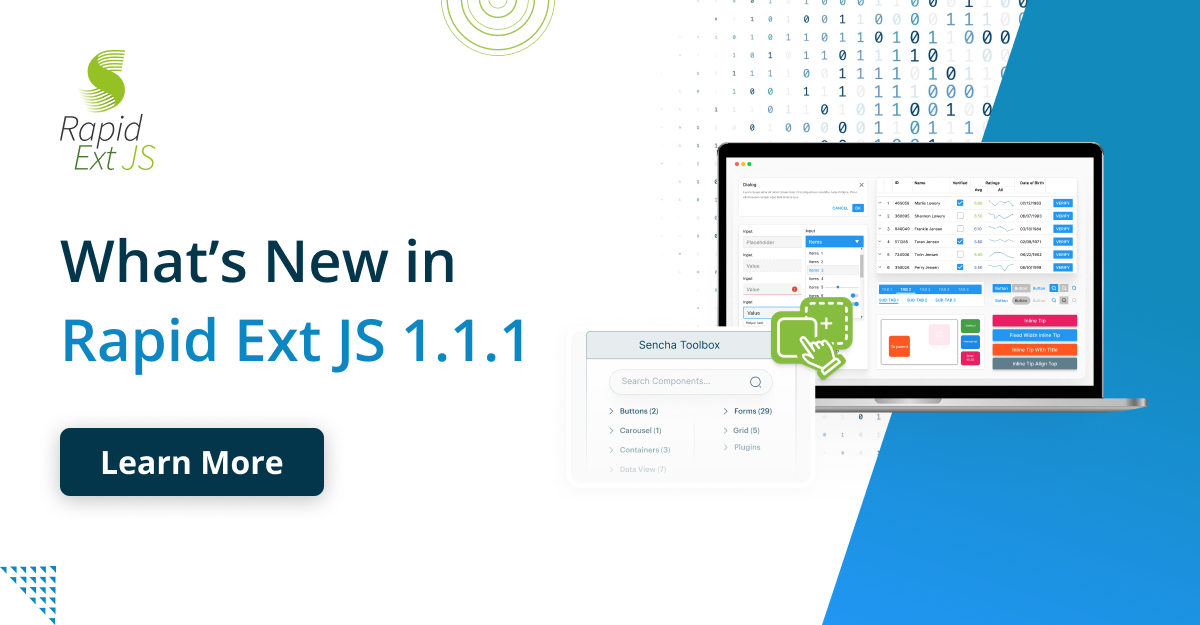
The Sencha team is excited to announce the latest Sencha Rapid Ext JS 1.1.1 release – designed to accelerate development, enhance maintainability, and empower you to build exceptional user experiences with ease. Following the Rapid Ext JS 1.1.0 release, which…
Subscribe to our newsletter
Be the first to learn about new Sencha resources and tips.