Useful And Best Data JavaScript Grid Libraries
Web and mobile applications currently use JavaScript grid libraries to great extent. Given their capacity to incorporate potent functionality into the online designs they produce, they are outstanding resources for just about any front-end web developer. The JavaScript grid framework also makes it possible to manipulate HTML tables that contain enormous amounts of data.
How does JavaScript Grid work?
When web pages have a grid layout, depending on the configured views, they will collaborate with multiple capabilities. For each row and column in the grid, there are many columns. However, occasionally we may need to position or insert the small values side-by-side in the page applications such as mobile views. This may depend on the mobile screens, but the scenario is that we have to position the values such as using navigation or buttons, or other UI elements. They have distinct layouts, which are generally unacceptable for mobile platforms since mobile screens have constricted screen widths.
JavaScript has a grid system that may be used to arrange HTML content in a variety of ways, including with equal width, a set width, row gap, column gap, dynamically changing grid template rows and columns, and so on. It would be ideal if we utilize a system that offers a number of options for horizontal and vertical lines’ alignment and pushes to the beginning, middle, and end, or justify around/in between. Offsetting columns are a different category of grid layouts that we might use. Grid layouts in JavaScript are used to push content using column offsets that are changed with the left margin to the extent necessary for offsetting columns. Learn more about how to Create a Grid in Javascript.
The following will be some of the top JavaScript data grid libraries, along with a feature comparison to assist you in choosing the best one for your upcoming project:
GRUI by Sencha
Modern, enterprise-grade grid solution GRUI by Sencha offers more than 100 data grid functions for React projects. Sencha GRUI can manage millions of records and load massive volumes of data rapidly and effectively. It also provides a number of functions, including grouping, filtering, and infinite scrolling. Sencha GRUI also supports data export in a number of formats, such as CSV, XLS, PDF, etc. It is also incredibly easy to use. Overall, it has every requirement for a dependable, high-performance grid.
Features
- Capable of managing millions of records with efficiency
- Complete customizing control is offered
- Enables simple integration of UI components with the grid
- Provides data export support for CSV, TSV, HTML, PDF, and XLS, among other formats.
- Includes a wide range of features, such as filtering, grouping, infinite scrolling, etc.
Installation
You can easily install GRUI by Sencha using NPM as follows:
npm add @sencha/sencha-grid
How to use
When you use Sencha grid, you have to load up the generated App component and replace the App source with this code:
import React from “react”;
import { SenchaGrid, Column } from “@sencha/sencha-grid”;
import “@sencha/sencha-grid/dist/themes/grui.css”;
export default class App extends React.Component {
render() {
const data = [
{ column1: “value 1”, column2: “data 1”, column3: 1.01 },
{ column1: “value 2”, column2: “data 2”, column3: 1.02 },
{ column1: “value 3”, column2: “data 3”, column3: 1.03 },
];
return (
<SenchaGrid data={data} style={{ width: “600px”, height: “400px” }}>
<Column field=”column1″ text=”First Column” flex=”1″ />
<Column field=”column2″ text=”Second Colum” />
<Column field=”column3″ text=”Third Column” align=”right” />
</SenchaGrid>
);
}
}
Finally, you can run the app with this command:
npm start
That is how GRUI is incorporated into React. The method is pretty straightforward, as you can see. And therefore, you won’t experience any problems.
React Table
React Table[1] is an extensible data table for React projects. It is a headless data table, thus you have no control over how your markup or UI elements are shown.
Features
- It is possible to edit columns in real time.
- There are grouping and filtering options that resemble those in Excel.
- Data can be exported in a variety of formats, including Excel, PDF, and CSV.
- It provides infinitely long table grid rows with virtual rendering.
- You can use Bootstrap for styling.
Installation
You can easily install React Table using NPM or Yarn as follows:
//with npm
npm install react-table
//with yarn
yarn add react-table
How to use
A wide range of distinctive Hooks are available in React Table for creating and altering tables.
The following example shows how to use the UseTable Hook:
import React, { useState, useEffect, useMemo ) from "react"; import axios from 'axios';import "./App.css"; function App() { const [data, setData] = useState([]); useEffect(() => (async () => { const result = await axios("https://jsonplaceholder.typicode.com/users"); setData(result.data); })(); }, []); const tabledata = useMemo( () => [ { Header: "Users", columns: [ { Header: "Id", accessor: "id" }, { Header: "Name", accessor: "name" }, { Header: "User Name", accessor: "username" } ] }, { Header: "Contact Details", columns: [ { Header: "Phone", accessor: "phone" }, {Header: "Email", accessor: "email" }, { Header: "Website", accessor: "website" } ] } ] } ], []}; return ( <div className="App"> <Table columns={tabledata} data=(data) /> </div> );} export default App;
Handonstable
Data grids are easy to create and can be done very quickly thanks to the JavaScript framework Handsontable. It is a commercial solution that integrates with all of the major JavaScript frameworks and libraries, including Angular, React, and Vue.
Features
- You may keep all of your user’s data on your servers by using Handsontable.
- Allows data filtering, sorting, and CRUD activities.
- Allows formatting under constraints.
- Compatible with all modern browsers.
- Features for validating and binding data.
- Scaling, shifting, hiding columns and rows, and moving.
Installation
You can easily install Handsontable using NPM as follows:
npm install handsontable
How to use
Include a blank div element that will serve as a spreadsheet.
<div id="example"></div>
Pass a reference to that <div id=”example”> element and populate it with sample data in the following step:
var data = [ ['', 'Ford', 'Tesla', 'Toyota', 'Honda'], ['2017', 10, 11, 12, 13], ['2018', 20, 11, 14, 13], ['2019', 30, 15, 12, 13] ]; var container = document.getElementById('example'); var hot = new Handsontable(container, { data: data, rowHeaders: true, colHeaders: true, filters: true, dropdownMenu: true });
That will get your Handsontable up and ready.
