6 Amazing and Useful New JavaScript Features in ES13
JavaScript, with its ability to create highly interactive user interfaces, is undoubtedly the cornerstone of every modern web application. Responding to user actions, such as keyboard inputs, clicks, and form submission, adding animations, and creating interactive elements like buttons, carousels, dropdown menus, etc., are all possible because of JavaScript and JS frameworks, such as React, Angular, and Ext JS JavaScript framework. And the best thing about JavaScript is that it is continuously evolving. New impressive features are continuously added to the programming language with a new specification version (ECMAScript) every year, enabling developers to meet the evolving needs of modern web app development.
For example, in ES13 or ES2022, the JavaScript standard released in 2022, various amazing features were introduced, enhancing JavaScript’s capabilities and enabling developers to write more concise and maintainable code. This article will explore six exciting and useful JavaScript features added to ES13.
Top-Level Await
Before ES2017 or ES8, handling asynchronous operations in JavaScript was quite complex. It often resulted in callback hell and made the code difficult to read and maintain. Fortunately, ES8 introduced an efficient asynchronous programming model through ‘async’ and ‘await’. These operators enable developers to write asynchronous code in a synchronous style. However, until ES13, ‘await’ could only be used inside ‘async’ functions.
ES13 allows developers to use the ‘await’ keyword outside async functions as well. In other words, we can use ‘await’ in the global scope at the top level of a module. Await basically stops execution until ‘Promise’ is fulfilled or rejected.
Top-level await is useful in many cases. For example, when performing asynchronous operations during module initialization, top-level await simplifies the code and makes it more straightforward.
Here is an example of top-level await:
// Importing an asynchronous function from another module import { fetchData } from './api'; // Using await at the top level const data = await fetchData(); console.log(data);
Class Field Declarations
ES13 has introduced class field declarations, which allow you to define and initialize class fields directly inside the class body or outermost scope of the class. Before this feature, we could declare class properties only in the constructor.
Class field declarations simplify the syntax, allowing for a more concise, organized, and readable code. We can use them to initialize properties with default values or based on calculations outside of the constructor.
Here is an example demonstrating the use of class field declarations:
Before ES13:
class House { constructor() { this.type = 'apartment'; this.rooms = 3; } } const house = new House(); console.log(house.type); // apartment console.log(house.rooms); // 3
Class field declarations in ES13:
class House { type = 'apartment'; rooms = 3; } const house = new House(); console.log(house.type); // apartment console.log(house.rooms); // 3
Private Class Fields
Before ES13, we couldn’t declare private fields or members in a class. This means class fields and methods could be accessed globally and modified from outside the class. If developers wanted to mark class fields private, they would prefix it with an underscore (_). However, these fields could still be accessed and modified from outside the class.
With ES13, developers can prefix class fields and methods with a hash (#) and make them private. These fields and methods cannot be accessed from outside the class. In other words, we can only access private fields inside the class where they are declared. Declaring private class fields can be helpful in various scenarios. For example, we can use it to prevent access to sensitive/confidential data or critical functionality within the class and enhance security.
Here is an example code demonstrating the use of private class fields:
class Employee { #firstName = 'Mark'; #lastName = 'Anderson'; #salary = 50000; get fullName() { return `${this.#firstName} ${this.#lastName}`; } get salaryDetails() { return `Salary: $${this.#salary}`; } } const employee = new Employee(); console.log(employee.fullName); // Mark Anderson console.log(employee.salaryDetails); // Salary: $50000 // Attempting to access private fields directly will result in an error // console.log(employee.#firstName); // Error: Private field '#firstName' is not defined
RegExp Match Indices
ES13 has introduced Match Indices for Regular Expressions. This feature allows us to retrieve both the starting and ending indices of the matched substring. Before ES13, we could only get information about the start index of a RegExp match in a substring. To get both the start and end indices, you simply need to use the /d flag in the regular expression. This feature is helpful when parsing text or extracting structured data from text strings.
Here is an example code demonstrating how to use RegExp match indices:
const phrase = 'earth and sky'; const pattern = /and/d; const result = pattern.exec(phrase); /** [ 'and', index: 5, input: 'earth and sky', groups: undefined, indices: [ [ 5, 8 ], groups: undefined ] ] */ console.log(result);
Static Class Fields and Static Private Methods
ES13 allows us to declare static class fields and static private methods for a class. These fields and methods can be accessed without class instantiation. We can declare static class fields and static private methods by simply using the ‘static’ keyword. Moreover, we can use the ‘this’ keyword to enable the static methods to access other static members (private or public) in the class.
Static class fields and methods are valuable when defining utility functions that don’t involve creating instances. This helps write more readable and organized code.
at() method for Arrays and Indexing
Before ES13, we used square brackets [] to get any element of an array. This is a simple process. We simply need to pass the index of the element inside the square bracket. However, if we need to get an element from the end of an array or get the elements backwards, we have to use ‘arr.length – N’. In other words, we couldn’t use negative indexing inside square brackets [].
