How To Create A React Responsive Grid
A grid is a powerful method to display a large volume of information to the users within a website. It allows users to perform various tasks like sorting, filtering, and more. In this article, we will tell you how to build enterprise-ready React data grids. The React grid layout is the React library that enables the creation of powerful grids if you use React in your front end. However, robust and fully-responsive grids are critical requirements for many applications that process large amounts of information. You can have a better React responsive grid if you choose a modern enterprise-grade grid solution like Sencha GRUI, which has 100+ amazing data grid features. This article will describe how to create a React responsive grid and why you should use Sencha for better results.
How Can You Create A Grid In React?
First, let’s see how you can use React grid layout using a React data grid example. First, we will create a React app from scratch. To create a react app, you can use the ‘create-react-app’ command and create a sample application template.
- Run npx create–react–app my-grid-app
- Run cd my–grid–app
Next, you need to install the ‘react-grid-layout’ package, which contains the modern react grid components and functions you can use to create responsive grids. Following is the command to install the React grid.
- npm install react–grid–layout
Then we can start creating our React grid component. First, you must import the GridLayout Component from the ‘react-grid-layout’ package.
- import GridLayout from “react-grid-layout”;
How To Extend The React Component Class To Create The Grid?
After importing it, extend the React component class and create a component class to start using the GridLayout. You can use the ‘data grid’ property to set the width, height, x and y-axis, and minimum and maximum width of each child grid in the grid layout.
class MyGrid extends React.Component {
render() {
return (
<GridLayout className=“layout” cols={10} rowHeight={25} width={900}>
<div key=“one” data–grid={{ x: 0, y: 0, w: 1, h: 2, static: true }}>
1
</div>
<div key=“two” data–grid={{ x: 1, y: 0, w: 3, h: 2, minW: 2, maxW: 4 }}>
2
</div>
<div key=“three” data–grid={{ x: 4, y: 0, w: 1, h: 2 }}>
3
</div>
</GridLayout>
);
}
}
This will create a basic grid layout you can use to extend to add more functionalities to the UI. If you are unable to work with React grid view, this article might help you. Other libraries provide the same capability with more powerful features and efficiency that React gives to the grid layout. Sencha GRUI is one such intuitive grid that you can use to build more modern and responsive web applications.
How Can You Create A Grid In Sencha GRUI?
GRUI by Sencha, which has been created using Ext JS grid, consists of more than 100 grid features and enables users to create enterprise-grade grid solutions for React UI. This grid provides all the features of the React grid layout, but it is easier to use and performs better. Let’s see how you can create a React grid using a Sencha react grid example that uses the “sencha-grid” package.
First, create a react application using the create-react-app command as in the previous example.
- Run npx create–react–app my-grid-app
- Run cd my–grid–app
Next, you must install the sencha sencha-grid component package using the following command.
- Run npm add @sencha/sencha–grid
Then, after importing the React component from the beginning, you need to import the SenchaGrid and Column Components, the main components you can use to create the grid layout.
- import React from “react”;
- import { SenchaGrid, Column } from “@sencha/sencha-grid”;
How To Extend The React Component Using SenchaGrid?
After importing them, extend the React component class and create a default component class to start using the SenchaGrid. Note that you can use the components in a React app differently, but in our example, we will create a class-based component. Next, define some values to include in the grid. Use the data property of the SenchaGrid Component to load the data to the grid.
export default class App extends React.Component {
render() {
const data = [
{ col1: “val1”, col2: “data1”, col3: 1.01 },
{ col1: “val2”, col2: “data2”, col3: 1.02 },
{ col1: “val2”, col2: “data3”, col3: 1.03 },
];
return (
<SenchaGrid data={data}>
<Column field=“col1” text=“Column 1” flex=“1” />
<Column field=“col2” text=“Column 2” />
<Column field=“col3” text=“Column 3” align=“right” />
</SenchaGrid>
);
}
}
This code will create a grid with three columns containing data. As you can see, creating a React grid in Sencha is pretty straightforward, and it is easier to understand what you are designing.
What Is A React Responsive Grid?
However, the static grids like these above two examples are not enough to provide a complete grid experience to the user. We need to go beyond this basic grid layout and provide responsiveness to it. A responsive grid is a grid that responds to events like increasing and decreasing the number of columns, drag, and drop, and more. To make it possible, our code needs to listen to such changes in the grid and adjust the grid layout according to the event. Adding responsiveness is straightforward in react. You just have to use the “ResponsiveReactGridLayout” component and add the function that handles the grid.
Furthermore, you can also add “responsive breakpoints,” which are points where the layout will adjust to offer the user experience you want to provide. You can either provide the breakpoints, or set it to auto-generate the breakpoints. Let’s see how you can create responsive grids using the React ResponsiveReactGridLayout component.
How Can You Create A React Responsive Grid?
First, import the ResponsiveGridLayout from the react grid layout.