Grid.js (Open-Source JavaScript Table Plugin)
Grid.js is a TypeScript-based JavaScript table plugin. It supports JavaScript, Angular, React, and Vue.
Features
- React Native support.
- Simple to use Support for modern web browsers.
- Its pipeline can be easily improved and expanded.
- Grid.js has an internal pipeline for handling data processing and caching.
- With all plugins, it is only 12kb in size.
Installation
Grid.js is available as NPM and Yarn packages. You can install it as follows:
//with npm
npm install gridjs
//with yarn
yarn add gridjs
How to use
Grid.js must first be imported:
import {Grid} from "gridjs";
import "gridjs/dist/theme/mermaid.css";
Next, the grid’s settings must be made, and an HTML placeholder must be made:
const grid = new Grid({ data: [ ['John', '[email protected]'], ['Mike', '[email protected]'] ] }).updateConfig({ columns: [‘Name’, ‘Email’], });
AG Grid
AG Grid is a fully operational JavaScript data grid with assured performance. It integrates effortlessly with the most well-liked JavaScript frameworks, including React, Angular, and Vue.js, and is independent of all third parties.
AG Grid provides solutions for both enterprises and communities, just like Handsontable does.
Features
Let’s see some of the features of AG Grid.
- You can alter the look and content of cells.
- Excel and CSV exporting are both supported.
- There is support for filtering, sorting, and pagination.
- Allows instantaneous updates.
- Carries out operations on the server’s records.
- Enables resizing, rearranging, and pinning of columns.
Installation
Using NPM or Yarn, you can quickly install AG Grid as seen below:
//with npm
npm install ag-grid-community
//with yarn
yarn add ag-grid-community
How to use
The AG Grid and styles must first be imported into your component:
import {Grid} from ‘ag-grid-community’;
@import “ag-grid-community/dist/styles/ag-grid.css”;
@import “ag-grid-community/dist/styles/ag-theme-alpine.css”;
The grid needs an HTML placeholder, which you must then create:
var gridOptions = { columnDefs: [ { headerName: 'Article', field: 'article' }, { headerName: 'Readers', field: 'readers' }, { headerName: 'Views', field: 'views' } ], rowData: [ { article: 'Article 1', readers: 100, views: 200}, { article: 'Article 2', readers: 200, views: 400}, { article: 'Article 3', readers: 300, views: 600} ] }; var grid = document.querySelector('#article-stats'); new Grid(grid, this.gridOptions);
jsGrid
JsGrid is a straightforward client-side data grid toolkit that uses jQuery. You can alter the components and style thanks to its adaptability.
Along with these extra features, jsGrid also includes a variety of fascinating capabilities, like data validation, data summaries, callbacks, and localization.
Features
- With jsGrid, data entry, validation, and modification are possible.
- Many languages have translations of jsGrid. Another choice is to create your own localizations.
- Provide callbacks to enable control and modification of behavior.
- JsGrid provides the ability to sort data using both human input and API.
Installation
You can easily install jsGrid using NPM as follows:
npm install jsgrid
How to use
Include the CSS grid stylesheet and jsGrid script in your website:
<link type="text/css" rel="stylesheet" href="jsgrid.min.css" />
<link type="text/css" rel="stylesheet" href="jsgrid-theme.min.css" />
<script type="text/javascript" src="jsgrid.min.js"></script>
For the grid, add a div to your web page’s markup:
<div id="jsGrid"></div>
Create a grid using the jQuery plugin jsGrid and configure it as follows:
<script> var clients = [ { "Name": "Otto Clay", "Age": 25, "Country": 1, "Address": "Ap #897-1459 Quam Avenue", "Married": false }, { "Name": "Connor Johnston", "Age": 45, "Country": 2, "Address": "Ap #370-4647 Dis Av.", "Married": true }, { "Name": "Lacey Hess", "Age": 29, "Country": 3, "Address": "Ap #365-8835 Integer St.", "Married": false }, { "Name": "Timothy Henson", "Age": 56, "Country": 1, "Address": "911-5143 Luctus Ave", "Married": true }, { "Name": "Ramona Benton", "Age": 32, "Country": 3, "Address": "Ap #614-689 Vehicula Street", "Married": false } ]; var countries = [ { Name: "", Id: 0 }, { Name: "United States", Id: 1 }, { Name: "Canada", Id: 2 }, { Name: "United Kingdom", Id: 3 } ]; $("#jsGrid").jsGrid({ width: "100%", height: "400px", inserting: true, editing: true, sorting: true, paging: true, data: clients, fields: [ { name: "Name", type: "text", width: 150, validate: "required" }, { name: "Age", type: "number", width: 50 }, { name: "Address", type: "text", width: 200 }, { name: "Country", type: "select", items: countries, valueField: "Id", textField: "Name" }, { name: "Married", type: "checkbox", title: "Is Married", sorting: false }, { type: "control" } ] }); </script>
Final Thoughts
Most web application needs a JavaScript Datagrid library. The Datagrid js framework is currently used by practically all Javascript web application development companies to show their records on websites. The top JavaScript libraries are essentially covered in this article, which will finally solve your issues while creating JavaScript tables for your online apps.
Choosing the right JS grid library for your application is entirely up to you. But, if you’re confused which to choose from the sea of options, try Sencha for free today!
The grid components have been rewritten from the ground up for Ext JS 4 and…
Although most modern mobile devices have good hardware specs, almost all of them are still…
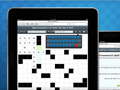
We've been interviewing the developers that created the amazing apps for our most recent Sencha…