How To Create An HTML CSS Grid
An efficient and robust Javascript data grid is vital for building a data-intensive web app. The massive volumes of data generated by small and medium enterprises call out for the need of good JavaScript developer tools that can help you build web apps capable of handling millions of data points. Ext JS meets all your requirements ranging from building a simple HTML CSS grid to a more complex and sophisticated responsive HTML 5 data grid.
Continue reading for step by step instructions on how to create an HTML5 grid. To keep things simple we are creating an app using just a single HTML file. You can put all the JavaScript code in the same file. The final JavaScript data grid we’ll build looks as follows:
Step 1: Which Files do I Need to Import to Create HTML CSS Grid?
As a first step, link the CSS stylesheet in your HTML 5 grid project. This file will import the CSS grid layout.
Create an empty HTML file and add the following line anywhere in the header of the HTML file:
<link href="https://cdnjs.cloudflare.com/ajax/libs/extjs/6.2.0/classic/theme-classic/resources/theme-classic-all.css" rel="stylesheet" type="text/css" />
Next, you need to include the Ext JS library to import the objects of the HTML CSS. Add this to the HTML file:
<script src="https://cdnjs.cloudflare.com/ajax/libs/extjs/6.2.0/ext-all.js" type="text/javascript"></script>
Step 2: How do I Create the Data Model for the HTML CSS Grid?
To set up the data grid in JavaScript, you need to define the data model with all the fields of our grid. This is defined in the onReady()
method that you can add to the script section of the HTML file.
Ext.onReady(function() { Ext.define("com.extjsGrid.Sencha", { extend: "Ext.data.Model", fields: ["Product", "Environment", "Description"] });
The above code defines a grid model com.extjsGrid.Sencha
with three data fields named Product, Environment and Description. Note this onReady()
function is not complete yet. We still have to define the data store and display method inside it.
Step 3: How do I Create the Data Store for the HTML CSS Grid?
As a third step for creating the JavaScript data grid, you need to create a data store for our HTML 5 data grid. We’ll create a variable senchaStore
that is tied to our com.extjsGrid.Sencha
data model. We’ll populate the data grid using the data
key of our JSON data store object. Append the onReady()
method with the following code.
var senchaStore = Ext.create("Ext.data.Store", { model: "com.extjsGrid.Sencha", data: [ {'Product': 'Ext JS', 'Environment': 'Javascript', 'Description':'Ext JS is the most comprehensive JavaScript framework for building feature-rich, cross-platform web applications'}, {'Product': 'React Grid', 'Environment': 'React', 'Description':'React Grid by Sencha is a perfect modern enterprise-grade grid solution for React UI that comes with 100+ amazing data grid features.'}, {'Product': 'Ext Angular', 'Environment': 'Angular', 'Description':'ExtAngular provides the most complete set of components for building data-intensive web apps using Angular.'}, {'Product': 'Ext React', 'Environment': 'React', 'Description':'ExtReact is the most complete set of React components for building data-intensive web apps using React'}, {'Product': 'ExtWebComponents', 'Environment': 'Javascript', 'Description':'ExtWebComponents provides a framework-agnostic set of over 140+ UI components for application development.'} ] });
Step 4: How do I Display the HTML 5 Grid?
You can display the HTML 5 data grid using the Ext JS panel. Add the following code after adding the data store of step 3.
Ext.create("Ext.grid.Panel", { renderTo: document.body, store: senchaStore, title: "Sencha Products", width: 1000, columns: [{ text: 'Prodcut', dataIndex: 'Product', autoSizeColumn: true }, { text: 'Environment', dataIndex: 'Environment', autoSizeColumn: true }, { text: 'Description', dataIndex: 'Description', width: 700, autoSizeColumn: true }] });
As you can see, we can individually configure each and every column of the data grid view HTML5. The dataIndex
key links the corresponding value with the field name specified in the data view. Now you can add the closing parenthesis and brackets to the onReady()
method.
