ES7 JS Frameworks | Their Role in Asynchronous Programming
Have you ever been confused when dealing with asynchronous tasks in JS frameworks? Fear not! Our blog guides you through the JavaScript framework ES7 features. You must know that this blog will simplify the complexities of asynchronous programming. Suppose you’re working on your JavaScript project. At the same time, you are fetching data from the internet, and suddenly, your code becomes a mess. This is where ES7 steps in to make your life easier.
Why does asynchronous programming matter so much in today’s JavaScript framework development? What challenges do developers often face? We’ll answer these questions in this blog. At the same time, we will also explore the significance of asynchronous programming in the modern coding landscape. Get ready for a journey into the heart of ES7’s new features.
Let’s begin reading this article till the end!
What is Asynchronous Programming?
Asynchronous programming is like doing multiple things at once in our code. Instead of waiting for one task to finish before starting the next, it lets the program keep going with other jobs. This is super important when dealing with tasks that take time. One such common example is fetching data from the internet.
But, making code asynchronous can also be challenging for developers. Unlike the usual way of writing code, things happen one after the other. Understanding and fixing issues in asynchronous code can be tough.
Despite these challenges, asynchronous programming makes software work better for users.
Suppose using an app that doesn’t freeze every time it’s doing something in the background. That’s the magic of asynchronous programming. It keeps the app responsive and smooth. As a result, it also helps us give users a better experience.
How Did Asynchronous Features Evolved in JavaScript?
JavaScript’s journey with asynchronous features has evolved significantly over the years. In the early days, JavaScript primarily ran in browsers and was mainly used for simple tasks. However, as web applications became popular, the need to handle asynchronous operations arose.
In the earlier versions of JavaScript, developers relied on callbacks to handle asynchronous tasks. Callbacks, though functional, led to “callback hell” or deeply nested callback structures. This made code hard to read and maintain.
Developers gained a more structured and readable way to handle asynchronous operations. This was all possible due to the introduction of Promises in ES6. Promises helped mitigate callback hell. Moreover, it also offers a cleaner syntax and better error handling.
However, ES6 Promises still had limitations. Furthermore, developers eagerly awaited more advancements. That’s where we need ES7 JS Frameworks. Interestingly, ES7 brought the async/await syntax to JavaScript. ES7 aimed to simplify asynchronous code even further. Besides, it makes the code look and behave more like synchronous code.
Async/await made it easier for developers to write asynchronous, readable and maintainable code.
Async/Await: Simplifying Asynchronous Code
It simplifies tricky asynchronous tasks. It makes code easier to read and follow.
Here’s the trick: if you put ‘async’ before a function, it tells the computer, “Hey, there’s some async stuff happening here.” Then, when you use ‘await’ before a task, it says, “Hold on, let’s finish this before moving to the next job.”
The cool part is that it makes your code look more normal, almost like everyday step-by-step tasks. No more confusing callback mess or long chains of ‘.then()’ statements.
Suppose you’re fetching data from the internet. With Async/Await, your code looks neat and clear, showing each step in order. This simplicity is incredible, especially when dealing with tricky things like
- Handling user logins
- Communicating to multiple parts of a website at once.
How Do You Achieve Improved Error Handling with ES7?
Handling errors in asynchronous code can be a challenging task. However, we can achieve it using ES7. You must know that errors in the ASYNC code can be hard to catch and understand.
ES7 introduces the `async/await` syntax we talked about earlier. This makes writing async code easier and improves how errors are handled. With `async/await`, you can use traditional `try` and `catch` blocks like in regular synchronous code. This means you can spot and handle errors more efficiently.
For better error management, ES7 also brings in the `Promise.catch()` method. This method is like a safety net for errors in Promises. It allows you to catch errors at the end of a chain of promises.
When dealing with ES7 and async code, best practices include using `try` and `catch` blocks around your asynchronous operations. This ensures you can identify and handle errors in a more organized way. Also, use the `Promise.catch()` method to capture any overlooked errors.
How Do You Simplify Parallel Execution with ES7?
Parallel execution is a big deal for making programs run faster. ES7 has some amazing features that make handling parallel tasks easier and more effective.
In simple terms, when discussing parallelism, we mean doing tasks simultaneously to boost performance. ES7 brings in the `async/await` syntax we’ve discussed.
This isn’t just for making code readable – it’s also a powerhouse for parallel execution.
`async/await`, can help us handle multiple tasks without making your code a confusing mess. It lets you start a task, pause, switch to another, and return when the first one’s done.
Let’s suppose that there is a web page that needs to load images, fetch user data, and get some news – all at the same time. ES7 makes this easy with its async capabilities. You start each task and await the results without locking up your program.
