Email Application: An Ultimate Guide to Develop it in 2025
Have you ever wondered what goes into creating an email application that seamlessly handles millions of messages daily? Building an email application client is a complex but fascinating endeavor. It combines elements of design, usability, and robust backend development.
In this guide, we will explore the entire process, from initial setup to advanced features like calendar integration and task management. You’ll learn why developing a custom email application can provide unique benefits. Moreover, we will also explain how to implement those features step-by-step.
What Is an Email Client Application?
An email client is a type of software that allows users to access, manage, and send emails. It provides a user-friendly interface for viewing and organizing email messages. These clients can be standalone software, web-based platforms, or mobile apps.
Beyond basic email functionalities, they often offer additional features such as:
👉Integrating calendars
👉Managing contacts
👉Organizing tasks
👉Filtering emails.
What Are the Best Email Client Applications?
Here are some of the best email client applications for Windows:
✔️eM Client
Highly customizable with features like templates, video chat, and cloud storage integrations.
✔️Thunderbird
Free, open-source, and expandable with numerous add-ons.
✔️Mailbird
Ideal for heavy email users with extensive app integrations.
✔️Windows Mail
Simple and focused with basic functionalities.
✔️Microsoft Outlook
Robust with advanced features and Microsoft 365 integration.
✔️Kiwi for Gmail
Perfect for Gmail users seeking enhanced features.
✔️Twobird
Combines email with to-do list functionalities.
Why Another Email App?
Building another email app can make sense for several reasons.
- Current email apps may not meet everyone’s needs.
- Users might want better features, such as easy integration with other tools, stronger security, or a more user-friendly interface.
- New technology allows for improvements in speed and functionality.
- Additionally, there are opportunities to create unique features, such as smart email organization using AI.
How Should I Start Making an App Like Gmail?
To start making an app like Gmail using Ext JS, follow these steps:
1. Set Up the Foundations
Begin by setting up your development environment and structuring your project. Here are the steps:
👉Download Ext JS and Sencha Cmd
Ensure you have the Ext JS framework and the latest Sencha Cmd installed. You can download the trial versions from Sencha’s website.
👉Generate a New Application
Use Sencha Cmd to create a new universal application:
sencha generate app ExtMail ../ext-mail
👉Application Structure
Organize your project into folders for shared code, Classic toolkit, and Modern toolkit.
Example folder structure:
ext-mail/
├── app/
├── classic/
├── modern/
└── data/
👉Static Data Sources
Set up static JSON files for contacts, labels, and messages:
- `contacts.json`
- `labels.json`
- `messages.json`
- Place these files in the `data` folder.
👉Create Data Models
Define data models in the `app/model` folder. Example for a message model:
Ext.define('ExtMail.model.Message', {
extend: 'Ext.data.Model',
fields: [
{ name: 'firstName' },
{ name: 'lastName' },
{ name: 'fullName', calculate: function(data) {
var firstName = data.firstName || '';
var lastName = data.lastName || '';
return Ext.String.trim(firstName + ' ' + lastName);
}},
{ name: 'email' },
{ name: 'date', type: 'date', dateFormat: 'c' },
{ name: 'subject' },
{ name: 'message' },
{ name: 'labels', type: 'auto', defaultValue: [] },
{ name: 'unread', type: 'boolean' },
{ name: 'draft', type: 'boolean' },
{ name: 'outgoing', type: 'boolean' },
{ name: 'sent', type: 'boolean' }
]
});
👉Create Data Stores:
Define stores in the `app/store` folder to hold collections of models. Example for a message store:
Ext.define('ExtMail.store.Messages', {
extend: 'Ext.data.Store',
alias: 'store.Messages',
model: 'ExtMail.model.Message',
autoLoad: true,
sorters: [{ property: 'date', direction: 'DESC' }],
proxy: {
type: 'ajax',
url: 'data/messages.json',
reader: { type: 'json', rootProperty: 'rows' }
}
});
2. Enhance User Experience
Add labels, trees, and dynamic actions to improve the user interface. Here are the code examples:
Starring and Unstarring Messages:
columns: [
{
xtype: 'actioncolumn',
width: 25,
items: [
{
glyph: '★',
tooltip: 'Star',
getClass: function(v, meta, rec) {
if (rec.get('starred')) {
return 'x-hidden-display';
}
},
handler: function(view, rowIndex, colIndex, item, e, rec) {
view.grid.fireEvent('starmessage', rec);
}
},
{
glyph: '★',
tooltip: 'Un-star',
getClass: function(v, meta, rec) {
if (!rec.get('starred')) {
return 'x-hidden-display';
}
},
handler: function(view, rowIndex, colIndex, item, e, rec) {
view.grid.fireEvent('unstarmessage', rec);
}
}
]
},
// other columns...
