Exploring Advanced JavaScript Topics in the 2025 Tutorial
JavaScript has moved from being a basic scripting language to a mandatory language for web development because of JS frameworks. These frameworks have changed how developers build websites by making the coding process easier and web applications more advanced. It’s important to keep learning the new features of JavaScript and how the new JavaScript framework works so that you can make websites that are up-to-date and run smoothly with the latest additions. This article will go through some advanced JavaScript topics in 2024.
Setting the Stage
Before moving into advanced JavaScript topics, in this section let’s look at some fundamental JavaScript concepts. The basic JavaScript concepts include variables and data types, operators, functions, and control structures such as if-else statements. These are the building blocks required for developing JavaScript web applications.
Now, let’s also explore ECMAScript features. Although ECMAScript is not a standalone language, it plays an important role by defining the syntax and semantics followed by JavaScript and other web scripting languages. This standardization is important as it guarantees consistency and compatibility across different web browsers and environments. Additionally, with each update to the ECMAScript standard, a range of new features are introduced. These include features like arrow functions, innovative data structures, and advanced programming methods like async/await. Therefore, these enhancements contribute to improvements in the capabilities and overall efficiency of JavaScript as well.
Advanced JavaScript Fundamentals
Advanced JavaScript concepts include closures, asynchronous programming with promises and async/await, as well as design patterns. They enhance code efficiency, scalability, and maintainability in complex web applications. Let’s look deeper into each of these topics.
Closures
Closures are when a function is defined within another function and it can access its outer function’s variables. Some practical applications of closures are it include maintaining the state between function calls and implementing callback functions for event handling. It also creates functions that generate specialized functions with shared environments and data. These applications make closures a valuable tool for solving a wide range of programming challenges.
Asynchronous programming
Moving onto asynchronous programming. It allows you to continue to execute JavaScript code without waiting for long-running tasks to finish. This overall prevents task blocking and improves responsiveness. A clean way to handle asynchronous code is by using Promises since it provides a structured way to handle both successful scenarios and errors.
Moving on from promises, let’s look into what async/await is. Async/await is a syntactical improvement over promises, and this makes asynchronous code more readable and easier to manage. When a function is marked as asynchronous using the ‘async’ keyword, it means that this function can perform asynchronous operations without blocking the rest of the code. Also, the ‘await’ keyword is used within an asynchronous function to pause its execution until a promise is resolved or fulfilled. This concept is commonly used in JS frameworks since it simplifies code and avoids complex nesting.
Design Patterns
Design patterns help in creating maintainable code by offering reusable solutions to address software design challenges. JavaScript uses several design patterns, including the Singleton pattern for guaranteeing a single instance of a class and the Factory pattern for object creation. It also has the Observer pattern for event and notification handling, and the Module pattern for code encapsulation. These design patterns overall enhance code maintainability by ensuring modularity, reusability, and scalability. They also facilitate testing and documentation by promoting modular and isolated components, simplifying the process of documenting and verifying individual functionalities.
Functional Programming in JavaScript
Functional programming in JavaScript is a method that focuses on creating programs using functions without changing data. In numerous JavaScript frameworks, functional programming adheres to principles, such as treating functions as values which means functions can be assigned to variables, passed as arguments to other functions, and returned from functions.
Similarly, higher-order functions are also a key principle of functional programming. These functions can accept other functions as arguments, return functions as results, or both. Common examples include map, filter, and reduce, they handle repetitive tasks such as going through elements and this makes the code cleaner, more functional, and easier to manage.
In functional programming, data remains immutable which means it’s never directly changed. Instead, you can create new data structures with the necessary changes. This approach offers several benefits within a JavaScript framework. Some of them are immutable data ensuring data consistency and this leads to more predictable and reliable code. It also reduces the risk of bugs, simplifies debugging, and supports thread safety by avoiding data modifications in the middle of a process. This makes concurrent and parallel programming more straightforward.
Reactive Programming with Ext JS
When creating web applications, reactive programming is a way of writing code that makes your application automatically update when something changes. Unlike JavaScript frameworks like React, Sencha Ext JS doesn’t strictly adhere to reactive programming but it uses patterns and features that enable similar concepts. In the next sections, we’ll further explore Ext JS.
Introduction to Ext JS programming concepts
Ext JS is a popular JavaScript framework for building web applications. Its key concepts are MVC architecture and a component-based structure which means it allows the creation of interactive user interfaces with reusable elements, such as a JavaScript grid. Additionally, Ext JS uses features like data binding, event-driven programming, and observables for handling asynchronous tasks.
