where you wish to create the app:
cd <folder-to-create-the-app>
Next, generate your app:
sencha generate app modern -ext MyApp ./MyApp
Go to the new app folder:
Navigate to the new application folder
Run the app:
sencha app watch
Building a Foundation: Ext JS Essentials
Understanding Ext JS core concepts, such as components, data stores, the layout system, and events, is the key to mastering cross-platform web development with the framework.
Components and Their Role in Cross-Platform Development
In Ext JS, Components are the main building blocks for creating the UI (user interface). They are essentially individual parts of the UI. These components can be grids, menus, text fields, panels, buttons, charts, etc. Each component in the UI has its own functionality and appearance. However, all the components inherit from the main Ext.Component class. This means these components are a subclass of the Ext.Component class.
Another Ext JS core concept to learn here is containers. These are special Components where we can add other components/child components or even other containers.
Pre-built Components
Ext JS provides an extensive range of pre-built UI components to create cross-platform web applications. These include icons, dropdown menus, buttons, carousels, tabs, panels, forms, etc. All these responsive components are designed to work consistently across devices and platforms. These components can efficiently adapt to various screen sizes and orientations. Hence, they give your app a native look and feel on various devices.
Moreover, Ext JS abstracts several platform-specific features and capabilities. Thus, it allows you to write code only once that works seamlessly across various operating systems and browsers.
Layouts and Responsive Design in Ext JS
Ext JS has a powerful layout system that enables efficient management of the UI components’ layout. It enables users to ensure that all the components are responsive and displayed correctly on different devices, screen sizes and orientations. For instance, you can use Ext JS layouts to define the child components’ size and how they need to be positioned in a container.
Utilizing Ext JS Store for Data Management
Data Stores and Models in the Ext JS framework allow for seamless data management in your apps. These two classes play a pivotal role in handling data and interactions with the server, as most of your app’s data is sent, retrieved, organized, and modelled using these classes.
Data Models are used to create the structure of a specific type of data using the ‘Ext.data.Model’ class. You can define fields, associations, validations, etc. A Store is a kind of data repository for managing collections of data records of a Data Model.
The example code shows how to set up a model to use in the Store:
// Set up a model to use in our Store
Ext.define('User', {
extend: 'Ext.data.Model',
fields: [
{name: 'firstName', type: 'string'},
{name: 'lastName', type: 'string'},
{name: 'age', type: 'int'},
{name: 'eyeColor', type: 'string'}
]
});
var myStore = Ext.create('Ext.data.Store', {
model: 'User',
proxy: {
type: 'ajax',
url: '/users.json',
reader: {
type: 'json',
rootProperty: 'users'
}
},
autoLoad: true
});
Cross-Platform Development with Ext JS: Implementation
Designing User Interfaces for Multiple Platforms
Ext JS facilitates the development of responsive layouts with several layout options and components like Containers and Panels. It enables you to define the behaviour of components based on different screen sizes. For instance, you can utilize the Viewport component and ‘HBox layout’ to create a responsive design:
Ext.application({
name: 'MyApp',
launch: function () {
Ext.create('Ext.container.Viewport', {
layout: 'hbox',
items: [
{
xtype: 'panel',
title: 'Left Panel',
flex: 1,
html: 'Left panel content goes',
},
{
xtype: 'panel',
title: 'Right Panel',
flex: 2,
html: 'Right panel content goes here',
}
]
});
}
});
You can also apply platform-specific styles using CSS media queries. This will allow you to adjust styles depending on the device, screen size or platform. Here is an example code to apply different font sizes for desktop and mobile devices:
@media (max-width: 768px) {
.text {
font-size: 14px;
}
}
@media (min-width: 769px) {
.text {
font-size: 18px;
}
}
Integrating and Managing Data Across Platforms
You can define an Ext JS Store to manage data easily and fetch data from various sources. Ext JS also supports two-way data binding, automatically updating UI components as the data changes. Moreover, Ext JS offers an ‘Ext.os’ object that provides valuable information about the current platform and OS. You can use this object to detect the OS and define conditional actions depending on the detected OS:
if (Ext.os.is.Windows) {
// Windows specific code here
}
if (Ext.os.is.iOS) {
// iPad, iPod, iPhone, etc.
}
console.log("Version " + Ext.os.version);
Handling Cross-Platform Compatibility Challenges
Since different platforms can have their own browsers, platform-specific features and capabilities, you may encounter cross-platform compatibility issues. Here is how to address common issues:
- Use feature detection, shims, and polyfills to resolve cross-browser compatibility issues.
