How to Create React App Typescript?
React is one of the most popular and effective front-end libraries that uses a component-based approach to build web-based applications. However, TypeScript is a strict, statically typed programming language that enhances the predictability and robustness of JavaScript code. One of the key motivations for the creation of TypeScript was to enable developers to create products that are always predictable in terms of their behavior. Applications in React are typically created with the create-react-app command. On the other hand, TypeScript integrates seamlessly with React through a specific template, allowing developers to combine the flexibility of React with the structure and security of TypeScript.
In this article, we will explore how to use TypeScript in your React project and discuss several best practices for integrating TypeScript into your development workflow. Whether you are starting a new React project or adding TypeScript to an existing one, you’ll find valuable insights and detailed examples for effective development.
Create React App With TypeScript
Just like setting up a standard React application, TypeScript applications also utilize create-react-app, but with a special TypeScript template. This template pre-configures TypeScript for you, saving a significant amount of setup time and effort. Here’s how you can quickly set up a React app with TypeScript:
npx create-react-app ts-app --template typescript
This command will scaffold a React TypeScript application for you and create a file structure similar to what you would see in a typical JavaScript-based React app. If you are familiar with React JS with TypeScript structures, the setup process will feel intuitive.
The beauty of React with TypeScript is that it provides you with type-checking from the start, helping avoid runtime errors before they even occur. Additionally, using TypeScript provides better support for code completion and easier refactoring, especially when working in large teams or handling more complex React projects. This integration makes React apps more maintainable and more robust over time.
How to Create a React App with TypeScript
React is one of the most popular and effective front-end frameworks, utilizing a component-based approach to build web-based applications. When paired with TypeScript, a statically typed programming language, React development becomes even more robust. TypeScript enhances the predictability of JavaScript code, allowing developers to catch potential errors early, resulting in cleaner, more maintainable code. By integrating TypeScript with React, you get the flexibility of React alongside the structure and security TypeScript provides.
Here’s how you can create a React app with TypeScript:
Step 1: Create React App with TypeScript Template
To set up a React application with TypeScript, you use the create-react-app command, but with a special TypeScript template. This template comes pre-configured, so you don’t have to manually set up TypeScript. Here’s the command to run in your terminal:
npx create-react-app ts-app --template typescript
This command will generate a new React app with TypeScript, ensuring that your file structure is organized and TypeScript is ready to go. You can then navigate to the project folder with:</
cd ts-app
The beauty of using React with TypeScript is that it provides type-checking right from the start. This helps you avoid runtime errors before they even happen, ensuring that your app is stable as development progresses.
Step 2: Install TypeScript in an Existing Project
If you’re adding TypeScript to an existing React project, you can follow these steps:
Install TypeScript and necessary type definitions:
For npm:
npm install --save typescript @types/node @types/react @types/react-dom @types/jest
For yarn:
yarn add typescript @types/node @types/react @types/react-dom @types/jest
- Rename JavaScript files to TypeScript files: Change your .js files to .tsx files if they contain JSX, or .ts if they don’t.
- Create a tsconfig.json file: This file configures the TypeScript compiler for your project. Here’s an example of what it should look like:
{ "compilerOptions": { "target": "es6", "lib": ["dom", "dom.iterable", "esnext"], "allowJs": true, "skipLibCheck": true, "esModuleInterop": true, "allowSyntheticDefaultImports": true, "strict": true, "forceConsistentCasingInFileNames": true, "noFallthroughCasesInSwitch": true, "module": "esnext", "moduleResolution": "node", "resolveJsonModule": true, "isolatedModules": true, "noEmit": true, "jsx": "react-jsx" }, "include": ["src/**/*"] }
- Restart your development server: After making these changes, restart your development server to ensure everything is working as expected.
Step 3: Start Building with TypeScript
Once your React app with TypeScript is set up, you can start leveraging TypeScript’s powerful features. From typing props and state to adding type annotations to event handlers and functions, TypeScript enhances every part of your development process. This integration ensures that your React app will be more maintainable, scalable, and less prone to runtime errors.
