5 Main Steps to DOM Manipulation Using JS Frameworks
If you are a web developer, you must have encountered multiple challenges when working on web applications. One of the most common challenges is dealing with JavaScript errors. Whether you are a beginner or a professional developer, it is important to tackle these issues for building stable and robust web applications. In this comprehensive guide, we will cover the basics of error handling in JavaScript. We will also tell you how JS frameworks help us achieve DOM manipulation. One popular JavaScript Framework is Ext JS.
Moreover, we will also explore the Document Object Model (DOM) and how to manipulate it using JavaScript. We will also explain the importance of the DOM in web development. You will also learn to implement it using JavaScript Framework Ext JS in your projects.
What Is the Document Object Model (DOM)?
Before you learn how to handle errors, it is essential to understand the true meaning of the Document Object Model (DOM). The Document Object Model represents the structure of a web page. It provides a way for JavaScript to interact with and modify the structure and content of a web document.
In other words, the Document Object Model acts as a bridge between the dynamic world of JavaScript and the static HTML or CSS.
What Is the Importance of DOM Manipulation in the Web Development Process?
Now that you have learned about the Document Object Model, it’s important to understand its significance in web development projects. DOM manipulation helps us create dynamic and interactive websites by modifying the content of web page elements.
Our websites need to perform Document Object Model manipulation to be responsive and stable. This leads to a poor user experience that modern users do not expect. As a result, it may significantly affect your user experience and may also lead to losing customers.
What Are the Steps to DOM Manipulation Using JavaScript for Web Development?
Here are some simple steps for DOM manipulation using JavaScript for web development.
Understanding the DOM
First, you must know that the Document Object Model structure gives a tree-like representation of your web page. When a browser processes CSS and HTML, it creates a DOM tree. The interesting part to note is that each element on our web page becomes a node in this tree. It can be buttons, paragraphs, headings, or anything else.
Understanding the Document Object Model structure is essential for effective manipulation. Without a solid grasp of the Document Object Model structure, putting effort into manipulation can be a waste of time.
Now, let’s explore the process.
Our browser parses the CSS and HTML files, and then it turns those files into a DOM tree. Understanding this process is essential to comprehend how the Document Object Model reflects our web page.
In the Document Object Model, everything is a node. There might be elements like <div>, <p>, <span>, etc. These are all nodes. If there is any text inside those elements, that text also acts as a node. You must understand the difference between these nodes, as it helps us achieve targeted manipulation.
Selecting DOM Elements
This step is the fundamental part of Document Object Model manipulation. It’s important to know that we have various methods for this purpose. Some examples are `getElementById`, `querySelector`, and more. Let’s look at some examples of selecting elements using these methods.
// Selecting an element by its ID
const elementById = document.getElementById('elementId');
// Selecting elements using querySelector
const elementsByQuery = document.querySelectorAll('.className');
When implementing these methods, remember to follow some best practices. For example, you can minimize the use of complex selectors. This can help improve maintainability and performance.
Manipulating DOM Elements
After you’ve selected the elements, this is the point where you can change their titles, attributes, and content using JavaScript. You can also modify, remove, or add elements. Let me show you some common manipulation techniques with code examples.
// Changing the content of an element
elementById.innerHTML = 'New Content';
// Modifying attributes
elementById.setAttribute('data-custom', 'new value');
// Adding a new element
const newElement = document.createElement('div');
newElement.textContent = 'Dynamic Element';
elementById.appendChild(newElement);
// Removing an element
elementById.removeChild(newElement);
Handling Events
When creating dynamic web applications, you must remember event handling. You can attach event listeners to DOM elements using JavaScript. It enables you to respond to user interactions. Common events include inputs, clicks, and much more. Let me show you an example.
// Attaching a click event listener
elementById.addEventListener('click', () => {
alert('Element clicked!');
});
// Handling input events
const inputElement = document.getElementById('inputField');
inputElement.addEventListener('input', (event) => {
console.log('Input changed:', event.target.value);
});
Updating the DOM Dynamically through JS Frameworks
One of the most important aspects is updating the Document Object Model dynamically. This is a common scenario in web development. Whether the website is based on data changes or user interactions, we need effective techniques for efficient data updates.
At this point, we are considering using JavaScript frameworks like React, Ext JS, Angular, and Vue.js. These frameworks help simplify the process and optimize our performance.
Ext JS – One of the Best JS Frameworks
This framework helps us create a user interface for our web applications and achieve cross-browser functionality for our websites. It’s important to note that this framework is built for desktop-based applications. They are commonly used for enterprise-level applications. The best part here is that they support all modern browsers, including IE6+, Firefox, Chrome, Safari 6+, Opera 12+, and more.
On the other hand, Sencha Touch is used for mobile applications. It’s important to note that Ext JS uses the MVC/MVVM architecture. Let’s explore Document Object Model manipulation through this framework.
To return an object of an element, use the below code:
<div id = 'divId' />
Here is how to get an element by ID in this framework:
Ext.define(appName.view.GridName , {
Id = 'gridId',
Other properties...
