Learn Ext JS Online: Best Ext JS Tutorials for Beginners in 2024
Did you know that the Ext JS JavaScript framework is becoming increasingly popular among web developers in 2024? Among JS frameworks, Ext JS stands out for beginners since it offers valuable tools for creating a modern and interactive web application. Similarly, Ext JS also simplifies the overall development process with user-friendly tools and comprehensive documentation. Let’s look into the basics of Ext JS to find out how it helps beginners in developing web applications.
Getting Started with Ext JS
Ext JS is among the popular JavaScript frameworks created to build data-intensive web apps and mobile applications. Moreover, it’s popular for its rich component library, MVC architecture, and cross-platform capabilities. This means you can build complex applications with pre-built interactive user interfaces like grids, charts, and menus while making sure it’s consistent across various devices.
Setting Up Your Ext JS Development Environment
1. Download: Head to Sencha Website and choose your preferred download option (free trial or paid license).
2. Install: Follow the installation instructions based on your chosen download
method.
3. Create Project: Use the Sencha Cmd tool to create a new project and set up the basic structure.
4. Choose an IDE: While not mandatory, using an IDE with Ext.JS support can enhance your development experience. Popular options include Visual Studio Code with the Sencha plugin, Eclipse with the Ext JS plugin, or JetBrains WebStorm.
5. Explore Resources: Familiarize yourself with the Ext JS documentation, tutorials, and community forums for learning and support.
Understanding the Ext JS Architecture
When looking into the architecture, Ext JS uses a Model-View-Controller (MVC) to organize the application. This thereby enhances manageability, scalability, and testing. First of all, the “Model” component is focused on the application’s data and it also includes its structure and rules. Following that, the “View” section is used to display a UI component, such as grids and forms, and it dynamically updates based on the data changes. Lastly, the “Controller” takes charge of managing user interactions, updating data, and modifying the UI.
In addition to its MVC architecture, as said Ext JS also has a rich library of pre-built UI elements, known as components, such as buttons and menus. These web components are organized within containers, like panels and windows, to construct complex layouts and functionalities. Therefore, this helps in efficient UI design and management as well.
Ext JS offers comprehensive data management capabilities, which include defining data structures with models, organizing data into stores, using proxies for data loading and saving, and maintaining dynamic views through integration with the MVC architecture.
Building Your First Ext JS Application
Let’s start by building your first Ext JS JavaScript framework Application:
- Creating a simple application structure.
- Download Ext JS from the official website and set up the project using Sencha Cmd.
- Exploring the Ext.JS toolkit.
- Look into the pre-built components like panels, grids, buttons, etc., by reading the documentation.
- Learn about layout management and data binding mechanisms as well.
- Adding components to your application.
- Start with a main container panel and add a button with a click event handler.
- Example: In your JavaScript code file, create a panel using “Ext.create()” as shown in the example, and add a button inside it using the “panel.add()”. Then, define a click event handler for the button using the button.on(‘click’, handlerFunction).
- Nest components to create a hierarchical structure within your application.
- Example: Add additional components inside the panel by nesting them within each other using the “panel.add()”.
- Start with a main container panel and add a button with a click event handler.
- Running the Application.
- Use the Sencha Cmd tool to build your project, which generates production-ready files.
- Run a local web server like an HTTP server for server-side rendering.
- Access the index.html file in your browser to see your running application with the button you created.
Ext.application({
name: ‘MyApp’,
launch: function() {
Ext.create(‘Ext.panel.Panel’, {
title: ‘My First Ext JS App’,
layout: ‘hbox’,
items: [{
xtype: ‘button’,
text: ‘Click Me!’,
handler: function() {
alert(‘Hello from Ext JS!’);
}
}]
}).render(document.body);
}
});
By following these steps and examples, you can begin building your first Ext JS application and familiarize yourself with the JavaScript framework capabilities.
Also Read: Understanding the Link Between Style and Speed in JavaScript Frameworks
Working with Data in Ext JS
As mentioned before, the best JavaScript framework Ext JS offers powerful tools for managing and manipulating data in your web applications and this makes it easier to connect with backend servers through a variety of methods. It starts with using Restful APIs for basic operations like data retrieval and updates. Furthermore, for more complicated tasks, methods like direct remoting allow for direct calling of server-side methods, while web sockets ensure real-time communication for instant updates.
