React Data Grid – An Ultimate Guide for Beginners in 2024
Applications that leverage data account for over 70% of the business. The key to these applications’ performance is handling and presenting large amounts of information. In React applications, one of the best ways to manage vast datasets is with the first React Data Grid. A React data grid is essential for building modular, adaptive, and efficient user interfaces.
This blog will teach you the specifics of using a React Data Grid. First, we will explain the importance of data grids for dynamic applications and managing rows data efficiently. Then, you will learn how to install and configure a React Data Grid in six simple steps. After that, we will show how to modify grids using props, handle row object configurations, and add features like sparklines and left frozen columns.
You will also learn how to handle constantly updating data sources, including inserting a new rows array and managing both the updated rows. Additionally, we will demonstrate how to manage the header row and ensure smooth integration with functionality. Finally, we will share tips for styling grids, such as infinite scrolling and advanced features like column editors, ensuring you know how to optimize each column object. Let’s get started.
How To Install React Data Grid?
You can install the React Data Grid component from the data grid npm by running the following command in the root directory of your project.
npm install --save react-data-grid
Importing React Data Grid
The following import statement allows you to import the React Data Grid easily.
import ReactDataGrid from 'react-data-grid';
Minimum Configuration
The following code shows how to set the minimum configurations for the data grid you create. The ‘columns’ array describes the columns of the data grid.
<ReactDataGrid
columns={columns}
rowGetter={i => rows}
rowsCount={3} />
Props
The React Data Grid can be configured by passing various Prop values to the component, as shown below. These Props allow you to customize the grid the way you want. While there are many available props, the following three props are essential to render the data grid.
const columns = [
{ key: "id", name: "ID" },
{ key: "name", name: "Name" },
{ key: "amount", name: "Amount" }
];
Preparing Your Own Data Grid In React
The React community has developed many React Data Grid components to simplify the creation of sophisticated data grids. These components come packed with all the necessary functionality. Thus, users can use them to create intuitive data grids in minutes.
The following code is a simple react data grid example.
import * as React from "react";
const TableContainer = ({ striped, children }) => (
<table className={striped ? "table-striped" : ""}>{children}</table>
);
const TableHeader = ({ children }) => <thead>{children}</thead>;
const TableBody = ({ children }) => <tbody>{children}</tbody>;
const TableRow = ({ children }) => <tr>{children}</tr>;
const TableCell = ({ children }) => <td>{children}</td>;
const MyTable = () => (
<TableContainer striped>
<TableHeader>
<TableRow>
<TableCell>ID</TableCell>
<TableCell>Name</TableCell>
<TableCell>Age</TableCell>
</TableRow>
</TableHeader>
Data Grid Controls Take Care Of Everything
The most critical benefit of using this data grid component in your React application is the simplified development process. Instead of coding from scratch to create the data grid, you can rely on built-in features. You can achieve this with just a few lines of code. The data grid controls will handle the rest of the work for you.
In addition to the broad range of features, Data Grid offers more support. It is constantly improving.
How A React Data Grid Works
Let’s understand the functionality of a Data Grid using the following code block.
import React from "react";
import ReactDataGrid from "react-data-grid";
import { Sparklines, SparklinesLine, SparklinesSpots } from "react-sparklines";
const Sparkline = ({ row }) => (
<Sparklines
data={[row.jan, row.feb, row.mar, row.apr, row.may, row.jun]}
margin={6}
height={40}
width={200}
>
<SparklinesLine
style={{ strokeWidth: 3, stroke: "#336aff", fill: "none" }}
/>
<SparklinesSpots
size={4}
style={{ stroke: "#336aff", strokeWidth: 3, fill: "white" }}
/>
</Sparklines>
);
const columns = [
{ key: "year", name: "Year" },
In the above code, we have defined the columns and rows as objects.
In some cases, you may need to source additional libraries and plugins from third parties to use graphs and charts in grid tables. Here, we have imported React Sparklines and used it to draw a sparkline. The data set is used to generate the sparkline. It was important to design the sparkline and sparklinespots by establishing the style property.