However, ES13 has introduced the () method for accessing the elements of an array or string, which also supports negative indexing. While the at() method is similar to the square bracket [] method, it provides a more efficient way to access specific elements of an array or string. This allows for a more readable and maintainable code.
Example:
const array = ["red", "yellow", "green"] // Using the length property const lastelement = array console.log(lastelement) // Output: green // Using the at method const lastitm = array.at(-1) console.log(lastitm) // Output: green console.log(array.at(-2)) // Output: yellow console.log(array.at(0)) // Output: red
Also read: JS Frameworks: JavaScript for Device Characteristic Detection.
Conclusion
ES13, also known as ES2022, is a JavaScript standard or version introduced in 2022. It has introduced various helpful and valuable JavaScript features that enable developers to write more efficient, organized, and maintainable code. Here are the six exciting features of ES13 that we’ve covered in this blog:
- Top-level await
- Class field declarations
- Private class fields
- RegExp match indices
- Static class fields and static private methods
- at() method for array and indexing
FAQs
How can developers gradually adopt ES13 features into existing projects?
Here are the key steps to adapting ES13 features to existing projects:
1) Learn the features thoroughly
2) Ensure the features you want to implement are supported across browsers
3) Implement new features gradually into your codebase. Start with less crucial parts of your app.
4) Test your code thoroughly once you’ve implemented the new features.
