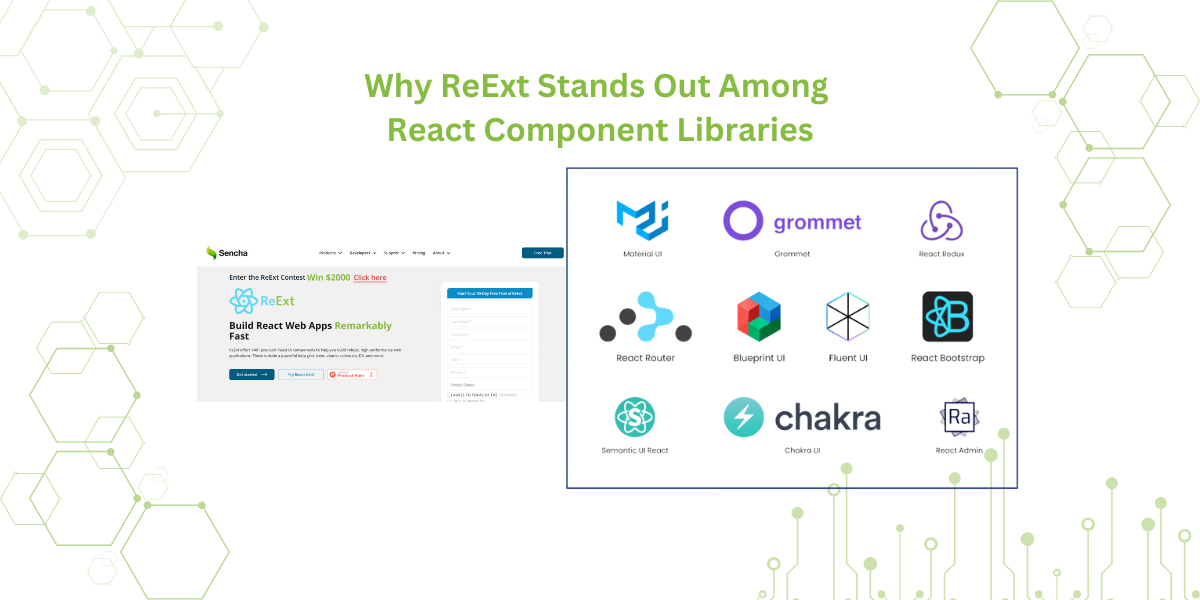
When it comes to React development, developers must make many decisions. One of the most important decisions involves choosing a component library. This decision significantly influences how the application grows and functions. Among the many options, ReExt stands out as…
Subscribe to our newsletter
Be the first to learn about new Sencha resources and tips.