]
Toolbar Actions:
{
tooltip: 'Archive',
iconCls: 'x-fa fa-archive',
handler: 'onArchiveClick',
hidden: true,
bind: {
hidden: '{!selectedMessage}'
}
},
{
tooltip: 'Delete',
iconCls: 'x-fa fa-trash',
handler: 'onDeleteClick',
hidden: true,
bind: {
hidden: '{!selectedMessage}'
}
},
{
tooltip: 'Mark as Unread',
iconCls: 'x-fa fa-envelope',
handler: 'onMarkAsUnreadClick',
hidden: true,
bind: {
hidden: '{!selectedMessage}'
}
}
Message Count Summary:
items: [
// other toolbar items...
'->',
{
xtype: 'component',
tpl: '{count} messages',
data: {},
bind: {
hidden: '{selectedMessage}',
data: {
count: '{messages.count}'
}
}
}
]
Labels Tree:
Ext.define('ExtMail.view.labels.LabelsTree', {
extend: 'Ext.tree.Panel',
alias: 'widget.labels-LabelsTree',
initComponent: function() {
Ext.apply(this, {
rootVisible: false
});
this.callParent(arguments);
},
columns: [
{
xtype: 'treecolumn',
header: false,
text: 'Name',
dataIndex: 'name',
flex: 1
}
]
});
items: [
{
xtype: 'labels-LabelsTree',
region: 'west',
width: 300,
bind: {
store: '{labels}',
selection: '{selectedLabel}'
}
},
// other items...
]
// MainModel.js
data: {
selectedLabel: null,
// other data...
},
constructor: function() {
this.callParent(arguments);
this.bind('{selectedLabel}', this.onSelectedLabelChange, this);
},
onSelectedLabelChange: function(labelRecord) {
this.getStore('messages').clearFilter();
this.getStore('messages').filterBy(function(messageRecord) {
return labelRecord ? messageRecord.hasLabel(labelRecord.getId()) : false;
});
},
Label Unread Count:
calculateUnreadCounts: function() {
this.getStore('labels').each(function(labelRecord) {
var count = this.getStore('messages').queryBy(function(messageRecord) {
return (
messageRecord.hasLabel(labelRecord.getId()) &&
messageRecord.get('unread')
) || (
messageRecord.hasLabel(labelRecord.getId()) &&
messageRecord.get('draft')
);
}).getCount();
labelRecord.set('unreadCount', count);
}, this);
}
{
xtype: 'treecolumn',
header: false,
text: 'Name',
dataIndex: 'name',
flex: 1,
renderer: function(value, meta, record) {
var hasUnread = record.get('unreadCount') > 0;
meta.tdStyle = 'font-weight: ' + (hasUnread ? 'bold' : 'normal');
var unreadTpl = Ext.String.format('<span> ({0})</span>', record.get('unreadCount'));
return Ext.String.format('<span>{0}</span>{1}', value, hasUnread ? unreadTpl : '');
}
}
3. Compose Workflow
Implement the compose workflow and draft message features. Here are the steps to achieve that:
Add a Compose Button
Add a compose button to the `LabelsTree` component:
initComponent: function() {
Ext.apply(this, {
rootVisible: false,
dockedItems: [
{
xtype: 'toolbar',
dock: 'top',
weight: -1,
items: [
'->',
{
xtype: 'button',
scale: 'large',
text: 'Compose',
iconCls: 'x-fa fa-edit',
width: 150,
handler: function() {
this.fireEvent('compose');
},
scope: this
},
'->'
]
}
],
});
this.callParent(arguments);
}
Create the Compose Form
Create a form with recipient, subject, and message fields:
Ext.define('ExtMail.view.compose.ComposeForm', {
extend: 'Ext.form.Panel',
alias: 'widget.compose-ComposeForm',
defaultListenerScope: true,
padding: 10,
layout: {
type: 'vbox',
align: 'stretch'
},
items: [
{
xtype: 'combobox',
emptyText: 'Recipient',
width: '100%',
displayField: 'email',
valueField: 'email',
queryMode: 'local',
allowBlank: false,
bind: {
store: '{contacts}',
selection: '{selectedRecipient}',
value: '{messageRecord.email}'
}
},
{
xtype: 'textfield',
emptyText: 'Subject',
bind: {
value: '{messageRecord.subject}'
}
},
{
xtype: 'textareafield',
flex: 1,
bind: {
value: '{messageRecord.message}'
}
}
],
dockedItems: [
{
xtype: 'toolbar',
dock: 'bottom',
margin: 0,
items: [
{
xtype: 'button',
scale: 'medium',
text: 'Send',
formBind: true,
handler: 'onSendClick'
},
'->',
{
xtype: 'button',
iconCls: 'x-fa fa-trash',
tooltip: 'Discard',
handler: 'onDiscardClick'
}
]
}
],
onSendClick: function() {
this.fireEvent('send', this.getViewModel().get('messageRecord'));
},
onDiscardClick: function() {
this.fireEvent('discarddraft', this.getViewModel().get('messageRecord'));
}
});
Add the Compose Window
Create a window to hold the compose form:
Ext.define('ExtMail.view.compose.ComposeWindow', {