Real-Time Data Grid Creation
In real-time data scenarios, you may need Data Grids created with live data feeding. Sencha’s data grid component allows binding live data to grids for more effective development. Once the data is updated, the legend is optional.
Data Binding To The React Data Grid
The first step in creating a data source for the React Data Grid is to prepare an array of data items. In the following example, we created a JSON object with ten data items. This data is arranged in columns, including those defined in the previous step.
import * as React from 'react';
import * as ReactDOM from 'react-dom';
import { Grid, GridColumn as Column } from '@progress/kendo-react-grid';
import products from './products.json';
const App = () => {
const [data, setData] = React.useState(products);
return (
<>
<Grid data={data}>
<Column field="ProductID" title="ID" width="80px" filterable={false} />
<Column field="ProductName" title="Name" width="250px" />
<Column field="UnitsInStock" title="In stock" filter="numeric" width="100px" cell={InStockCell} />
<Column field="UnitPrice" title="Price" filter="numeric" width="150px" />
</Grid>
</>
);
};
Updating Data Randomly
The variables declared below will help track the state of a specific component.
const [data, setData] = React.useState(products);
const [pausedTimer, setPausedTimer] = React.useState(true);
const [buttonLabel, setButtonLabel] = React.useState('Start');
const changeIntervalRef = React.useRef(null);
The following code block demonstrates a grid table that updates the data at random intervals. Here, we randomly update the field called UnitsInStock with a random number between -4 and 4.
// Randomly selects a set of data items from our data and updates the UnitsInStock field
const randomizeData = (passedData) => {
let newData = passedData.slice();
for (
let i = Math.round(Math.random() * 10);
i < newData.length;
i += Math.round(Math.random() * 10)) {
updateStock(newData);
}
return newData;
};
// Randomly adds or removes 0-4 from UnitsInStock and changes the changeType from negative to positive.
const updateStock = (passedRow) => {
let oldUnitsInStock = passedRow.UnitsInStock;
let updatedUnitsInStock = updateStockValue();
updatedUnitsInStock < 0 ? (passedRow.changeType = 'negative') : (passedRow.changeType = 'positive');
passedRow.isChanged = true;
passedRow.UnitsInStock = oldUnitsInStock - updatedUnitsInStock;
};
const updateStockValue = () => {
return Math.floor(Math.random() 4) (Math.round(Math.random()) ? 1 : -1);
};
The purpose of the three functionalities defined in the above code is as below.
- randomizeData – accepts a collection of data and randomly selects values that need to be updated.
- updateStock – accepts the selected values from randomized data and calculates the amount to add or subtract from UnitsInStock. It also helps identify the updated fields by setting their isChanged property to true.
- updateStockValue – updateStock function uses this function to add or subtract a value between 0 – 4.
After defining the above functions, we have used the setInterval() and clearInterval() JS functions to randomly update data. We can use them inside two separate functions, as shown below.
// Kicks off when we click on the "Start" button and updates data randomly every second
const startDataChange = () => {
clearInterval(changeIntervalRef.current);
changeIntervalRef.current = setInterval(() => {
let newData = randomizeData(data);
setData(newData);
}, 1000);
};
// Pauses the data being updated
const pauseDataChange = () => {
clearInterval(changeIntervalRef.current);
};
The above startDataChange function will call the randomizeData function each second. It will update values in several rows randomly resulting in an increase or decrease in UnitsInStock.
Updating Cell Styles
Now we have a functional data grid with live data streaming and updated at random intervals. So let’s move on to see how to update the UI of the data grid to reflect the data changes.
In the previous example, we are updating the field “UnitsInStock” with a positive or negative value. So let’s see how to style cells to differentiate these two states, which are positive updates and negative updates.
