How to Efficiently Add Elements to the Beginning of an Array in JavaScript
Object and array in JavaScript are fundamental structures for storing and managing data. Efficiently adding elements to the beginning of an array in JavaScript is important for optimizing code performance, especially within the context of a JavaScript framework. Adding to array JavaScript allows dynamic data storage with efficient operations. This blog looks into various techniques, including those offered by popular JS frameworks, and looks at their efficiency in achieving this task. Understanding effective methods for adding elements to the beginning of arrays is important, as the array’s initial position can significantly impact the overall program execution. Adding elements to array JavaScript is simple using methods like push() and unshift(). This knowledge helps improve code efficiency and performance.
Traditional Approach: Array.unshift()
The ‘Array.unshift()’ is a built-in method in JavaScript frameworks like Sencha Ext JS which is used to insert one or more elements at the beginning of an array. The method not only modifies the original array permanently but also returns the new length of the array as well. Thereby, this allows for an instant check of its size change without additional steps such as print statements. JavaScript size of array is determined using the length property to count elements. Given below is an example with the “Array.unshift” method in JavaScript code.
const fruits = ["apple", "banana"];
// Adding a single element
const newLength = fruits.unshift("orange");
console.log(fruits); // Output: ["orange", "apple", "banana"]
console.log(newLength); // Output: 3
// Adding multiple elements
fruits.unshift("mango", "kiwi");
console.log(fruits); // Output: ["mango", "kiwi", "orange", "apple", "banana"]
In a JavaScript framework some of the performance considerations and potential drawbacks of “Array.unshift()” are as follows.
- Adding elements to the beginning of an array with unshift() is usually slower than using push() for large JavaScript arrays. This is because unshift() needs to shift existing elements to the right to make room for new elements at the start which is a computationally costly method.
- The time of unshift() is O(n), where n is the number of elements in the array. This means that the execution time grows linearly with the size of the array.
- Also, if there’s a need for frequent additions to the beginning of a very large array, unshift() might not be the most suitable choice, and it might lead to slow performance which will affect the interactive user interfaces as well.
ES6 Spread Operator in JS Frameworks
The ES6 spread operator is an important feature in JavaScript that makes working with arrays and objects easier. It lets you quickly add elements to the start of an array. This operator works by spreading out or expanding elements of iterable objects like arrays and strings. It’s represented by three dots (…) followed by the object you want to expand. Adding an object to an array JavaScript helps manage complex data structures effectively. In the context of JavaScript frameworks, the spread operator is used for easily handling and manipulating data structures, turning iterables into separate elements in different use cases.
Here’s how to use the spread operator to efficiently add elements to the beginning of an array in the web development process.
const oldArray = [3, 5, 7];
const newElements = [1, 2];
// Efficiently combine new elements and old array using spread operator
const newArray = [...newElements, ...oldArray];
console.log(newArray); // Output: [1, 2, 3, 5, 7]
Let’s also look at a comparison of “Array.unshift()” and the spread operator.
Array.concat() Method
Moving on to Array.concat(), it is a built-in method in a JS framework. Array merge in JavaScript is possible through concat() or spread syntax for seamless combination. JavaScript merge two objects with Object.assign() or spread syntax to combine their properties. It makes a new array by combining elements from one array with zero or more other arrays or values. Yet, it does not change the original arrays. Moreover, the order of elements in the result follows the order of the provided arrays and values.
How to use Array.concat() to prepend elements in a JS Framework
In this example, concat() is used to create a new array (newArray) by combining elementsToPrepend with originalArray. As a result, the elements from elementsToPrepend appear at the beginning of the new array.
// Original array
let originalArray = [3, 4, 5];
// Elements to prepend
let elementsToPrepend = [1, 2];
// Using Array.concat() to prepend elements
let newArray = elementsToPrepend.concat(originalArray);
console.log(newArray); //Output is [1, 2, 3, 4, 5]
Also, keep in mind that concat() doesn’t change the original array since it returns a new array. If you want to update the original array, you need to reassign the result to it.
originalArray = elementsToPrepend.concat(originalArray);
console.log(originalArray);
Advantages and Disadvantages Compared to Other Methods in JS Frameworks
First, let’s look at some advantages.
- This handles merging many arrays or values into a single new array. Therefore this makes it versatile for combining data from different sources.
- It leaves the original arrays unchanged. This promotes immutability, unlike other methods such as direct array manipulation.
Moving on to the disadvantages.
- Every call to concat() creates a new array, which can be memory-intensive for very large datasets.
- Compared to the ES6 spread operator for prepending elements, concat() might have slightly lower performance for very large arrays due to the new array allocation.
