Top Mistakes Developers Make When Using React UI Component Library (And How to Avoid Them)
React’s everywhere. If you’ve built a web app lately, chances are you’ve already used it. Around 40% of developers use React JS for web development, making it the most widely adopted JavaScript framework.
And with that, UI component libraries have exploded. They help you skip the boring stuff like buttons, inputs, and tables and speed things up. Plus, you get polished, accessible components and support for complex components, too.
But not every library fits every project. Use the wrong one, and things get messy real fast. Your app starts to lag. The layout looks off. And suddenly, a small change breaks five other things.
Honestly, it’s rarely the library’s fault. It’s more about how we set things up. One quick fix or messy tweak can lead to hours of cleanup down the road.
This blog dives into the common mistakes devs make when working with a React UI component library. We’ll walk through design issues, performance pitfalls, and outdated tools that sneak into projects. We’ll see how skipping documentation and best practices affects the developers.
Along the way, you’ll learn how to avoid these traps and how a modern tool like ReExt can keep your UI fast, clean, and easy to scale. Let’s get started.
Mistake #1 – Choosing the Wrong React UI Component Library for the Project
We’ve all done it. You’re starting a new React project, and you grab the first UI library that looks cool. Maybe it has a slick demo or a ton of stars on GitHub. It seems like the safe choice.
But what works for someone’s portfolio site might not work for your actual app. That “quick pick” can lead to a lot of cleanup later. You get bloated bundles, features you never use, or worse, hit a wall when your app needs to scale.
Some React UI component libraries just aren’t built to handle real-world demands.
How to Avoid It
The trick is to pause and think about what your project actually needs. Not just now, but a few months from now.
Here’s what to look at:
- Is your UI simple or packed with complex components?
- Think your app might grow more users, more traffic, and more complexity down the line?
- Will you need it to run smoothly across web, mobile, maybe even desktop?
- Is your team comfortable picking it up, or will it take weeks to figure out?
- Is the library still active? Are they shipping updates or just letting it sit?
A good pick should offer solid React UI components,not just pretty ones. ReExt gets this right.
It comes with pre styled components, an intuitive API, and is built for serious React apps development. It won’t box you in later when things get big.
Mistake #2 – Inconsistent Design Across Components
We’ve all seen apps that feel like a Frankenstein of UI parts. A button looks one way, a form input another. Maybe someone dropped in components from three different React UI libraries, added custom styles, and hoped it would blend. Spoiler: it rarely does.
When design isn’t consistent, users notice. They might not point it out, but it makes your app feel off. And for developers, it turns the application development process into a constant patch job.
Tiny things like spacing, font sizes, or shadows end up eating your time.This usually starts when teams skip using a full UI framework for React. Or they mix React UI components without any shared design logic.
That approach doesn’t scale, especially in bigger or cross-team projects.
How to Avoid It
Keep it simple. Choose one UI library that actually covers what your app needs.
Ask yourself:
- Is this built for modern web applications, not just toy projects?
- Does it work with server-side rendering?
- Will it play nicely with Tailwind CSS or Headless UI?
- Are the components accessible and styled consistently?
ReExt checks those boxes. It gives you a full set of reliable, consistent React UI components with great community support and all the key features you’d expect. No design drift.
Just a smooth, unified look that holds up as your app grows.
Mistake #3 – Over-Customising Pre-built Components
We get it. Pre-built components are great until you want them to look “just a bit different.” That’s where things start to go sideways. You dig into the styles, override half the defaults, and before you know it, you’re maintaining a mess.
Over-customising kills the whole point of using a Web Application framework or React UI library. It adds bloat, slows down rendering, and creates bugs that only show up at the worst times. And let’s be honest, hunting down CSS conflicts is no one’s idea of fun.
How to Avoid It
The better route is to work with the theming system and config options the library gives you.
A good library offers:
- Built-in support for things like dark mode
- Simple APIs for styling changes
- Integration with React hooks and smooth animations
- Clean overrides without breaking the core
ReExt gives you that balance. You get full control over styles without touching the guts. Whether you’re building with Ant Design, Shadcn UI, or other React frameworks, keeping the design system clean is key.
In bigger react applications, where lazy loading and performance matter. ReExt’s theming keeps your components flexible but stable. Like solid building blocks that won’t fall apart.
Mistake #4 – Neglecting Performance Optimisation
When you’re just getting started, performance isn’t usually top of mind. Your app loads fast, everything feels smooth in dev, and it seems like you’re in the clear.