- import { Responsive as ResponsiveGridLayout } from “react-grid-layout”
After importing them, extend the react component class and create a component class to start using the ResponsiveGridLayout component.
class ResonsiveExample extends React.Component {
render() {
const layout = [
{ i: “ex1”, x: 0, y: 0, w: 1, h: 1 },
{ i: “ex2”, x: 1, y: 0, w: 1, h: 1 },
{ i: “ex3”, x: 2, y: 0, w: 1, h: 1 },
{ i: “ex4”, x: 3, y: 0, w: 1, h: 1 },
{ i: “ex5”, x: 4, y: 0, w: 1, h: 1 }];
return (
<ResponsiveGridLayout
className=“layout”
layouts={layouts}
breakpoints={{ lg: 1200, md: 996, sm: 768, xs: 480, xxs: 0 }}
cols={{ lg: 12, md: 10, sm: 6, xs: 4, xxs: 2 }}
>
<div key=“1”>1</div>
<div key=“2”>2</div>
<div key=“3”>3</div>
</ResponsiveGridLayout>
);
}
}
Use the ‘breakpoints’ property to define your customized breakpoints and the ‘cols’ property to the number of columns each breakpoint requires. Next, you must define the maximum number of columns in ‘lg’ and a minimum number of columns in xxs. Likewise, you can use this React component to build more responsive grids according to your preference. Also, you need to know exactly how to define the breakpoints and set the other properties to respond to the event.