extend: 'Ext.window.Window',
alias: 'widget.compose-ComposeWindow',
viewModel: {
data: {
messageRecord: null,
},
},
config: {
messageRecord: null,
},
minimizable: true,
resizable: false,
draggable: false,
title: 'New Message',
layout: 'fit',
constrain: true,
constrainHeader: true,
items: [
{
xtype: 'compose-ComposeForm',
bubbleEvents: ['send', 'discarddraft'],
},
],
updateMessageRecord: function(messageRecord) {
this.getViewModel().set('messageRecord', messageRecord);
},
});
Start the Compose Flow
Implement the `onComposeMessage` function in the `MainControllerBase` class:
onComposeMessage: function() {
var messageRecord = Ext.create('ExtMail.model.Message', {
labels: [ExtMail.enums.Labels.DRAFTS],
outgoing: true,
draft: true
});
this.getViewModel().getStore('messages').add(messageRecord);
this.getViewModel().getStore('messages').commitChanges();
this.showComposeWindow(messageRecord);
}
Show the Compose Window
Define the `showComposeWindow` function:
showComposeWindow: function(messageRecord) {
var win = Ext.create('ExtMail.view.compose.ComposeWindow', {
messageRecord: messageRecord,
height: 500,
width: 500
});
win.show();
}
Handle Compose Window Events
showComposeWindow: function(messageRecord) {
var win = Ext.create('ExtMail.view.compose.ComposeWindow', {
messageRecord: messageRecord,
height: 500,
width: 500
});
win.on({
send: Ext.bind(this.onSendMessage, this, [win], true),
discarddraft: Ext.bind(this.onDiscardDraftMessage, this, [win], true),
scope: this
});
win.show();
},
onSendMessage: function(messageRecord, e, composeWindow) {
messageRecord.removeLabel(ExtMail.enums.Labels.DRAFTS);
messageRecord.addLabel(ExtMail.enums.Labels.SENT);
messageRecord.set({
draft: false,
sent: true,
date: new Date()
});
messageRecord.commit();
composeWindow.close();
},
onDiscardDraftMessage: function(messageRecord, e, composeWindow) {
this.getViewModel().getStore('messages').remove(messageRecord);
composeWindow.close();
}
Edit a Draft
Modify `onMessageClick` to handle draft messages:
onMessageClick: function(grid, messageRecord, rowEl, index, e) {
if (e.getTarget('.x-action-col-icon')) {
return;
}
if (messageRecord.get('draft')) {
this.showComposeWindow(messageRecord);
} else {
this.getViewModel().set('selectedMessage', messageRecord);
}
}
4. Mobile Optimization
Use the Ext JS Modern Toolkit to optimize the email client for mobile devices. Here are the steps:
Create the Main View
Define a root component using `Ext.NavigationView` for a native-like feel:
Ext.define('ExtMail.view.main.Main', {
extend: 'Ext.NavigationView',
xtype: 'app-main',
fullscreen: true,
requires: ['ExtMail.view.main.MainModel'],
viewModel: 'main'
});
```
### 2. Create Messages Grid
Create a messages grid using `Ext.grid.Grid`:
```javascript
Ext.define('ExtMail.view.messages.MessagesGrid', {
extend: 'Ext.grid.Grid',
alias: 'widget.messages-MessagesGrid',
cls: 'messages-grid',
columns: [
{
dataIndex: 'firstName',
width: 60,
cell: { encodeHtml: false },
tpl: [
'<div class="avatar" style="background-color: {[this.getAvatarColour(values.firstName)]};">',
' <span>{[values.firstName.substring(0, 1).toUpperCase()]}</span>',
'</div>',
{
getAvatarColour: function(name) {
var alphabet = 'ABCDEFGHIJKLMNOPQRSTUVWXYZ'.split('');
var colours = ['#e6194B', '#3cb44b', '#ffe119', '#4363d8', '#f58231', '#911eb4', '#42d4f4', '#f032e6', '#bfef45', '#fabed4', '#469990', '#dcbeff', '#9A6324', '#fffac8', '#800000', '#aaffc3', '#808000', '#ffd8b1', '#000075', '#a9a9a9', '#ffffff', '#000000'];
var initial = name.substring(0, 1).toUpperCase();
return colours[alphabet.indexOf(initial)] || '#e6194B';
}
}
]
},
{
dataIndex: 'subject',
flex: 1,
cell: { encodeHtml: false },
tpl: [
'<div class="{[values.unread ? "unread" : ""]}">',
' <div class="top-line">',
' <span class="name">{fullName}</span>',
' <span class="date">{date:date("j M 'y")}</span>',
' </div>',
' <div class="subject">{subject}</div>',
' <div class="message">{message}</div>',
'</div>'
]
}
]
});
Add the Messages Grid to Main View
Include the grid in the main view and bind it to the messages store:
Ext.define('ExtMail.view.main.Main', {
// ... previous configuration ...