But then traffic grows. More features are added. It works great in dev, but once you go live, the slowdown hits hard. Things feel smooth until real users get on it, and then it starts crawling.
Most of the time, the slowdown happens because everything gets loaded at once. Rather than just pulling in what’s actually needed, the whole library gets dumped in upfront. That makes the app heavier than it needs to be.
When it’s not optimised, performance takes a hit. It’s even worse for enterprise apps or users on slower internet.
Laggy clicks, delayed animations, and long load times start creeping in. It’s frustrating. And if you’re building inclusive applications, performance directly affects accessibility. People using assistive tools, or navigating with a keyboard, feel it even more.
How to Avoid It
A few smart steps early on can save a lot of trouble later:
- Break your app into smaller chunks using code-splitting
- Use lazy loading for non-critical parts of your UI
- Import only what you need at the component level
- Pick tools that support responsive design and solid customisation options
- Make sure things like keyboard navigation and React Aria are built-in
- Align choices with your project requirements and team workflow
ReExt is built with this in mind. It offers fast performance, easy customisation, and a smooth developer experience, without the weight you get from other frameworks. Whether you’re building simple React applications or something large-scale, it won’t slow you down.
Mistake #5 – Using Outdated or Unmaintained Libraries
It’s tempting to grab a library that “just works” and move on. We’ve all done it. But if that library hasn’t been updated in a year, or worse, has no active community. You’re setting yourself up for trouble.
Old code doesn’t play well with newer versions of React. You start running into bugs, missing features, or styling issues that you can’t easily fix. Suddenly, your navigation bars break, your colour schemes are inconsistent, and you’re patching things just to keep the app running.
If you’re trying to build applications that last, this stuff can’t be ignored. Maintenance should be a top priority, especially when accessibility is on the line. Outdated tools usually fall short on WAI ARIA guidelines, which hurts users who rely on screen readers or keyboard support.
How to Avoid It
You don’t need anything fancy. Just pick tools that are actually being looked after.
- Check the repo. Has it seen any updates in the last few months?
- Are issues being answered?
- Are devs keeping up with the latest React changes?
- What kind of library do you want? One that’s evolving, not sitting untouched.
- Don’t skip on accessibility. Look for tools that clearly support WAI ARIA guidelines. If a library isn’t thinking about that, it’s already behind.
ReExt is solid on all fronts. It’s current, maintained, and works well with things like React-grid-layout, React data grid, and newer colour schemes. You can build serious apps with it without wondering if it’ll break the next time React updates.
In short, if your project needs long-term health, go with a library that’s active and built to last.
Mistake #6 – Skipping Documentation and Best Practices
Let’s be real, we’ve all done it. You set up a new framework, glance at the docs (maybe), then go straight to dropping in a component and hoping it works.
It works at first. But later, something breaks. Or the layout looks weird. Or you realise you’ve been doing it wrong for hours. That’s time lost debugging stuff that was already explained in the docs you never read.
When you skip documentation, you miss out on a lot, like features built for edge cases, or how to customise components properly. You start solving problems that were already solved, just not by you.
How to Avoid It
Slow down a bit. Take time to go through the docs before you build.
Here’s why that matters:
- You see how things are meant to work
- You find smarter ways to implement stuff
- You understand how to handle different use cases
- You avoid redoing things halfway through development
ReExt actually makes it easy. The docs are clean, real, and packed with examples. Whether you’re just starting or scaling, you stay in control. And for busy developers, that saves a lot of headaches.
Why ReExt Helps Developers Avoid These Pitfalls
What’s nice about ReExt? It takes all the solid stuff from Ext JS and makes it work naturally with React. No weird setup.
No stitching things together from random libraries. You just drop in the pieces you need. They behave like they should, React components that are ready to go when you are.
Unlike some React component libraries that only work well for small apps, ReExt is built to scale. It helps avoid all the usual pain points: inconsistent design, bloated bundles, and tricky overrides. You also get exceptional accessibility support baked in, which is huge if you’re building for a wider audience.
Working with ReExt feels easier than wrestling with tools like Material UI or Radix UI. It offers clean custom theme support, real cross platform compatibility, and a gentler learning curve.
It’s updated regularly with new features, so you’re not stuck on old patterns. For devs building serious apps, ReExt keeps things fast, maintainable, and under control without the usual React headaches.