Using Array.prototype.unshift() with the Rest Parameter
In some of the best JavaScript frameworks Array.prototype.unshift() works with the rest parameters from ES6. However, it’s not the best way in a JavaScript framework to add many elements to the start of an array. Let’s look at a code sample.
const numbers = [3, 5, 7];
const newElements = [1, 2, 4];
numbers.unshift(...newElements);
console.log(numbers); // Output: [1, 2, 4, 3, 5, 7]
Here the rest parameter (…) gathers the remaining arguments into an array. Then, it collects all elements from “newElements” and passes them as individual arguments to unshift(). Finally, unshift() inserts these elements at the beginning of the “numbers” array. The benefit of using the rest parameter to add multiple elements to the beginning of an array lies in its conciseness. The rest parameter can provide a more concise way to unpack an array and add its elements to unshift(). Apart from the benefits for large arrays, unshift() can be slow as it shifts existing elements, becoming more costly as the array grows. Some of the best practices that can be used are as follows.
- Use the spread operator (…) for simple prepending and create a new array for better readability.
- Consider alternatives like concat() or the spread operator. This is for better performance and readability. It’s especially helpful with large arrays and frequent insertions at the beginning.
Immutable Approach: Creating a New Array
In JavaScript, immutability means avoiding changes to existing data. When working with arrays, a best practice in a top JavaScript framework particularly in the context of two-way data binding is to use an immutable approach. This means creating a new array with the desired changes instead of changing the original one. Some of the advantages of the immutable approach, particularly in building user interfaces are as follows.
- Immutability leads to clearer code as modifications are explicit and don’t cause unexpected side effects.
- It simplifies debugging by making it easier to track changes and identify where data was modified.
- Immutability aligns well with functional programming principles, which often favor creating new data structures rather than mutating existing ones.
- In multi-threaded environments, immutability can help prevent race conditions where many threads might try to change the same data simultaneously.
Apart from the above-mentioned advantages, the immutable approach is highly recommended in most popular JavaScript frameworks with even component-based architecture for several reasons. First of all, when adopting a functional programming style, it naturally becomes a preferred choice. This is because functional programming emphasizes the use of pure functions, which do not change external states and produce predictable outcomes based solely on their input. Therefore, in enterprise software development, choosing immutability is important for managing the state predictably and maintaining code effectively in the JS library as well. Lastly, when dealing with shared data in a JavaScript library or web components, going for immutability ensures data integrity, preventing unintended modifications.
Performance Comparison and Best Practices
Best Practices: Choosing the Right Method
Some of the best practices to follow when choosing the most suitable method based on specific use cases and requirements are as follows.
- Prepending a small number of elements: Use unshift for optimal performance.
- Prepending a larger number of elements or concatenating: Opt for the spread operator (…) for a good balance of performance and readability.
- Concatenating many arrays: The spread operator excels in this scenario.
- Maintaining immutability: If you need to preserve the original array, use spread or slice to create a new array before modifications.
Considerations for Maintaining Code Readability and Scalability in JS Frameworks
When considering readability and scalability in array manipulation, comparing spread syntax, concat, and unshift methods is essential. Spread syntax excels in clarity for complex manipulations, while both spread and concat handle arrays of different lengths. Unshift may require adjustments when the number of elements to prepend is uncertain. Moreover, consider the micro-optimizations carefully by benchmarking various methods for your specific use case and array sizes. In complex array manipulations, focus on clear code by improving the readability enhancing spread operator. All in all, by understanding these factors, you can choose the most suitable method for your array manipulation needs.
Common Pitfalls and Mistakes
Some of the common mistakes to avoid when adding elements to the beginning of an array in the JavaScript frameworks are as follows.
- 1. Using push instead of unshift will add elements to the end, not the beginning.
- 2. If you need to preserve the original array, use spread (…) or slice to create a new array before prepending elements.
- 3. If you’re prepending to an empty array, using unshift is perfectly fine. However, some methods like spread ([…emptyArray, element]) might need extra checks to avoid unexpected behavior.
- 4. While unshift is fast for small numbers, prepending a large number of elements can be inefficient. Therefore, consider using a loop and building a new array if performance is critical.
As mentioned, to avoid performance bottlenecks in array manipulation, don’t use unshift for a larger number of elements. Instead, go for a loop and create a new array, which can be more efficient. Additionally, cut unnecessary array creation by avoiding methods like spread that introduce overhead.
