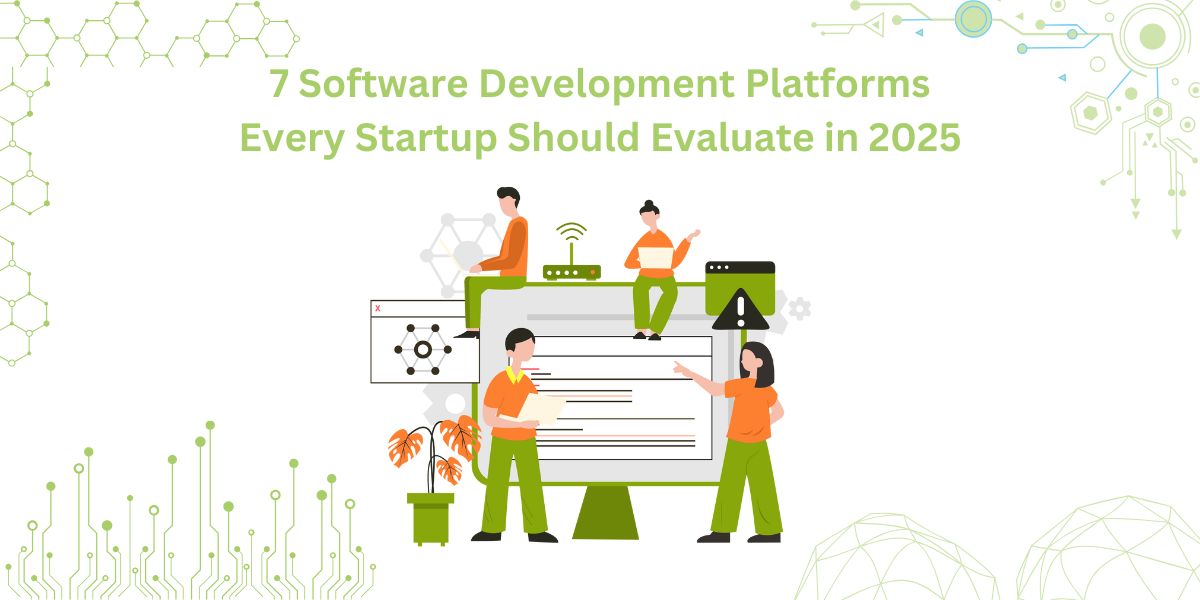
Did you know that nearly 90% of startups fail? And one of the biggest reasons is choosing the wrong tech early on? Yeah, that one decision, your development platform, can either speed things up or totally hold your product back.…
Subscribe to our newsletter
Be the first to learn about new Sencha resources and tips.