Conclusion
React makes it fast to build, but that speed can lead to messy decisions. You pick a UI library that looks nice, mix in a few custom styles, maybe throw in another tool like Chakra UI. Before you know it, your app feels bloated and hard to manage.
That stuff adds up, especially in enterprise applications. You start losing time fixing design issues, fighting inconsistent layouts, or trying to untangle your own overrides. It’s frustrating and avoidable.
The better move is to pick tools that are built for the long run. Something that gives you real customisation options, not just a few tweaks. A setup where colour schemes are easy to change. Where easy customisation doesn’t come at the cost of breaking things.
ReExt makes that possible. It’s a solid choice for devs who want clean code, consistent UI, and a customizable foundation that won’t fight back as your app grows.
FAQs
What Should I Consider When Customising React UI Components?
Know what can be changed safely. Some customisation options break the layout or mess with functionality.
Can I Use Multiple React UI Libraries in the Same Project?
Sure, but expect styling headaches. It makes easy customisation a lot harder.
What Role Does Documentation Play in Using the React UI Component Library?
It shows how to use things properly and what customisation options are built in.
How Can I Avoid Performance Issues in My React Application?
Only load what’s needed. Keep components lightweight, even when adding easy customisation features.
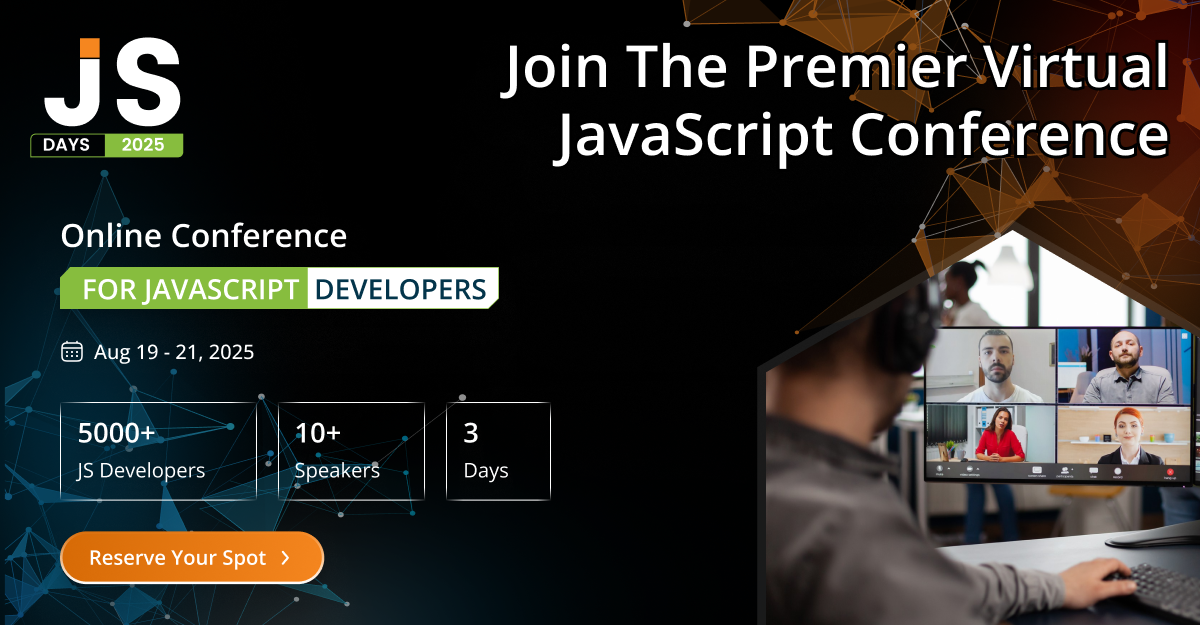
Join 5,000+ developers at the most anticipated virtual JavaScript event of the year — August…
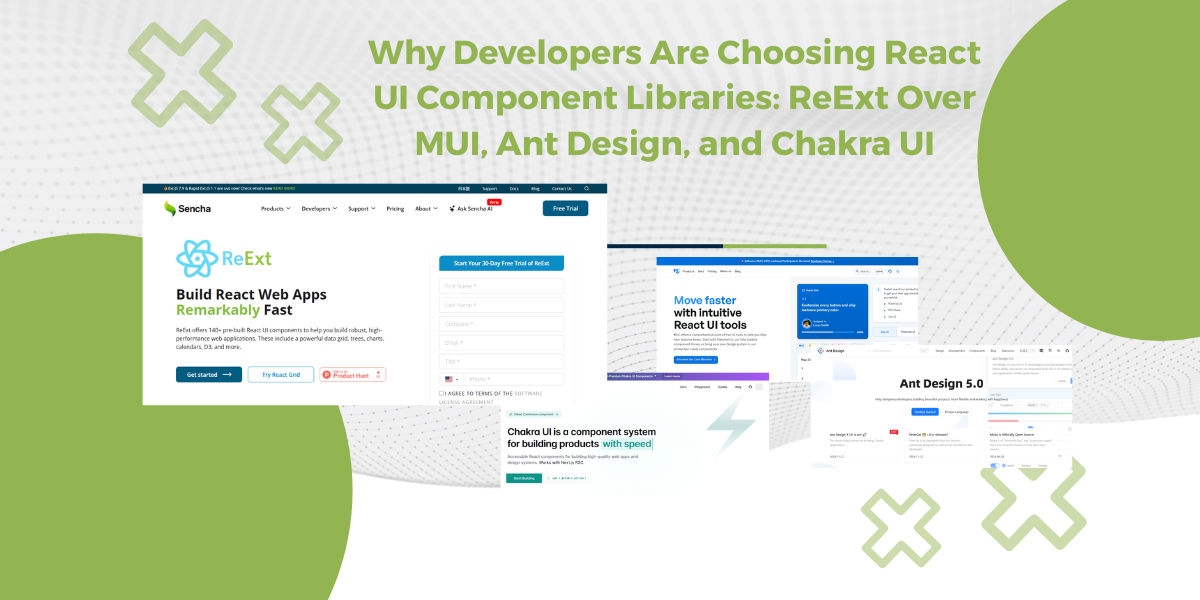
These days, React is the backbone of tons of websites. React is used by over…
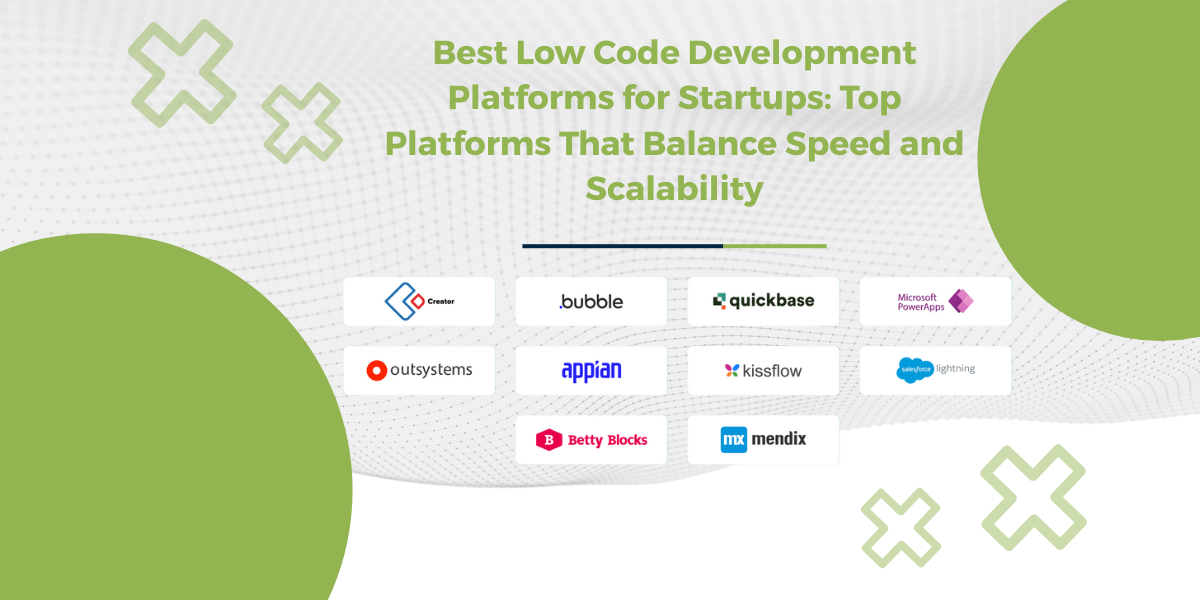
Startups don’t have the luxury of time, they’ve got to move, and move fast. Building…