});
An example to change the value of an element:
<input type = 'text' id = 'studentName'>
Here is an example of selecting multiple elements at once:
<div id = 'div1' class ='divClass' />
<div id = 'div2' class ='divClass' />
Also Read: Comprehensive Guide to JavaScript Frameworks for Web Development
What Are the Real-World Examples That Leverage DOM Manipulation Using JS Frameworks?
Let’s explore some real-world examples and case studies that showcase powerful Document Object Model manipulation using JavaScript.
The first example is Facebook. It’s important to note that Facebook relies on Document Object Model manipulation for its real-time and dynamic News Feed and updates. They use virtual DOM manipulation in React, which helps enhance user experience and performance.
The second example is Google Maps. Google Maps employs complex Document Object Model manipulation to display route information, markers, and maps. This optimization ensures smooth rendering and provides an efficient user experience.
Finally, there’s Trello. This platform uses dynamic Document Object Model updates to manage user interactions with cards and boards. The smooth drag-and-drop functionality is made possible through Document Object Model manipulation. These examples illustrate the importance of effective DOM manipulation in creating dynamic and interactive web applications.
JS Frameworks: Conclusion
In this developer-focused guide, we’ve explored the basic steps to Document Object Model manipulation using JavaScript. It’s crucial to understand the Document Object Model structure, select elements, manipulate them, handle events, and update the Document Object Model dynamically. These are key skills for any developer looking to master these concepts.
Also, remember that using JavaScript frameworks can make Document Object Model manipulation easier. One such example is Ext JS, which we discussed in the article above.
JS Frameworks: FAQs
Why Is DOM Manipulation Important?
It helps us create interactive user interfaces for our website and applications.
How Can Event Handling Be Implemented in DOM Manipulation?
We can implement event handling in document object model manipulation through getElementById.
Can JS Frameworks Enhance DOM Manipulation?
Yes. They make it easier to manipulate DOM.
How Do You Select DOM Elements in JavaScript?
To select elements in DOM, we can use the following code:
<div id = 'div1' class ='divClass' />
<div id = 'div2' class ='divClass' />
Master Error Handling in JavaScript for Seamless, Stable Applications With Sencha Now!
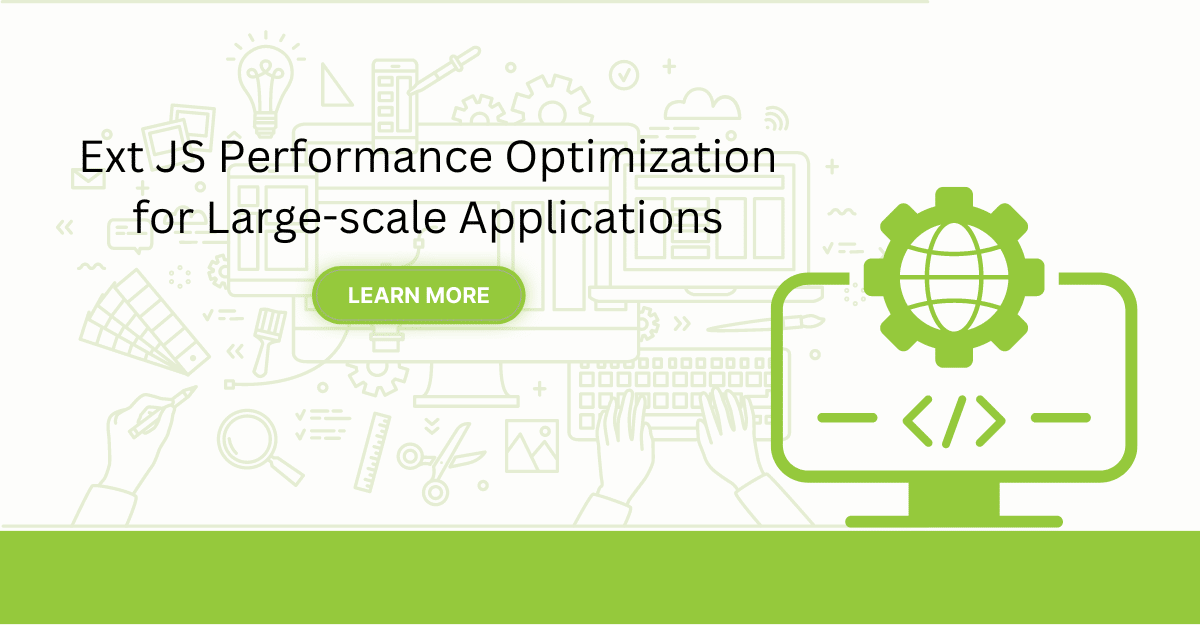
Are you facing issues with Ext JS applications’ performance as they scale up? Don’t worry!…
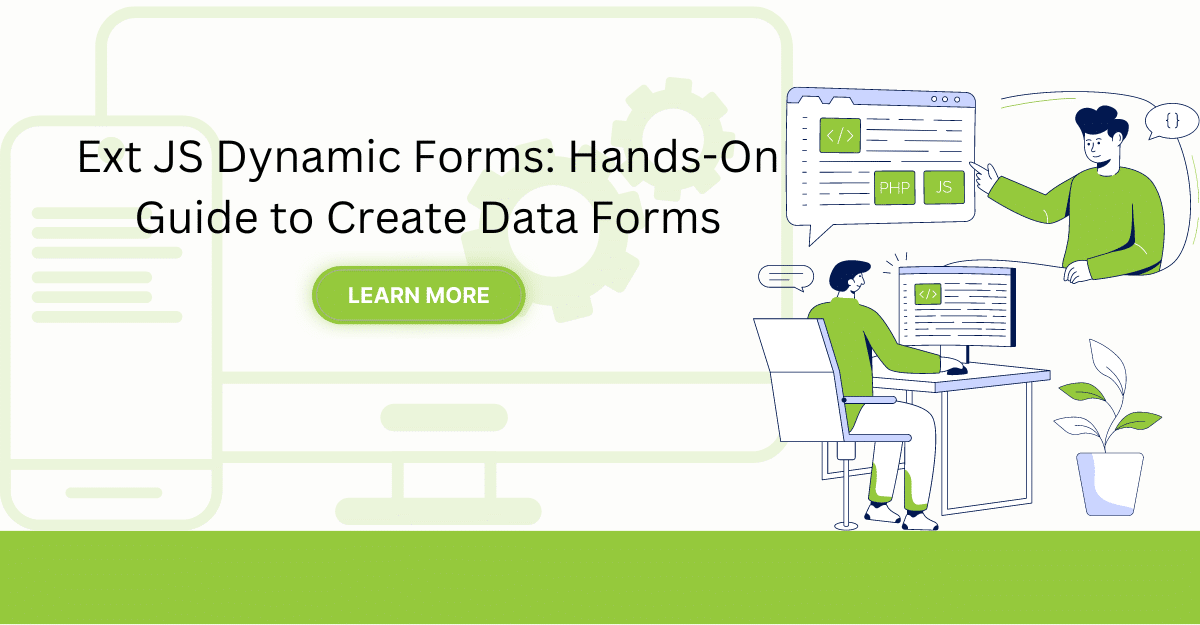
Dynamic forms are changing the online world these days. ExtJS can help you integrate such…
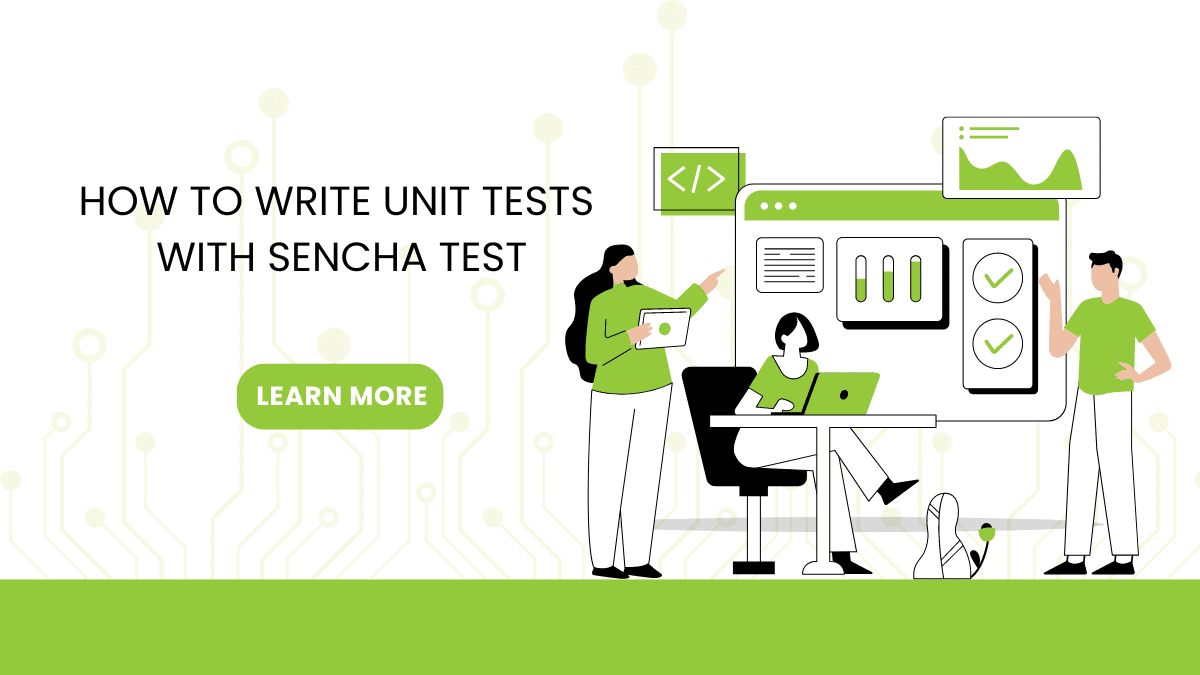
In modern software development, unit testing has become an essential practice to ensure the quality…