- Continuously monitor platform-specific issues and update your code accordingly by releasing platform updates.
- Use conditional code for different platforms to address platform-specific functionalities.
- Test your app extensively across devices and platforms and resolve any detected issues.
Advanced Techniques for Cross-Platform Success
Optimizing Performance in Cross-Platform Apps
One of the most effective ways to optimize the performance of your cross-platform web application is to minimize DOM manipulations. You can utilize Ext JS component-based architecture, data binding, and templates to update the UI effectively.
You can also make use of Ext JS virtual rendering/data buffering technique for lists and grids. Virtual rendering renders only the visible part of the data in the viewport. Hence, it significantly reduces the number of DOM elements created. Moroever, you can implement code-splitting to load only the necessary JavaScript modules and improve the loading times of your app. Continuous testing and profiling are also essential for optimizing your app’s performance.
Implementing Custom Components and Widgets
Ext JS allows you to create custom components depending on your specific project requirements. These components can handle complex UIs and component behavior. Moreover, they can contain third-party integrations. You can also utilize Ext JS templates to create dynamic HTML content in your custom components.
Extending Functionality with Plugins and Extensions
Plugins allow you to extend the functionality of existing Ext JS components or add new features to the components without the need to modify their core code. You can also utilize tested third-party extensions and plugins provided by the Ext JS community.
Debugging Strategies for Cross-Platform Apps
Cross-Platform Testing Best Practices
- Check for issues and errors related to your app’s core logic. These issues and inconsistencies can occur on all platforms.
- Once you’ve resolved platform-agnostic issues, test your app for platform-specific issues.
- Test your app on real devices with different OS (iOS, Android, Windows, etc.)
- Utilize testing tools like Sauce Labs and BrowserStack that provide access to a number of devices, platforms and browsers for testing.
- Release the beta version of your app to test it with real users. Gather user feedback and improve your app’s performance accordingly.
Debugging Techniques and Tools in Ext JS
Sencha Inspector
Sencha Inspector is a debugging tool built specially for Ext JS applications. It offers direct access to components, variables, objects, etc. Using Sencha Inspector, you can assess your app’s code for issues like overnesting of components, the number of layout runs, etc.
Chrome and Firefox DevTools
Chrome and Firefox offer powerful built-in debugging and profiling tools to examine your app’s performance. For instance, you can analyze Chrome’s memory and CPU usage or Firefox’s performance profiler to assess and improve your app’s performance.
Visual Studio Code Extensions
Visual Studio Code offers extensions that support Ext JS development. These extensions can help write, test, and debug your Ext JS code directly within the Visual Studio code editor.
Conclusion
In today’s ever-evolving app development landscape, developing web applications that work flawlessly across browsers, devices, and platforms is essential. Cross-platform app development saves time and resources by enabling developers to write the app code once and then compile it for various platforms. Developers usually utilize cross-platform JS frameworks to create apps for various platforms quickly. A JavaScript framework helps accelerate cross-platform development by providing features like responsive components, two-way data binding, layouts, etc. Ext JS is an example of such a framework, offering a wide range of impressive cross-platform development features.
Looking to create high-performance cross-platform apps? Try Ext JS today!
Frequently Asked Questions (FAQs)
How to deploy cross-platform apps developed using Ext JS?
You can deploy cross-platform apps built with Ext JS as web apps. You can host the app on a web server and enable access through a URL.
Can Ext JS be used for real-world applications?
Ext is one of the leading JS frameworks for building data-intensive enterprise-grade web apps. A number of real-world apps utilize the platform for creating interactive web apps.
Where to find further resources to learn Ext JS for cross-platform development?
Ext JS has extensive documentation that consists of all the information about the framework’s features, functionality, and capabilities. It also has various coding examples to help you get started quickly. Sencha also has an impressive Resource Center where you can find numerous supporting videos, whitepapers, webinars, and ebooks.
What are the best JavaScript frameworks?
Examples of popular JavaScript libraries and JS frameworks include Ext JS, React and Angular. Ext JS is a cross-platform framework for developing data-intensive mobile and web apps. React is an open-source JavaScript framework known for features like component-based architecture and virtual DOM/virtual document object model. Angular is another open-source JavaScript library for building single-page applications.
The grid components have been rewritten from the ground up for Ext JS 4 and…
Although most modern mobile devices have good hardware specs, almost all of them are still…
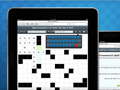
We've been interviewing the developers that created the amazing apps for our most recent Sencha…