items: [
{
xtype: 'messages-MessagesGrid',
hideHeaders: true,
titleBar: false,
bind: { store: '{messages}' },
listeners: { childtap: 'onMessageTap' }
}
]
});
Handle Message Tap
Define the event handler to display message details:
Ext.define('ExtMail.view.main.MainController', {
extend: 'ExtMail.view.main.MainControllerBase',
alias: 'controller.main',
onMessageTap: function(grid, location) {
this.handleMessageClick(location.record);
}
});
Add Toolbar Actions
Create a shared toolbar and add it to the main view:
Ext.define('ExtMail.view.messages.MessagesToolbarBase', {
extend: 'Ext.Toolbar',
buildItems: function() {
return [
{ xtype: 'button', tooltip: 'Refresh', iconCls: 'x-fa fa-redo', handler: this.makeHandler('refresh'), scope: this, bind: { hidden: '{!visibleMessageButtons.refresh}' } },
{ xtype: 'button', tooltip: 'Back', iconCls: 'x-fa fa-arrow-left', handler: this.makeHandler('back'), scope: this, hidden: true, bind: { hidden: '{!visibleMessageButtons.back}' } },
{ xtype: 'button', tooltip: 'Archive', iconCls: 'x-fa fa-archive', handler: this.makeHandler('archive'), scope: this, hidden: true, bind: { hidden: '{!visibleMessageButtons.archive}' } },
{ xtype: 'button', tooltip: 'Delete', iconCls: 'x-fa fa-trash', handler: this.makeHandler('delete'), scope: this, hidden: true, bind: { hidden: '{!visibleMessageButtons.delete}' } },
{ xtype: 'button', tooltip: 'Mark as Unread', iconCls: 'x-fa fa-envelope', handler: this.makeHandler('markunread'), scope: this, hidden: true, bind: { hidden: '{!visibleMessageButtons.markUnread}' } },
'->',
{ xtype: 'component', tpl: '{count} messages', data: {}, bind: { hidden: '{!visibleMessageButtons.messageCount}', data: { count: '{messages.count}' } } }
];
},
makeHandler: function(event) {
return function() {
this.fireEvent(event);
};
}
});
Ext.define('ExtMail.view.messages.MessagesToolbar', {
extend: 'ExtMail.view.messages.MessagesToolbarBase',
alias: 'widget.messages-MessagesToolbar',
initialize: function() {
this.setItems(this.buildItems());
this.callParent(arguments);
}
});
{
xtype: 'messages-MessagesToolbar',
docked: 'top',
listeners: {
refresh: 'onRefreshMessages',
back: 'onBackToMessagesGrid',
delete: 'onDeleteMessage',
markunread: 'onMarkMessageUnread',
archive: 'onArchiveMessage'
}
}
5. Modern Interface
Integrate a modern interface with sliding menus and compose functionality. Here are the steps:
Create the Sliding Menu
Ext.define('ExtMail.view.menu.Menu', {
extend: 'Ext.panel.Panel',
alias: 'widget.menu-Menu',
requires: ['ExtMail.view.labels.LabelsTree'],
defaultListenerScope: true,
scrollable: 'vertical',
layout: 'fit',
items: [
{
xtype: 'labels-LabelsTree',
style: { background: 'white' },
bind: { store: '{labels}', selection: '{selectedLabel}' },
listeners: { selectionchange: 'onLabelSelectionChange' }
}
],
onLabelSelectionChange: function() {
this.fireEvent('closemenu');
},
getReveal: function() { return true; },
getCover: function() { return false; },
getSide: function() { return 'left'; }
});
Setup Menu in Application
setupMenu: function() {
Ext.Viewport.setMenu(Ext.create('ExtMail.view.menu.Menu', {
width: 250,
viewModel: this.getMainView().getViewModel(),
listeners: {
closemenu: function() {
Ext.Viewport.hideMenu('left');
}
}
}));
}
Add Menu Button
Add a button to toggle the menu:
buildItems: function() {
var items = this.callParent(arguments);
items.unshift({
xtype: 'button',
iconCls: 'x-fa fa-list',
bind: { hidden: '{selectedMessage}' },
handler: this.onMenuButtonTap,
scope: this
});
return items;
},
onMenuButtonTap: function() {
if (Ext.Viewport.getMenus().left.isHidden()) {
Ext.Viewport.showMenu('left');
} else {
Ext.Viewport.hideMenu('left');
}
}
Create Floating Compose Button