Tips for Debugging and Optimizing Array Manipulation Code within JavaScript Frameworks
Also, when it comes to debugging, consider immutability. The virtual DOM introduces strategies for reducing re-renders and improving web app performance. Adding element to array JavaScript DOM manipulates the array dynamically based on user interaction. JavaScript adding array elements to dropdown list enables dynamic user interface updates. Similarly, let’s also look at some tips for debugging and optimizing array manipulation code execution.
- Use browser developer tools or other profiling tools to identify performance bottlenecks. It can pinpoint which part of your code is taking the most time.
- Break down complex logic into smaller, more manageable functions. This makes it easier to identify the source of issues and reasons for performance implications.
- Write unit tests to ensure your code works as expected after making optimizations.
- Debugging may be hard due to modifying the original array’s side effects. Consider using spread or slice to make new arrays. This will make the code clearer and easier to understand.
Always remember in web applications that are using UI frameworks, the best approach depends on your specific needs.
Also Read: JS Frameworks: JavaScript for Device Characteristic Detection
Conclusion
To add elements to the start of a JavaScript array, you need to pick a method that gives good performance, and readability, and is ideal for the specific use cases. Developers should try different methods, weigh the pros and cons, and stay updated on array manipulation best practices in JavaScript. Balancing micro-optimizations with code clarity is crucial as well. Moreover, developers stay curious, refine techniques, and adapt to new techniques for optimal array handling in projects.
FAQs
Why is it important to efficiently add elements to the beginning of an array in JavaScript?
Efficiently adding elements to the beginning of an array in JavaScript is crucial for optimal performance and enhanced code readability, particularly within JS frameworks. This operation, often used in JS frameworks, contributes to a smoother user experience by ensuring quick and responsive interface updates. Moreover, within the context of JS frameworks, maintaining a consistent application state relies on the ability to adeptly manipulate array elements, showcasing the significance of this capability in dynamic web development.
What is the ES6 spread operator, and how can it be used to add elements to the beginning of an array?
The ES6 spread operator is a syntax in JavaScript and is used in JS frameworks. This allows elements of an iterable like an array or string to be expanded into individual elements. It can be used to add elements to the beginning of an array by placing the spread operator followed by the array to be expanded inside the square brackets of a new array. For example, let newArray = […newElements, …existingArray], will prepend newElements to existingArray, resulting in newArray containing the new elements at the beginning.
Are there any best practices for optimizing array manipulation code in JavaScript?
Yes, use built-in methods like ‘map’ and ‘filter’ for concise and performant array operations. Additionally, use modern ES6 features such as the spread operator and destructuring for efficient array manipulation in JS frameworks.
How To Store An Array In Javascript?
In JavaScript arrays need to be stored with square bracket notation. JavaScript add to array allows seamless insertion of new data items into arrays. The array items contains numerical data as shown in the statement let items = [1, 2, 3, 4]; Arrays maintain their contents in a definite order. Adding items to array JavaScript ensures flexible handling of data collection and management. Add element in list React using setState() to manage state and re-render components.
What Is ARRAY?
An array is a data structure. It stores multiple items sequentially. The array starts its count from zero at its first index. JavaScript an array of objects stores multiple data entries with varied attributes in one array. You can define an array JavaScript by using square brackets [] to create a list of elements.
How To Declare Array In Javascript?
Declare JavaScript arrays using brackets. The creation of arrays can be done through two methods where one uses square brackets like let colors = [“red”, “blue”] and the other uses the new Array() method.
How To Add To An Array?
Array adding element JavaScript is straightforward with the built-in push() method. The .push() method allows addition of items to arrays. The operation to add new items is available through arr.push(“item”) or arr.unshift().
How To Create A Array In Javascript?
Create arrays easily in JavaScript. Arrays in JavaScript can start with either allow let fruits = [“apple”, “banana”] or allow let fruits = new Array().
How To Concatenate Strings In Javascript?
Slides string declarations inside JavaScript code with the + operator. The creation of the fullName variable requires combining firstName and lastName via + operator or utilizing the .concat() method.
How To Add To An Array In Javascript?
JavaScript adding element to array can be achieved using the push() or unshift() methods. To insert items at the first position of a JavaScript array use .unshift(). Adding array elements in JavaScript is easy with methods like splice() and concat(). Adding all elements in an array JavaScript can be done using loops or array functions.
How To Force Submit A Form With Javascript Array Added?
Force-submit forms with arrays in JavaScript. Hide form inputs contain array data through the use of JavaScript. Then call .submit() method.
How To Prioritize Adding Elements To An Array First Javascript?
The correct method to insert new values at the beginning of an array is .unshift(). The unshift method adds new elements to the start of a JavaScript collection which operates with efficiency.
How To Declare An Array In JS?
