JavaScript Design Patterns: A Hands-On Guide with Real-world Examples
As a web developer, you know how popular JavaScript is in the web app development landscape. It powers thousands of web applications, from simple to complex, adding interactivity and enhancing the user experience. While JavaScript helps create high-performance, functional apps, developers must implement best practices for writing cleaner, maintainable and organized codebases. This makes it easy to maintain and scale your app and add new features as it grows in size and scope. This is where JavaScript design patterns and JS frameworks come in.
JavaScript design patterns are essentially reusable code sequences that help developers tackle commonly occurring or repeated problems in JavaScript web development. Thus, they speed up the web development process. A modern JavaScript framework like Ext JS or React facilitates the implementation of design patterns. Thus making the web development process even quicker and more efficient.
Understanding Design Patterns
As aforementioned, JavaScript design patterns are reusable code blocks or sequences that solve common web development problems and challenges. Thus, developers don’t have to write repeatable code for these problems from scratch. This makes web app development quicker and more efficient.
Importance of Design Patterns in Web Development
Here are the key reasons why implementing design patterns is imperative:
Code Reusability
Design patterns provide reusable solutions to frequently occurring problems. Thus, developers don’t have to reinvent the wheel. Instead, they can use reusable code blocks to solve common challenges and issues. This accelerates the web development process.
Moreover, since these patterns provide a standard way of solving issues, they are designed to ensure that the architecture of the app remains reusable and flexible.
Tried and Tested
Design patterns provide a tried-and-tested solution to your issues (common web development problems). Thus, you don’t have to look for alternate solutions, saving time and effort.
Cleaner and Maintainable Code
Design patterns enable developers to write cleaner, more maintainable code that others can understand. By implementing standard patterns, you make your code predictable. This is particularly beneficial for large, complex applications, such as enterprise-grade apps, where various developers collaborate on one project.
Using design patterns also makes fixing bugs and maintaining your app easier, even when it grows in complexity.
Scalability
Design patterns promote modular and loosely coupled code. This modular approach makes it easy to scale your apps and add new features as they grow in size and scope.
Collaboration
Design patterns provide a common approach and vocabulary that most developers understand. Thus, they facilitate collaboration among developers.
Types of Design Patterns
There are a total of 23 fundamental design patterns, also known as the Gang of Four (GoF). These patterns serve as the foundation for all other patterns. These 23 patterns are typically divided into the following main categories:
- Creational: Helps solve issues related to creating and managing new objects in JS.
- Structural: Designed to solve problems related to handling the schema/structure of JavaScript objects.
- Behavioral: Helps tackle problems related to passing the control and responsibility between various objects in JavaScript.
Creational Design Patterns
Creational design patterns help deal with problems related to object creation mechanisms in JavaScript. Adopting a basic approach to creating and managing object instances can add complexity to the design, causing design problems. Creational design patterns help solve this challenge. For instance, we can utilize it to ensure that a class has only one object instance. Or we can use it for complex tasks, such as defining an interface for creating objects but enabling subclasses to alter the type of created objects.
Commonly used creational design patterns include:
- Singleton
- Factory
- Abstract Factory
- Builder
Implementation examples of creational design patterns
Singleton
We can use Singleton Creational patterns to ensure that a class has only one instance throughout the application:
// Singleton Pattern example
class Logger {
constructor() {
if (!Logger.instance) {
this.logs = [];
Logger.instance = this;
}
return Logger.instance;
}
log(message) {
this.logs.push(message);
console.log(`[Logger] ${message}`);
}
}
// Usage
const logger1 = new Logger();
const logger2 = new Logger();
console.log(logger1 === logger2); // true, same instance
logger1.log('Message 1');
logger2.log('Message 2');
Factory Method
As a developer, you know sometimes we need to create objects that involve complex initialization logic or create objects based on certain conditions. Factory creation patterns offer a flexible way to manage the complexity of object creation:
// Factory Method Pattern example
class ShapeFactory {
createShape(type) {
switch (type) {
case 'circle':
return new Circle();
case 'square':
return new Square();
default:
throw new Error('Invalid shape type');
}
}
}
// Usage
const factory = new ShapeFactory();
const circle = factory.createShape('circle');
const square = factory.createShape('square');
In the above code, the Factory method pattern encapsulates the object creation logic within a single class (ShapeFactory). This makes it easier to manage and maintain the code. It also promotes loose coupling between the client code and the specific classes being instantiated. This is because the client code interacts only with the factory class. It doesn’t need to know the details of how each shape is created.