Create and style a floating button for composing messages:
Ext.define('ExtMail.view.compose.ComposeButton', {
extend: 'Ext.Button',
alias: 'widget.compose-ComposeButton',
floated: true,
width: 60,
height: 60,
cls: 'compose-button',
iconCls: 'x-fa fa-edit'
});
// CSS (SASS) for Compose Button
.compose-button.x-button {
background: #d8372d;
border-radius: 50%;
position: absolute;
right: 40px;
bottom: 40px;
.x-icon-el { color: #FFF; }
}
Setup Compose Button in Application
setupComposeButton: function() {
this.composeButton = Ext.create('ExtMail.view.compose.ComposeButton', {
hidden: false,
handler: function() {
this.getMainView().getController().onComposeMessage();
},
scope: this
});
this.getMainView().getViewModel().bind('{selectedMessage}', function(selectedMessage) {
var setDelay = selectedMessage ? 0 : this.getMainView().getLayout().getAnimation().getDuration();
setTimeout(Ext.bind(function() {
this.composeButton.setHidden(selectedMessage);
}, this), setDelay);
}, this);
},
launch: function() {
this.setupMenu();
this.setupComposeButton();
}
Create and Integrate Compose Form
Ext.define('ExtMail.view.compose.ComposeForm', {
extend: 'ExtMail.view.compose.ComposeFormBase',
alias: 'widget.compose-ComposeForm',
defaultListenerScope: true,
padding: 10,
layout: { type: 'vbox', align: 'stretch' },
items: [
{
xtype: 'combobox',
placeholder: 'Recipient',
displayField: 'email',
valueField: 'email',
queryMode: 'local',
required: true,
bind: { store: '{contacts}', selection: '{selectedRecipient}', value: '{messageRecord.email}' }
},
{ xtype: 'textfield', placeholder: 'Subject', bind: { value: '{messageRecord.subject}' } },
{ xtype: 'textareafield', placeholder: 'Compose email', flex: 1, bind: { value: '{messageRecord.message}' } },
{
xtype: 'toolbar',
docked: 'bottom',
margin: 0,
items: [
{ xtype: 'button', scale: 'medium', text: 'Send', handler: 'onSendClick' },
'->',
{ xtype: 'button', iconCls: 'x-fa fa-trash', tooltip: 'Discard', handler: 'onDiscardClick' }
]
}
],
onSendClick: function() {
if (this.validate()) {
this.callParent(arguments);
}
}
});
6. Scalability
The transition from static JSON files to a RESTful API for better scalability. Here are the steps:
Create a Base URL Utility Class
Ext.define('ExtMail.util.BaseUrl', {
singleton: true,
requires: ['Ext.Ajax'],
config: { baseUrl: '' },
constructor: function(config) {
this.initConfig(config);
Ext.Ajax.on('beforerequest', this.onBeforeAjaxRequest, this);
},
onBeforeAjaxRequest: function(connection, options) {
options.url = this.getBaseUrl() + options.url;
}
});
Set Up the Base URL
Ext.application({
extend: 'ExtMail.Application',
name: 'ExtMail',
requires: ['ExtMail.*'],
onBeforeLaunch: function() {
ExtMail.util.BaseUrl.setBaseUrl('https://<your-base-url>/');
this.callParent(arguments);
}
});
Update Store Proxies
Change the proxy type from `ajax` to `rest` and update the URLs to point to your REST API endpoints.
proxy: {
type: 'rest',
url: 'data/contacts/',
reader: {
type: 'json',
transform: function(data) {
return Ext.Array.map(data.result, ExtMail.util.Object.snakeCaseToCamelCase);
}
}
}
Handle Data Transformation
Use the `transform` function to ensure compatibility between API responses and the application’s data structure.
reader: {
type: 'json',
transform: function(data) {
var transformRow = function(row) {
row = ExtMail.util.Object.snakeCaseToCamelCase(row);
if (row.children && Ext.isArray(row.children)) {
row.children = Ext.Array.map(row.children, transformRow);
}
return row;
};
return Ext.Array.map(data, transformRow);
}
}
Refactor Message Labels
Implement a `hasMany` association between `Message` and `MessageLabel` models to handle the normalized data structure.