The array creation in JS occurs through brackets with the initialization let nums = [1,2,3] but using constructor becomes an option through new Array(1,2,3).
Transform your web applications with Sencha – explore our platform today!
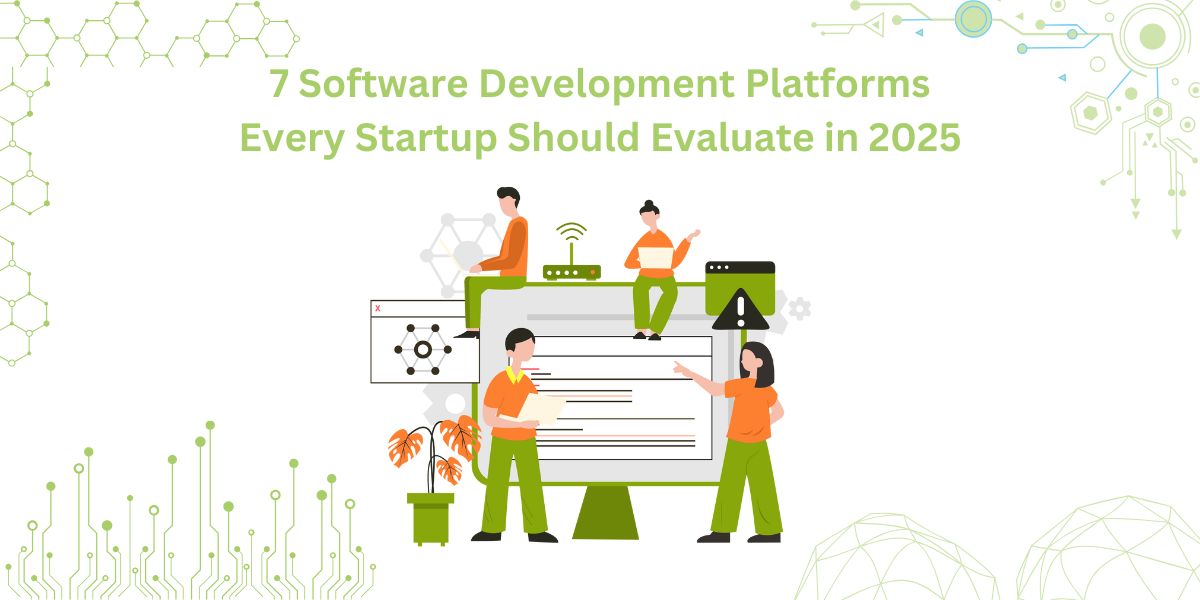
