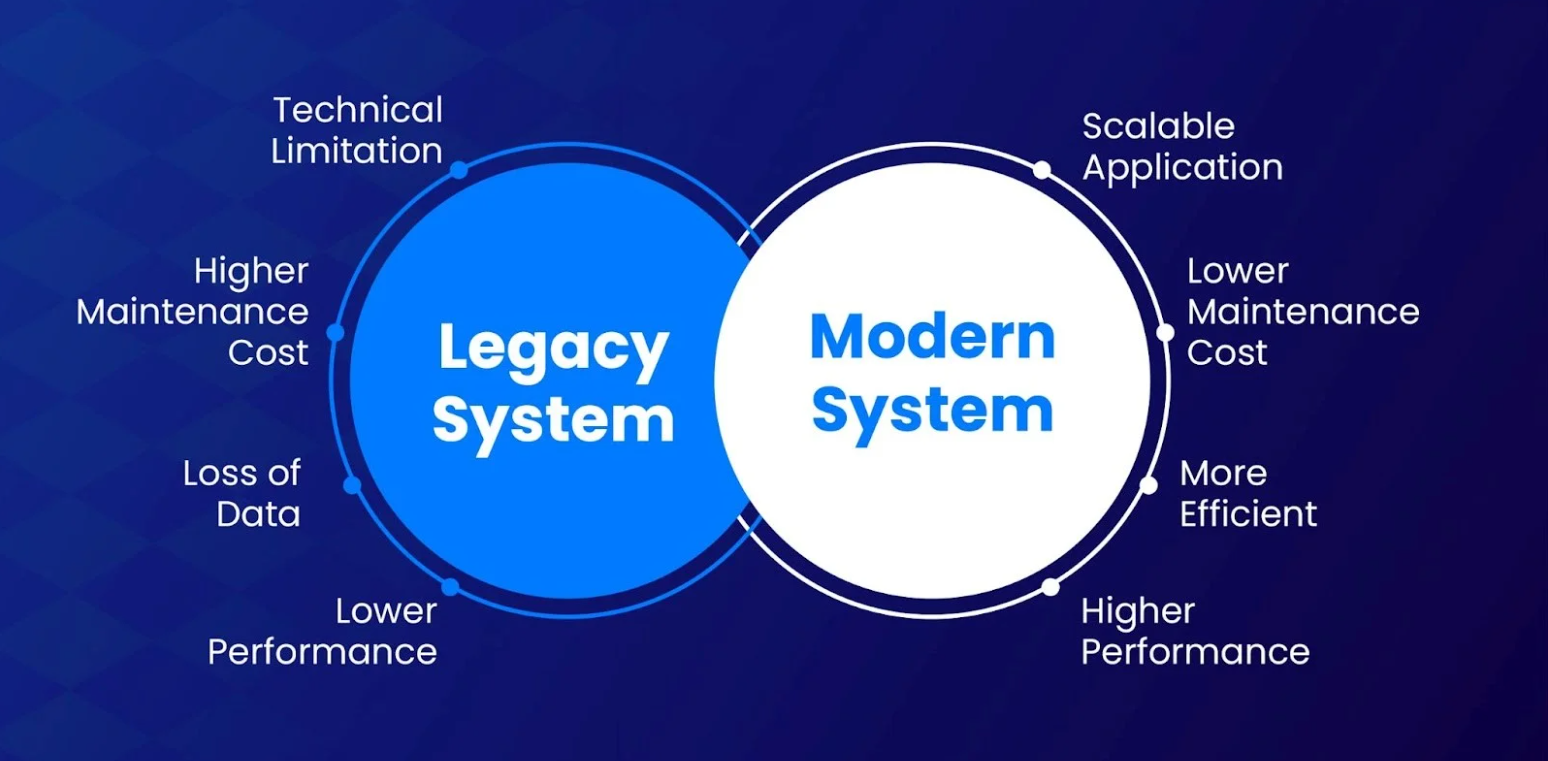
Is your team still clinging to legacy software that’s slow, hard to maintain, or frustrating for users? You’re not alone. Many organizations continue to rely on outdated applications that were once revolutionary but now hold back innovation. If that sounds…
Subscribe to our newsletter
Be the first to learn about new Sencha resources and tips.