Why Should You Use Sencha?
Responsive grids are critical components of modern web and mobile applications that allow users to do certain activities and change the layout according to the way they want. As discussed in this article, React provides the React grid layout package to build grids, but Sencha GRUI is a more powerful and efficient way you can create grids. To add responsiveness to the React grid, you need to use the ResponsiveGridLayout and define the properties accordingly. Instead, you can use Sencha GRUI to build more intuitive response grid layouts and offers grids with high performance. You can read a comparison between Sencha GRUI and React Grid here. If you have any questions, you can clarify them by contacting the Sencha team.
Leverage the power of Sencha GRUI for building high-performing React Grids!
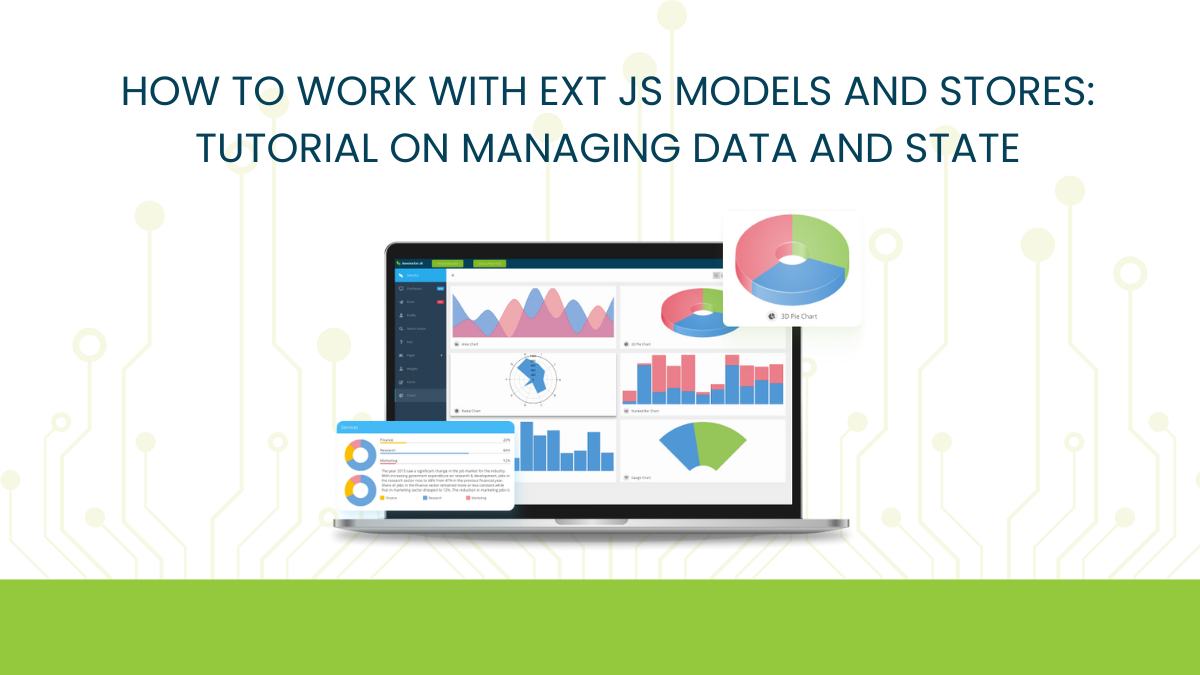
Ext JS is a popular JavaScript framework for creating robust enterprise-grade web and mobile applications.…
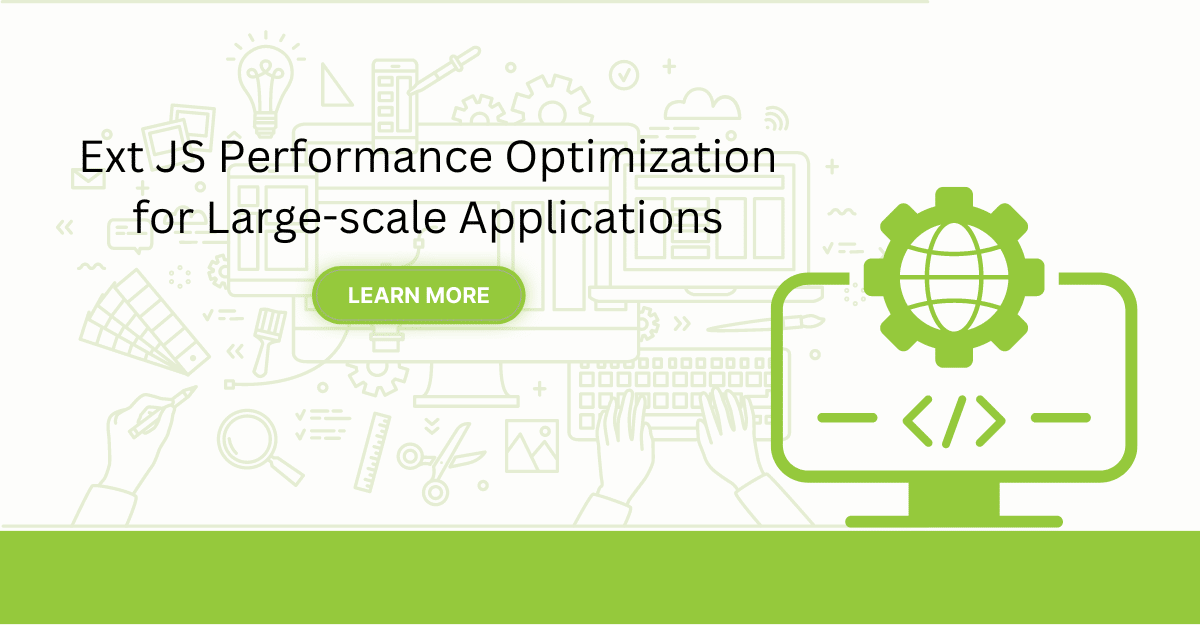
Are you facing issues with Ext JS applications’ performance as they scale up? Don’t worry!…
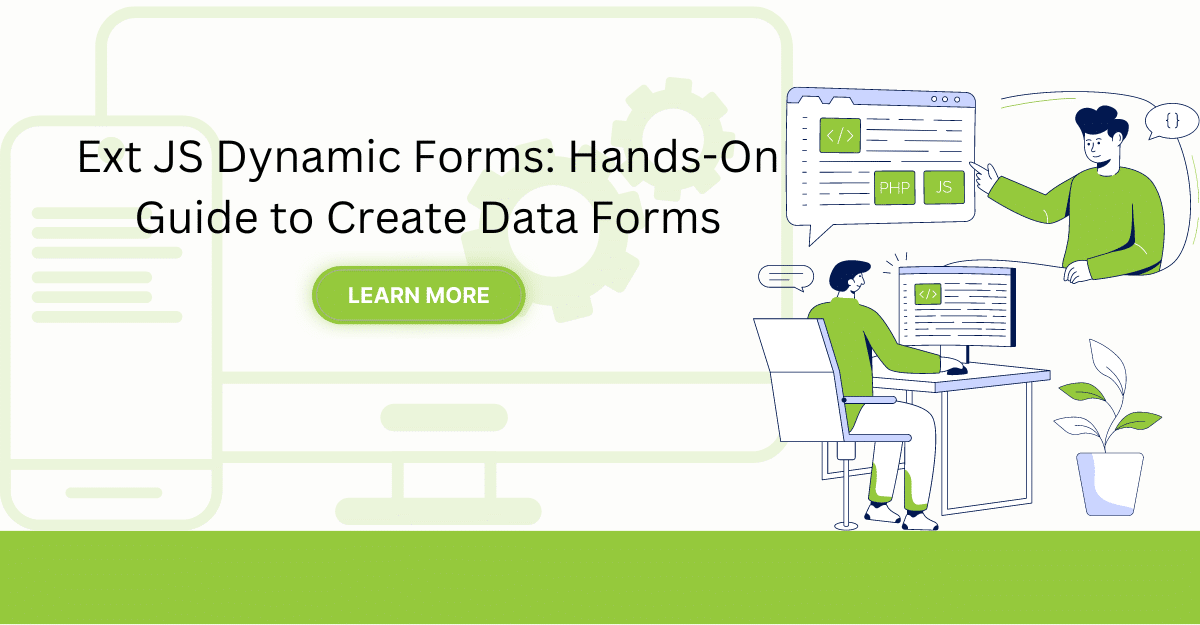
Dynamic forms are changing the online world these days. Ext JS can help you integrate…