Implementing reactive patterns using Ext JS
In Ext JS they allow you to implement reactive patterns within your application. For example, by using the data system and event-handling capabilities, you can receive automatic updates in response to data changes.
Similarly, the ‘Ext.util.Observable’ class enables your web components to emit and respond to signals which facilitates UI updates without manual intervention. Additionally, data binding simplifies synchronization by reflecting data model changes directly on the screen. When you link data stores to UI components, any data updates become immediately visible. This approach ensures app responsiveness without additional efforts and enhances the user experience thereby making your application dynamic and interactive with minimal coding.
Real-world examples of reactive programming in JavaScript applications
Some of the real-world applications that benefit from reactive patterns include those displaying live data updates, like real-time data dashboards. Similarly, form validations also use reactive checks to provide immediate feedback on entered data. Furthermore, these patterns enhance complex user interactions, such as dynamically enabling or disabling a submit button based on form validity. This reactivity makes sure that applications are responsive and customized to the user’s inputs.
Also Read: Recursion vs. Iteration: Choosing the Right Approach in Ext JS
Web Performance Optimization
The role of JavaScript framework in web application development emphasizes the need for optimizing performance to enhance speed, efficiency, and user experience. This section discusses strategies for analyzing and optimizing JS framework performance, including methods like minification, tree shaking, and code splitting. It also explores the practical use of browser developer tools for profiling and debugging.
Analyzing and optimizing JavaScript performance
To analyze and optimize JavaScript performance, you can start by using browser developer tools, specifically the performance tab in developer tools. This helps identify slow scripts and rendering issues. Also, speed can be improved by setting cache headers for static assets to reduce server requests. Similarly, you can also combine files to reduce HTTP requests and implement lazy loading for images and scripts that aren’t immediately required. Additionally, efficient code writing is also important since it minimizes browser reflows, repaints, and virtual DOM manipulations. Keep in mind, to re-evaluate the need for third-party scripts and load them asynchronously to prevent site slowdown.
Minification, tree shaking, and code-splitting techniques
Given above are some key techniques for optimizing the performance of JavaScript frameworks when developing user interfaces. Starting with minification it reduces the file size by removing unnecessary characters and thereby reduces the load time.
Similarly, tree shaking removes unused code which reduces bundle size and improves loading speed.
When it comes to automatic code splitting, it divides code into smaller parts, and the smaller code parts enhance the initial loading speed.
These techniques together enhance website performance by reducing the amount of code browsers must execute when building user interfaces.
Utilizing browser developer tools for profiling and debugging
Browser developer tools also play an important role in enhancing web performance in both new and existing projects. It helps in scenarios like profiling and debugging. Profiling identifies slow functions which slows down the performance, while debugging helps locate and correct errors. The performance tab in the developer tool shows a visual analysis of performance bottlenecks, while the console helps in troubleshooting. These tools simplify the process by enhancing the smoothness and speed of web applications by efficiently addressing inefficiencies and errors, thereby enhancing the user experience.
Server-Side JavaScript
While Ext JS mainly focuses on the client side in enterprise software development, its compatibility with various backend services enables integration into server-side JavaScript architecture. In server-side JavaScript with Ext JS, there are three primary approaches to consider.
Server-Side Rendering: This approach involves rendering Ext JS components on the server and this results in improved SEO, faster initial load times, and reduced client-side JavaScript execution.
Headless Ext JS: Headless Ext JS focuses on using data-binding and visualization capabilities on the server and this delivers pre-rendered HTML content to the client.
Serverless Functions: This approach uses platforms like AWS lambda or Azure functions to execute Ext JS code, and this provides scalability and cost-efficiency.
Each of these approaches has its own advantages and limitations and thereby it’s important to select the one that aligns best with the specific project requirements and constraints.
Modern Frontend Frameworks
In this section, let’s explore the latest updates in the best JavaScript framework.
Ext JS
The latest Ext JS provides enhanced data visualization through ExtReactCharts, improved accessibility, and a simplified API for component development. It promotes inclusivity and simplifies development for greater efficiency.
React
The latest update in the popular JavaScript library, React, introduces concurrent mode and suspense, it improves the performance by enabling smoother concurrent updates and data fetching. Streamlined server-side rendering with Next.js or Gatsby also provides SEO benefits and performance enhancements. Moreover, The Hooks API simplifies state management and side effects and this results in cleaner and more concise code.
