Unlocking JS Frameworks Potential: 6 Hidden Features to Know
Nowadays, learning JavaScript has become an essential task for developers. At the same time, experts are learning new JavaScript technologies to excel in web development. In other words, we can say that JavaScript is the backbone of web development. For example, you can also see JS frameworks being popular for creating stunning applications. The right JavaScript framework can be a game-changer for your applications.
In this article, we will not mainly focus on a JavaScript framework. Rather, we will show you six hidden features to excel in JavaScript development. These features make your life easier if you develop applications regularly. Let’s continue reading till the end.
What Are the Six Hidden Features of JavaScript?
Here are the six hidden features of JavaScript
1. Destructuring Assignment: Unleashing the Power of Simplicity
Destructuring assignments is one of the most concise and powerful features. Besides, it allows developers to extract values from arrays or objects. After extracting values, it assigns them to variables in a single statement.
Code Example
// Array Destructuring
const [first, second, third] = [1, 2, 3];
// Object Destructuring
const { name, age } = { name: 'John Doe', age: 30 };
Best Practices
- Use destructuring to improve code readability by avoiding repetitive variable assignments.
- Combine array and object destructuring for complex data structures.
- Employ default values for handling undefined or missing properties.
2. The Spread Operator: Beyond Arrays and Objects
The spread operator (…) is a versatile tool in JavaScript. Besides, it enables the expansion of arrays, objects, or other iterable elements.
Code Example
// Array Spreading
const originalArray = [1, 2, 3];
const newArray = [...originalArray, 4, 5];
// Object Spreading
const originalObject = { a: 1, b: 2 };
const newObject = { ...originalObject, c: 3 };
Best Practices
- Use the spread operator for creating shallow copies of arrays and objects.
- Combine with functions like Math.max to find the maximum value in an array effortlessly.
- Employ spreading to merge arrays or objects concisely.
3. Memoization: Boosting Performance with Caching Techniques
Memoization is a performance optimization technique. Moreover, it involves caching the results of expensive function calls to avoid redundant computations.
Code Example
function memoize(func) {
const cache = {};
return function (...args) {
const key = JSON.stringify(args);
return cache[key] || (cache[key] = func(...args));
};
}
const memoizedAdd = memoize((a, b) => a + b);
Best Practices
- Apply memoization to functions with repetitive calculations.
- Be cautious with functions that have side effects, as memoization may yield unexpected results.
- Consider using libraries like Lodash for more advanced memoization techniques.
4. Proxies: A Gateway to Advanced JavaScript Manipulation
Proxies in JavaScript allow developers to intercept and customize fundamental operations on objects. As a result, they provide a way to implement advanced behaviors.
Code Example
const handler = {
get: function (target, prop, receiver) {
console.log(`Getting ${prop}`);
return Reflect.get(target, prop, receiver);
},
};
const proxyObj = new Proxy({ name: 'John Doe' }, handler);
console.log(proxyObj.name); // Outputs: Getting name, John Doe
Best Practices
- Use proxies for implementing features like:
- Object validation
- Logging
- Access control.
- Be mindful of performance implications, as proxies introduce an additional abstraction layer.
5. Optional Chaining: Simplifying Nested Property Access
Optional chaining is a concise syntax in JavaScript. Moreover, it allows developers to handle nested property access without explicitly checking each level for null or undefined.
Code Example
const user = {
address: {
city: 'New York',
},
};
const city = user?.address?.city;
Best Practices
- Employ optional chaining to gracefully handle nested property access without causing runtime errors.
- Combine optional chaining with nullish coalescing (??) for providing default values.
6. Web Workers: Harnessing Parallelism for Improved Performance
Web Workers enable parallelism in JavaScript. It happens by allowing the execution of scripts in the background, separate from the main thread. As a result, it enhances performance for computationally intensive tasks.
Code Example
// main.js
const worker = new Worker('worker.js');
worker.postMessage('Hello from main!');
// worker.js
onmessage = function (event) {
console.log(`Worker received message: ${event.data}`);
};
Best Practices
- Utilize Web Workers for tasks that can run independently in the background. These tasks can be data processing or image manipulation.
- Be cautious with data sharing between the main thread and workers to avoid synchronization issues.
Also Read: How to Create Professional Design with JavaScript Grid
What Are the Real-world Use Cases of the Above JS Frameworks Techniques?
Here are the real-world use cases for the above techniques:
- Simplify extracting relevant data from a complex API response through the destructing assignment.
- Combine default configurations with user-provided options readably through the spread operator.
- Improve the performance of recursive functions by caching previously calculated results through memoization.
- Implement a secure and auditable data model by intercepting property changes through proxies.
- Safely navigate and extract information from deeply nested data structures through option chaining.
- Enhance the responsiveness of an app by offloading intensive computations to a separate thread using web workers.
JS Frameworks: Conclusion
The above article discussed some hidden JavaScript features for JS frameworks. Developers can implement these tricks when working with JS frameworks. These features make it easy for developers to achieve quality and optimization. Moreover, we also shared some real-life use cases for each feature.