Common Errors When Creating a React TypeScript Project
Errors are bound to happen during the setup process. If you encounter any issues, especially during initial installation, try forcing the React app to install again using the command:
npx create-react-app@latest my-ts-app --template typescript
For developers adding TypeScript to an existing create-react-app project, you can use the following commands to install TypeScript and its required type definitions:
For npm:
npm install --save typescript @types/node @types/react @types/react-dom @types/jest
For yarn:
yarn add typescript @types/node @types/react @types/react-dom @types/jest
Once TypeScript is installed, rename any .js file extensions to .tsx and create a tsconfig.json configuration file in the root of your project. Below is an example of a tsconfig.json file, which controls the behavior of the TypeScript compiler for your project:
{
"compilerOptions": {
"target": "es6",
"lib": ["dom", "dom.iterable", "esnext"],
"allowJs": true,
"skipLibCheck": true,
"esModuleInterop": true,
"allowSyntheticDefaultImports": true,
"strict": true,
"forceConsistentCasingInFileNames": true,
"noFallthroughCasesInSwitch": true,
"module": "esnext",
"moduleResolution": "node",
"resolveJsonModule": true,
"isolatedModules": true,
"noEmit": true,
"jsx": "react-jsx"
},
"include": ["src/**/*"]
}
It’s always a good idea to restart your development server and IDE to ensure everything is working as expected. Once the setup is complete, run the following command to start the application:
npm start
Boost Your React Development with TypeScript
Now that you know how to create a React app Development with TypeScript, it’s time to take full advantage of the additional features and capabilities TypeScript brings. Here are some key practices for integrating TypeScript into your React project, especially when building more complex apps or working with React Native TypeScript.
When building large-scale applications or working in teams, using React with TypeScript can save you significant time and effort. It allows you to catch errors earlier in the development process, ensuring the app’s stability. Additionally, TypeScript improves code readability and collaboration, making it easier for team members to understand and contribute to the codebase.
Typing Props in a React TypeScript Project
TypeScript shines when it comes to defining object/data types. It provides more precision in your code, and one of the best uses of TypeScript in React is typing props for components. This helps avoid errors and ensures that the right data is passed to your components.
Here’s how you can define prop types using TypeScript:
type IProps = {
disabled: boolean,
title: "Hello" | "Hi"
};
interface Props {
disabled: boolean,
title: string
}
const propsTypes: IProps = {
disabled: false,
title: "Hello"
};
An example of an interface component is as follows:
import React from 'react';
interface EmployeeProps {
name?: string;
age?: number;
country: string;
children?: React.ReactNode;
}
function Employee({name = 'Alice', age = 29, country}: EmployeeProps) {
return (
< div>
< h2>{name}< /h2>
< h2>{age}< /h2>
< h2>{country}< /h2>
< /div>
);
}
export default function App() {
return (
< div>
< Employee country="Austria" />
< /div>
);
}
Notice that the name and age props are optional because they are marked with a ? in the EmployeeProps interface. This allows you to omit them when using the component. Using TypeScript with React props ensures that your components are more maintainable and reduces the risk of passing incorrect data types.
Inserting State with the UseState Hook in React TypeScript
One of the key features of React is its hooks, and useState is one of the most commonly used hooks. When using TypeScript with React, you can ensure type safety by specifying the type of your state directly. This eliminates common bugs related to incorrect state types and enhances code readability.
Here’s an example of how to type the state using useState:
import { useState } from 'react';
function App() {
const [strArr, setStrArr] = useState< string[]>([]);
const [objArr, setObjArr] = useState< {name: string; age: number}[]>([]);
setStrArr(['a', 'b', 'c']);
setObjArr([{ name: 'A', age: 1 }]);
return (
< div className="App">
< div>Hello world< /div>
< /div>
);
}
export default App;
In this example, we define the state types for strArr as an array of strings (string[]), and objArr as an array of objects that have name and age properties. By doing this, TypeScript will alert you if you try to assign a value that doesn’t conform to the expected type.
Typing Events in React TypeScript
React provides a variety of events such as onClick and onChange. TypeScript can help you handle these events more safely by typing them. This is especially useful when working with React event handlers and ensures that you handle the events correctly.
Here’s an example of how you can type an event handler for a click event:
const App = () => {
const handleClick = (event: React.MouseEvent< HTMLButtonElement, MouseEvent>) => {
console.log(event.target);
console.log(event.currentTarget);
};
return (
< div>
< button onClick={handleClick}>Click< /button>
< /div>
);
};
export default App;
In this code, the handleClick function receives a typed event argument. TypeScript will infer the correct type for the event based on the DOM element, in this case, an HTMLButtonElement. This improves type safety and reduces errors related to incorrect event handling.
React Component TypeScript
When working with React components in TypeScript, you must ensure that your components are typed correctly, whether you’re using functional components or class components. For React function components TypeScript, you can use interfaces or types to define props and state, which helps to make the codebase more predictable and maintainable.