Structural Design Patterns
These patterns are designed to deal with issues related to object composition and relationships. They essentially deal with managing the schema or structure of JS objects. For example, we can implement structural design patterns to:
- Integrate a library or API with a different interface from what your app is designed to handle.
- Abstract away certain features of an object for particular users using the Proxy structural pattern.
- Dynamically add functionality to objects without changing their interface using the Decorator pattern.
Commonly used structural design patterns include:
- Adapter
- Decorator
- Composite
- Facade
- Proxy
Implementation examples of structural design patterns
Adapter
Here is an example of how we can use the Adapter structural pattern to integrate a legacy/old API into a modern app (JavaScript code):
class LegacyAPI {
request(data) {
// Legacy API request logic
}
}
class NewAPIAdapter {
constructor() {
this.legacyAPI = new LegacyAPI();
}
makeRequest(data) {
// Adapt legacy API to match new API interface
const adaptedData = adaptData(data);
return this.legacyAPI.request(adaptedData);
}
}
const adapter = new NewAPIAdapter();
adapter.makeRequest(newData);
Decorator
We can use the Decorator pattern to extend the behavior of a UI component with additional features:
class UIComponent {
render() {
return 'Base UI component';
}
}
class Decorator {
constructor(component) {
this.component = component;
}
render() {
return this.component.render();
}
}
class ExtendedDecorator extends Decorator {
render() {
return super.render() + ', extended functionality';
}
}
const component = new UIComponent();
const decoratedComponent = new ExtendedDecorator(component);
decoratedComponent.render(); // Output: 'Base UI component, extended functionality'
Behavioral Design Patterns
These patterns deal with solving problems related to communication and synchronization of information between different or disparate objects. They essentially focus on passing control and responsibility between different objects. For instance, behavioral design patterns can be used for:
- Establishing a dependency relationship between objects. This way, when the state of one object changes, all the dependents will be updated automatically.
- Establishing a single entity to control access to various types of objects.
Commonly used behavioral design patterns include:
- Observer
- Strategy
- Command
- Iterator
Implementation examples of behavioral design patterns
Observer
We can use the Observer pattern to implement event handling in a UI where multiple components need to react to changes in a shared data model:
class Subject {
constructor() {
this.observers = [];
}
addObserver(observer) {
this.observers.push(observer);
}
notifyObservers() {
this.observers.forEach(observer => observer.update());
}
}
class Observer {
constructor() {}
update() {
console.log('Observer notified');
}
}
const subject = new Subject();
const observer1 = new Observer();
const observer2 = new Observer();
subject.addObserver(observer1);
subject.addObserver(observer2);
subject.notifyObservers(); // Output: 'Observer notified' (twice)
Strategy
Strategy pattern is a great way to implement different payment methods, such as credit cards and PayPal, in an e-commerce app:
class PaymentStrategy {
pay(amount) {
throw new Error('Method not implemented');
}
}
class CreditCardPayment extends PaymentStrategy {
pay(amount) {
console.log(`Paid ${amount} via Credit Card`);
}
}
class PayPalPayment extends PaymentStrategy {
pay(amount) {
console.log(`Paid ${amount} via PayPal`);
}
}
class PaymentProcessor {
constructor(strategy) {
this.strategy = strategy;
}
processPayment(amount) {
this.strategy.pay(amount);
}
}
const creditCardPayment = new CreditCardPayment();
const paymentProcessor1 = new PaymentProcessor(creditCardPayment);
paymentProcessor1.processPayment(120); // Output: 'Paid 120 through Credit Card'
const paypalPayment = new PayPalPayment();
const paymentprocessor2 = new PaymentProcessor(paypalPayment);
paymentProcessor2.processPayment(70);
In the above example, we’ve used the Strategy pattern to separate the ProcessingPayment class (client code) from the payment strategies/options implementation. The code also allows us to easily add new payment methods (when needed) without making changes to the existing client code.
Real-world Applications
Numerous real-world applications implement different types of design patterns using the most popular JavaScript frameworks like Ext JS and React. These JS frameworks make it easier to implement design patterns, allowing developers to write cleaner and maintainable code.
