Learn how to build React based web applications with the Sencha ReExt
Did you know that over 65% of developers prefer React for modern web applications? React 16 introduced powerful features like asynchronous rendering and error boundaries. These features improve performance and give developers better control over large applications.
Data grids are a key part of data-driven web apps. They help display large datasets in an easy-to-use way. However, building a grid from scratch is tough. It requires deep React knowledge and DOM manipulation skills. That’s where Sencha’s ReExt Grid comes in.
In this blog, we’ll show you how to use React 16 with ReExt Grid. First, we’ll guide you through setting up a React project. Then, we’ll cover integrating the ReExt Grid step by step. You’ll learn how to add sorting, filtering, and data export features without extra coding. We’ll also discuss how to optimize performance when handling large datasets.
Whether you’re new to React or have experience, this guide will help you build scalable data apps. Let’s get started!
Why Should You Create a React Web Application?
Here are the reasons to create a React web application:
Faster Web Development
One of React’s main advantages is faster web development. It allows developers to reuse components already built for other apps. This eliminates the need to write the same code for similar features repeatedly. As a result, the development process becomes quicker and more efficient. This leads to improved productivity, especially when managing multiple projects.
High-Performance Applications
React is known for building high-performance apps. It uses a virtual DOM (Document Object Model) that exists in memory. Instead of modifying the browser’s DOM directly, it first updates the virtual DOM. This process reduces the time and resources needed to render changes. The result is faster, smoother app performance, even with complex data and interactions.
High Flexibility
React’s modular structure adds flexibility to the development process. You can make changes to one part of the app without affecting other areas. This makes maintenance and updates much easier to manage. Additionally, React isn’t limited to web development. It also supports mobile app development, which expands its versatility for developers working on different platforms.
React is a preferred framework for developers due to its many benefits.
- It speeds up development, enhances app performance, and offers a flexible, modular structure.
- Its ability to reuse components and efficiently manage updates makes it a strong choice.
- Plus, its support for both web and mobile apps makes it an ideal tool for building dynamic, high-performance applications.
What is React 16?
React 16 is the first version of React built on the new core architecture called “Fiber.” It was designed from the ground up to support asynchronous rendering. This allows React to process large component trees without blocking the main execution thread.
React 16 comes with several key features. It introduces error boundaries for catching exceptions. It also allows returning multiple components from render. File sizes are reduced, and it supports the MIT license.
If you’re developing a data-driven web application with React 16, a grid or spreadsheet interface will likely be essential. Users often expect grids to have certain capabilities. These include scrolling with a fixed header, sorting by clicking column headers, and showing or hiding columns. Other common features include paging, grouping, cell editing, exporting data to Excel, and expanding rows for more details.
Building a grid in React can be tricky. It requires significant React expertise and the ability to dig into the DOM. Fortunately, ReExt Grid provides all these features and more. In this article, we’ll create an example using the Sencha ReExt Grid to display stock and equities data. With ReExt Grid, features like sorting, resizing, and paging come built-in. Let’s get started building an application using the ReExt Grid!
Getting Started with ReExt App Generation
To start developing a React application with ReExt components, follow these steps:
Set up your Node environment
First, ensure you have Node 8.11+ and NPM 6+ installed on your system. Download the latest Node version from the Node website if needed. If you already have Node installed, check the versions by running these commands:
Copy code
node -v
npm -v
Get your login credentials for the ReExt npm repo
ReExt npm packages are hosted on Sencha’s private npm repo. To access these packages, log in to the repo. Visit the ReExt 30-Day Free Trial page, fill out the form, and receive an email with login credentials and useful resources, including docs and sample projects.
Log in to the ReExt npm repo and install the ReExt app generator
Log in to Sencha’s private npm repo by running this command:
npm login — registry=http://npm.sencha.com — scope=@sencha
Next, install the ReExt app generator package:
npm install -g @sencha/ext-react-gen
Create your first React app.
Use the Yeoman generator to create your first ReExt app:
ext-react-gen app your-app-name-here -i
Follow the prompts to name your app, choose the npm package, and select a theme (Material theme is a good starting point). The generator will create a new directory and download the necessary files.
Run your React app
Navigate to your new app directory and run the following command:
npm start
This will launch your empty React app, which will display the app’s title. The main component will be a full-screen container with a layout set to “fit.” See the full code on GitHub.