Angular
The latest update in the popular JavaScript framework Angular introduces Angular Material, which provides advanced UI components for building interactive experiences. Similarly, the Angular Ivy renderer enhances performance and reduces application size as well. Additionally, Angular Universal enables efficient server-side rendering and this also results in improved SEO and faster initial loading times.
Vue.js
In Vue’s latest version, the composition API helps organize and reuse code, which is great for larger projects. Vue 3 makes applications faster and smaller. Additionally, it also supports server-side rendering with Nuxt.js, which is important for SEO and performance.
In order to build high-performing applications with frontend frameworks, follow key principles like component-based architecture, lazy loading, and code splitting. Also keep in mind to implement performance optimization techniques such as profiling, asset optimization, efficient DOM manipulation, and data handling to increase speed and efficiency. Moreover, make sure to implement state management and routing in frontend frameworks to effectively manage application states for consistency and reactivity.
Testing and Debugging Strategies
Today, various JavaScript testing frameworks are available to support diverse testing scenarios, ranging from unit tests to end-to-end tests. Notably, Jest and Mocha are experts in unit testing. Jest is favored for its user-friendly nature, while Mocha offers flexibility.
Karma is a good choice for browser-based tests that don’t involve a user interface.
Additionally, advanced options for e2e testing are offered by Cypress, Puppeteer, and Playwright. Also to ensure comprehensive testing, consider using test runners and tools to monitor code coverage and seamlessly integrate tests into your CI/CD pipeline for automation.
Moreover similar to writing tests you should also learn how to debug effectively so that you can figure out bugs both easily and faster. You can use browser developer tools to check variables and network requests and also add console.log statements to monitor values and flow. You can also set breakpoints to pause your code at critical spots and check if the correct values are passed. Debugging skills are quite important for maintaining and optimizing your JavaScript code effectively.
Nowadays, developers are using Test-Driven Development as a means to improve code quality, gain a deeper understanding of requirements, and catch bugs early in the development process. This method involves starting with a failing test, then writing code to make the test pass, and finally refining the code while making sure the test remains successful. TDD not only ensures thorough testing but also promotes maintainability and clarity in projects, although it requires effort and may not be suitable for every project. Community support can also be beneficial for developers who work with TDD to share insights and best practices.
Future Trends in JavaScript
JavaScript continues to evolve with the introduction of new features and concepts. Some noteworthy trends and proposals include private fields and methods, that enhance data privacy within classes. Also, the addition of top-level await simplifies asynchronous handling, while record syntax offers cleaner data models. Similarly, decorators provide advanced customization options, and logical assignment operators contribute to neater conditionals. All the above features represent ECMAScript proposals and additions aimed at improving the language’s functionality.
In addition to these, developers are also exploring the potential of WebAssembly to further enhance JavaScript’s capabilities. WebAssembly is a low-level language compiled into efficient machine code and empowers the creation of high-performance applications. It extends development possibilities by enabling the integration of various languages with JavaScript. This is important in areas such as game development, graphics rendering, machine learning, and cryptography since they are normally high-performance applications.
Furthermore, in web development, there are emerging frontend trends, which include progressive web apps, serverless functions, headless CMS, and Jamstack architecture. Another emerging trend is the expanding role of JavaScript in virtual and augmented reality. Conversely, backend trends include enhancements in Node.js, the utilization of serverless frameworks, GraphQL for efficient data handling, and the embrace of microservices architecture for scalability and maintainability.
Conclusion
This tutorial has covered a variety of JavaScript topics, from its evolution to the importance of staying updated with advanced concepts. It has also explored fundamental concepts, advanced JavaScript fundamentals, and functional programming principles. Additionally, this tutorial dives into reactive programming using Ext JS, web performance optimization, and future trends in JavaScript. However, since JavaScript is always changing, make sure to stay curious and experimental, as that will help you grow as a developer.
Supercharge Your JavaScript Skills with Sencha!
FAQs
What makes functional programming relevant in JavaScript development?
Functional programming in JavaScript encourages writing cleaner, more predictable code by treating functions as first-class citizens. This emphasizes immutability and statelessness, which leads to fewer side effects and easier debugging.
Why should I bother learning advanced JavaScript topics?
Learning advanced JavaScript topics enhances your ability to build sophisticated and efficient web applications using a web application framework. It enables you to solve complex problems more effectively and opens up opportunities for career advancement in web development.
Will this tutorial cover emerging trends and future features in JavaScript?
This tutorial focuses on current practices and established concepts. It also looks upon emerging trends and future features in JavaScript to give you some insights and prepare you for upcoming changes.