Example of a React function component TypeScript:
interface Props {
title: string;
}
const MyComponent: React.FC< Props> = ({ title }) => {
return < h1>{title}< /h1>;
};
In this example, MyComponent is typed to accept a title prop, and the type of this prop is strictly enforced by TypeScript.
React Native with TypeScript
If you’re developing React Native TypeScript applications, the process of adding TypeScript is very similar to that of React for the web. TypeScript adds type safety to your React Native projects, making it easier to handle complex mobile development tasks. You can start by setting up a new React Native project with TypeScript using the following command:
npx react-native init MyApp –template react-native-template-typescript
This will generate a React Native TypeScript project where TypeScript is pre-configured, allowing you to take advantage of static typing from the very beginning. You can also add TypeScript to an existing React Native project by installing the necessary TypeScript dependencies and renaming your .js files to .tsx.
Using TypeScript in React Native projects provides several advantages, such as better error checking, improved code completion, and a more predictable development process. With TypeScript’s strict typing system, you can easily manage complex state structures and optimize your app’s performance, whether you’re working on a React Native TypeScript or React Web TypeScript project.
React Testing Library with TypeScript
When working with React Testing Library, you can integrate TypeScript for a stronger typing system in your tests. TypeScript helps catch type-related errors during testing, ensuring that you are testing your components correctly. For example, here’s how to write tests using React Testing Library and TypeScript:
import { render, screen, fireEvent } from '@testing-library/react';
import MyComponent from './MyComponent';
test('renders a button and fires click event', () => {
render(< MyComponent />);
const button = screen.getByRole('button');
fireEvent.click(button);
expect(screen.getByText(/clicked/i)).toBeInTheDocument();
});
By typing the tests with TypeScript, you can make your testing process more reliable and robust, reducing the chances of runtime errors in your React app.
Additional Tips for Setting Up React TypeScript Projects
When working with React TypeScript projects, it’s helpful to follow best practices to ensure smooth development:
- Consistent Typing: Always define types or interfaces for props, state, and events to catch issues early and improve maintainability.
- Use useRef with TypeScript: TypeScript provides great support for typing refs, which is crucial when accessing DOM elements in a React component.
- Type Your Functions: Ensure that all of your functions, including event handlers and callbacks, are typed correctly to avoid bugs.
- Enable Strict Mode: TypeScript’s strict mode enforces better type checking and helps prevent many common issues.
FAQs
Can I use TypeScript with React?
Yes, React fully supports TypeScript. TypeScript enhances React development by adding static typing to JavaScript, which helps catch errors early during development.
Can React use TypeScript?
Yes, React works very well with TypeScript. You can either create a new React app with TypeScript using the create-react-app TypeScript template or add TypeScript to an existing React project.
Can TypeScript be used with React?
Absolutely! TypeScript can be easily integrated into React projects, whether you’re starting from scratch or adding TypeScript to an existing React app.
Can you mix TypeScript and JavaScript in React?
Yes, you can mix TypeScript and JavaScript in a React project. You can gradually introduce TypeScript into a JavaScript project by renaming files from .js to .tsx and adding type annotations over time.
Does React support TypeScript?
React natively supports TypeScript, and it has extensive documentation and community support for TypeScript-based projects.
How to add TypeScript to an existing React project?
To add TypeScript to an existing React project, install TypeScript and type definitions via npm or yarn, rename .js files to .tsx, and create a tsconfig.json file in the root of your project.
How to build a component library with React and TypeScript?
Building a react ui component library with React and TypeScript involves creating reusable components, writing TypeScript types or interfaces for props, and ensuring that your components are well-documented and easy to use. Use create-react-library or similar tools to help streamline the process.
How to create a functional component in React with TypeScript?
To create a functional component in React with TypeScript, define your component using a function and specify the props type through interfaces or types. Here’s an example:
interface Props {
title: string;
}
const MyComponent: React.FC< Props> = ({ title }) => {
return < h1>{title}< /h1>;
};
How to create a React app with TypeScript in VS Code?
You can create a React app with TypeScript in Visual Studio Code by opening the terminal and running the following command:
npx create-react-app my-app --template typescript
How to use React Navigation with TypeScript?
To use React Navigation with TypeScript, install react-navigation and its dependencies, then type your navigation props using TypeScript interfaces. This ensures that your navigation structure and props are well-typed.
Conclusion
In this article, we’ve covered how to set up a React app with TypeScript and explored some common practices for working with TypeScript in React, including typing props, state, events, and more. React and TypeScript together make an excellent pairing for building robust, predictable applications. Whether you’re building a React Native TypeScript app or a simple React component TypeScript project, TypeScript provides the static typing and error reduction that can save time and improve code quality.