Here is how we can use a JavaScript library or JavaScript framework like Ext JS to implement design patterns easily:
Ext JS JavaScript Framework
Ext JS is a leading JS framework that enables developers to build high-performance enterprise-grade web and mobile apps. The framework offers a diverse range of high-performance UI components and tools. These tools and components enable developers to build responsive and highly interactive user interfaces with ease. It also facilitates the development of cross-platform and cross-browser apps. Additionally, Ext JS has comprehensive documentation with various coding examples.
Implementing Design Patterns With Ext JS
Ext JS offers various options and approaches to implement different design patterns:
- Ext JS supports component-based architecture. It provides over 140 pre-built and fully tested UI components, including a high-performance and optimized JS grid. Developers can customize these components as needed. Moreover, they can be reused across projects. These web components are also designed to ensure readability, consistency, and maintainability. Thus, developers can utilize these components to implement design patterns directly within their app’s UI.
- Ext JS has built-in components and classes that implement common Structural patterns, such as Composite and Decorator. For example, Ext JS containers (Ext.container.Container) utilize the Composite pattern. Containers can contain other components as well as other containers. This forms a hierarchical structure. This enables developers to build complex UIs from simple components.
- Ext JS supports the MVVM (Model-View-ViewModel) architecture. The MVVM architecture enables us to clearly separate or decouple the app’s business logic from its UI controls. When implementing components in the MVVM architecture, developers often use specific design patterns. They can be used to solve problems related to data management, presentation, and interaction. For example, developers can implement the Observer pattern with event handling mechanisms to establish communication between components.
- Ext JS allows developers to nest components of an app within each other. Thus, developers can create composite structures where individual components behave as a single unit. For example, we can create complex forms with nested fieldsets and panels, each containing various input fields and buttons.
- Ext JS supports component customization through plugins and extensions. This enables developers to extend component functionality dynamically.
Best Practices and Pitfalls
Best Practices
- Identify the parts and sections of your codebase that could benefit from improved flexibility, readability, and maintainability.
- Understand the problem or issue you’re trying to solve and choose a design pattern accordingly.
- Apply design patterns where they are sure to improve code readability and maintainability. Using design patterns excessively can make code unnecessarily complex.
- Follow fundamental design principles, such as SOLID. This ensures your code remains flexible, maintainable, and easy to understand.
- Document the purpose of using a design pattern and what problem it helps solve.
Pitfalls
- Overusing complex design patterns unnecessarily. This makes the codebase complex and harder to understand and maintain.
- Choosing the wrong design pattern for a specific problem.
- Using design patterns without a solid understanding of their implementation.
Testing Design Patterns
- Divide your codebase into testable units, such as functions, classes, or modules.
- Use mocks, stubs, or fakes to separate the components under test from their dependencies.
- Focus on testing the behavior of your code.
- Make sure to write tests that cover all situations. These include edge cases, boundary conditions, and error scenarios.
Also Read: JS Frameworks Trends and Technologies in 2024 | Future-Proofing Web Development
Conclusion
JavaScript design patterns provide a structured and streamlined way to tackle commonly occurring problems in web development. They enable developers to write cleaner, maintainable, and scalable code. This makes it easier to add new features to the app when it grows. Common design patterns include Creational, Structural, and Behavioral.
FAQs
What are design patterns in JavaScript, and why are they important?
Design patterns in JavaScript provide reusable code that helps solve common problems developers face during web development. They allow us to write cleaner, maintainable, and scalable code.
How can I test JavaScript code that utilizes design patterns?
You can utilize unit testing and integration testing and techniques like mocks, stubs, or fakes to separate the components under test from their dependencies.
How can I refactor existing JavaScript codebases to incorporate design patterns?
First, identify the areas of your codebase that need improved flexibility, readability, and maintainability. Then, choose a design pattern for each problem accordingly.
What are the best JavaScript frameworks in 2024?
Best JS libraries and frameworks include React and Ext JS for creating interactive user interfaces. React is an open-source JavaScript framework or JS library. It is known for its reusable components, Virtual DOM/ Virtual Document Object Model, and excellent community support. Ext JS is a robust JS framework for creating enterprise-level web apps. Its prominent features include 140+ pre-built and fully customizable components, two-way data binding, MVVM architecture, and a robust layout system.
The grid components have been rewritten from the ground up for Ext JS 4 and…
Although most modern mobile devices have good hardware specs, almost all of them are still…
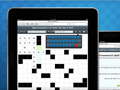
We've been interviewing the developers that created the amazing apps for our most recent Sencha…