The process of loading and showcasing data in Ext JS begins with stores that fetch data from sources such as APIs. Next, models are used to define the structure and rules of your data. Furthermore, by integrating stores and models with user interface components like grids or charts through data binding, data is automatically displayed and updated. Additionally, templates offer the flexibility to customize how data is shown as well.
When it comes to modifying or removing data, Ext JS simplifies this with editable grids or forms by using plugins like CellEditing for grids. Similarly, proxies handle the transmission of changes to the server which ensures that updates or deletions are processed correctly. Before any changes are finalized, model validation rules are also applied to maintain data integrity.
Styling and Theming in Ext JS
In the best JavaScript framework Ext JS, styling, and theming are key to creating a unique and visually appealing user interface. The JavaScript framework has many pre-built themes such as Neptune, Triton, and Material, each providing unique styles and color schemes. Additionally, you also have the option to create custom themes, either by extending existing ones or starting from the beginning, and also customizing elements like colors, fonts, and shadows to your preference. Furthermore, the theming system uses SASS variables and mixins, which helps in easy customization as well.
To further customize styles and appearance, you can apply CSS classes to components for detailed styling control as well. Additionally, adding inline styles directly to components allows for precise adjustments. Furthermore, Sencha offers a paid tool called Sencha Themer, which provides a visual interface for theme creation and management, thus enhancing the customization capabilities available to developers.
Also, you should note that creating a visually appealing UI extends beyond just styling and theming. It involves a deep commitment to UI/UX principles, ensuring usability, accessibility, and responsiveness. Some of the ways to achieve it are, to choose a consistent color palette to support branding and information hierarchy. Additionally, effectively use typography to enhance readability. Furthermore, use layout, spacing, and contrast to direct users’ attention as well. Finally, make sure to test across various devices and browsers to guarantee consistency.
Event Handling and DOM Manipulation
In some of the most popular JavaScript frameworks like Ext JS, managing user interactions and manipulating the DOM are smoothly integrated to enhance application responsiveness and interactivity. The Ext.util.Observable mixin plays an important role here, by enabling components to handle events that range from standard types like click and change to custom ones you define. Initially, you attach event handlers to components or virtual DOM elements using the on or addListener methods. Subsequently, these handlers are equipped with information about the event, such as the target element and any additional data, allowing for customized responses to various user interactions.
Furthermore, to help direct manipulation of the virtual DOM, JS frameworks like Ext JS also provide a lot of methods like getEl(), query(), and select(). This enables developers to access and modify DOM elements with ease. Also, creating new elements is straightforward with “createEl()” or “insertHtml()”, and existing elements can be removed using the remove() method as well. Moreover, dynamic updates to styles and classes are made possible through setStyle() and addCls(). This makes sure that the application’s visual elements remain dynamic and responsive. Additionally, Ext.dom.Query offers powerful tools for selecting and manipulating groups of DOM elements while further enhancing the developer’s ability to manage the application’s appearance and functionality.
Debugging and Testing Ext JS Applications
Debugging is made much easier with browser developer tools. These tools, such as Chrome DevTools, Firefox Developer Tools, Microsoft Edge DevTools, and Safari Web Inspector, allow developers to inspect JavaScript, HTML, and CSS. Additionally, Sencha Inspector is a dedicated tool to debug Ext JS. Moreover, developers can use logging, breakpoints, and version control systems like Git for effective debugging and collaboration.
In Ext JS, unit testing uses frameworks like Jasmine, Mocha, and Karma. This provides clear syntax and efficient mocking capabilities. Furthermore, developers isolate components using mocking and stubbing for focused testing, measure test coverage and adhere to best practices by writing maintainable tests. They also integrate unit testing into their web development process for thorough testing.
Best Practices for Troubleshooting an Ext JS Web Application
1. Understand error messages by carefully reading them.
2. Isolate the issue by identifying consistent steps leading to the error.
3. Break down the problem into smaller, manageable parts.
4. Use debugging tools effectively, such as developer tools and Sencha Inspector.
5. Search Ext JS documentation, forums, and Stack Overflow for solutions.
6. Maintain a troubleshooting log documenting steps and solutions.
7. Work together with colleagues or the Ext JS community for assistance.
Advanced Topics in Ext JS
In the JS framework Ext JS, a key advanced concept that can be used to create web applications is inheritance. It lets subclasses use properties and methods for customization, which saves developers from redoing everything. Additionally, plugins also smoothly integrate component functionality, while mixins facilitate shared functionality. Furthermore, templates are used to define custom HTML structures and overrides by carefully modifying Ext JS methods to prevent unintended issues.