Create an account at ReExt and create stunning React projects today.
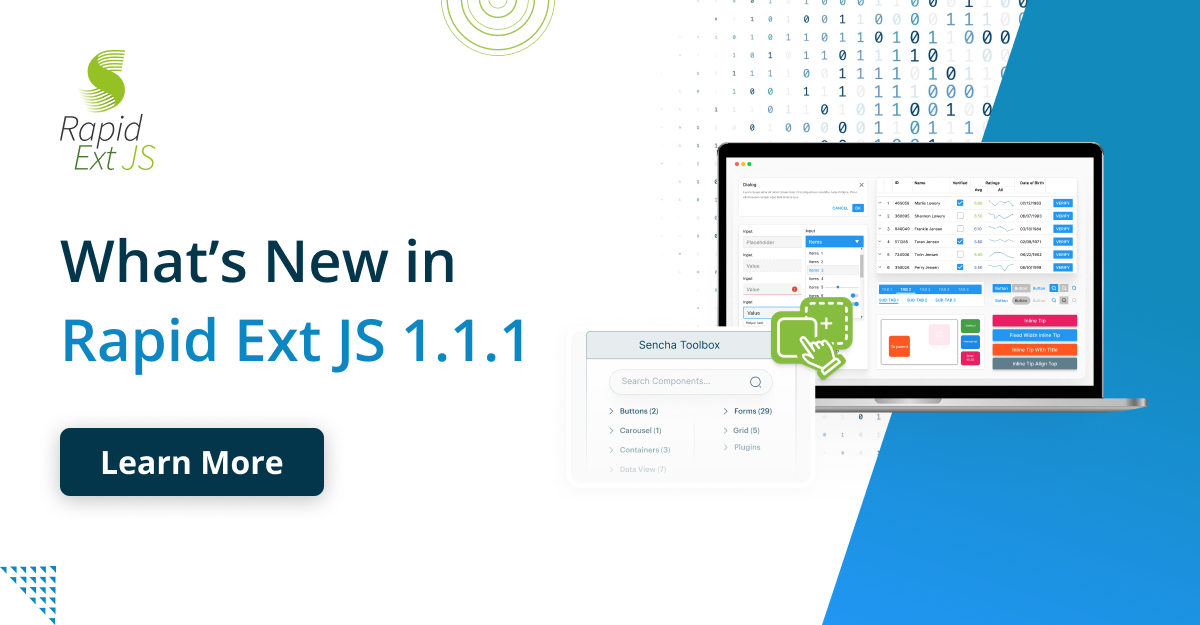
The Sencha team is excited to announce the latest Sencha Rapid Ext JS 1.1.1 release…
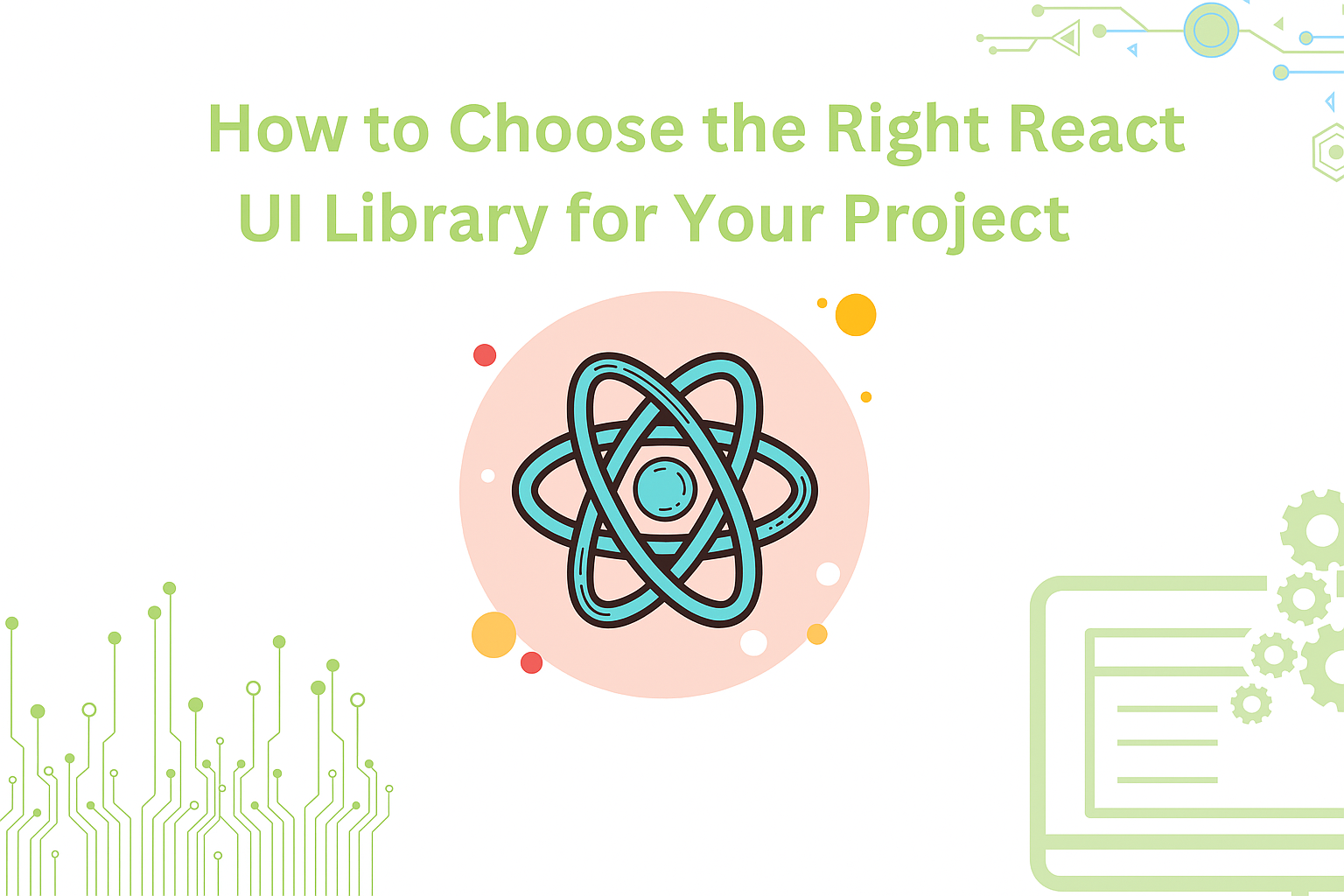