hasMany: [{
model: 'ExtMail.model.MessageLabel',
name: 'labels',
storeConfig: { type: 'MessageLabels' }
}]
Configure the MessageLabels Store
Ext.define('ExtMail.store.MessageLabels', {
extend: 'Ext.data.Store',
alias: 'store.MessageLabels',
model: 'ExtMail.model.MessageLabel',
autoSync: true
});
Update Message Model Methods
hasLabel: function(labelId) {
return this.labels().findExact('labelId', labelId) >= 0;
},
addLabel: function(labelId) {
this.labels().add({ messageId: this.getId(), labelId: labelId });
},
removeLabel: function(labelId) {
var index = this.labels().findExact('labelId', labelId);
this.labels().removeAt(index);
}
7. Deep Linking and Routing
Add deep linking and router classes to the application. Here are the steps:
Add Routes Configuration
In your main controller, define the routes:
routes: {
'label/:label': {
name: 'label',
before: 'onBeforeViewLabel',
action: 'onViewLabel'
},
'view/:messageId': {
name: 'message',
before: 'onBeforeViewMessage',
action: 'onViewMessage'
},
'draft/:messageId': {
name: 'draft',
action: 'onDraftMessage'
}
}
Define Before Handlers
Ensure data is loaded before routing:
onBeforeViewLabel: function(label, action) {
var labelsStore = this.getViewModel().getStore('labels');
if (labelsStore.loadCount > 0) {
action.resume();
} else {
labelsStore.on('load', function() { action.resume(); }, this, { single: true });
}
},
onBeforeViewMessage: function(messageId, action) {
var store = this.getViewModel().getStore('messages');
if (store.loadCount > 0) {
action.resume();
} else {
store.on('load', function() { action.resume(); }, this, { single: true });
}
}
Define Action Handlers
Update the application state based on the route:
onViewLabel: function(label) {
var labelsStore = this.getViewModel().getStore('labels');
var labelRecord = labelsStore.findRecord('slug', label) || labelsStore.first();
this.getViewModel().set('selectedMessage', null);
this.getViewModel().set('selectedLabel', labelRecord);
},
onViewMessage: function(messageId) {
var store = this.getViewModel().getStore('messages');
var messageRecord = store.getById(messageId);
this.getViewModel().set('selectedMessage', messageRecord);
if (!messageRecord) this.redirectTo({ message: null });
},
onDraftMessage: function(messageId) {
var messageRecord = this.getViewModel().getStore('messages').getById(messageId);
if (!messageRecord) {
this.redirectTo({ draft: null });
} else {
this.showDraftWindow(messageRecord);
}
}
Update UI Interaction
Trigger route changes from the UI as under:
handleMessageClick: function(messageRecord) {
var destination = {};
if (messageRecord.get('draft')) {
destination = { draft: `draft/${messageRecord.getId()}` };
} else {
destination = { message: `view/${messageRecord.getId()}` };
}
this.redirectTo(destination);
},
onLabelSelectionChange: function(labelTree, selectedLabelRecords) {
var selectedLabelRecord = selectedLabelRecords[0];
var slug = selectedLabelRecord ? selectedLabelRecord.get('slug') : '';
this.redirectTo({ label: `label/${slug}`, message: null });
}
Add Slug Field to Labels
Ensure labels have URL-friendly names:
{
name: 'slug',
calculate: function(data) {
return (data.name || '').toLowerCase().replace(/ /g, '-').replace(/[^\w-]+/g, '');
}
}
What Makes a Great Email Client?
A great email client excels in several key areas, such as:
- Simple, intuitive design that allows users to navigate effortlessly.
- Supports various email protocols (IMAP, POP3, SMTP) and integrates smoothly with multiple email services.
- Offers customization for layout, themes, and notifications to suit user preferences.
- Includes robust security measures such as encryption, spam filtering, and phishing protection.
- Provides powerful search tools to quickly find specific emails, attachments, and contacts.
- Integrates with calendars, task managers, and other productivity apps for streamlined workflows.
- Allows users to access and manage emails without an internet connection.
- Receives frequent updates for performance improvements, new features, and security patches.
- Offers reliable customer support to assist users with any issues or queries.
- Ensures fast load times and minimal lag, even with large volumes of emails.
How to Choose a Gmail Alternative That Meets Your Needs?
When selecting a Gmail alternative, consider the following factors:
👉Ensure the email service provides robust encryption and privacy protections.
👉Look for essential features such as spam filtering, organizational tools, calendar integration, and contact management.
👉Choose an email client with an intuitive and user-friendly interface.
👉Ensure compatibility with your devices and other software you use.
👉Opt for a service with good customer support and high reliability.
Frontend Development for Email Application Client
Frontend development for an email client involves designing and implementing the user interface. This includes creating components for reading, composing, and organizing emails. It requires knowledge of HTML, CSS, and JavaScript. You also need frameworks like Ext JS to build dynamic and responsive interfaces.
Building a Responsive Drag and Drop UI
- To build a responsive drag-and-drop UI, use JavaScript libraries like Dragula or interact.js.
- Ensure the UI is intuitive and responsive across different devices.
- Implement drag-and-drop events to enhance user interactions. Some examples are sorting emails or moving them between folders.
Backend Development for Email Application Client
Backend development involves setting up a server to handle email sending, receiving, and storage.
- Use RESTful APIs to communicate with the frontend.
- Ensure the backend supports protocols like IMAP, POP3, and SMTP.
- Implement security measures like encryption and authentication.
Ext JS Email Application Client
Ext JS is a powerful JavaScript framework used to build data-intensive applications. For an email client, Ext JS offers features like:
👉Data binding
👉Advanced UI components
👉State management
👉It helps in creating a rich, interactive, and responsive email client interface.
Apart from that, Ext JS also offers:
Mature and well-supported framework. It ensures that your email client is stable and reliable, which is essential for daily use.
It allows for creating an email client that is not only functional but also visually appealing and user-friendly.
Ext JS is known for its performance. It can handle large amounts of data efficiently, ensuring that even inboxes with thousands of emails remain responsive.
The framework allows for extensive customization. Users can tailor the email client to their specific needs, adding features like custom labels, filters, and workflows.
It supports multiple platforms, including desktops and mobile devices. This ensures a consistent experience across different devices.
Ext JS has a large community and extensive documentation. Users can find help easily, whether through community forums, tutorials, or official support channels.