We can use the green color (#bffdbc3) to highlight a positive update, while using the red color (#ffd1d1) to mark a negative update.
const InStockCell = (props) => {
const checkChange = props.dataItem.isChanged || false;
const field = props.field || '';
const value = props.dataItem;
if (checkChange === true) {
let changeType = props.dataItem.changeType;
let cellColors = {};
changeType === 'positive' ? ((cellColors.color = 'green'), (cellColors.backgroundColor = '#bfdbc3')) : ((cellColors.color = 'red'), (cellColors.backgroundColor = '#ffd1d1'));
return (
<td
style={{
color: cellColors.color,
background: cellColors.backgroundColor,
}}
colSpan={props.colSpan}
role={'gridcell'}
aria-colindex={props.ariaColumnIndex}
aria-selected={props.isSelected}
>
{value === null ? '' : props.dataItem.toString()}
</td>
);
} else { // Handles our initial rendering of the cells and can be used to restore cells that have not been updated in a while.
return (
<td
colSpan={props.colSpan}
role={'gridcell'}
aria-colindex={props.ariaColumnIndex}
aria-selected={props.isSelected}
>
{value === null ? '' : props.dataItem.toString()}
</td>
);
}
};
While React is a great front-end library, we recommend you to learn the differences of Extjs vs React vs Angular.
Why Choose Sencha For Your React Data Grid?
ReExt, from Sencha, is an easy way to add a Data Grid to your React code. The implementation is simple, involving a single component with five properties. X events make handling events like onClick easy, allowing you to track user activity smoothly.
Component reusability is a major benefit. ReExt lets you use existing Sencha Ext JS code and custom components in React. This preserves uniformity and speeds up development by reducing rework.
ReExt works directly within React without needing separation from D3.js-driven components. It functions ‘out of the box’ with no extra configuration to your React or Ext JS setup. You can just plug it in and start building your application.
ReExt supports future releases of React and Sencha Ext JS 7.x. It is available as an NPM package and works with build systems like Vite or create-react-app.
You can also use ReExt with other React components. This flexibility allows you to use Sencha’s themes and toolkits to enhance application performance and user experience.
Creating a React Responsive Grid by Using ReExt by Sencha
Check out this video to create a powerful grid by using Sencha ReExt.
https://www.youtube.com/watch?v=ZnVgOP3GDZQ
To get started, navigate to the Sencha website and click on the products tab. From there, you should choose ReExt:
Next, you should click on “Try React Grid.”
You will see the below screen where there are three types of Grids:
- Grid
- Treegrouped Grid
- Pivot Grid
This section gives you an idea of how the ReExt grid works. Next, you should navigate to the documentation:
In the documentation, you should navigate to the components section and then choose your desired grid:
Let’s suppose I choose the Pivot Grid. We will get the below documentation:
This documentation comes with a complete solution to implement the pivot grid within your applications.
Here is the code example of pivot grid:
{
xtype: 'pivotgrid',
matrix: {
leftAxis: [{
width: 80,
dataIndex: 'salesperson',
header: 'Salesperson'
}],
topAxis: [{
dataIndex: 'year',
header: 'Year',
direction: 'ASC'
}]
// ... more configs
}
}
It will give you results like the one given below:
You can learn more details by clicking here.
Conclusion
React Re Ext Grids address challenges faced when working with large data sets in React grid layout applications. They help developers design complex and interactive UIs with minimal coding. The grids are easy to install and offer numerous customization options, making them ideal for dynamic, data-driven applications.
Real-time data updates, infinite scrolling, and column editors are some of the features supported by React Data Grids. These grids also offer frozen columns, which are essential for keeping key data in view while scrolling through large datasets.
Features like the column scrollbar allow users to navigate through multiple columns with ease. A summary row type can be used to display aggregated data, providing clear insights at a glance. The column resize handle further enhances usability by enabling users to adjust column widths dynamically.
React Data Grids also offer flexibility through optional arrays, allowing developers to pass an array describing additional configuration options or other untouched rows that don’t need to be processed in real-time. Developers can choose to implement either a static or memoized component to optimize rendering and performance in their applications.
FAQs
How to Handle Large Data in React Grid?
Use pagination, infinite scrolling, and lazy loading for efficient data handling.