React is perhaps the most widely used web app-building framework right now. Many developers also…
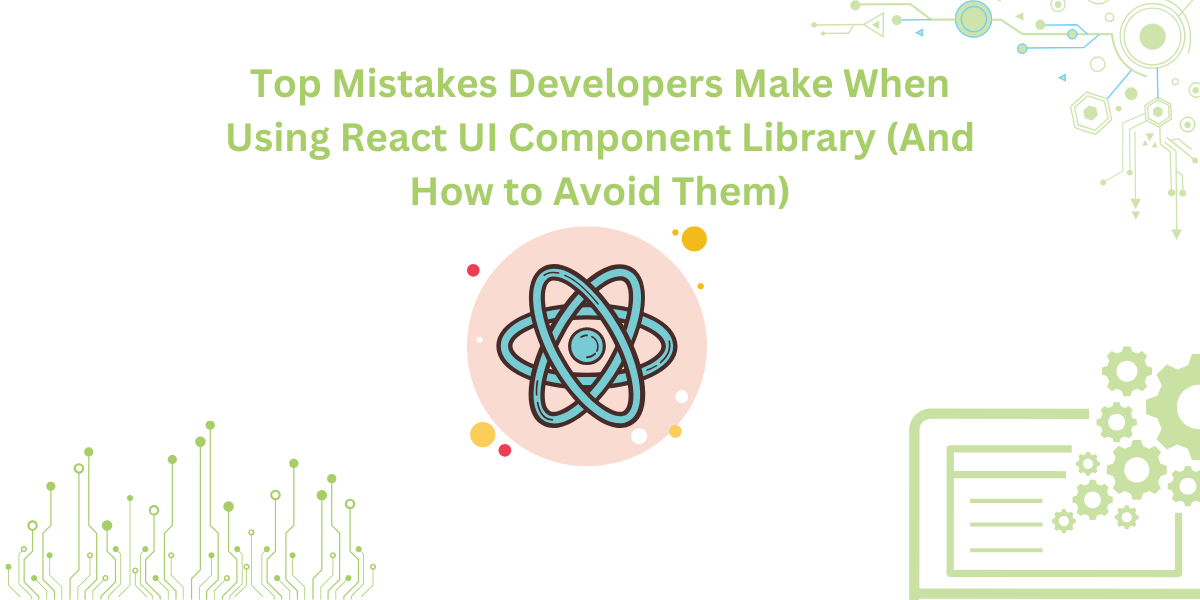
React’s everywhere. If you’ve built a web app lately, chances are you’ve already used it.…