Also Read: How to Add a JavaScript Pivot Grid to Your Web Application
How Do You Apply ES7 Features in Web Development?
ES7 JS frameworks also helps enhance both performance and user experiences. The implications of ES7 features on web development are profound.
Regarding user experiences, ES7 JS frameworks contribute to smoother and more responsive web applications. Developers can create interactive and quick interfaces, even when performing resource-intensive tasks in the background. This directly translates to improved user satisfaction and engagement.
Real-world case studies showcase the successful integration of ES7 in web applications. Let’s take an example. Consider an e-commerce website that utilizes async/await to:
- Manage product updates
- Shopping cart interactions
- User authentication efficiently.
The result is a seamless and enjoyable shopping experience for users. The website responds quickly to their actions without delays.
How Do You Implement ES7 JS Frameworks Functions?
When implementing JavaScript functions, Ensuring efficiency and cross-browser compatibility is important. Here are code snippets and considerations to help you get it right:
function getScreenSize() {
const screenWidth = window.innerWidth || document.documentElement.clientWidth || document.body.clientWidth;
const screenHeight = window.innerHeight || document.documentElement.clientHeight || document.body.clientHeight;
return { width: screenWidth, height: screenHeight };
}
2. Retrieving Browser Information:
function getBrowserInfo() {
const userAgent = navigator.userAgent;
const browserName = userAgent.includes("Chrome") ? "Chrome" : userAgent.includes("Firefox") ? "Firefox" : "Unknown";
return { browser: browserName, userAgent: userAgent };
}
3. Determining Device Type:
function getDeviceType() {
const isMobile = /iPhone|iPad|iPod|Android/i.test(navigator.userAgent);
const deviceType = isMobile ? 'Mobile' : 'Desktop';
return deviceType;
}
4. Checking Connection Speed:
function getConnectionSpeed() {
const connection = navigator.connection || navigator.mozConnection || navigator.webkitConnection;
const speedMbps = connection ? connection.downlink / 1024 : 'Not available';
return speedMbps;
}
Cross-Browser Compatibility Considerations
- Test your code on different browsers to ensure consistent behavior.
- Use feature detection rather than relying on user-agent strings for more reliable results.
Best Practices for Efficient Implementation
- Minimize DOM manipulations and optimize code for performance.
- Use modern JavaScript features and use asynchronous operations when fetching data.
- Keep functions modular and well-documented for easier maintenance.
What Are the Future Trends in User Environment Detection?
Here are some future trends for user environment detection:
As our devices get smarter, they will have cool sensors. Websites can use these sensors to know more about how users use their devices.
Computers will get better at learning from users. They can remember what you like and how you use websites. This means websites can give you a more personalized experience.
In the future, using websites will be smooth across different devices. So, whether you’re on your computer or phone, the website will work well and look nice.
As more people use virtual reality, websites might recognize when you’re in a virtual world. This opens up new and exciting possibilities for interactive experiences on the web.
In the future, websites will focus on making sure they adapt to you without compromising your privacy.
Websites will adapt to you everywhere, no matter your device.
Websites will change in real time based on what you’re doing, improving your experience.
In the future, websites will be easier and more consistent, no matter where or what you’re using.
ES7 JS Frameworks: Conclusion
Understanding how users interact with websites is one of the most important things. We talked about why it matters to know things like the size of their screens or their internet speed. To all the developers, you have the power to make websites awesome for everyone. Use cool features like `async/await` and adapt the look of your website based on the device people are using.
Looking into the future, things are getting even more exciting. Imagine websites being super smart, knowing what you like, and working seamlessly on any device. So, developers, keep up the good work! Make websites that people love to use. The future is bright, and you’re a big part of making it awesome for everyone online. Happy Coding!
ES7 JS Frameworks: FAQs
What Is User Environment Detection in ES7 JS Frameworks Web Development?
User environment detection in web development identifies and adapts to users’ devices, browsers, and preferences.
Why Is Detecting User Environments Important?
It helps us achieve a personalized experience.
What Is the Significance of Detecting Browser Information in Web Development?
It helps us get trustable leads and engaged user interfaces.
How Does JS Frameworks Allow User Environment Detection?
A JS framework enables user environment detection by providing tools and APIs to access:
- Device information
- Screen sizes
- Browsers.
Supercharge your web projects with Sencha – Where innovation meets simplicity in UI development
The grid components have been rewritten from the ground up for Ext JS 4 and…
Although most modern mobile devices have good hardware specs, almost all of them are still…
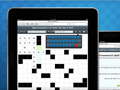
We've been interviewing the developers that created the amazing apps for our most recent Sencha…