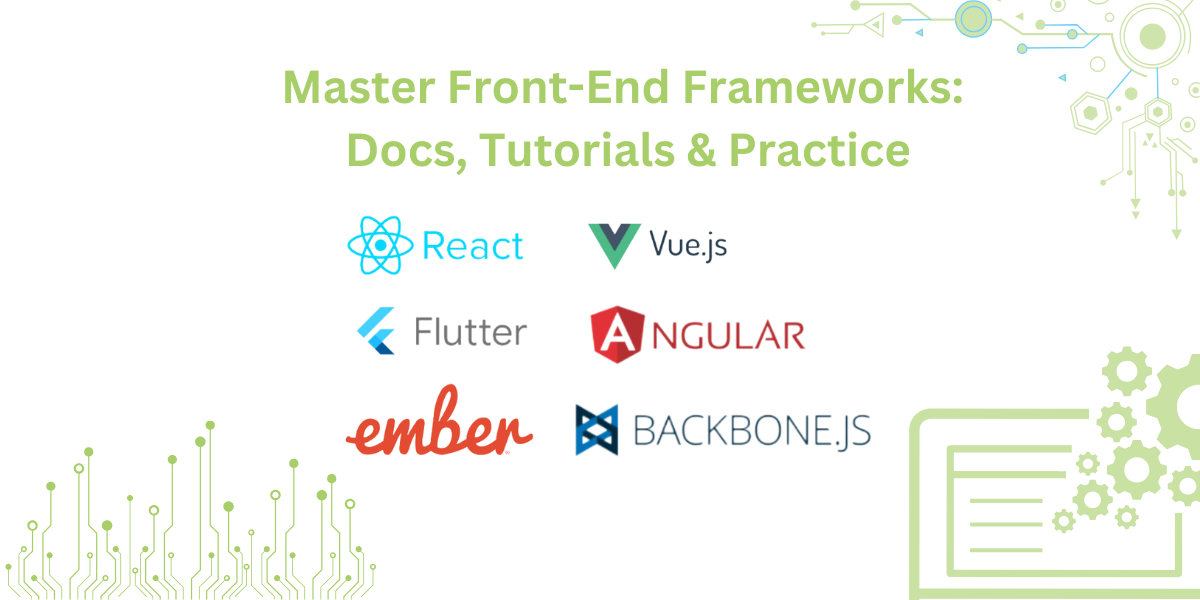
The latest evolution of the web, "The Web 2.0," is not possible without frontend frameworks. Ready-made components and features are available in website and application builders. This makes it easier to design and develop websites and applications. Prioritizing user experience…
Subscribe to our newsletter
Be the first to learn about new Sencha resources and tips.