Create React App: It’s Not As Difficult As You Think
Developers often find creating React apps difficult due to a vast array of build tools along with a ton of configuration files and dependencies. React is a JavaScript library established by Facebook that is frequently referred to as a frontend JavaScript framework. In addition to this, it is a tool for creating user interface components. It is fascinating because instead of directly altering the browser’s DOM, React constructs a virtual DOM in memory and performs all necessary manipulations there before modifying the browser DOM.
However, one disadvantage of working with React is that it lacks pre-built components. As a result, React developers must either create these components from scratch or rely on the community. One of the hassle-free ways to deal with the issues underlying React is to use Facebook’s command-line tool: create React app. But if you are in the search of a perfect solution to all of React’s problems, you are in the right place. Sencha’s GRUI is a revolutionary tool that features high performance bundled with 100+ data grid features that ensure faster creation of data-intensive web applications. Whether you are a complete newbie or a seasoned developer, GRUI by Sencha is going to be the only tool you’ll ever need to succeed in creating React apps. Read on to find out more about create React app, GRUI by Sencha, and what makes it the ideal companion for React developers.
What is Create React App?
Setting up build tools like Babel and Webpack is required while creating a React application. Because React’s JSX syntax is a language that the browser doesn’t comprehend, certain build tools are necessary. That’s where create React app comes in, it helps you create applications that are compliant and supported by React framework. The beauty of this tool lies in the fact that it allows you to focus all of your energy on building apps rather than on building configurations. This results in increased productivity and saves developers from needless hassle.
How to Get Started with Building Your GRUI App?
Building apps on GRUI by Sencha is a breeze. There is no need for additional plugins and Sencha’s GRUI integrates seamlessly within your existing framework. This process can be easily categorized into 5 small steps.
- To Create React app:
npx create-react-app --template minimal my-app
Run cd my-app
- Adding GRUI:
Run npm add @sencha/sencha-grid
- Building your own component by loading up the pre-generated app component and replacing its app source with:
import React from "react";
import { SenchaGrid, Column } from "@sencha/sencha-grid";
import "@sencha/sencha-grid/dist/themes/grui.css";
export default class App extends React.Component {
render() {
const data = [
{ col1: "value1", col2: "data1", col3: 1.01 },
{ col1: "value2", col2: "data2", col3: 1.02 },
{ col1: "value3", col2: "data3", col3: 1.03 },
];
return (
<SenchaGrid data={data} style={{ width: "500px", height: "300px" }}>
<Column field="col1" text="Column 1" flex="1" />
<Column field="col2" text="Column 2" />
<Column field="col3" text="Column 3" align="right" />
</SenchaGrid>
);
}
}
Initiating the app by running:
npm start
Setting license by visiting GRUI and finally adding:
SenchaGrid.setLicense("<Your_procured_license_key>")
How Convenient is It to Create a Column Editor in Sencha’s GRUI?
Sencha’s GRUI is a high-performance react grid for react applications that makes it dead easy to create a column editor. In addition to this, it features extensive documentation which makes it a child’s play to diagnose and troubleshoot problems during installation or integration. In order to create a column editor you have to type in the following React typescript:
/**
* 1. Define the imports
*/
import React, { Component } from "react";
import EditorProps from "../EditorProps";
import IEditor from "./IEditor";
/**
* 2. Define the editor properties and be sure to extend EditorProps.