JS Frameworks: FAQs
What Are Hidden Features in JavaScript?
The hidden features in JavaScript are listed below:
- Destructuring Assignment
- The Spread Operator
- Memoization
- Proxies
- Optional Chaining
- Web Workers
What Is a Destructuring Assignment?
Destructuring assignment unpacks values from arrays or objects in JavaScript for concise variable assignment.
What Is Memoization and Its Role in JavaScript?
Memoization optimizes function execution by caching results. Furthermore, it enhances performance in JavaScript applications.
Why Do We Choose JS Frameworks for Building Applications?
JS frameworks streamline app development. For example, they offer structure, efficiency, and tools. Hence, it makes them preferred for building robust, scalable applications.
Sign Up for free at Ext JS today – Build scalable applications with effectiveness.
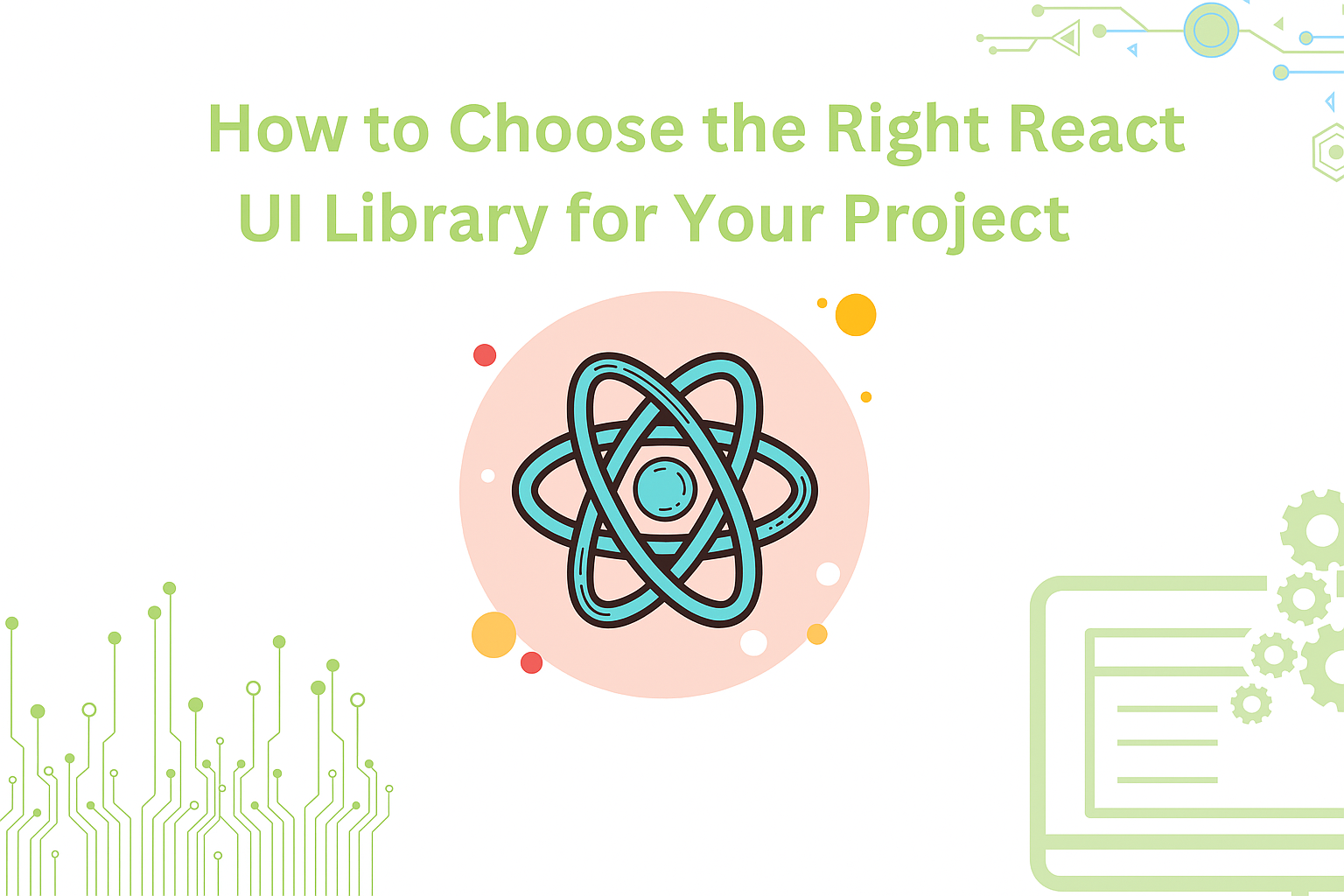
React is perhaps the most widely used web app-building framework right now. Many developers also…
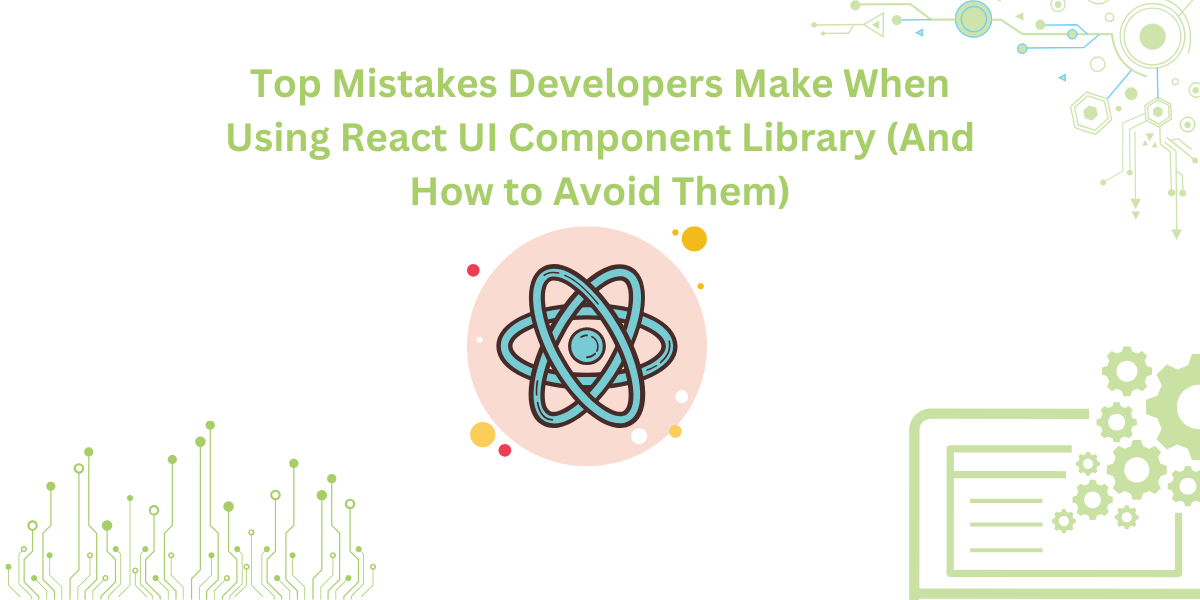
React’s everywhere. If you’ve built a web app lately, chances are you’ve already used it.…
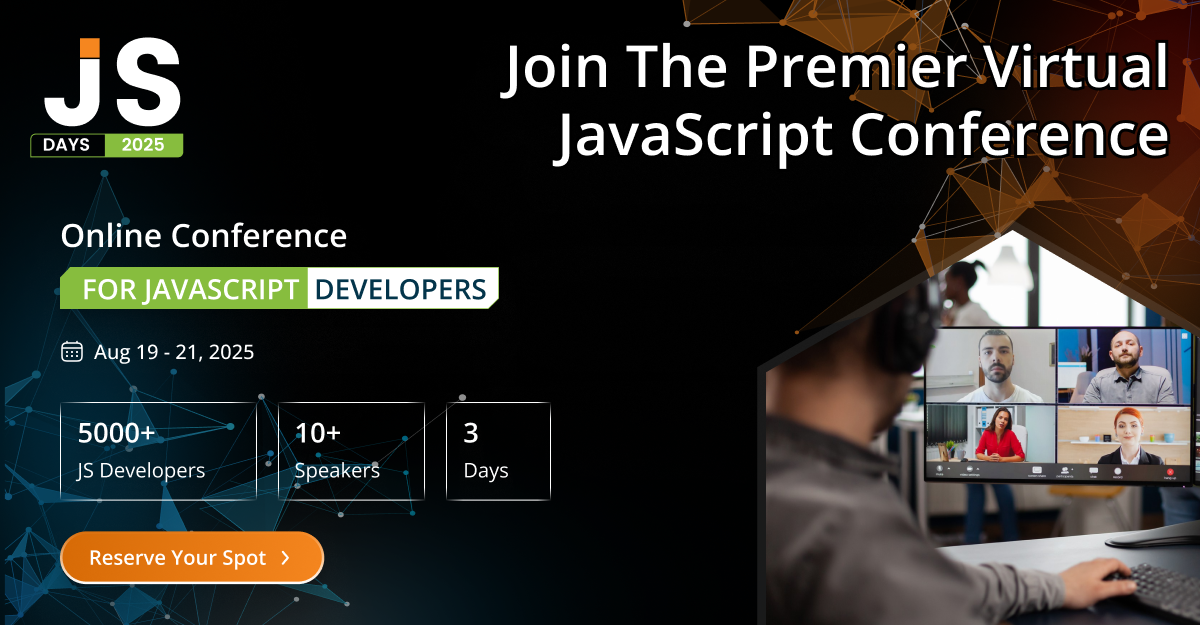
Join 5,000+ developers at the most anticipated virtual JavaScript event of the year — August…