Did you know that nearly 90% of startups fail? And one of the biggest reasons…
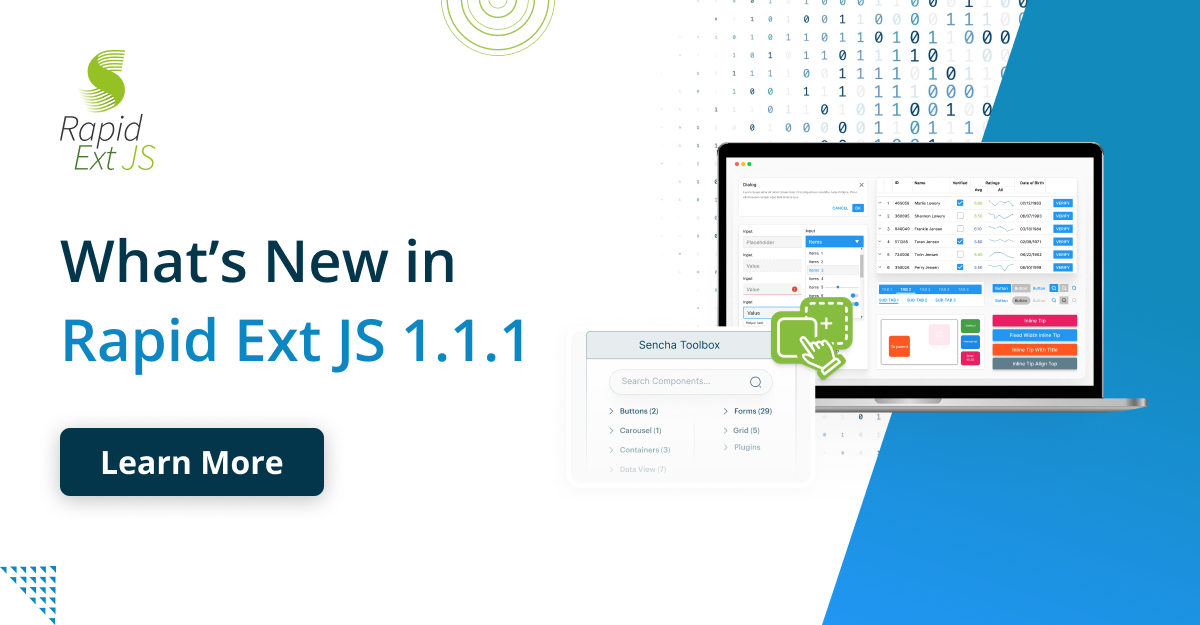
The Sencha team is excited to announce the latest Sencha Rapid Ext JS 1.1.1 release…
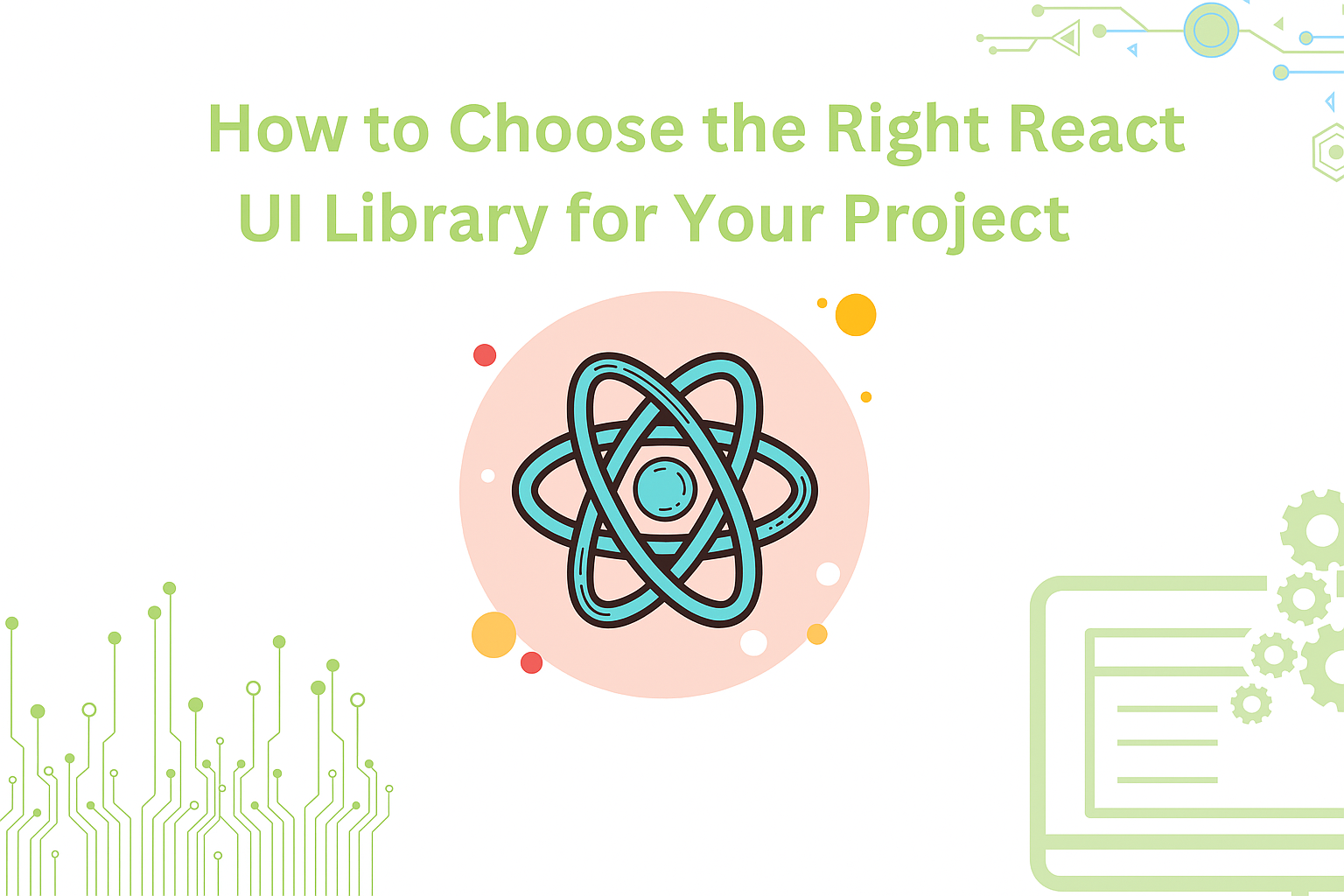
React is perhaps the most widely used web app-building framework right now. Many developers also…