Is There Consolidated Code to Create the HTML CSS Grid?
Here is the consolidated HTML code for creating the JavaScript data grid. You can create a blank HTML file and paste the following code in it:
<html> <head> <meta charset="ISO-8859-1"> <title>Sencha Ext JS HTML CSS Grid</title> <link href="https://cdnjs.cloudflare.com/ajax/libs/extjs/6.2.0/classic/theme-classic/resources/theme-classic-all.css" rel="stylesheet" type="text/css" /> <script src="https://cdnjs.cloudflare.com/ajax/libs/extjs/6.2.0/ext-all.js" type="text/javascript"></script> <script> Ext.onReady(function() { Ext.define("com.extjsGrid.Sencha", { extend: "Ext.data.Model", fields: ["Product", "Environment", "Description"] }); var senchaStore = Ext.create("Ext.data.Store", { model: "com.extjsGrid.Sencha", data: [ {'Product': 'Ext JS', 'Environment': 'Javascript', 'Description':'Ext JS is the most comprehensive JavaScript framework for building feature-rich, cross-platform web applications'}, {'Product': 'React Grid', 'Environment': 'React', 'Description':'React Grid by Sencha is a perfect modern enterprise-grade grid solution for React UI that comes with 100+ amazing data grid features.'}, {'Product': 'Ext Angular', 'Environment': 'Angular', 'Description':'ExtAngular provides the most complete set of components for building data-intensive web apps using Angular.'}, {'Product': 'Ext React', 'Environment': 'React', 'Description':'ExtReact is the most complete set of React components for building data-intensive web apps using React'}, {'Product': 'ExtWebComponents', 'Environment': 'Javascript', 'Description':'ExtWebComponents provides a framework-agnostic set of over 140+ UI components for application development.'} ] }); Ext.create("Ext.grid.Panel", { renderTo: document.body, store: senchaStore, title: "Sencha Products", width: 1000, columns: [{ text: 'Prodcut', dataIndex: 'Product', autoSizeColumn: true }, { text: 'Environment', dataIndex: 'Environment', autoSizeColumn: true }, { text: 'Description', dataIndex: 'Description', width: 700, autoSizeColumn: true }] }); }); </script> <center><img src="https://www.sencha.com/wp-content/uploads/2021/11/icon-product-ExtJS-removebg-preview.png" alt="Sencha icon", width="50"></center> <center><h1>Sencha's HTML 5 CSS Grid</h1></center> <center><h2>How to Make a Grid in HTML 5</h2></center> </head> </html>
The last part of the HTML file simply displays the Sencha icon and the page titles. You can add more to this HTML 5 according to the requirements of your project.
That’s it! We just created an awesome data grid in JavaScript and HTML in 4 simple steps!
Why should I Opt for Sencha JavaScript Grids?
Sencha offers a range of products. Ext JS has all the important JavaScript tools required for building a JavaScript data grid. In addition to this, if you are a React developer, then you can opt our fast and reliable React data grid. The React grid, like the Ext JS grid is capable of handling millions of records in a matter of milliseconds.
The fastest JavaScript grid offered by Sencha is no simple data grid. It is responsive and interactive. Moreover, you can add different web components to this grid and configure it 100% according to the needs of your clients. Give your users the capability of applying various operators to their data including sorting, filtering, grouping and more. Additionally, you can add various components such as a line chart, bar chart or sparklines to your JavaScript grid.
Are you ready to get started with the best JavaScript developer tools and the fastest JavaScript grid? Of course you are! Sign up today for your free Sencha Ext JS trial and start building awesome data-intensive apps.
The grid components have been rewritten from the ground up for Ext JS 4 and…
Although most modern mobile devices have good hardware specs, almost all of them are still…
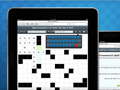
We've been interviewing the developers that created the amazing apps for our most recent Sencha…