What Is the Best Datagrid Component for React?
React Data Grid and AG Grid are popular for their features and performance.
What Is the Use of Data Grid in React?
Data grids display, edit, and manage large datasets in React applications.
What Are the Advantages of a Data Grid?
Data grids offer sorting, filtering, and real-time data updates for better user experience.
What Is the Concept of Data Grid Control?
Data Grid Control manages large datasets with features like pagination and sorting.
Worried about the performance of the data grid as your data grows? Try ExtJS and understand the difference!
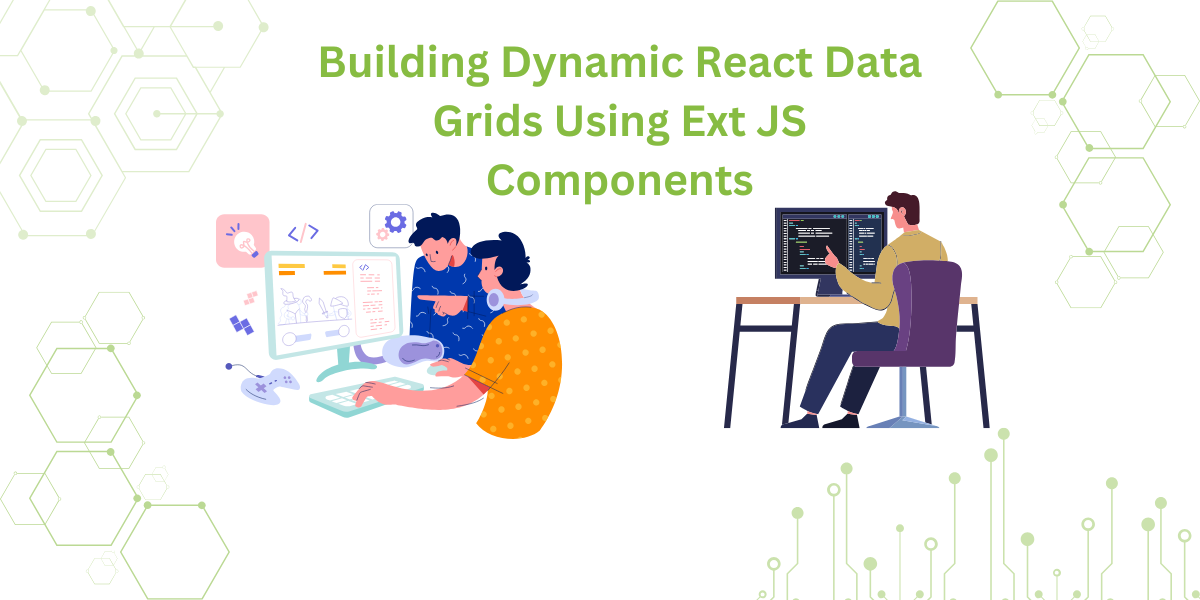
The latest industry reports show that around 60% of web applications rely on data grids.…
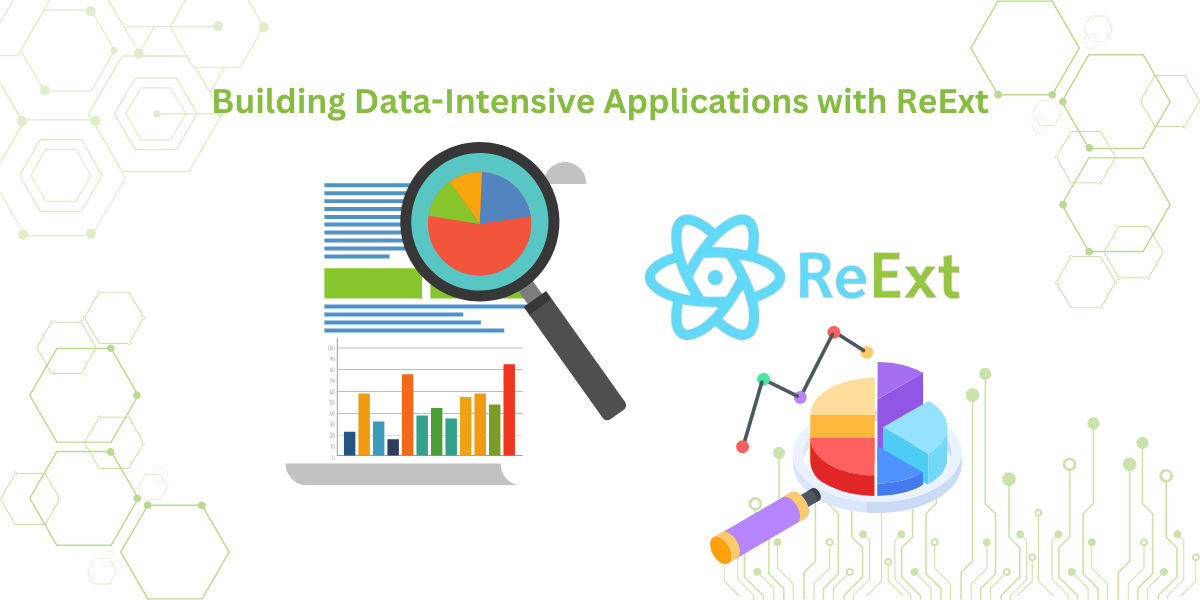
In the current age where data is wealth, almost 2.5 quintillion bytes of data are…
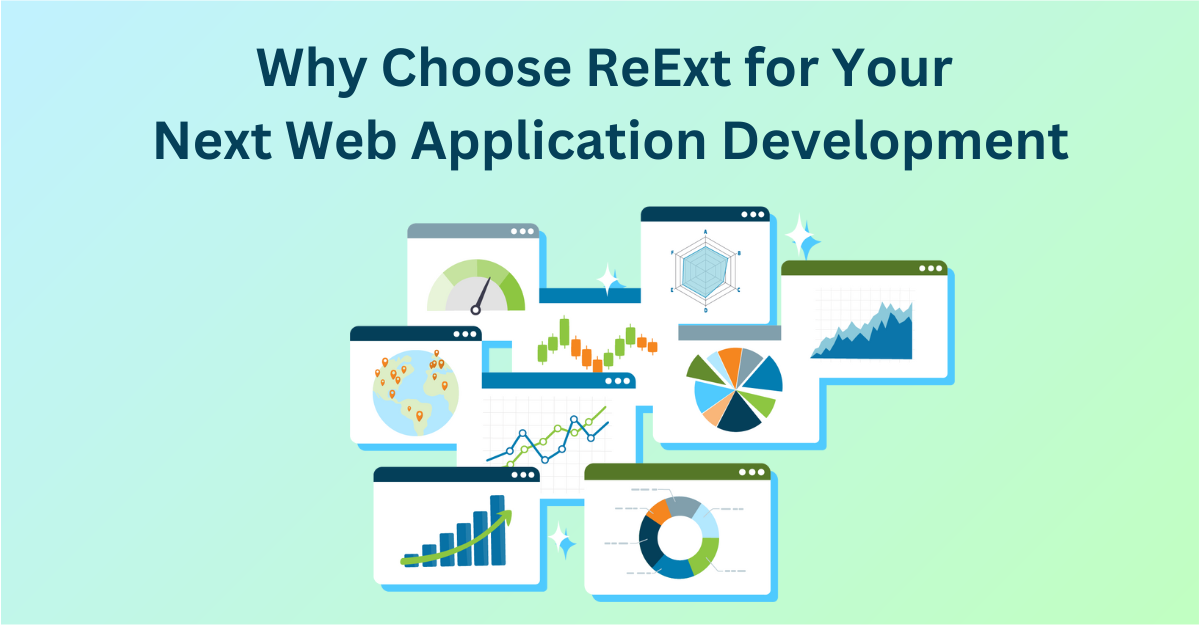
In the fast-paced world of web development, the demand for efficient, versatile tools is ever-increasing.…