Adding Grid to the application
Here are the steps that you should follow:
Add Stocks Data
We’ll be adding a sample data set called stocks.json to the application. This data set is fairly large, with around 10,000 rows in JSON format.
Each row represents a company or ticker symbol. It includes details such as the company name, ticker symbol, sector, and industries.
Additionally, it contains an array of ticks, representing the last five sales of the stock.
This is the data we’ll display in our grid. In a real-world application, this data would come from the back end. However, we’ll load this sample statically. It will still be loaded like you would fetch it from a real back-end API.
Creating a Basic Grid
In the StockGrid component’s render method, we will create a grid with columns. To define the columns, we use the Column component. Each column requires a dataIndex, which corresponds to the field name in the stocks.json data. The text prop defines the column header. You can also specify the width of the column using fixed or flexible widths, or a combination of both.
We will add column components for the company name, symbol, ticks, sector, and industry. Below is the setup for the new StocksGrid class:
// Your StocksGrid class code goes here
Add StockGrid to Class App
Now, we need to add StockGrid to the App component. Here’s how to do it:
export default class App extends Component {
render() {
return (
// Include StockGrid component here
);
}
}
See the GitHub code to check the code up to this step.
Binding Stock Data with Grid
In ReExt, a grid is a data table that pulls in and renders data from an Ext Data Store. The store is a data structure that allows sorting and filtering for the grid or other components like lists or charts.
Now, we will load the stocks data and create a store. The grid always retrieves its data from the store. Some interactions, like sorting, reloading, or paging, will trigger events on the store.
Creating the Store
Let’s create our store to handle the data. ReExt’s store is different from the typical Flux store in React. The key difference is the tight integration between the grid and the store.
You can pass data directly to the store. Alternatively, the store can pull data from a back-end using a proxy. With ReExt Grid, you get built-in interactive functionality, including sorting, paging, filtering, and grouping.
For this example, we’ll pass the stock data directly into the store. If you use a proxy, it enables features like remote paging, filtering, and sorting. We will also set autoLoad to true, so the grid loads the data automatically. Sorting is done on the client-side based on the “name” property.
this.store = new Ext.data.Store({
data: stocks,
autoLoad: true,
sorters: [{
property: 'name'
}],
listeners: {
update: this.onRecordUpdated
}
});
Assign the Store to the Grid
In the grid, assign the store configuration to the store we just created. This will allow the grid to display and interact with the data. Now, we have a fully functional grid displaying all the stock data.
Basic Grid with Data
With this simple code, you get many features for free. One of those features is sorting. You can click on any column header, and it will automatically sort the data. For example, in the symbol column, the sorting happens on the client side.
If we were using a real back-end API, we could configure the proxy for remote sorting. We would use an “order by” clause in the database to achieve this. You also get resizable columns without extra code. Even though we set a specific width for the columns, users can resize them by dragging the column edges.
Another useful feature is grouping. You can group the data by a specific field, such as industry. The grid will then organize the data by that field’s values. It also provides a pinned header for each group as you scroll through the data.
Grouping by Industry
You’ll notice that the data renders quickly, even with 10,000 records. This speed is due to a technique called buffered rendering.
Although the table appears fully loaded, it only initially renders a small portion. This portion corresponds to what is visible in the “viewport height.”
As you scroll, the grid replaces the content of the cells with new records. This happens dynamically as you page down in the store.
Conserving DOM elements keeps the DOM small. A smaller DOM uses less memory, which ensures high performance. The code for this step can be viewed on GitHub.
Styling your Grid
There are multiple ways to style the Grid to make data easier to analyze.
Using the Cell Prop
Let’s start with controlling the styling of individual cells. For example, we can make the “Name” field bold. The best way to do this is by using the cell prop. The cell prop takes various configurations that control the appearance of the cell. In this case, we’ll apply a style that sets the fontWeight to bold:
cell={{ style: { fontWeight: 'bold' } }}
Adding a Button in a Row
Now, let’s add something more practical. Imagine you want to add a button that lets you buy a stock. This button will appear in the left-hand side column.