*/
export interface TextEditorProps extends EditorProps {}
/**
* 3. Define the component state
*/
interface TextEditorState {
value: any;
}
/**
* 4. Define the editor component
*/
export class TextEditor
extends Component<TextEditorProps, TextEditorState>
implements IEditor
{
private ref = React.createRef<HTMLInputElement>();
isSpaceCharacter: boolean;
constructor(props: TextEditorProps) {
super(props);
this.getInputEl = this.getInputEl.bind(this);
this.setValue = this.setValue.bind(this);
this.getValue = this.getValue.bind(this);
this.validate = this.validate.bind(this);
this.getErrors = this.getErrors.bind(this);
this.handleChange = this.handleChange.bind(this);
this.handleKeyDown = this.handleKeyDown.bind(this);
this.isSpaceCharacter = false;
this.state = {
value: this.props.defaultValue,
};
}
setValue(value: string): void {
this.setState({
value: value,
});
}
getValue(): string {
return this.state.value;
}
validate(): boolean {
let valid = this.state.value !== "";
return valid;
}
getErrors(): string[] {
let errors: string[] = [];
if (!this.validate()) {
errors.push("Please enter a value");
}
return errors;
}
getInputEl() {
return this.ref.current;
}
handleKeyDown(event: React.KeyboardEvent<HTMLInputElement>) {
if (event.code === "Space") {
this.setState({
value: this.getValue() + " ",
});
this.isSpaceCharacter = true;
} else {
this.isSpaceCharacter = false;
}
}
handleChange(event: React.ChangeEvent<HTMLInputElement>) {
if (this.isSpaceCharacter) {
return;
}
this.setState({
value: event.currentTarget.value,
});
}
render() {
const inputStyle = {
width: "100%",
height: "100%",
};
return (
<input
type="text"
style={inputStyle}
ref={this.ref}
value={this.state.value}
onChange={this.handleChange}
onBlur={this.props.onFocusLeave}
onKeyDown={this.handleKeyDown}
/>
);
}
}
export default TextEditor;
How to Declare the Component in Your Column Renderer Component?
/**
* This component is incomplete, so take a look at the Column Renderers guide for more.
* Take note of the state.editor definition, where the value is the editor component.
*/
export class AddressColumn
extends Component<AddressColumnProps, AddressColumnProps>
implements ColumnConfig<AddressColumnProps>
{
constructor(props: AddressColumnProps) {
super(props);
// Set the column sorter, render and editor,
// which are other components and the data grid will forward the proeprties to those components
this.state = {
...this.props,
sorter: { sorterFn: this.sorterFn.bind(this) },
renderer: <AddressRenderer style={props.style} />,
// 1. Define your editor component
editor: <TextEditor />,
};
}
sorterFn(a: any, b: any) {
return a[this.state.field].localeCompare(b[this.state.field], undefined, {
numeric: true,
sensitivity: "base",
});
}
// This is required, as the data grid uses this internally.
getColumnConfig(): AddressColumnProps {
return this.state;
}
render() {
return <>{this.props?.children}</>;
}
}
What Makes Sencha’s GRUI the Ideal Data Grid for React JavaScript Applications?
If you are looking for an ideal companion to design your React JavaScript applications with, your search stops here. We guarantee that there is no other tool on the market that rivals Sencha’s GRUI in terms of value for money and performance. It is blazingly fast and relatively simple to use and integrate which makes it the optimal choice for novice developers.
You should sign up for Sencha GRUI instantly because it can handle millions of records with ease while allowing for total control over the outlook and feel of the tool, including styling and theming. GRUI ensures safety and compatibility through extensive pre-testing across a vast array of browsers and platforms.
Developers can enjoy complete support for all TypeScript data types along with extremely fast data processing within milliseconds.
You can easily, create feature-rich HTML5 applications. Sencha’s GRUI is all about empowering developers to render their data according to their preference and liking through ready-made column types and renderers. You can even experience the GRUI demo to get a better hang of it.
It allows for fast and easy React UI component integration while leaving a minuscule footprint. From processing millions of records to customizing every aspect, Sencha’s GRUI can do it all, you will find no other tool which comes close to it in terms of functionality and speed. Perhaps the biggest perk of GRUI is that it allows customers a free trial so they can predetermine if the tool is compliant with their existing framework. With these measures in place, one can never go wrong with GRUI.
So what are you waiting for? Head over to Sencha to get your hands on the best tool on the market!
The grid components have been rewritten from the ground up for Ext JS 4 and…
Although most modern mobile devices have good hardware specs, almost all of them are still…
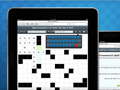
We've been interviewing the developers that created the amazing apps for our most recent Sencha…