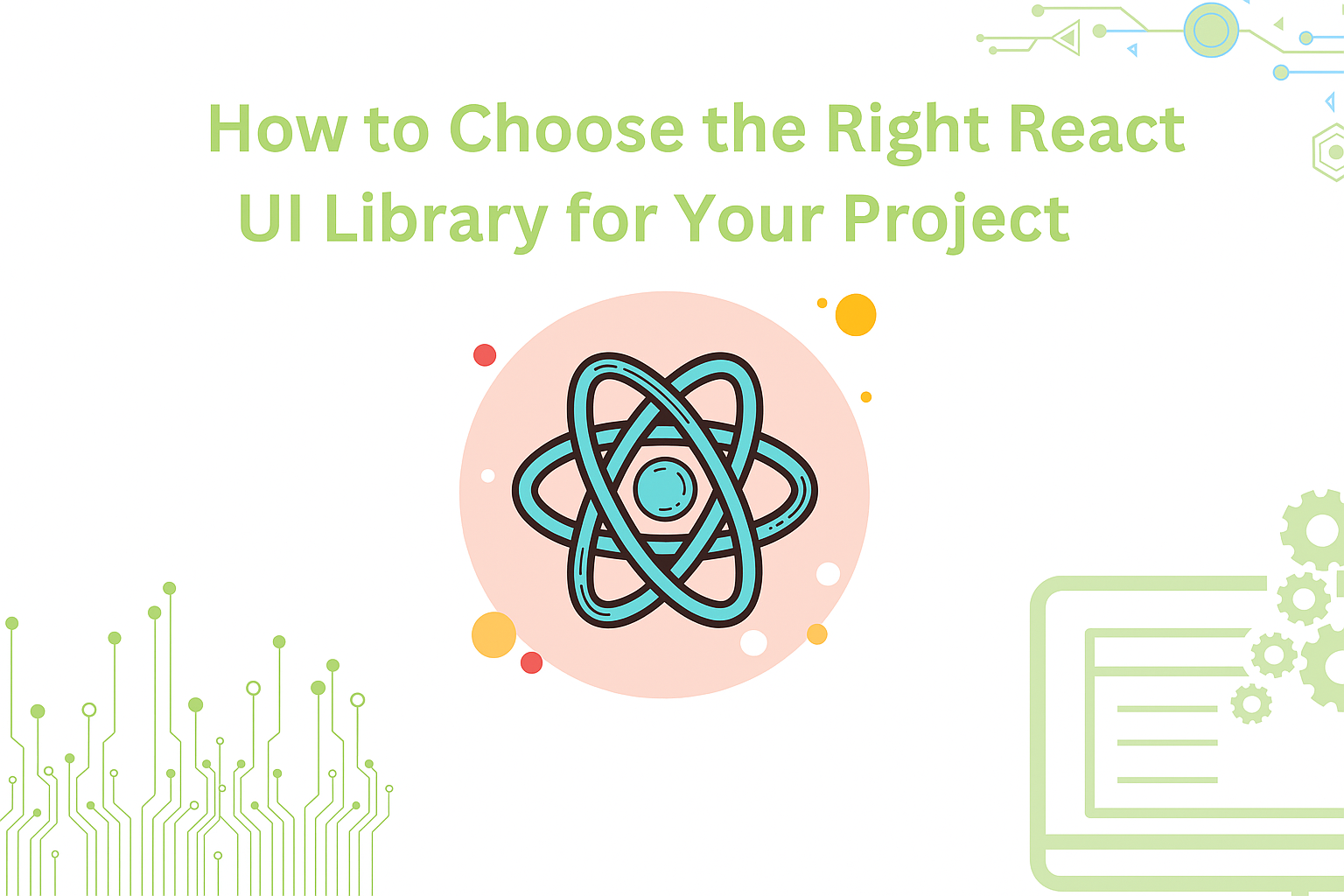
React is perhaps the most widely used web app-building framework right now. Many developers also…
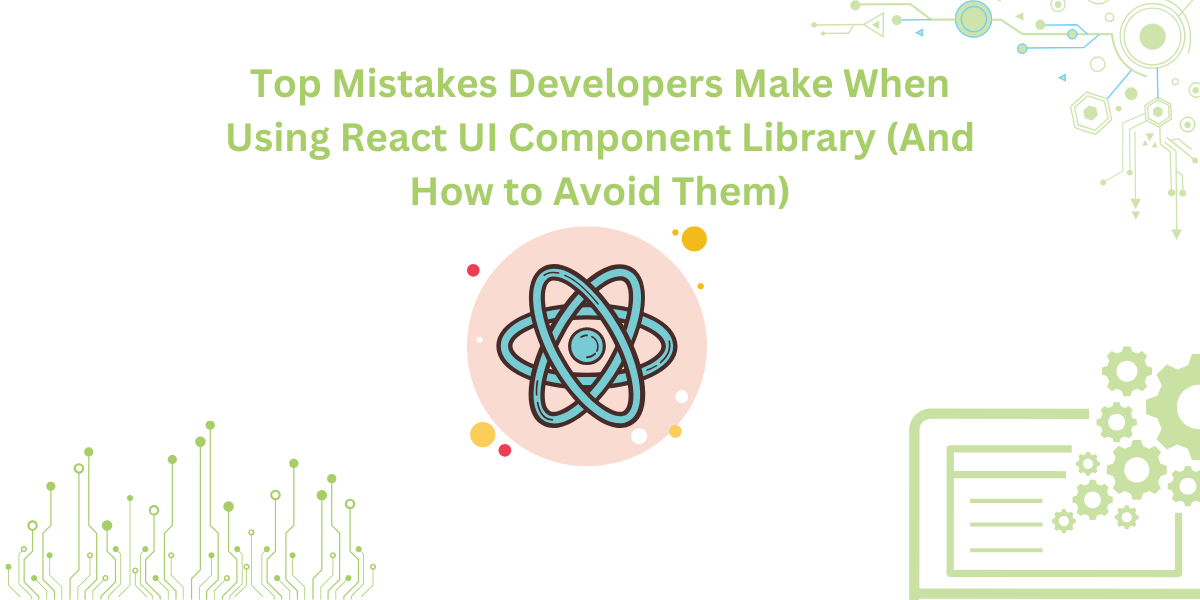