To do this, we add a column. This time, we don’t need to use a dataIndex because the button doesn’t correspond to any data field. Instead, we use a WidgetCell and place a button inside it. We can style the button to have a round action look:
// Example of WidgetCell with button
Handling the Button Click
Next, we define a simple buy handler. When the button is clicked, it will trigger an action. We’ll use ext.toast to display a toast message at the top. The message will show the symbol of the stock being purchased:
buyHandler = (button) => {
let gridrow = button.up('gridrow'),
record = gridrow.getRecord();
Ext.toast(`Buy ${record.get('name')}`);
}
This function will be called whenever the button is clicked. It fetches the record and shows the stock name in a toast message.
Adding a Button in a Grid
From this example, you can see that you can embed any ReExt component inside a grid cell. These components remain fully interactive. They function just like any other React component.
You can check the code up to this step on GitHub for more details.
Adding a Trends Sparkline Chart
In the Stock Data, we have an array of ticks representing the last five stock sales. Let’s embed this data as a Sparkline chart inside the grid.
We will use widgetcell to render the ReExt component inside a grid cell. This will display the chart within each cell.
As you hover your mouse over different points in the line graph, it will show the Y value. The value will be formatted with two decimal points for precision.
Trends Chart in a Grid
See the code up to this step on GitHub.
Exporting Data to Excel
In data-intensive applications, exporting data to Excel is a common requirement. ReExt simplifies this using the plugins prop for grids.
To add export functionality, we’ll include a few additional components. First, we’ll add a titlebar and dock it at the top of the grid. Then, we’ll include a menu inside the titlebar. When you click the export button, you can choose to export data to either Excel or CSV.
The export handler passes the type of export and the file extension:
export = (type) => {
this.grid.cmp.saveDocumentAs({ type, title: 'Stocks' });
}
Make sure you have the exporter dependency in package.json. For example:
"@sencha/ext-exporter": "~6.6.0"
Install the dependency using npm install and start the app with npm start. The exporter supports formats like XSLX, Excel XML, HTML, and CSV/TSV.
Exporting Grid Data
See the code up to this step on GitHub.
Adding Editing Capability to a Grid
We can make the grid more like a spreadsheet by enabling data editing. To do this, we’ll add the gridcellediting plugin. By marking columns as editable, users can double-click on any grid cell to edit the data. They can also tab through the cells to continue editing.
gridcellediting: true
Handling Edit Events
If you need to perform actions after editing, listen to the update event in the store. This event passes parameters like the store, updated record, and changed field names. In this example, we show a toast message when data is updated:
listeners: {
update: this.onRecordUpdated
}
onRecordUpdated = (store, record, operation, modifiedFieldNames) => {
const field = modifiedFieldNames[0];
Ext.toast(`${record.get('name')} ${field} updated to ${record.get(field)}`);
}
Adding a Select Option to a Grid Cell
You can also add a SelectField to a grid cell. Just place the SelectField ReExt component inside the required column to offer selection options.
Optimizing Cross-platform Experience
This setup works well for desktop, but editing on mobile may not be ideal. Use the platformConfig option to define different behavior for desktop and mobile. For desktop, we use gridcellediting, and for mobile, we use grideditable for a better editing experience:
platformConfig={{
desktop: { plugins: { gridexporter: true, gridcellediting: true }},
'!desktop': { plugins: { gridexporter: true, grideditable: true }}
}}
Best Practices for Building React 16 Apps with Sencha Grid
Here are the best practices for building React 16 apps with Sencha Grid:
Component Organization
React’s component-based architecture encourages dividing your app into smaller parts. For Sencha Grid, avoid embedding it within large components. Isolate the grid in its own component. This makes it easier to manage and reuse. Separating concerns improves maintainability.
Efficient State Management
The state is critical for managing data in React components. Use useState and useEffect to handle state in functional components. For large datasets, avoid putting too much data into the local state. Consider using state management tools like Redux. This is helpful when many components need to share or manipulate the same data.
Avoid Excessive Re-Rendering
React’s virtual DOM reduces unnecessary updates, but re-renders can still slow performance. Use React.memo or useMemo to limit the re-rendering of components. For Sencha Grid, ensure that the data and configurations passed to it remain stable. Unnecessary changes can trigger slowdowns.
Lazy Loading and Code Splitting
React 16 supports lazy loading and code splitting. Use React.lazy and Suspense to load the Sencha Grid only when needed. This prevents loading the grid at the initial render, speeding up the first page load and optimizing your application’s overall performance.