Integrating third-party JavaScript libraries can significantly enhance the functionality and capabilities of your web application. Some key factors to consider when integrating third-party JavaScript libraries are as follows.
- Utilize Ext.Loader.load() to dynamically load external files.
- Organize code with namespaces to prevent conflicts.
- Create adapters mapping Ext JS and JavaScript library APIs for seamless integration during code execution.
- Ensure library compatibility with the Ext JS version and browser support requirements.
How to Optimize Performance in Ext JS Applications
1. Implement lazy loading to load components when necessary.
2. Minimize unnecessary data binding operations and optimize data access
methods.
3.Improve grid performance with large datasets using virtualization, row buffering, and custom cell rendering techniques.
4. Optimize event handling by avoiding excessive listeners and using event delegation.
5. Identify performance bottlenecks with browser developer tools or Sencha Profiler to focus optimization efforts.
Real-world Projects and Case Studies
Ext JS showcases its versatility through real-world projects and case studies. Sencha Touch 2 demonstrates a live fleet management dashboard that provides real-time vehicle details. Additionally, Ext JS also powers a financial data visualization tool, offering interactive charts and a JavaScript grid for robust data analysis.
Furthermore, the Sencha Customer Showcase highlights successful Ext JS applications across industries like healthcare and finance. Moreover, the Ext JS GitHub repository hosts community-driven projects that showcase the framework’s potential through open-source applications and demos.
Resources and Next Steps
For those interested in Ext JS a variety of resources are available as given below.
Resources for Ext JS
1. Books: “Ext JS in Action” and “Mastering Ext JS 6” are comprehensive guides covering fundamental concepts and advanced techniques of Ext JS development. “Building Data-Driven Web Apps with Ext JS 7.5” focuses on Ext JS 7.5 for
constructing data-centric applications.
2. Blogs: Sencha Blog, Ext JS Medium, and Ext JS Central offer valuable insights, tutorials, and discussions contributed by developers worldwide.
3. Forums: Sencha Forums and Stack Overflow provide platforms for asking questions, seeking help, and engaging with the Ext JS community.
4. Online Tutorials and Courses: Platforms like Sencha Learn, Udemy, and Pluralsight
offer online tutorials and courses tailored for learners at all levels, from beginners to experienced developers.
Moreover, join the Ext JS Slack community for real-time interactions. Also, attend events and meetups since they provide opportunities to network with a fellow web developer as well. Also, contribute to the Ext JS open-source community which offers a rewarding experience of collaborative learning and growth.
Conclusion
Ext JS stands out as a robust tool for web development among popular JS frameworks. In this article, we have discussed the basics of Ext JS and some of its advanced concepts as well. To keep growing as a beginner, explore resources like books, blogs, and online courses. Moving forward, Ext JS also promises exciting advancements. So, stay curious and keep learning to shape the future of web development with Ext JS.
Elevate your skills in 2024: Master Ext JS, and build powerful apps with Sencha!
Frequently Asked Questions
How can I handle data in Ext JS applications?
In Ext JS applications, data handling involves defining data structures with models and handling data collections using stores. Additionally, in apps built with JS frameworks, you can also synchronize data between UI components and models through two-way data binding.
What debugging tools are available for Ext JS development?
For Ext JS development, debugging tools include Sencha Inspector for comprehensive insights into components and browser developer tools like Chrome DevTools, Firefox Developer Tools, Microsoft Edge DevTools, and Safari Web Inspector. Additionally, use logging, breakpoints, and Git for efficient debugging and collaboration.
How do I set up the Ext JS development environment?
To set up the Ext JS development environment, install Sencha Cmd and Sencha Visual Studio Code plugin. Then, create a new Ext JS project using Sencha Cmd’s generate command.
The grid components have been rewritten from the ground up for Ext JS 4 and…
Although most modern mobile devices have good hardware specs, almost all of them are still…
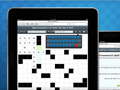
We've been interviewing the developers that created the amazing apps for our most recent Sencha…