Ext JS can scale with you as your needs grow. Whether you’re managing a small personal inbox or a large corporate email system, Ext JS can handle it.
Email Application: Conclusion
Creating an email app involves several key steps. Ext JS is a great tool for this because it offers advanced UI features and efficient state management. The process includes setting up your environment, implementing core features, optimizing for mobile, transitioning to a RESTful API, and adding deep linking. This ensures your email app is scalable, user-friendly, and meets various user needs. With this approach, you can build a robust and dynamic email application.
Email Application: FAQs
What is an Email Application?
An email application is software for managing, sending, and receiving emails.
Why Should We Build an Alternative Email Application for Gmail?
To meet unique user needs, enhance security, and offer better features.
Is It Safe to Trust Ext JS for Creating an Email App?
Ext JS is safe for creating an email app due to its robust features.
How Much Does it Cost to Create an Email App via Ext JS?
The cost to create an email app via Ext JS varies based on requirements and scale. The pro plan costs $6475, while the Enterprise plan costs $9475.
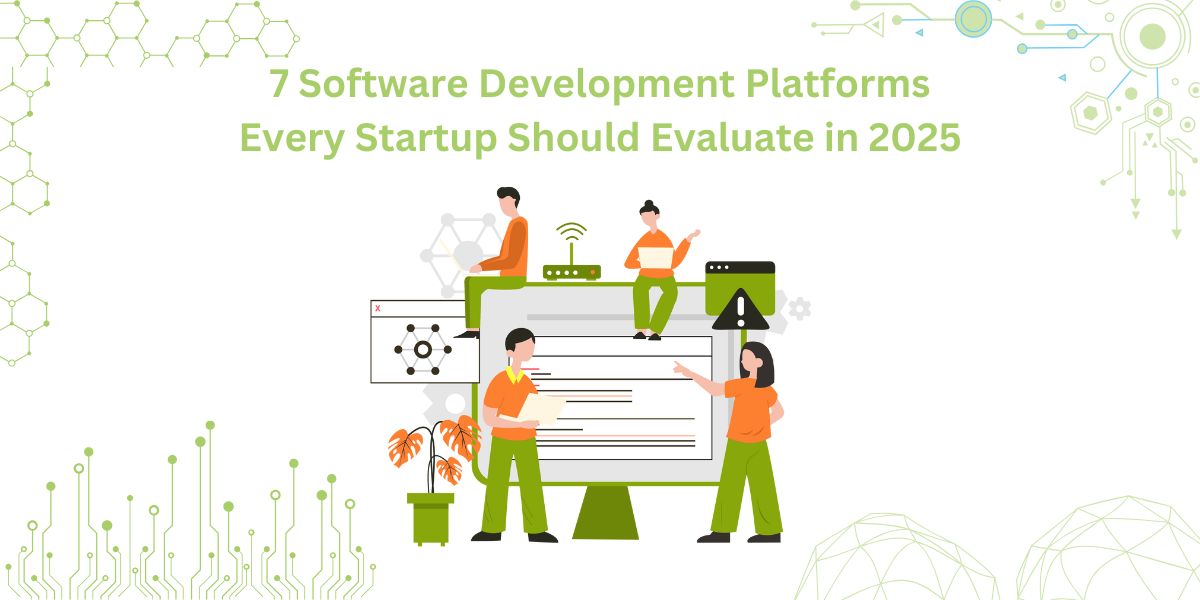
Did you know that nearly 90% of startups fail? And one of the biggest reasons…
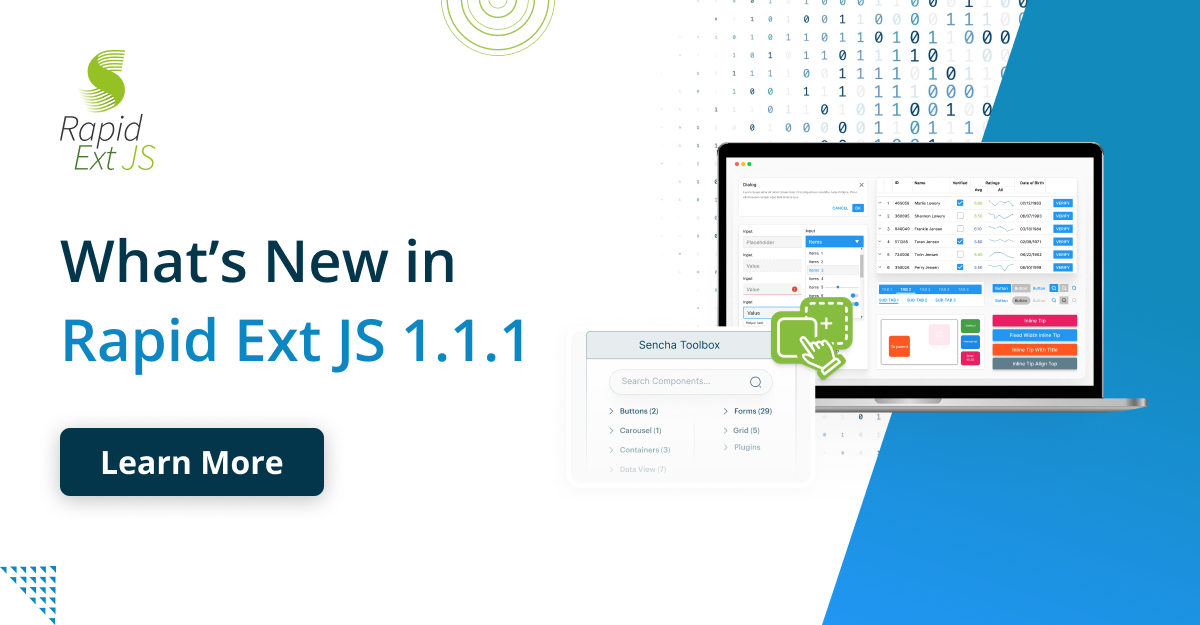
The Sencha team is excited to announce the latest Sencha Rapid Ext JS 1.1.1 release…
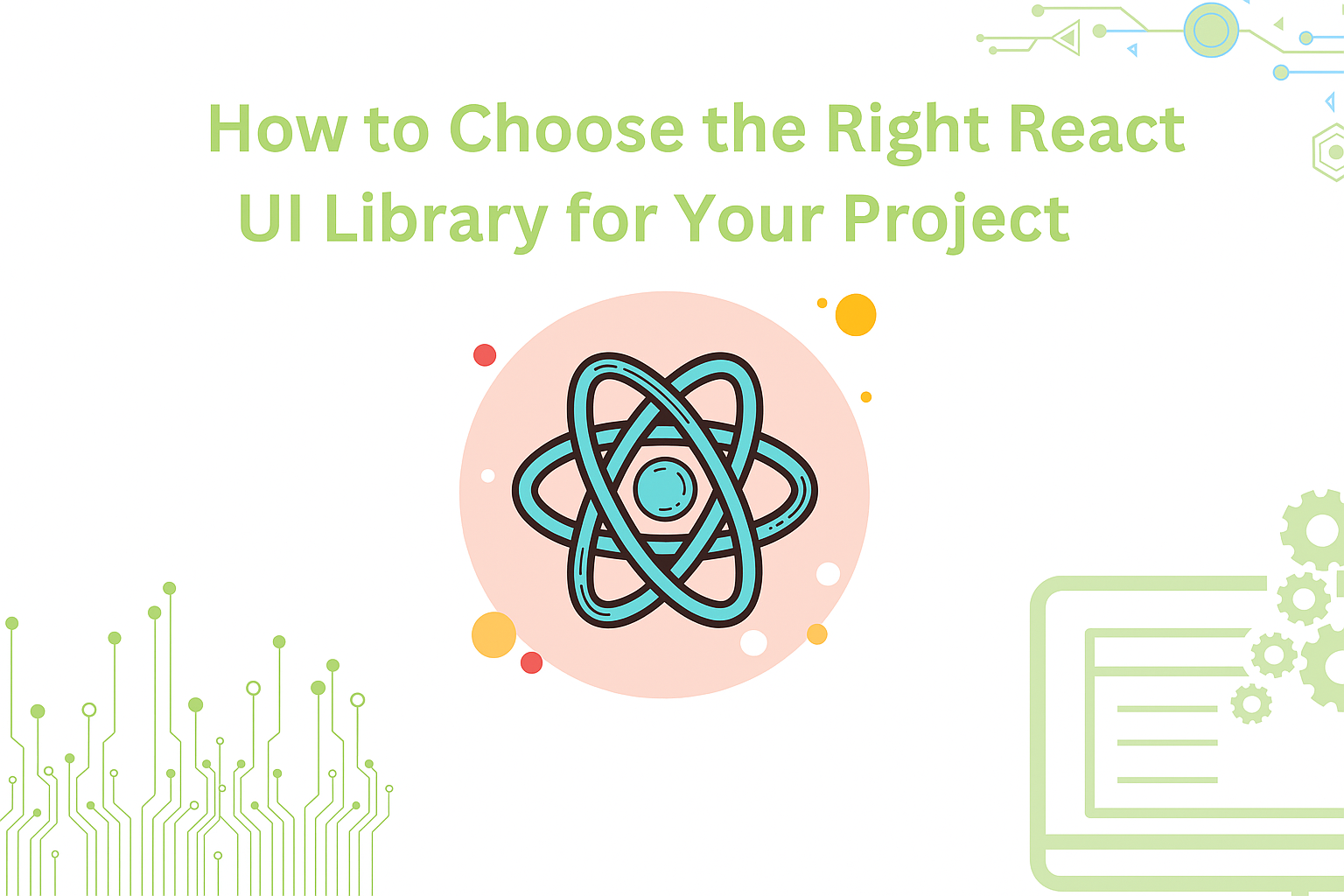
React is perhaps the most widely used web app-building framework right now. Many developers also…