React’s everywhere. If you’ve built a web app lately, chances are you’ve already used it.…
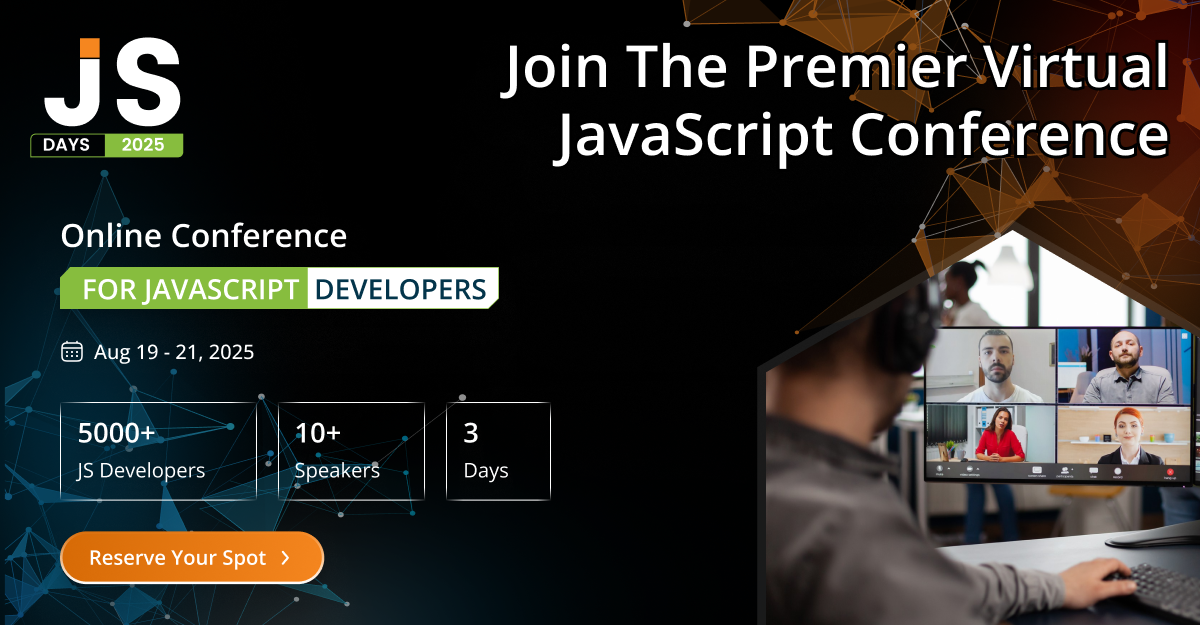
Join 5,000+ developers at the most anticipated virtual JavaScript event of the year — August…