Error Handling with Error Boundaries
React 16 introduced error boundaries. These catch errors in components without crashing the app. Wrap the Sencha Grid with an error boundary. This ensures that even if the grid encounters a problem, the rest of the app will continue functioning.
Conclusion
With ReExt, you can easily add a spreadsheet-like interface to your React 16 web applications. ReExt supports a variety of components, including grids, pivot grids, charts, and D3 visualizations. You can create an optimized package with only the components you need, ensuring a great user experience across both desktop and mobile platforms.
FAQs
What Are the Differences Between Ext JS and React JS?
Ext JS offers built-in components and complex features; React JS is lightweight and focuses on flexibility.
Which Framework Is Best for Frontend in 2024?
Ext JS is preferred for frontend development in 2024 due to its flexibility, scalability, and community support.
What is the Role of Grid in Creating Web Applications?
Grids organize and display data efficiently, enabling complex layout designs in web applications.
How Do You Choose the Right Framework for Your Applications’ Grid?
Consider your application’s complexity, performance requirements, and customization needs when selecting a framework.
Sign Up at Ext JS today to build amazing data-intensive applications for your businesses.
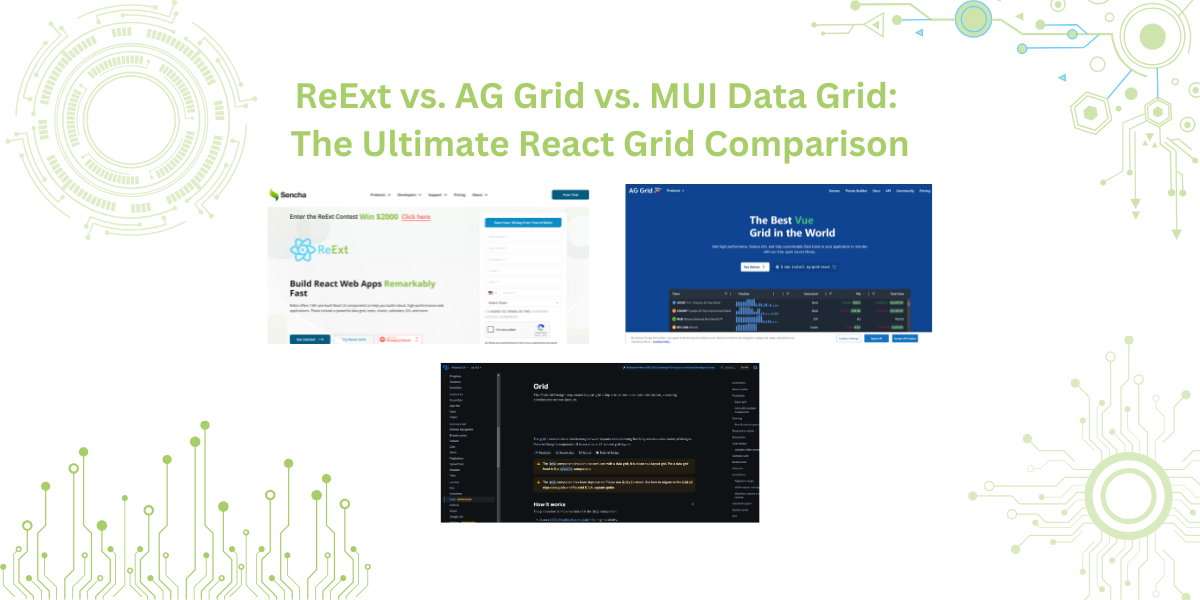
From financial dashboards to e-commerce platforms, your modern web applications rely on data grids. Integrating…
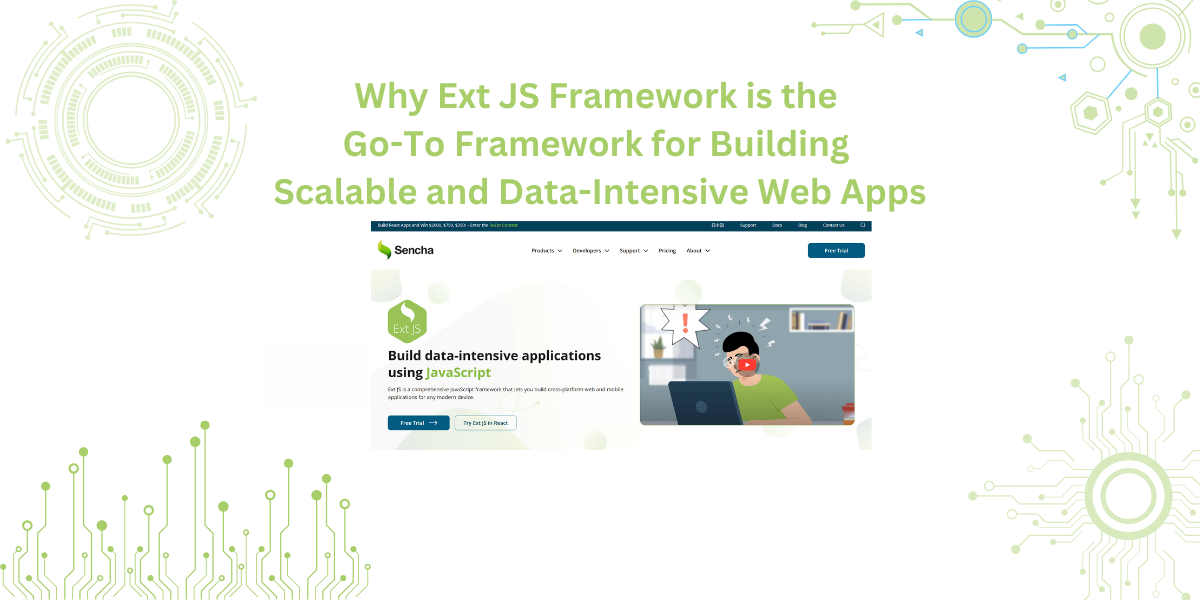
If you notice, you will see that modern web development apps now manage more data…
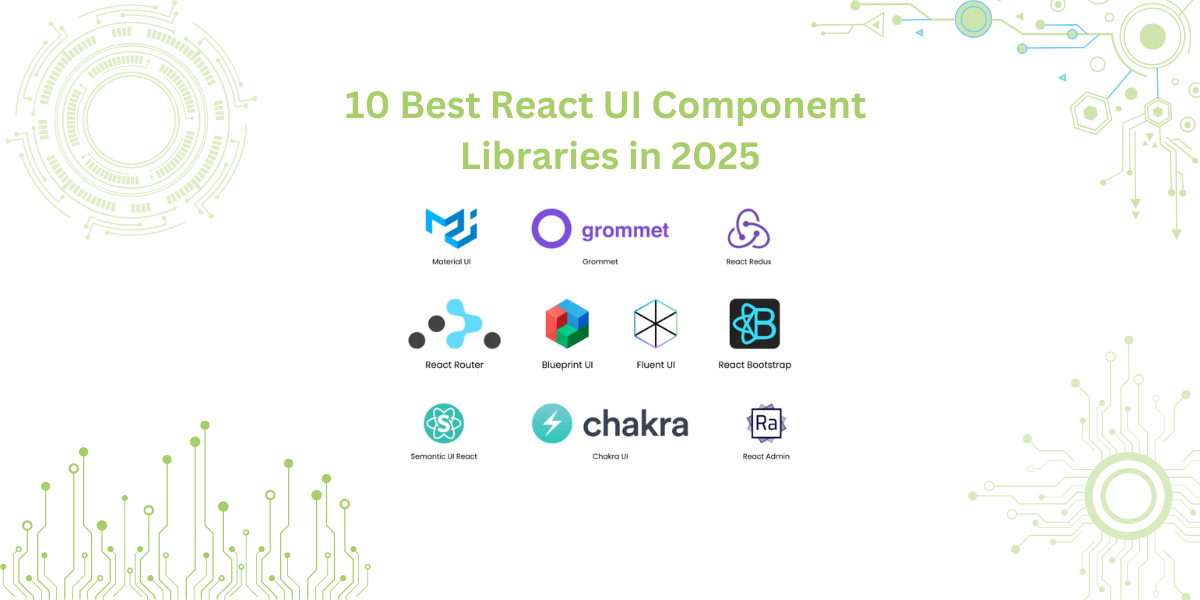
React continues to be the most popular front-end framework in 2025. Developers choose UI component…