Why should developers stay informed about the latest ECMAScript versions?
Staying informed about the latest ECMAScript versions enables you to use the latest JavaScript features to write more efficient, concise, readable, and maintainable code and create high-performance modern web applications.
What are the most popular JavaScript frameworks?
Examples of the most popular and modern JavaScript frameworks include React and Ext JS. React is an open-source JavaScript library that accelerates the web development process through its component-based architecture and features like virtual DOM.
Ext JS framework is the best javascript framework for creating enterprise-grade web and mobile apps. The popular JavaScript framework offers 140+ pre-built and high-performance components for building highly interactive and functional user interfaces quickly. Other prominent features of the framework include two-way data binding, support for MVVM architecture, a robust layout system, support for cross-platform development, and efficient event handling. Moreover, the framework is widely recognized for its security and cost-effectiveness.
Accelerate web app development with Sencha Ext JS – Try it now!
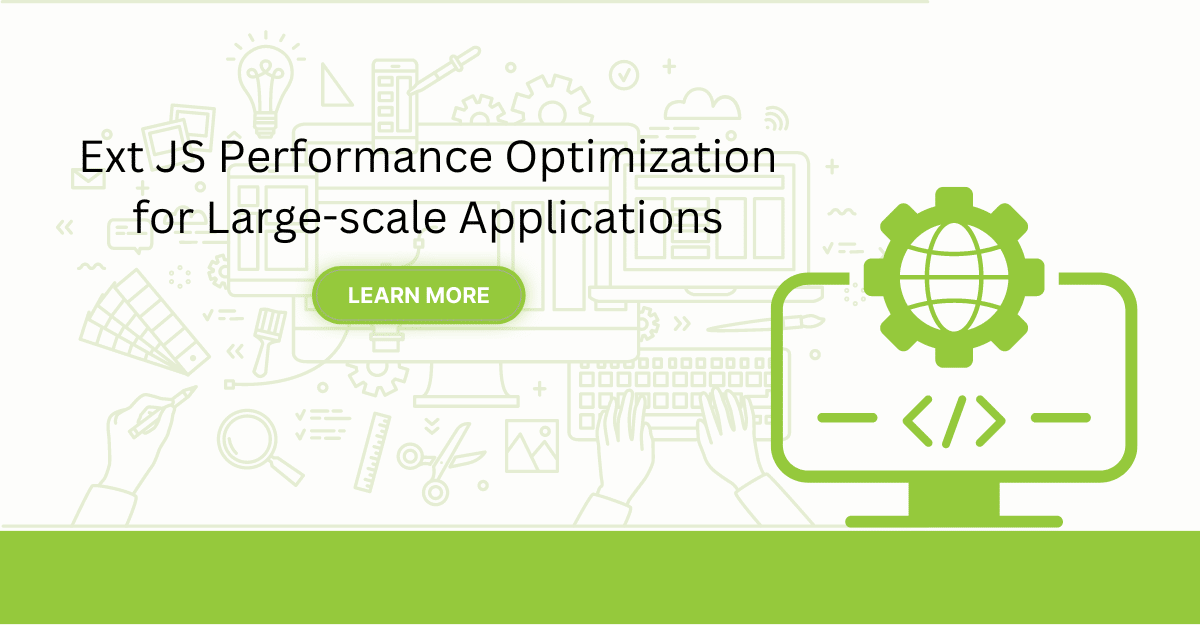
Are you facing issues with Ext JS applications’ performance as they scale up? Don’t worry!…
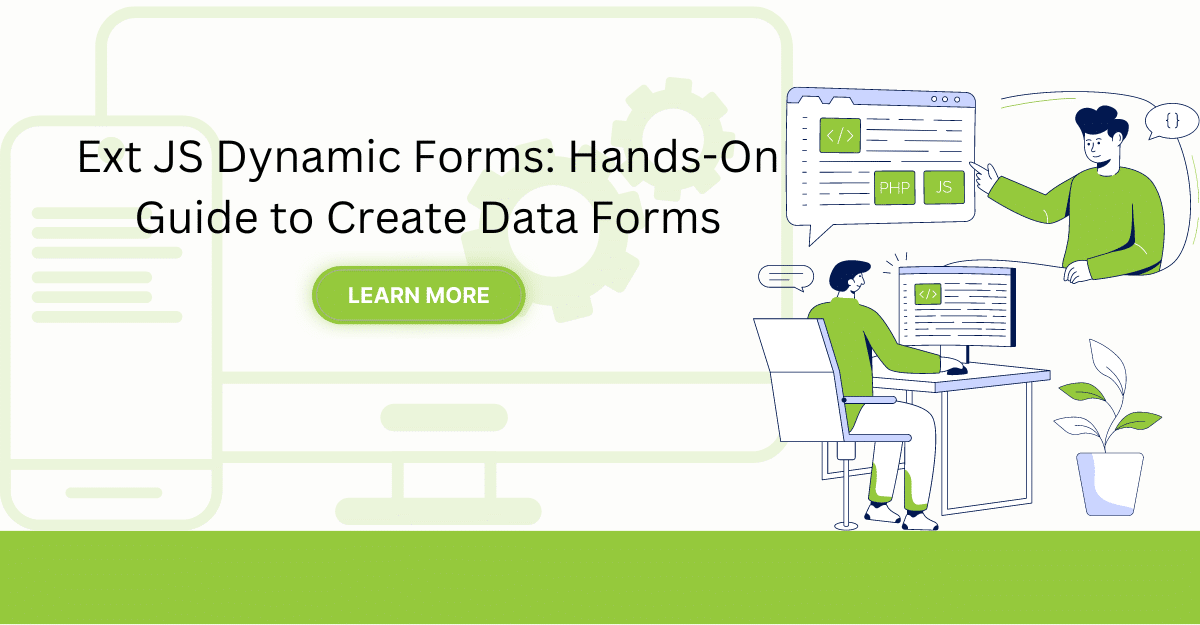
Dynamic forms are changing the online world these days. ExtJS can help you integrate such…
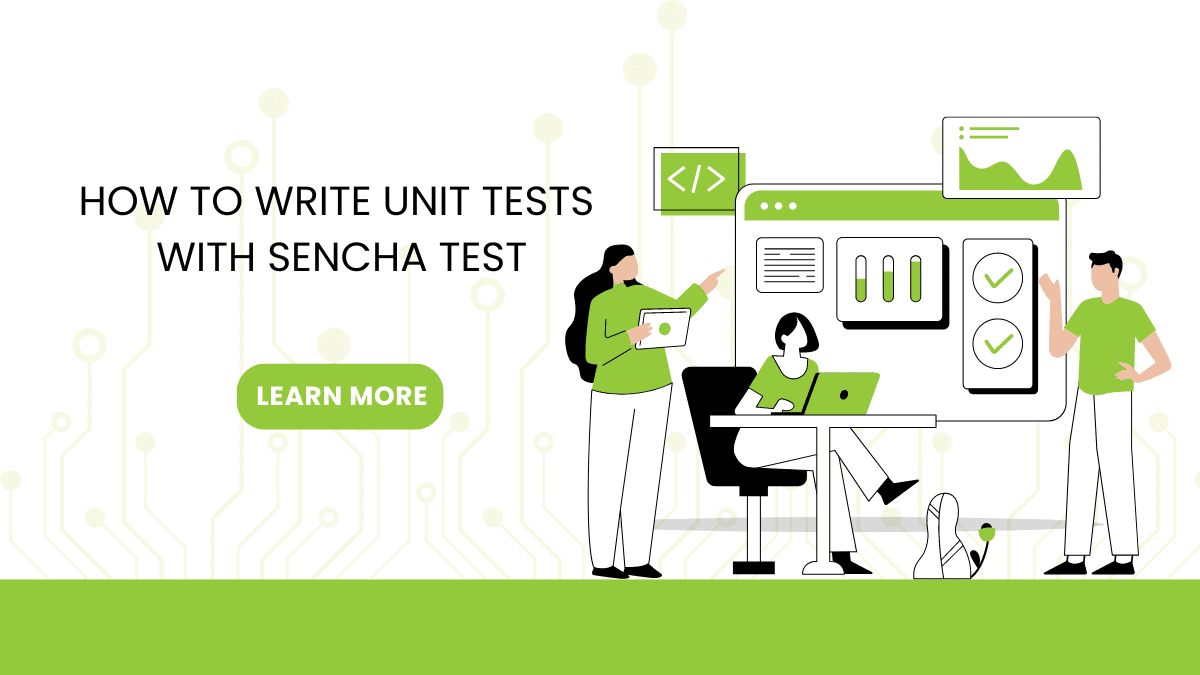
In modern software development, unit testing has become an essential practice to ensure the quality…